Channel State Information Reference Signals (CSI-RS) Configuration
This notebook shows the CSI-RS configuration examples for all 18 rows of 38.211 Table 7.4.1.5.3-1
[1]:
import numpy as np
import scipy.io
from neoradium import Carrier, PDSCH, Grid, CsiRsConfig, CsiRsSet
[2]:
carrier = Carrier(startRb=0, numRbs=24, spacing=15)
bwp = carrier.bwps[0]
bwp.print()
Bandwidth Part Properties:
Resource Blocks: 24 RBs starting at 0 (288 subcarriers)
Subcarrier Spacing: 15 KHz
CP Type: normal
bandwidth: 4.32 MHz
symbolsPerSlot: 14
slotsPerSubFrame: 1
nFFT: 2048
frameNo: 0
slotNo: 0
[3]:
# Example for 1st row of 38.211 Table 7.4.1.5.3-1
# 1 port, 1 of 12 bits set in freqMap (only first 4 bits are valid), CDM size is 1, and density is 3.
# The CSI-RS resources are at symbol 1 and REs 3, 7, and 11.
csiRsConfig = CsiRsConfig(csiType="NZP", bwp=bwp, symbols=[1], numPorts=1, freqMap="000000001000", density=3)
csiRsConfig.print()
grid = bwp.createGrid(csiRsConfig.numPorts)
csiRsConfig.populateGrid(grid)
print("Grid Stats:")
stats = grid.getStats()
for key, value in stats.items(): print(" %s: %d"%(key, value))
grid.drawMap(ports=range(csiRsConfig.numPorts), reRange=(0,36))
CSI-RS Configuration: (1 Resource Sets)
CSI-RS Resource Set:(1 NZP Resources)
Resource Set ID: 0
Resource Type: periodic
Resource Blocks: 24 RBs starting at 0
Slot Period: 4
Bandwidth Part:
Resource Blocks: 24 RBs starting at 0 (288 subcarriers)
Subcarrier Spacing: 15 KHz
CP Type: normal
bandwidth: 4.32 MHz
symbolsPerSlot: 14
slotsPerSubFrame: 1
nFFT: 2048
frameNo: 0
slotNo: 0
CSI-RS:
resourceId: 0
numPorts: 1
cdmSize: 1 (noCDM)
density: 3
RE Indexes: 3
Symbol Indexes: 1
Table Row: 1
Slot Offset: 0
Power: 0 db
scramblingID: 0
Grid Stats:
GridSize: 4032
UNASSIGNED: 3960
CSIRS_NZP: 72
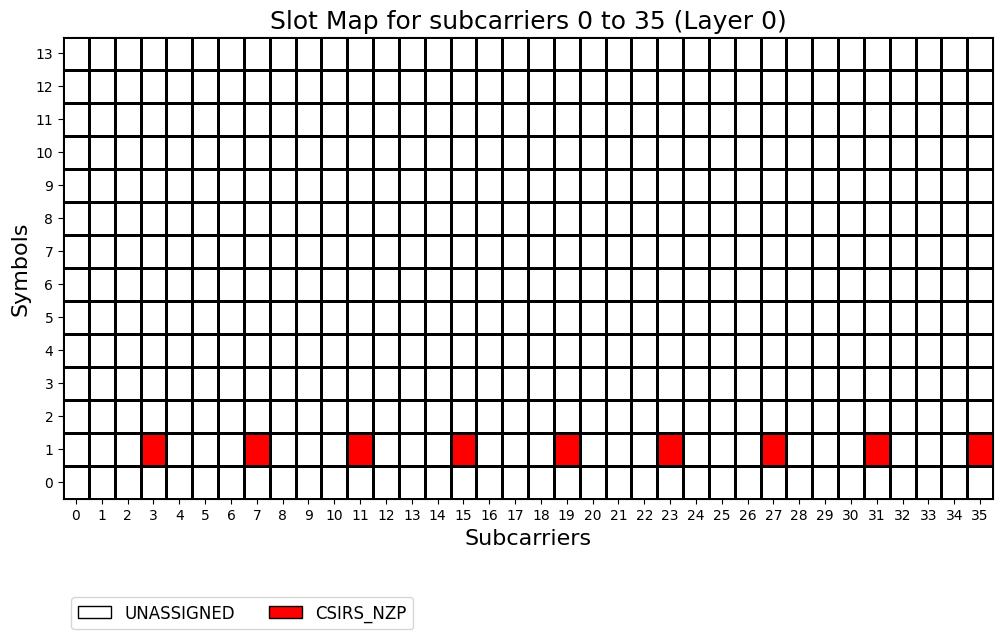
[4]:
# Example for 2nd row of 38.211 Table 7.4.1.5.3-1 with density=0.5
# 1 ports, 1 of 4 bits set in freqMap, CDM size is 1, and density is 0.5.
# The CSI-RS resources are at symbol 1 and RE 3. Every other RB is skipped.
csiRsConfig = CsiRsConfig()
csiRsConfig.addCsiRs(csiType="NZP", bwp=bwp, symbols=[1], numPorts=1, freqMap="1000", density=0.5)
csiRsConfig.print()
grid = bwp.createGrid(csiRsConfig.numPorts)
csiRsConfig.populateGrid(grid)
print("Grid Stats:")
stats = grid.getStats()
for key, value in stats.items(): print(" %s: %d"%(key, value))
grid.drawMap(ports=range(csiRsConfig.numPorts), reRange=(0,36))
CSI-RS Configuration: (1 Resource Sets)
CSI-RS Resource Set:(1 NZP Resources)
Resource Set ID: 0
Resource Type: periodic
Resource Blocks: 24 RBs starting at 0
Slot Period: 4
Bandwidth Part:
Resource Blocks: 24 RBs starting at 0 (288 subcarriers)
Subcarrier Spacing: 15 KHz
CP Type: normal
bandwidth: 4.32 MHz
symbolsPerSlot: 14
slotsPerSubFrame: 1
nFFT: 2048
frameNo: 0
slotNo: 0
CSI-RS:
resourceId: 0
numPorts: 1
cdmSize: 1 (noCDM)
density: 0.5
RE Indexes: 3
Symbol Indexes: 1
Table Row: 2
Slot Offset: 0
Power: 0 db
scramblingID: 0
Grid Stats:
GridSize: 4032
UNASSIGNED: 4020
CSIRS_NZP: 12
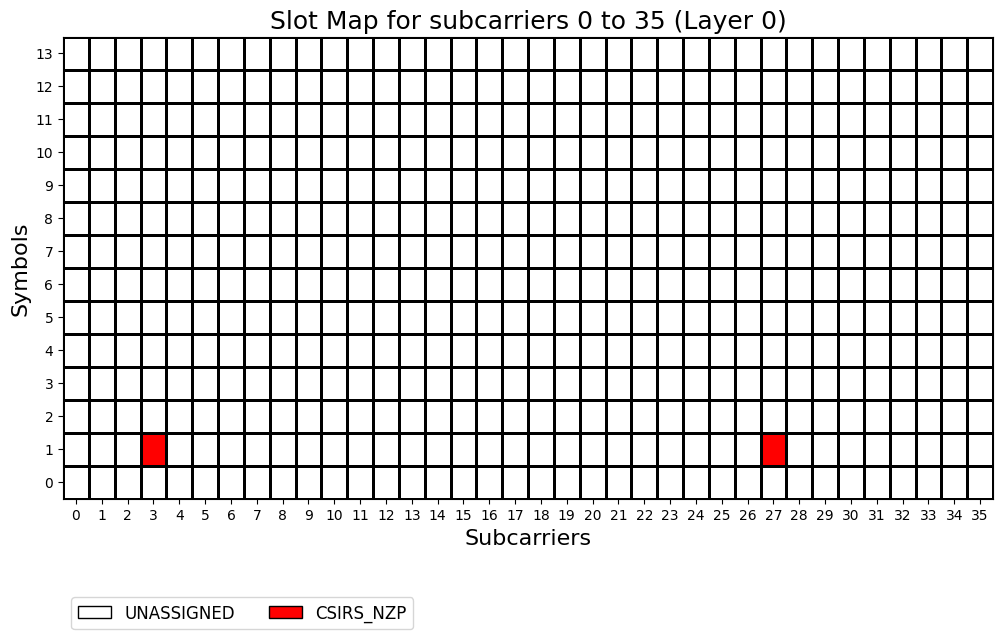
[5]:
# Example for 2nd row of 38.211 Table 7.4.1.5.3-1 with density=1 using a different symbol (3)
# 1 ports, 1 of 4 bits set in freqMap, CDM size is 1, and density is 1.
# The CSI-RS resources are at symbol 3 and RE 3.
csiRsConfig = CsiRsConfig([CsiRsSet("NZP", bwp, symbols=[3], numPorts=1, freqMap="1000", density=1)])
csiRsConfig.print()
grid = bwp.createGrid(csiRsConfig.numPorts)
csiRsConfig.populateGrid(grid)
print("Grid Stats:")
stats = grid.getStats()
for key, value in stats.items(): print(" %s: %d"%(key, value))
grid.drawMap(ports=range(csiRsConfig.numPorts), reRange=(0,36))
CSI-RS Configuration: (1 Resource Sets)
CSI-RS Resource Set:(1 NZP Resources)
Resource Set ID: 0
Resource Type: periodic
Resource Blocks: 24 RBs starting at 0
Slot Period: 4
Bandwidth Part:
Resource Blocks: 24 RBs starting at 0 (288 subcarriers)
Subcarrier Spacing: 15 KHz
CP Type: normal
bandwidth: 4.32 MHz
symbolsPerSlot: 14
slotsPerSubFrame: 1
nFFT: 2048
frameNo: 0
slotNo: 0
CSI-RS:
resourceId: 0
numPorts: 1
cdmSize: 1 (noCDM)
density: 1
RE Indexes: 3
Symbol Indexes: 3
Table Row: 2
Slot Offset: 0
Power: 0 db
scramblingID: 0
Grid Stats:
GridSize: 4032
UNASSIGNED: 4008
CSIRS_NZP: 24
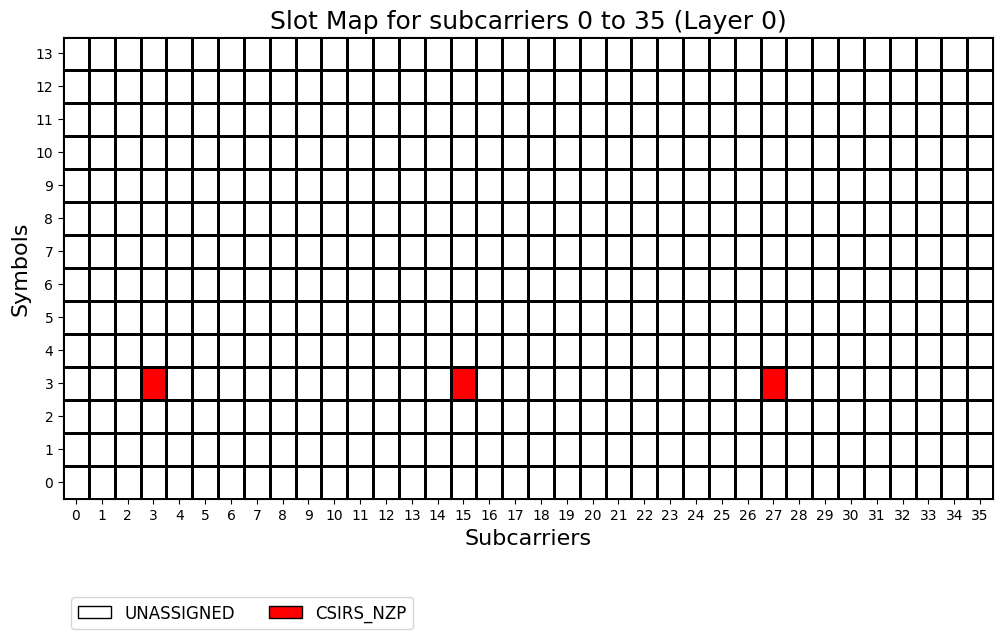
[6]:
# Example for 3rd row of 38.211 Table 7.4.1.5.3-1 with density=.5
# 2 ports, 1 of 6 bits set in freqMap, CDM size is 2, and density is 0.5.
# The CSI-RS resources are at symbol 3 and REs (0,1). Every other RB is skipped.
csiRsConfig = CsiRsConfig([CsiRsSet("NZP", bwp, symbols=[3], numPorts=2, freqMap="000001", density=0.5)])
csiRsConfig.print()
grid = bwp.createGrid(csiRsConfig.numPorts)
csiRsConfig.populateGrid(grid)
print("Grid Stats:")
stats = grid.getStats()
for key, value in stats.items(): print(" %s: %d"%(key, value))
grid.drawMap(ports=range(csiRsConfig.numPorts), reRange=(0,36))
CSI-RS Configuration: (1 Resource Sets)
CSI-RS Resource Set:(1 NZP Resources)
Resource Set ID: 0
Resource Type: periodic
Resource Blocks: 24 RBs starting at 0
Slot Period: 4
Bandwidth Part:
Resource Blocks: 24 RBs starting at 0 (288 subcarriers)
Subcarrier Spacing: 15 KHz
CP Type: normal
bandwidth: 4.32 MHz
symbolsPerSlot: 14
slotsPerSubFrame: 1
nFFT: 2048
frameNo: 0
slotNo: 0
CSI-RS:
resourceId: 0
numPorts: 2
cdmSize: 2 (fd-CDM2)
density: 0.5
RE Indexes: 0
Symbol Indexes: 3
Table Row: 3
Slot Offset: 0
Power: 0 db
scramblingID: 0
Grid Stats:
GridSize: 8064
UNASSIGNED: 8016
CSIRS_NZP: 48
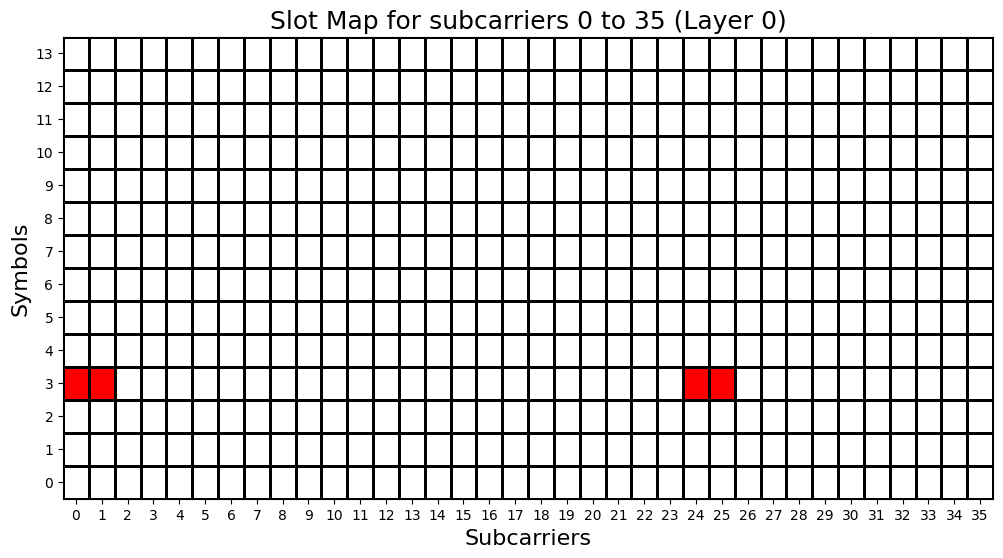
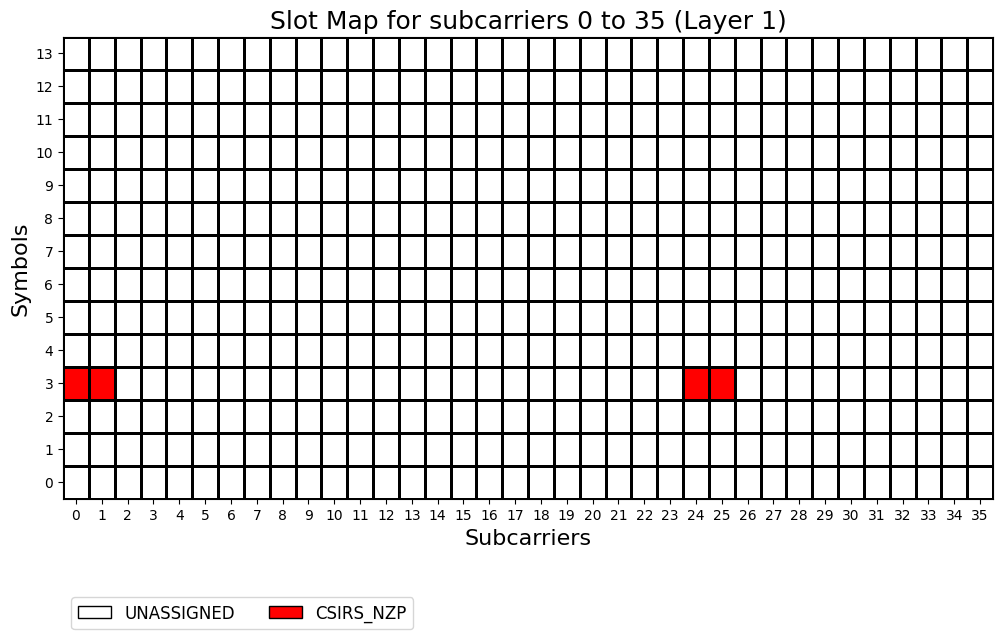
[7]:
# Example for 3rd row of 38.211 Table 7.4.1.5.3-1 with density=1 using a different symbol (6)
# 2 ports, 1 of 6 bits set in freqMap, CDM size is 2, and density is 1.
# The CSI-RS resources are at symbol 6 and REs (6,7).
csiRsConfig = CsiRsConfig([CsiRsSet("NZP", bwp, symbols=[6], numPorts=2, freqMap="001000", density=1)])
csiRsConfig.print()
grid = bwp.createGrid(csiRsConfig.numPorts)
csiRsConfig.populateGrid(grid)
print("Grid Stats:")
stats = grid.getStats()
for key, value in stats.items(): print(" %s: %d"%(key, value))
grid.drawMap(ports=range(csiRsConfig.numPorts), reRange=(0,36))
CSI-RS Configuration: (1 Resource Sets)
CSI-RS Resource Set:(1 NZP Resources)
Resource Set ID: 0
Resource Type: periodic
Resource Blocks: 24 RBs starting at 0
Slot Period: 4
Bandwidth Part:
Resource Blocks: 24 RBs starting at 0 (288 subcarriers)
Subcarrier Spacing: 15 KHz
CP Type: normal
bandwidth: 4.32 MHz
symbolsPerSlot: 14
slotsPerSubFrame: 1
nFFT: 2048
frameNo: 0
slotNo: 0
CSI-RS:
resourceId: 0
numPorts: 2
cdmSize: 2 (fd-CDM2)
density: 1
RE Indexes: 6
Symbol Indexes: 6
Table Row: 3
Slot Offset: 0
Power: 0 db
scramblingID: 0
Grid Stats:
GridSize: 8064
UNASSIGNED: 7968
CSIRS_NZP: 96
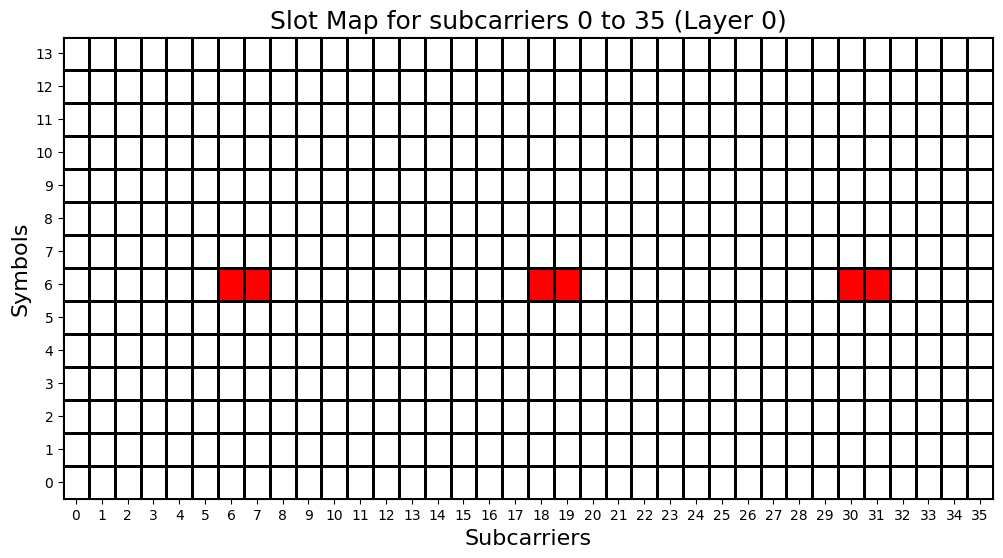
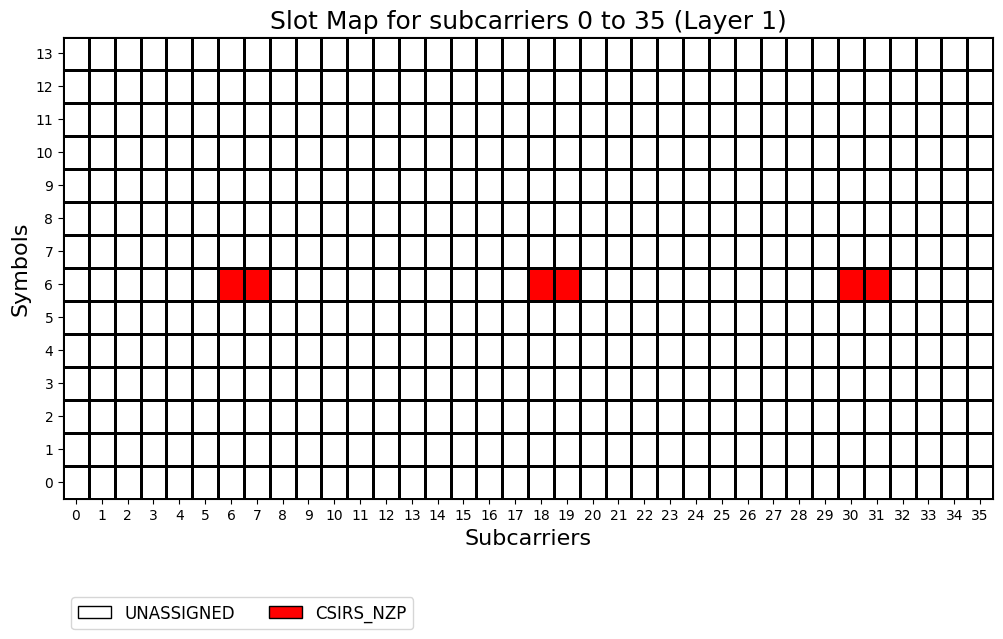
[8]:
# Example for 4th row of 38.211 Table 7.4.1.5.3-1
# 4 ports, 1 of 3 bits set in freqMap, CDM size is 2, and density is 1.
# The CSI-RS resources are at symbol 6 and REs (4,5) and (6,7).
# Note that REs (4,5) are used for the first 2 ports and REs (6,7) for the next 2.
csiRsConfig = CsiRsConfig([CsiRsSet("NZP", bwp, symbols=[6], freqMap="010", numPorts=4)])
csiRsConfig.print()
grid = bwp.createGrid(csiRsConfig.numPorts)
csiRsConfig.populateGrid(grid)
print("Grid Stats:")
stats = grid.getStats()
for key, value in stats.items(): print(" %s: %d"%(key, value))
grid.drawMap(ports=range(csiRsConfig.numPorts), reRange=(0,36))
CSI-RS Configuration: (1 Resource Sets)
CSI-RS Resource Set:(1 NZP Resources)
Resource Set ID: 0
Resource Type: periodic
Resource Blocks: 24 RBs starting at 0
Slot Period: 4
Bandwidth Part:
Resource Blocks: 24 RBs starting at 0 (288 subcarriers)
Subcarrier Spacing: 15 KHz
CP Type: normal
bandwidth: 4.32 MHz
symbolsPerSlot: 14
slotsPerSubFrame: 1
nFFT: 2048
frameNo: 0
slotNo: 0
CSI-RS:
resourceId: 0
numPorts: 4
cdmSize: 2 (fd-CDM2)
density: 1
RE Indexes: 4
Symbol Indexes: 6
Table Row: 4
Slot Offset: 0
Power: 0 db
scramblingID: 0
Grid Stats:
GridSize: 16128
UNASSIGNED: 15936
CSIRS_NZP: 192
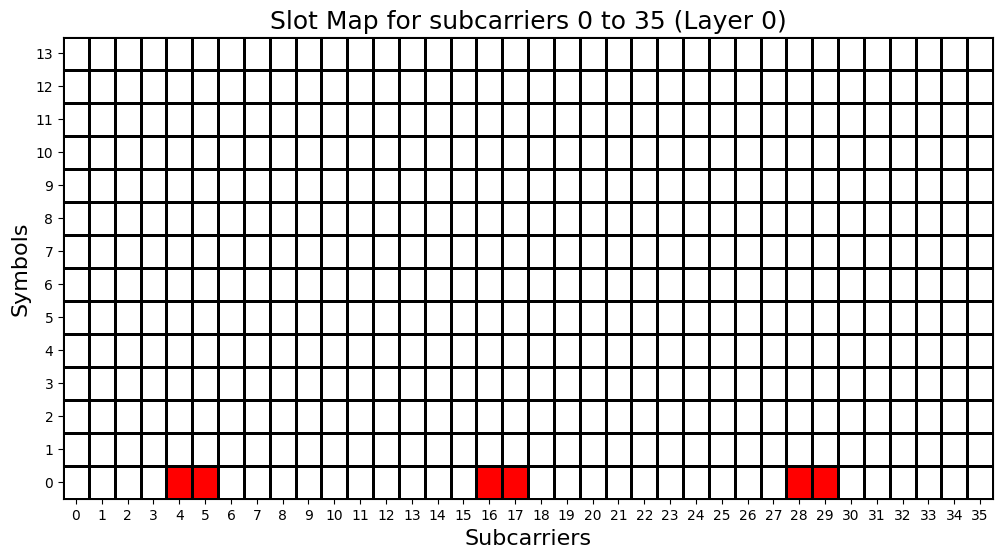
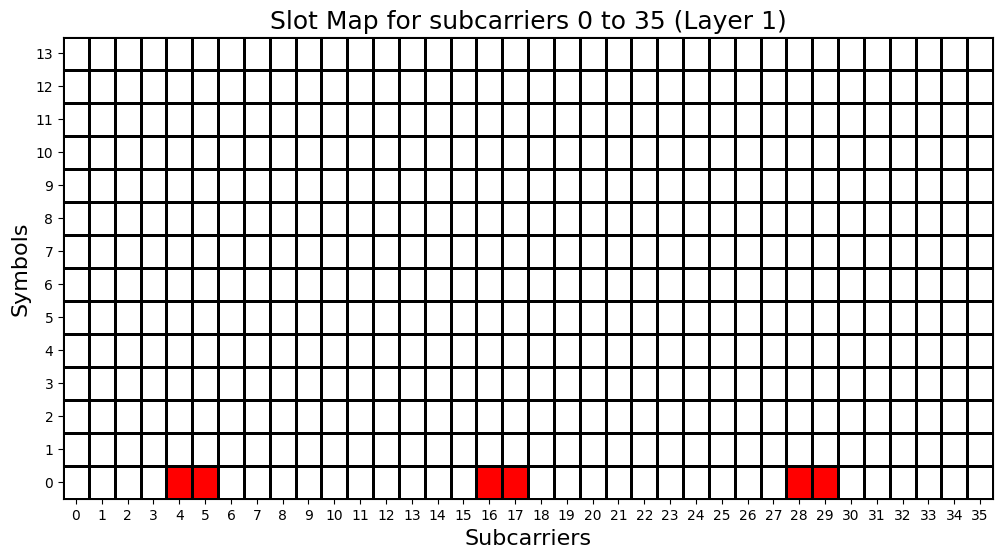
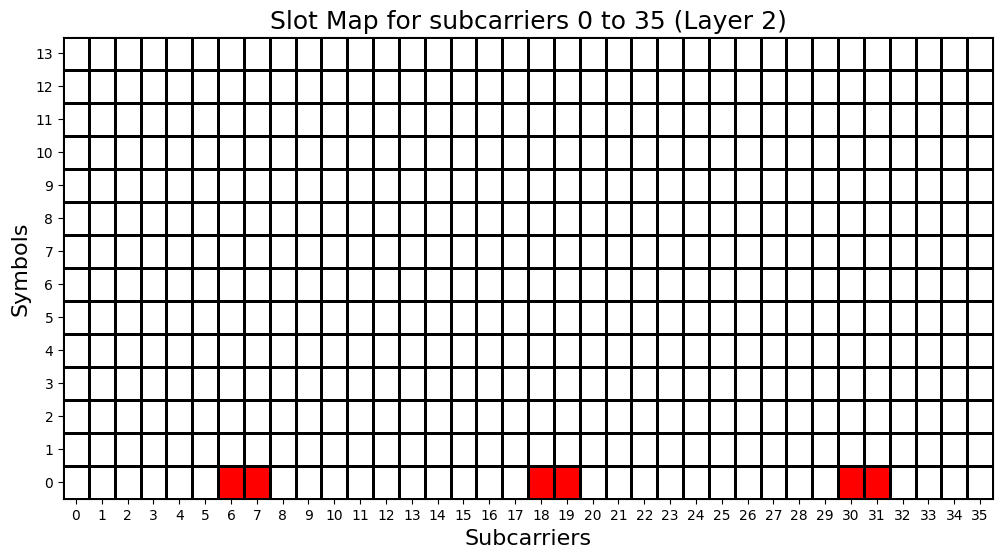
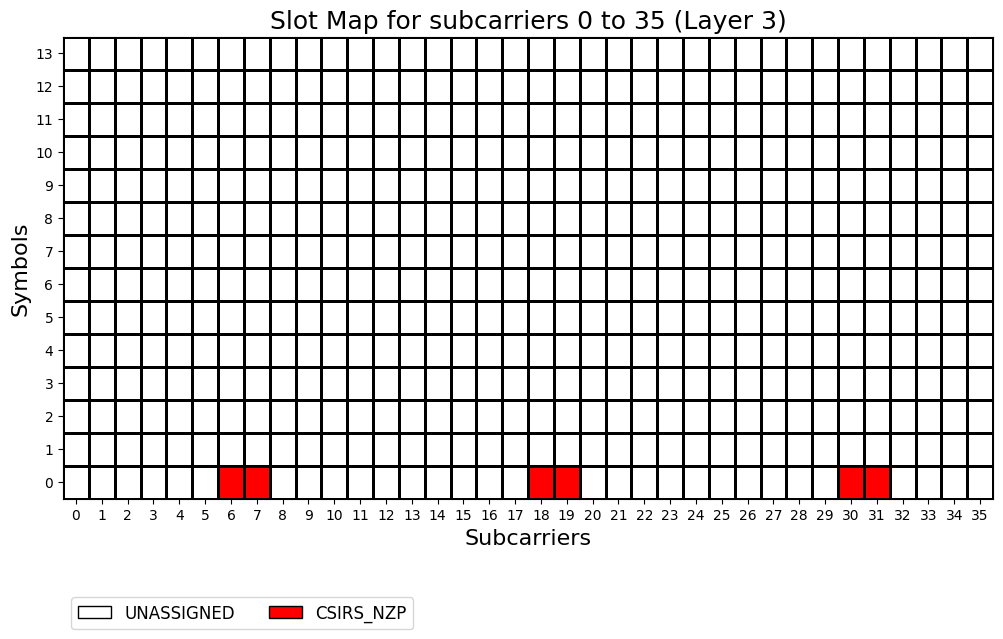
[9]:
# Example for 5th row of 38.211 Table 7.4.1.5.3-1
# 4 ports, 1 of 6 bits set in freqMap, CDM size is 2, and density is 1.
# The CSI-RS resources are at symbols 6,7 and REs (2,3).
# Note that symbol 6 is used for the first 2 ports and symbol 7 for the next 2.
csiRsConfig = CsiRsConfig([CsiRsSet("NZP", bwp, symbols=[6], numPorts=4, freqMap="000010")])
csiRsConfig.print()
grid = bwp.createGrid(csiRsConfig.numPorts)
csiRsConfig.populateGrid(grid)
print("Grid Stats:")
stats = grid.getStats()
for key, value in stats.items(): print(" %s: %d"%(key, value))
grid.drawMap(ports=range(csiRsConfig.numPorts), reRange=(0,36))
CSI-RS Configuration: (1 Resource Sets)
CSI-RS Resource Set:(1 NZP Resources)
Resource Set ID: 0
Resource Type: periodic
Resource Blocks: 24 RBs starting at 0
Slot Period: 4
Bandwidth Part:
Resource Blocks: 24 RBs starting at 0 (288 subcarriers)
Subcarrier Spacing: 15 KHz
CP Type: normal
bandwidth: 4.32 MHz
symbolsPerSlot: 14
slotsPerSubFrame: 1
nFFT: 2048
frameNo: 0
slotNo: 0
CSI-RS:
resourceId: 0
numPorts: 4
cdmSize: 2 (fd-CDM2)
density: 1
RE Indexes: 2
Symbol Indexes: 6
Table Row: 5
Slot Offset: 0
Power: 0 db
scramblingID: 0
Grid Stats:
GridSize: 16128
UNASSIGNED: 15936
CSIRS_NZP: 192
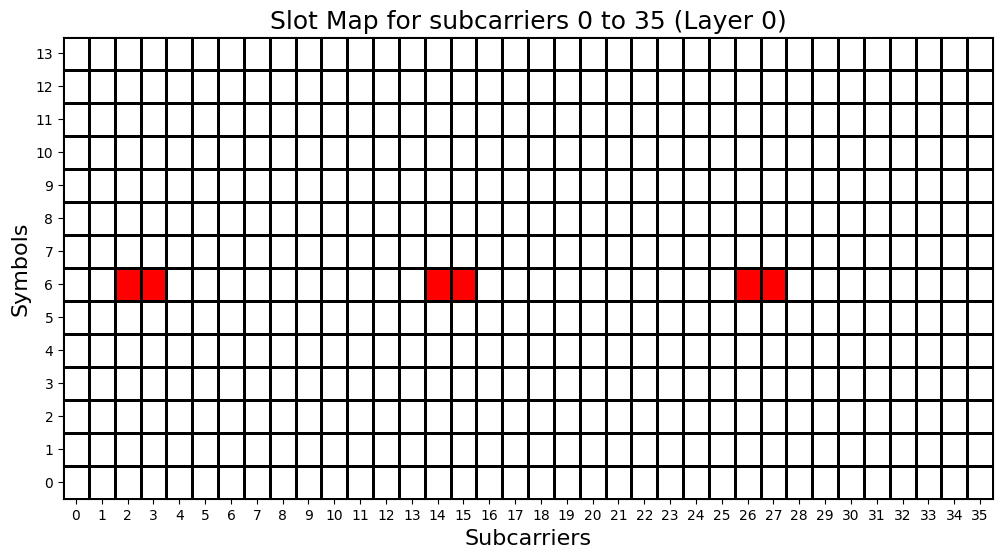
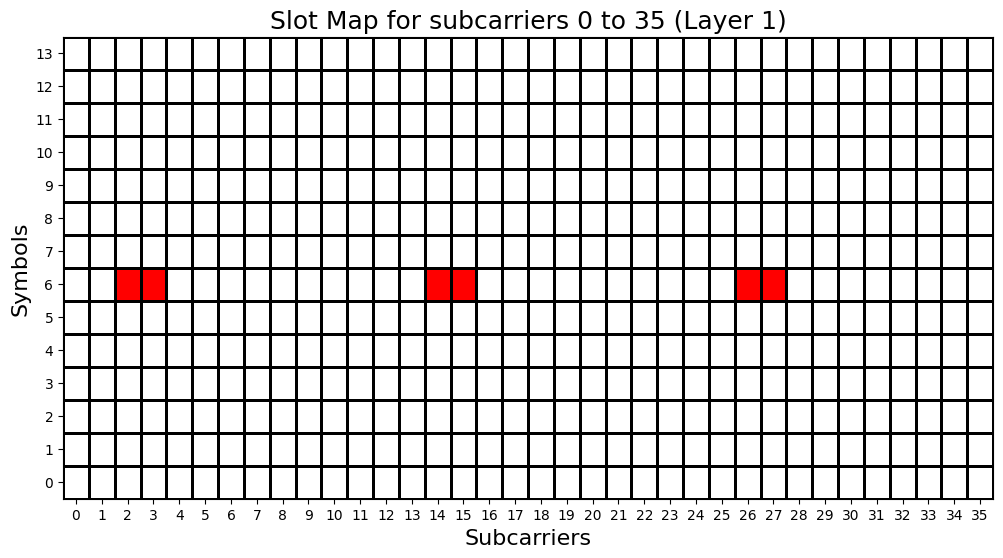
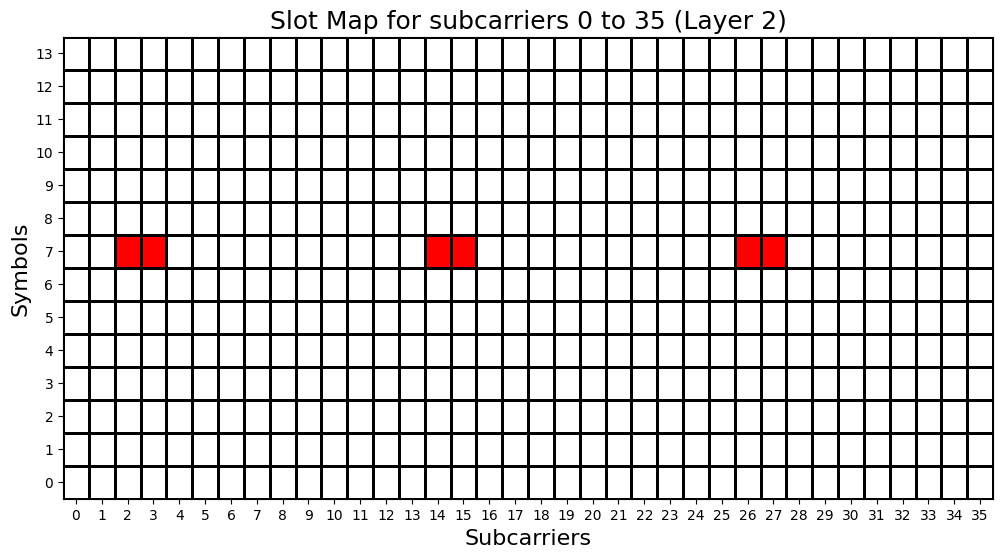
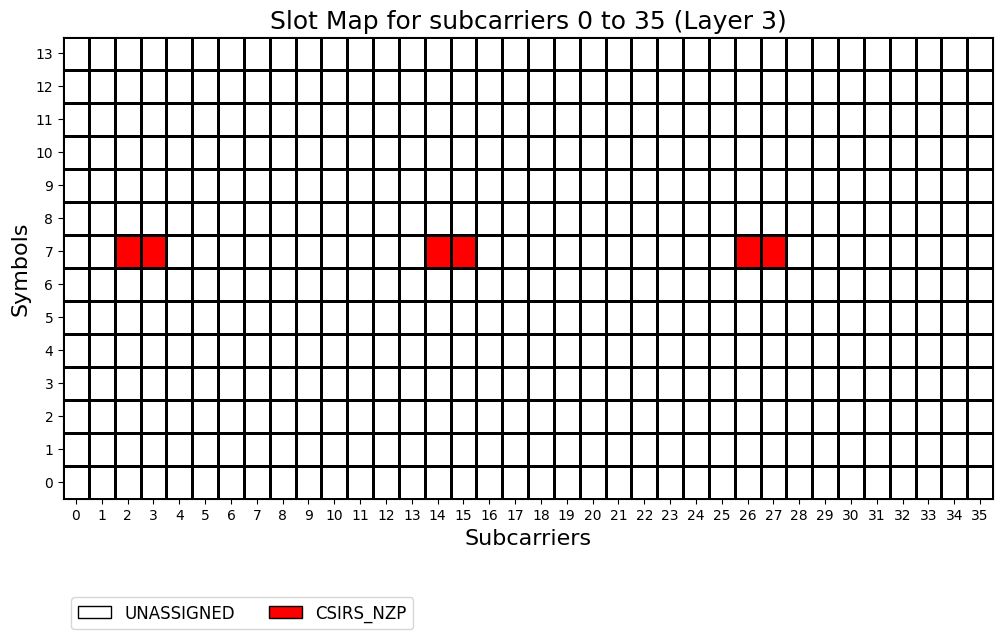
[10]:
# Example for 6th row of 38.211 Table 7.4.1.5.3-1
# 8 ports, 4 of 6 bits set in freqMap, CDM size is 2, and density is 1.
# The CSI-RS resources are at symbols 6 and REs (0,1), (4,5), (6,7), and (10,11).
csiRsConfig = CsiRsConfig([CsiRsSet("NZP", bwp, symbols=[6], numPorts=8, freqMap="101101")])
csiRsConfig.print()
grid = bwp.createGrid(csiRsConfig.numPorts)
csiRsConfig.populateGrid(grid)
print("Grid Stats:")
stats = grid.getStats()
for key, value in stats.items(): print(" %s: %d"%(key, value))
grid.drawMap(ports=range(csiRsConfig.numPorts), reRange=(0,36))
CSI-RS Configuration: (1 Resource Sets)
CSI-RS Resource Set:(1 NZP Resources)
Resource Set ID: 0
Resource Type: periodic
Resource Blocks: 24 RBs starting at 0
Slot Period: 4
Bandwidth Part:
Resource Blocks: 24 RBs starting at 0 (288 subcarriers)
Subcarrier Spacing: 15 KHz
CP Type: normal
bandwidth: 4.32 MHz
symbolsPerSlot: 14
slotsPerSubFrame: 1
nFFT: 2048
frameNo: 0
slotNo: 0
CSI-RS:
resourceId: 0
numPorts: 8
cdmSize: 2 (fd-CDM2)
density: 1
RE Indexes: 0 4 6 10
Symbol Indexes: 6
Table Row: 6
Slot Offset: 0
Power: 0 db
scramblingID: 0
Grid Stats:
GridSize: 32256
UNASSIGNED: 31872
CSIRS_NZP: 384
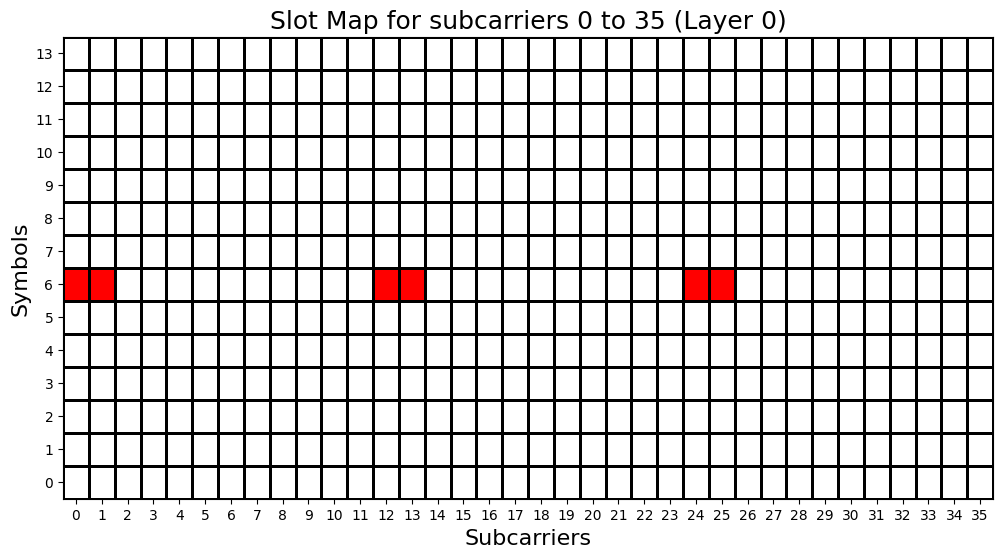
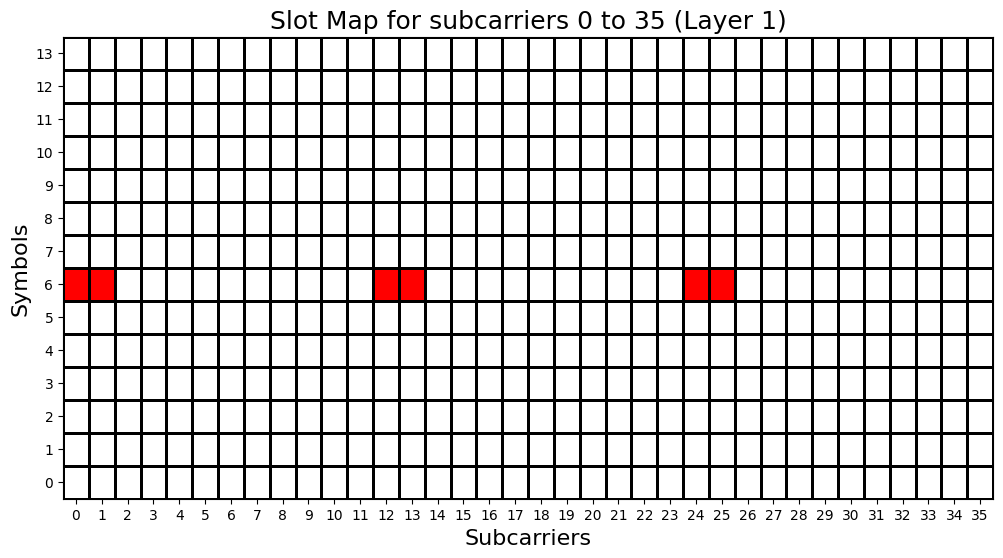
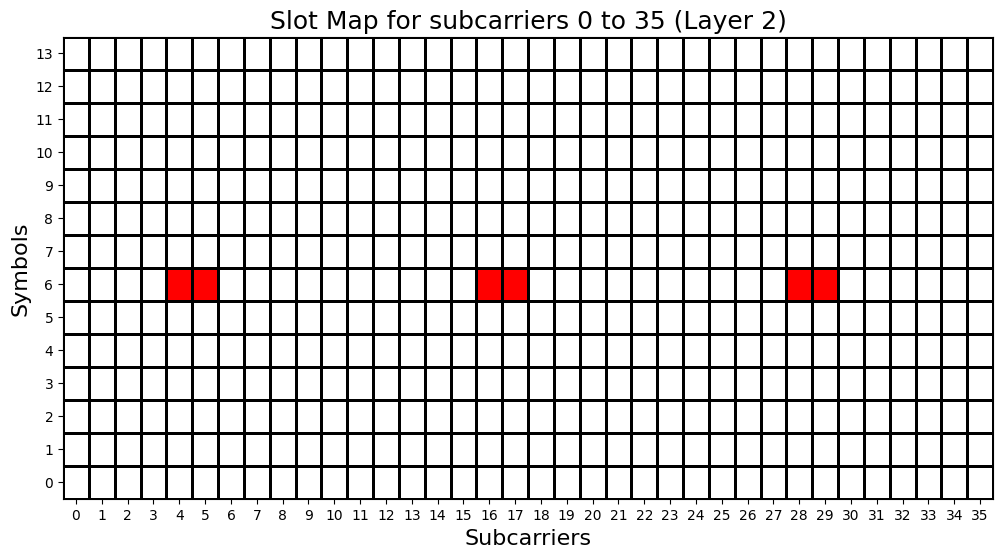
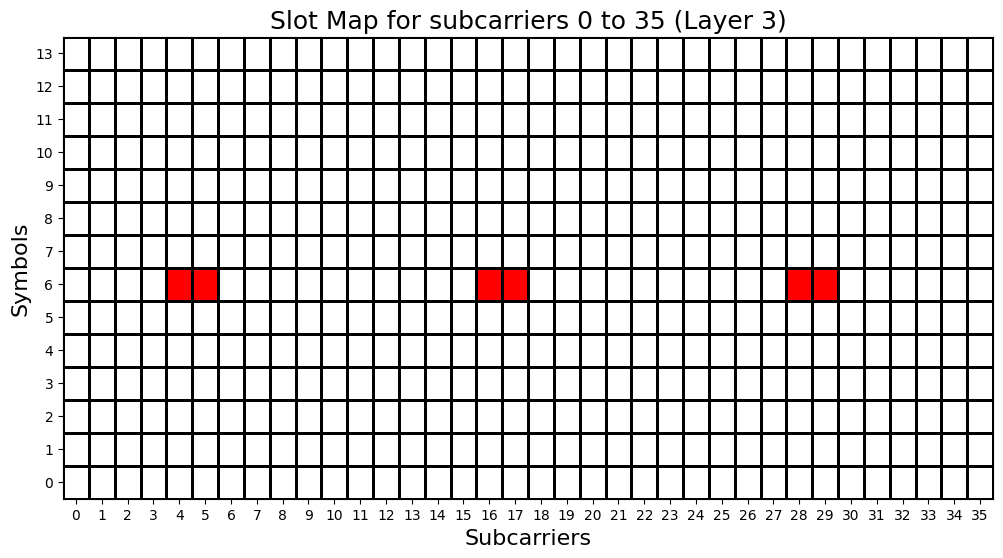
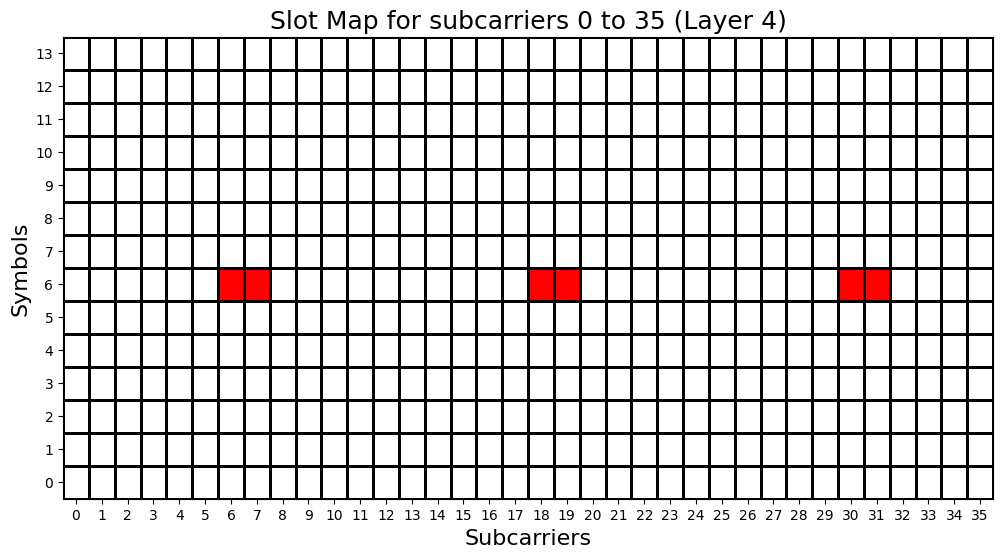
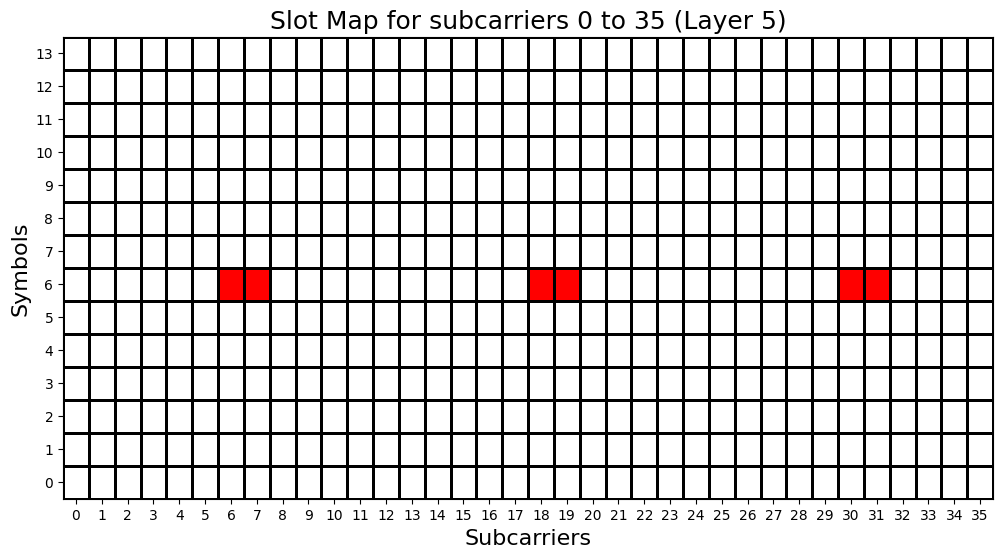
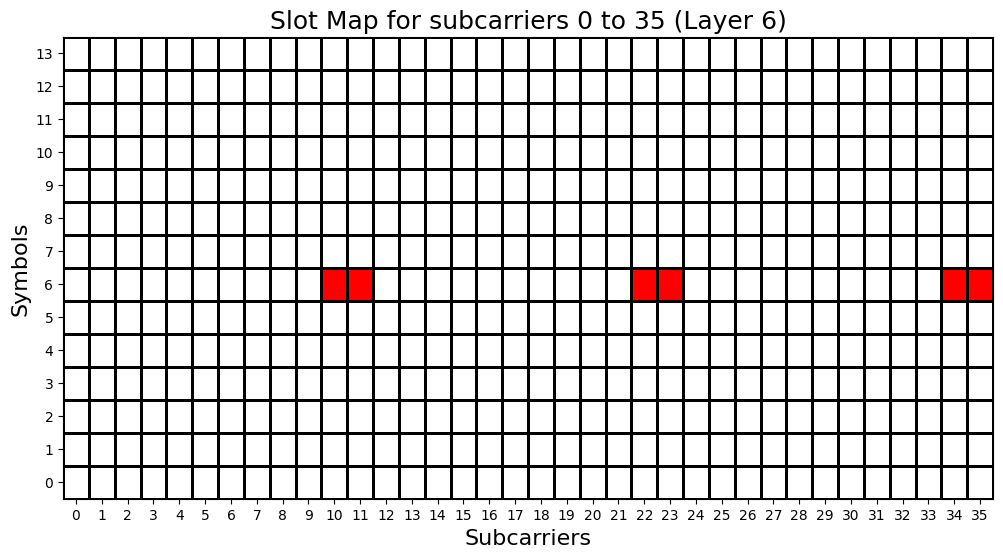
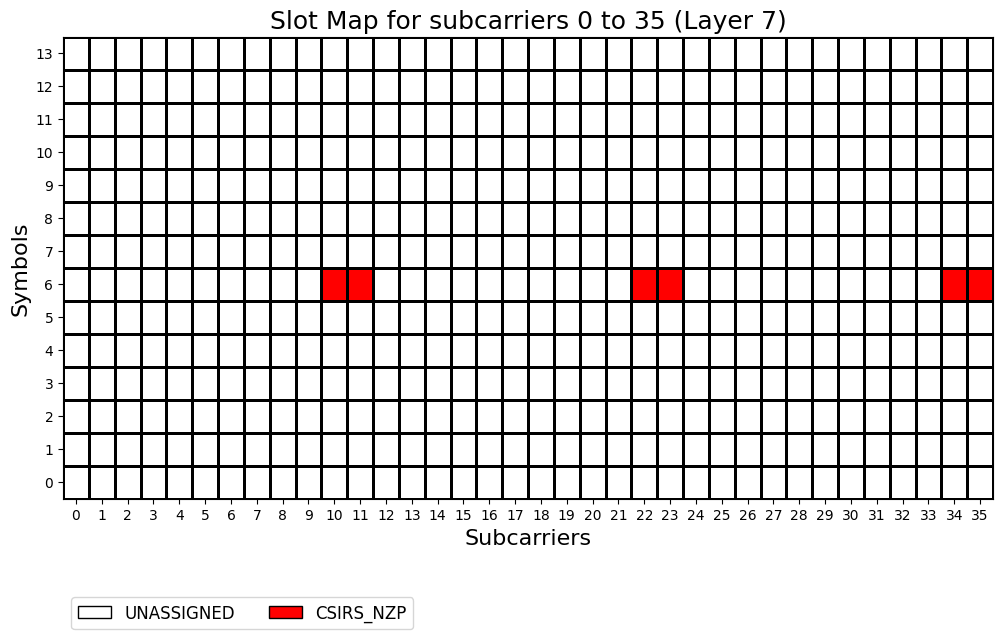
[11]:
# Example for 7th row of 38.211 Table 7.4.1.5.3-1
# 8 ports, 2 of 6 bits set in freqMap, CDM size is 2, and density is 1.
# The CSI-RS resources are at symbols 6,7 and REs (2,3), and (8,9).
csiRsConfig = CsiRsConfig([CsiRsSet("NZP", bwp, symbols=[6], numPorts=8, freqMap="010010")])
csiRsConfig.print()
grid = bwp.createGrid(csiRsConfig.numPorts)
csiRsConfig.populateGrid(grid)
print("Grid Stats:")
stats = grid.getStats()
for key, value in stats.items(): print(" %s: %d"%(key, value))
grid.drawMap(ports=range(csiRsConfig.numPorts), reRange=(0,36))
CSI-RS Configuration: (1 Resource Sets)
CSI-RS Resource Set:(1 NZP Resources)
Resource Set ID: 0
Resource Type: periodic
Resource Blocks: 24 RBs starting at 0
Slot Period: 4
Bandwidth Part:
Resource Blocks: 24 RBs starting at 0 (288 subcarriers)
Subcarrier Spacing: 15 KHz
CP Type: normal
bandwidth: 4.32 MHz
symbolsPerSlot: 14
slotsPerSubFrame: 1
nFFT: 2048
frameNo: 0
slotNo: 0
CSI-RS:
resourceId: 0
numPorts: 8
cdmSize: 2 (fd-CDM2)
density: 1
RE Indexes: 2 8
Symbol Indexes: 6
Table Row: 7
Slot Offset: 0
Power: 0 db
scramblingID: 0
Grid Stats:
GridSize: 32256
UNASSIGNED: 31872
CSIRS_NZP: 384
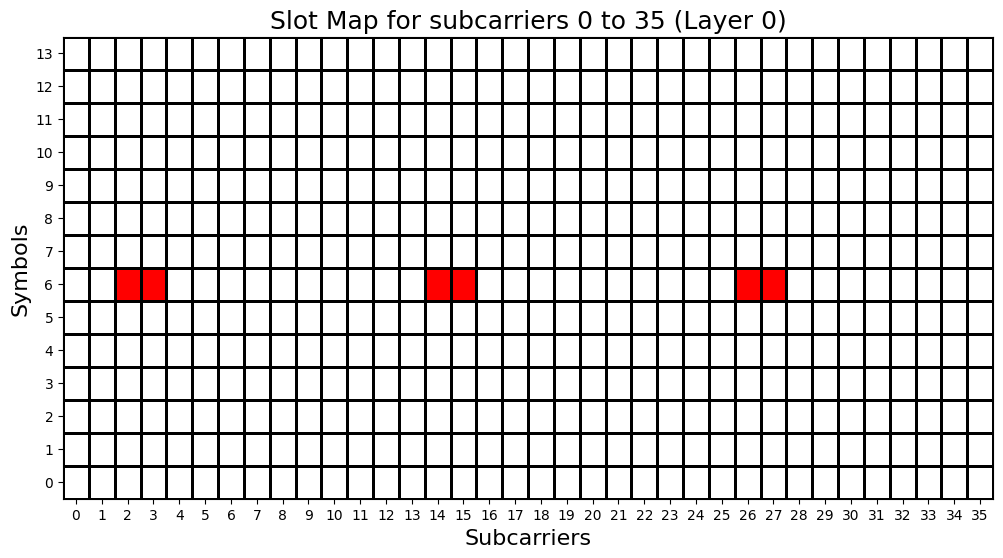
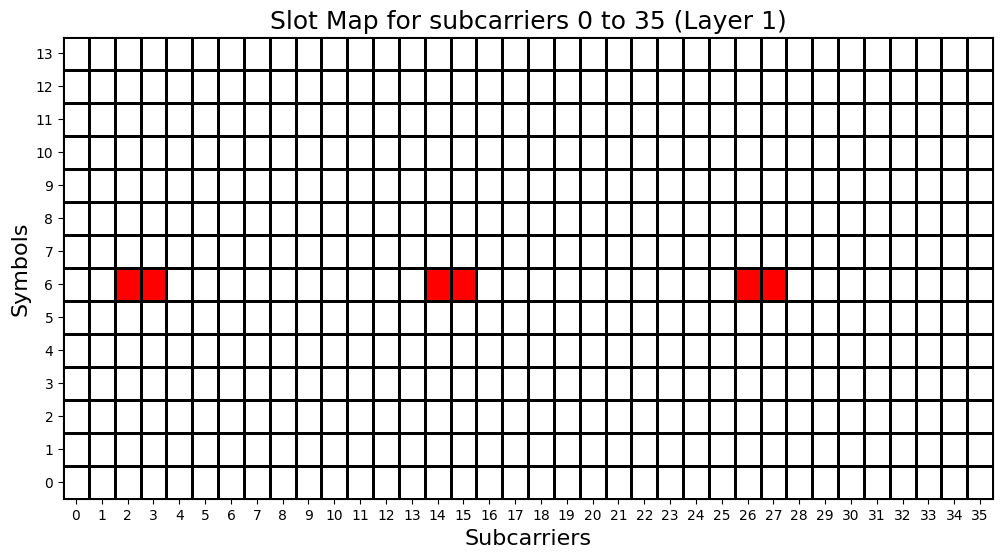
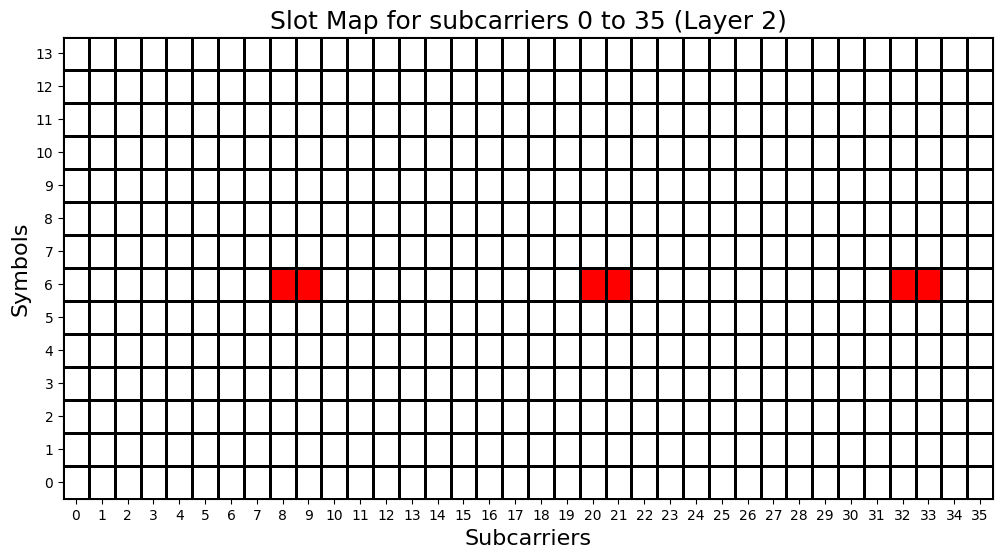
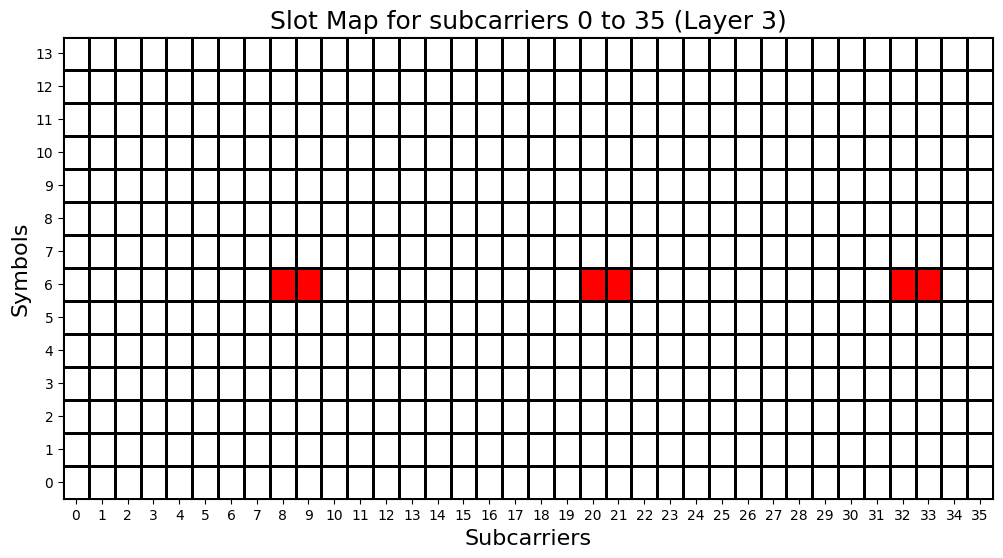
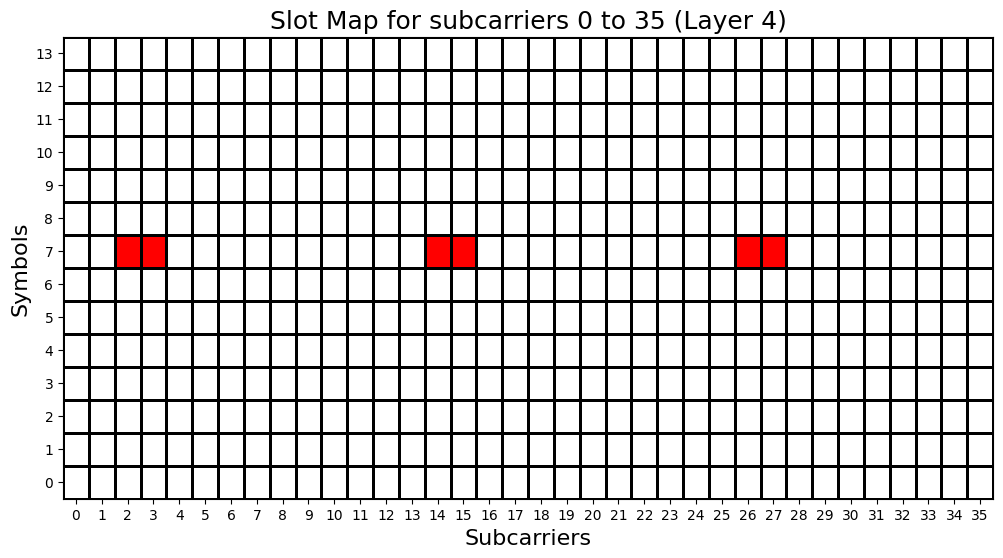
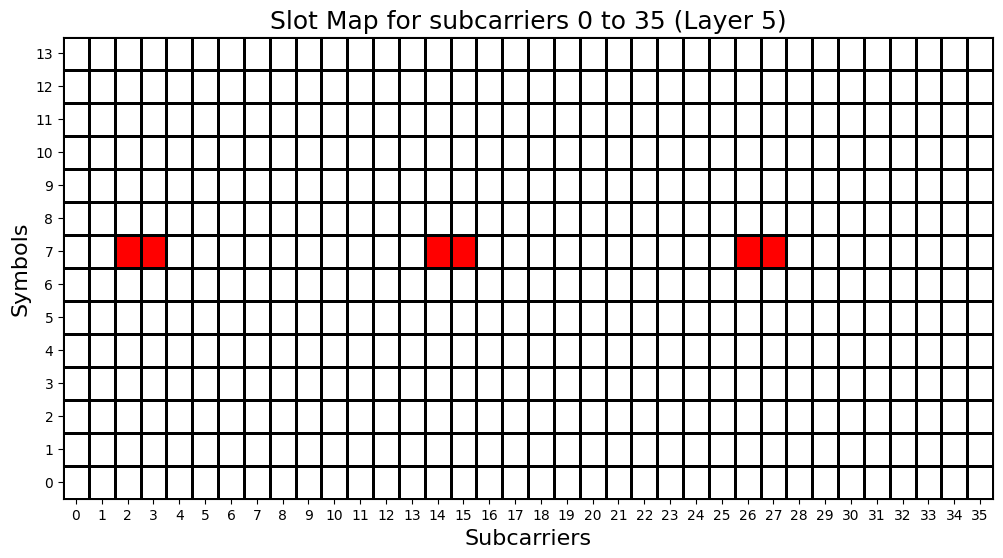
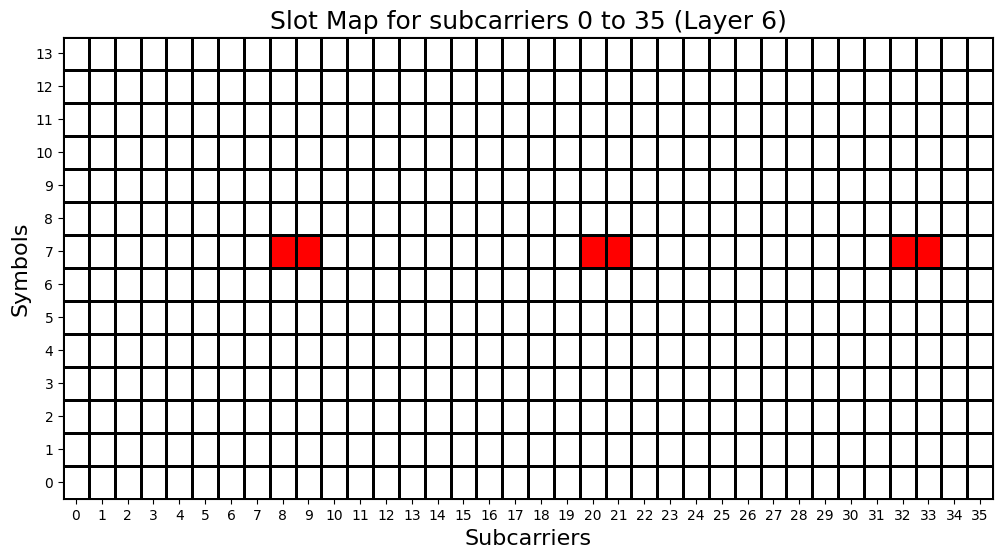
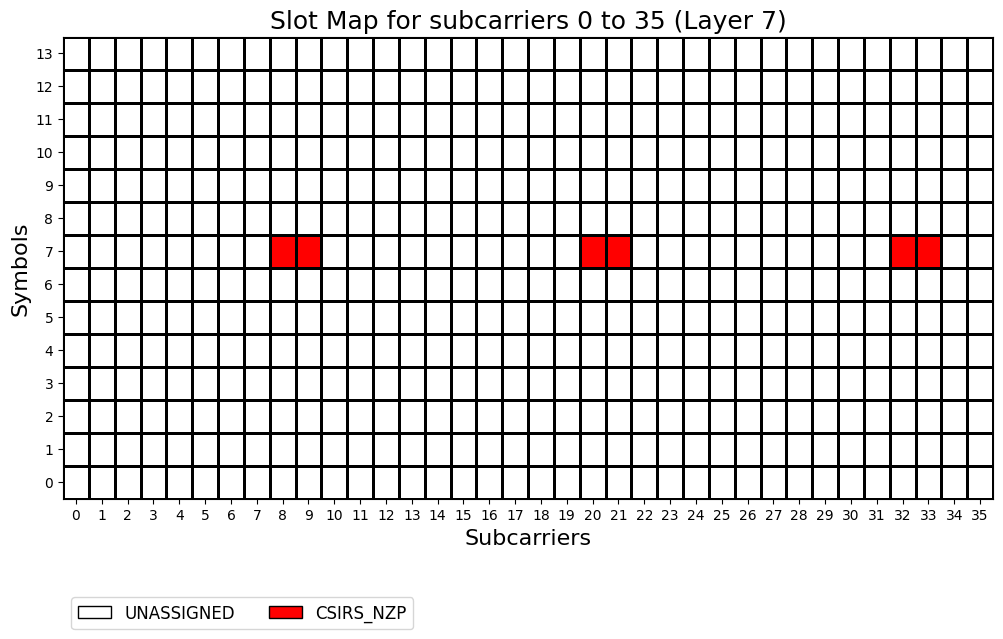
[12]:
# Example for 8th row of 38.211 Table 7.4.1.5.3-1
# 8 ports, 2 of 6 bits set in freqMap, CDM size is 4, and density is 1.
# The CSI-RS resources are at symbols (6,7) and REs (2,3), and (8,9).
csiRsConfig = CsiRsConfig([CsiRsSet("NZP", bwp, symbols=[6], numPorts=8, freqMap="010010", cdmSize=4)])
csiRsConfig.print()
grid = bwp.createGrid(csiRsConfig.numPorts)
csiRsConfig.populateGrid(grid)
print("Grid Stats:")
stats = grid.getStats()
for key, value in stats.items(): print(" %s: %d"%(key, value))
grid.drawMap(ports=range(csiRsConfig.numPorts), reRange=(0,36))
CSI-RS Configuration: (1 Resource Sets)
CSI-RS Resource Set:(1 NZP Resources)
Resource Set ID: 0
Resource Type: periodic
Resource Blocks: 24 RBs starting at 0
Slot Period: 4
Bandwidth Part:
Resource Blocks: 24 RBs starting at 0 (288 subcarriers)
Subcarrier Spacing: 15 KHz
CP Type: normal
bandwidth: 4.32 MHz
symbolsPerSlot: 14
slotsPerSubFrame: 1
nFFT: 2048
frameNo: 0
slotNo: 0
CSI-RS:
resourceId: 0
numPorts: 8
cdmSize: 4 (cdm4-FD2-TD2)
density: 1
RE Indexes: 2 8
Symbol Indexes: 6
Table Row: 8
Slot Offset: 0
Power: 0 db
scramblingID: 0
Grid Stats:
GridSize: 32256
UNASSIGNED: 31488
CSIRS_NZP: 768
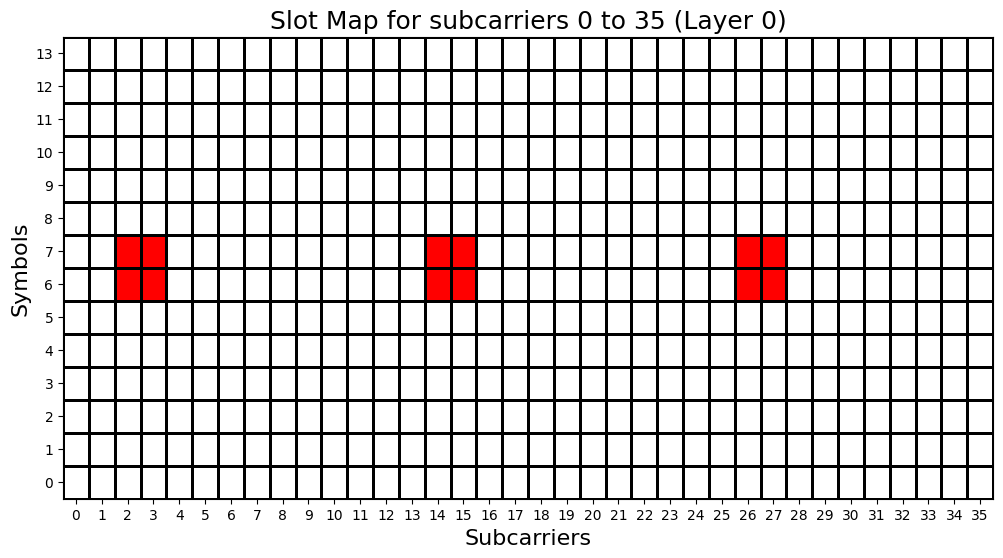
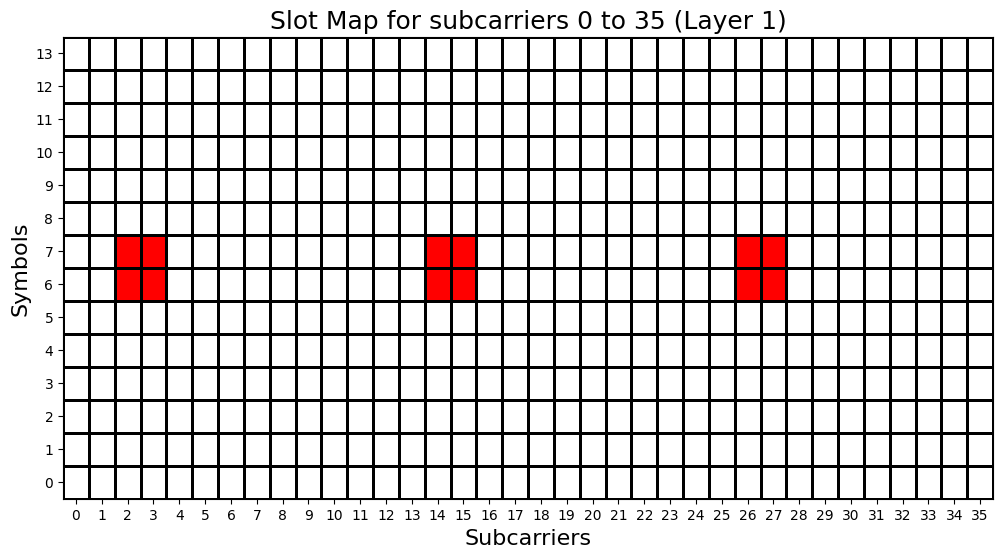
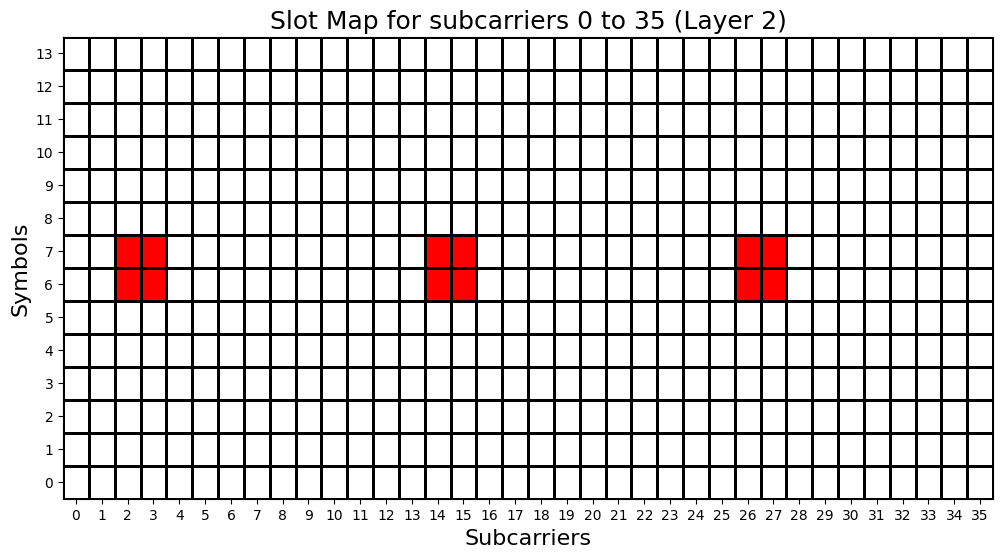
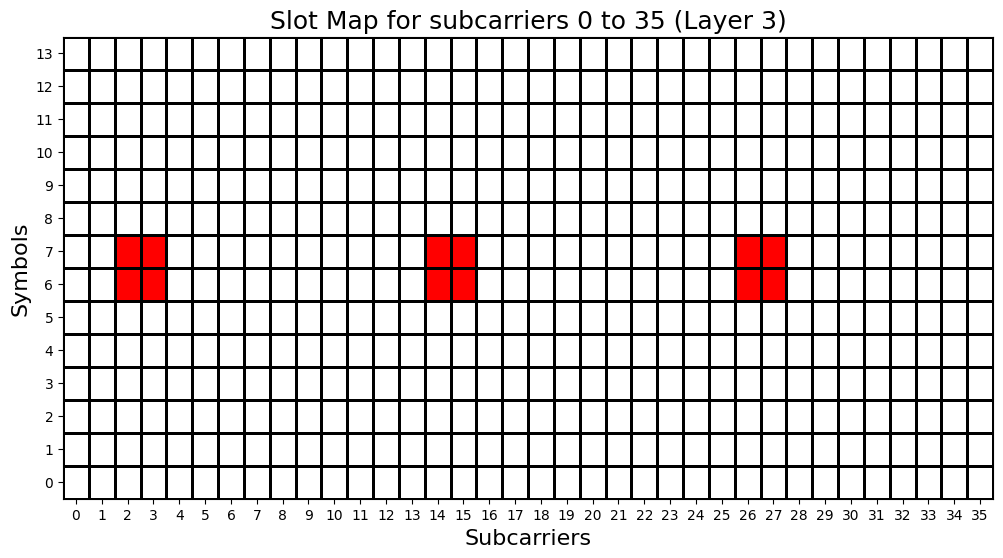
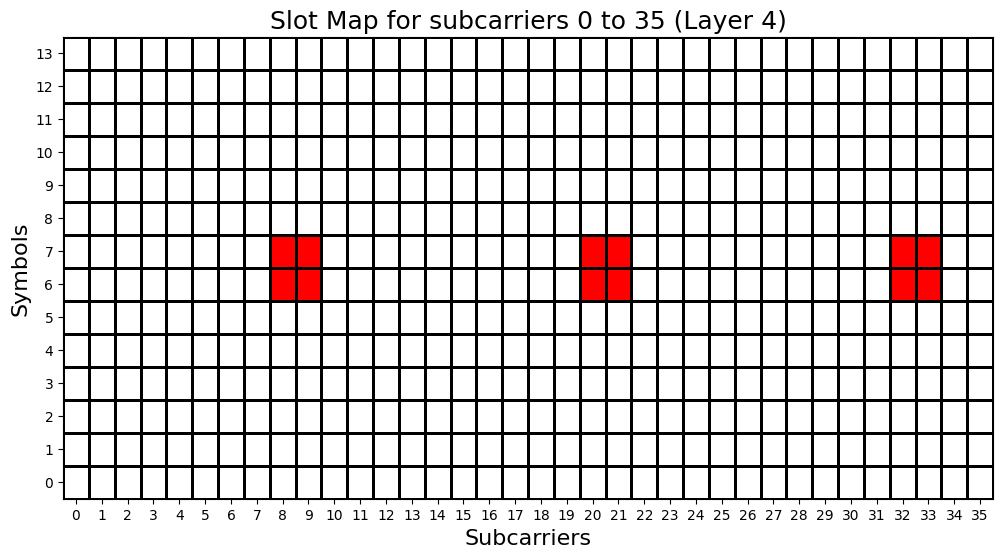
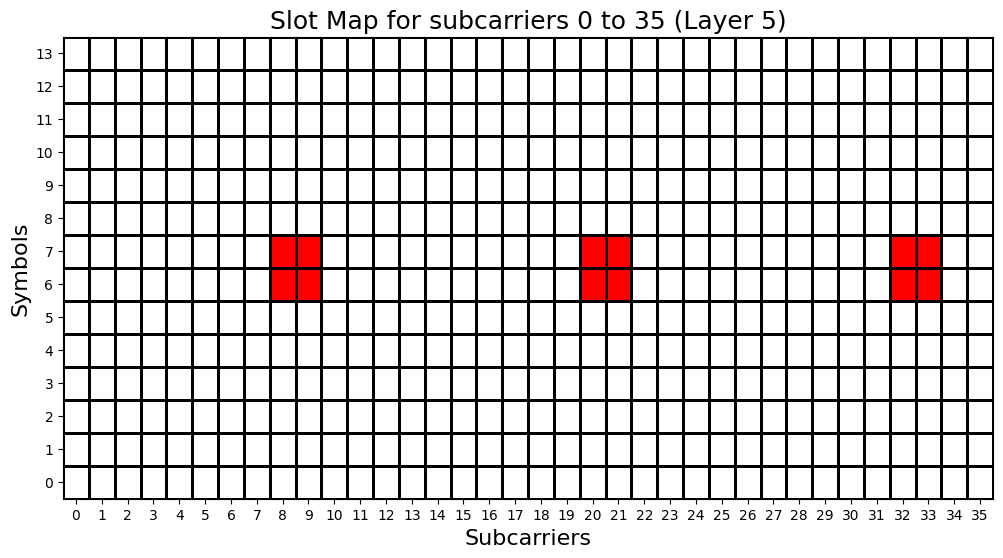
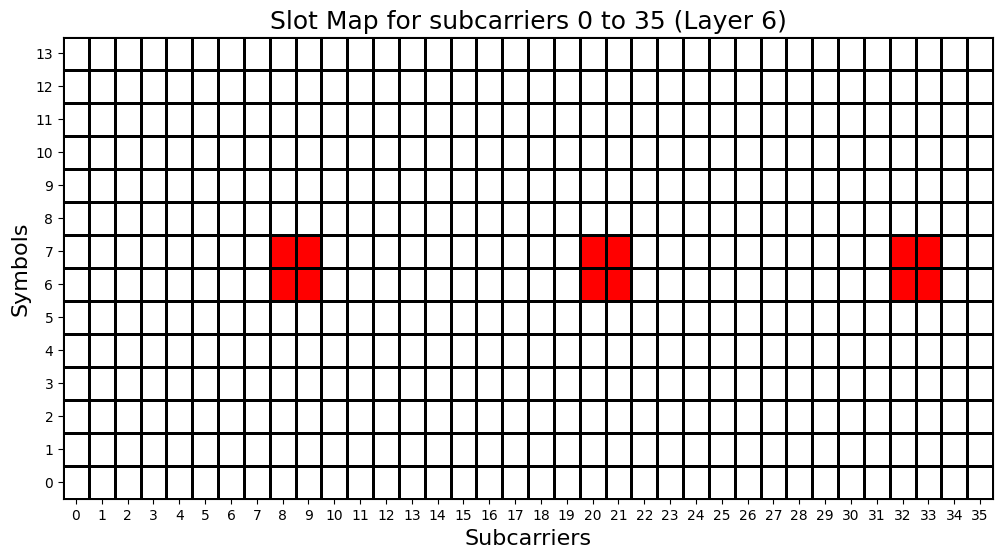
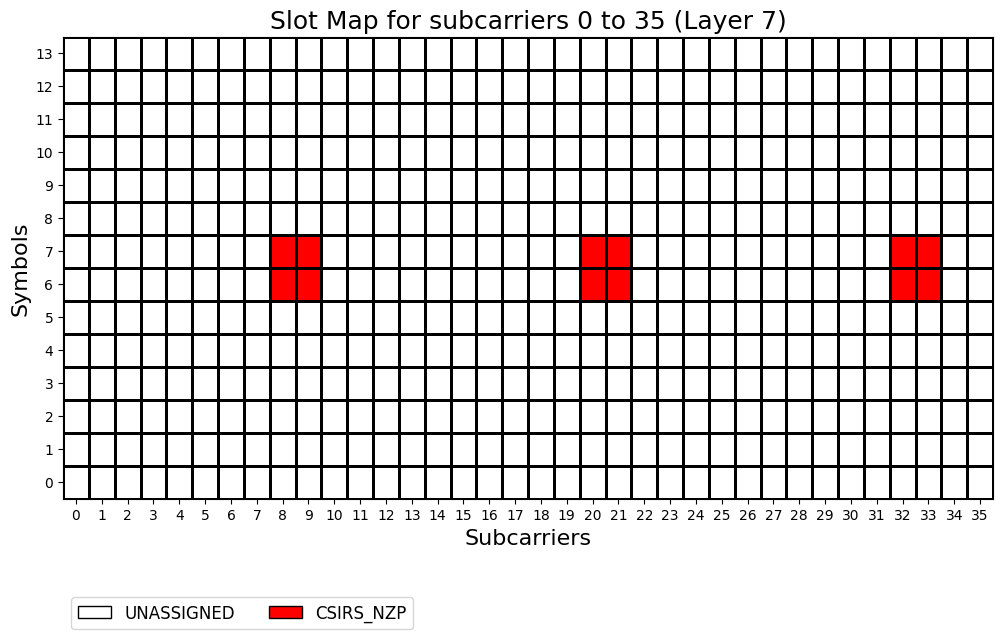
[13]:
# Example for 9th row of 38.211 Table 7.4.1.5.3-1
# 12 ports, all of 6 bits set in freqMap, CDM size is 2, and density is 1.
# The CSI-RS resources are at symbols 6 and all REs.
csiRsConfig = CsiRsConfig([CsiRsSet("NZP", bwp, symbols=[6], numPorts=12, cdmSize=2)])
csiRsConfig.print()
grid = bwp.createGrid(csiRsConfig.numPorts)
csiRsConfig.populateGrid(grid)
print("Grid Stats:")
stats = grid.getStats()
for key, value in stats.items(): print(" %s: %d"%(key, value))
grid.drawMap(ports=range(csiRsConfig.numPorts), reRange=(0,36))
CSI-RS Configuration: (1 Resource Sets)
CSI-RS Resource Set:(1 NZP Resources)
Resource Set ID: 0
Resource Type: periodic
Resource Blocks: 24 RBs starting at 0
Slot Period: 4
Bandwidth Part:
Resource Blocks: 24 RBs starting at 0 (288 subcarriers)
Subcarrier Spacing: 15 KHz
CP Type: normal
bandwidth: 4.32 MHz
symbolsPerSlot: 14
slotsPerSubFrame: 1
nFFT: 2048
frameNo: 0
slotNo: 0
CSI-RS:
resourceId: 0
numPorts: 12
cdmSize: 2 (fd-CDM2)
density: 1
RE Indexes: 0 2 4 6 8 10
Symbol Indexes: 6
Table Row: 9
Slot Offset: 0
Power: 0 db
scramblingID: 0
Grid Stats:
GridSize: 48384
UNASSIGNED: 47808
CSIRS_NZP: 576
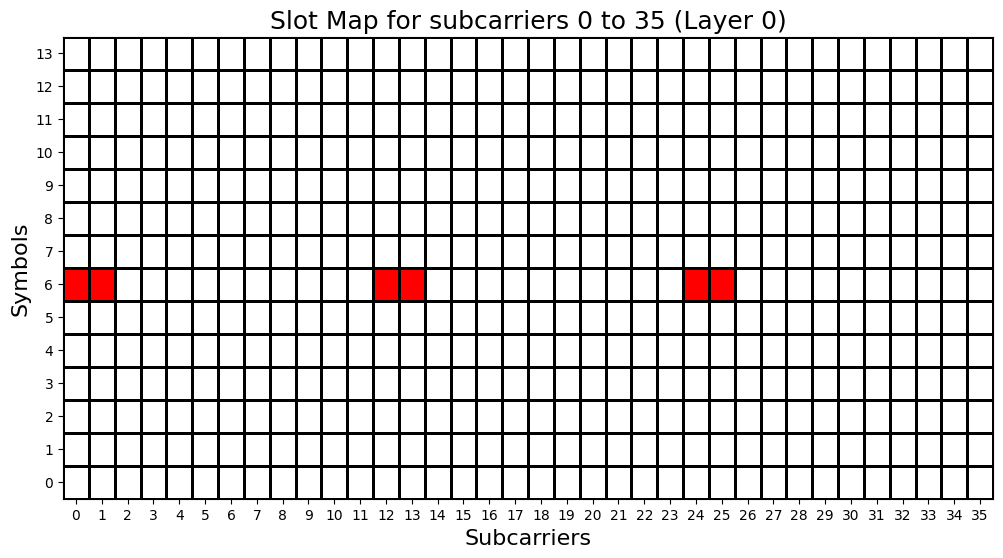
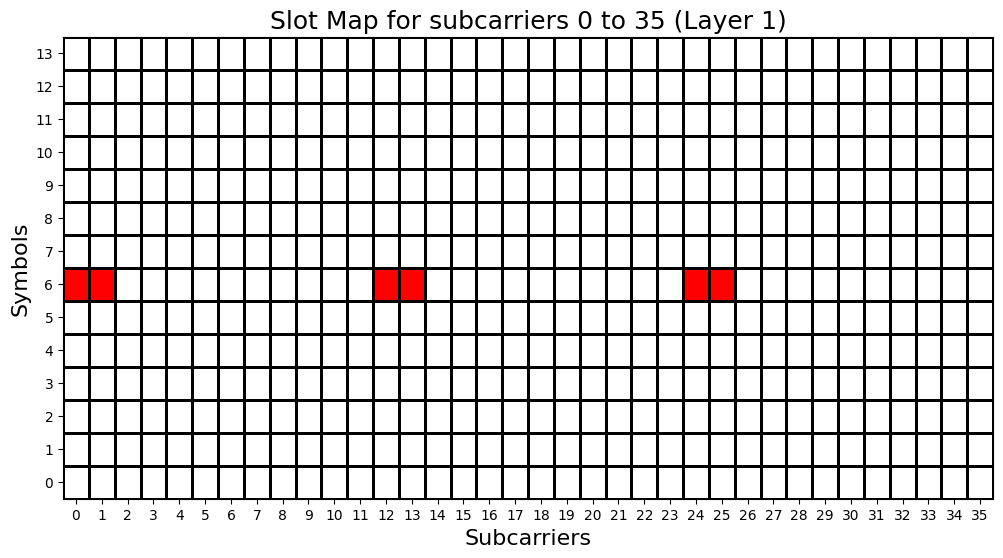
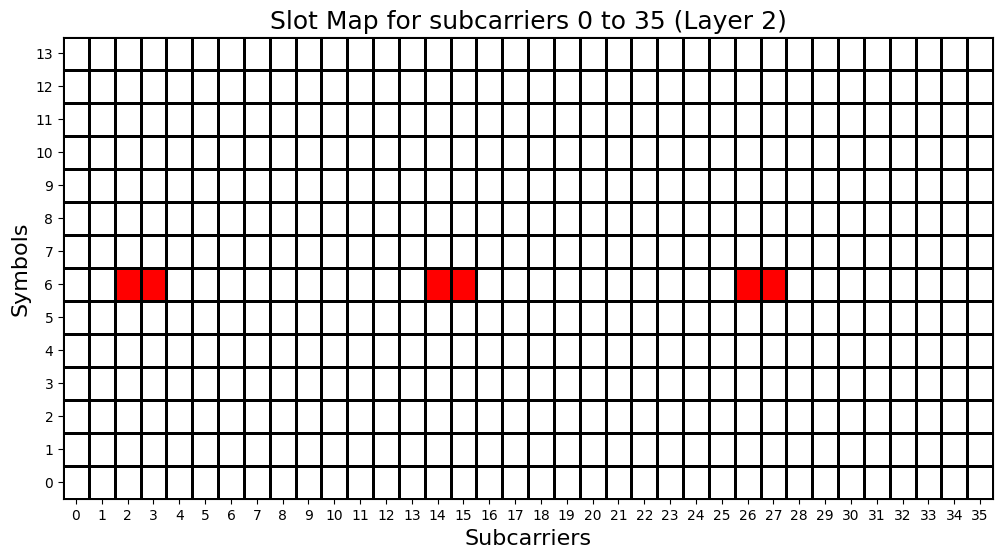
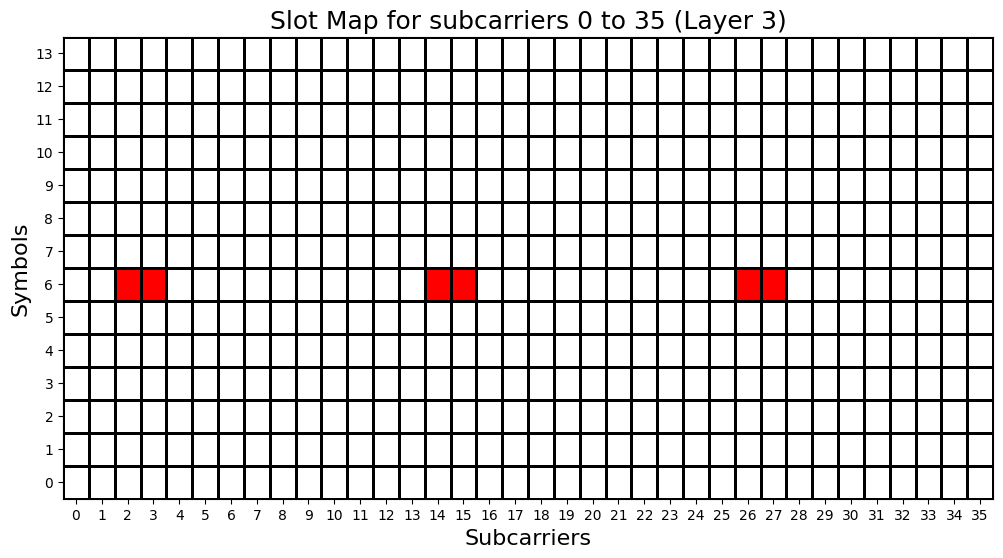
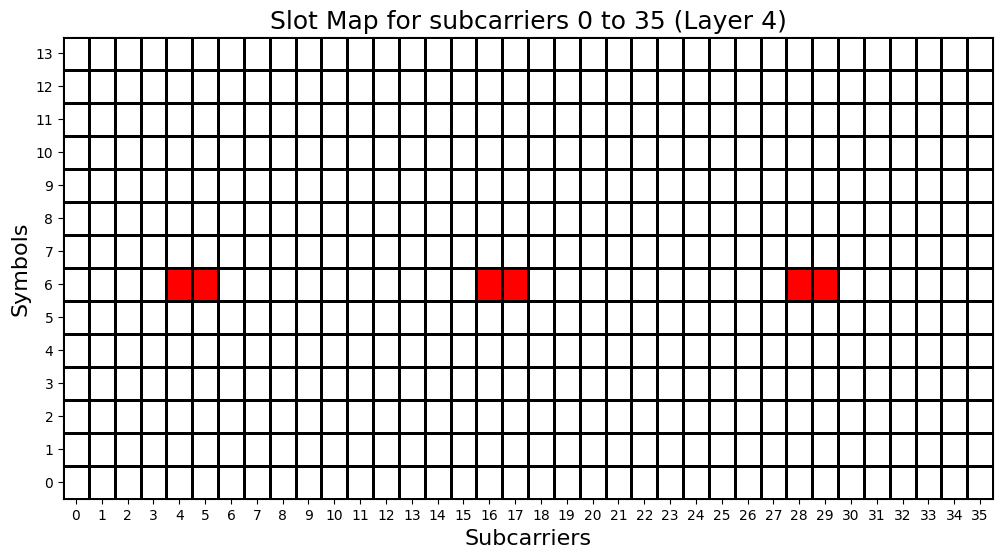
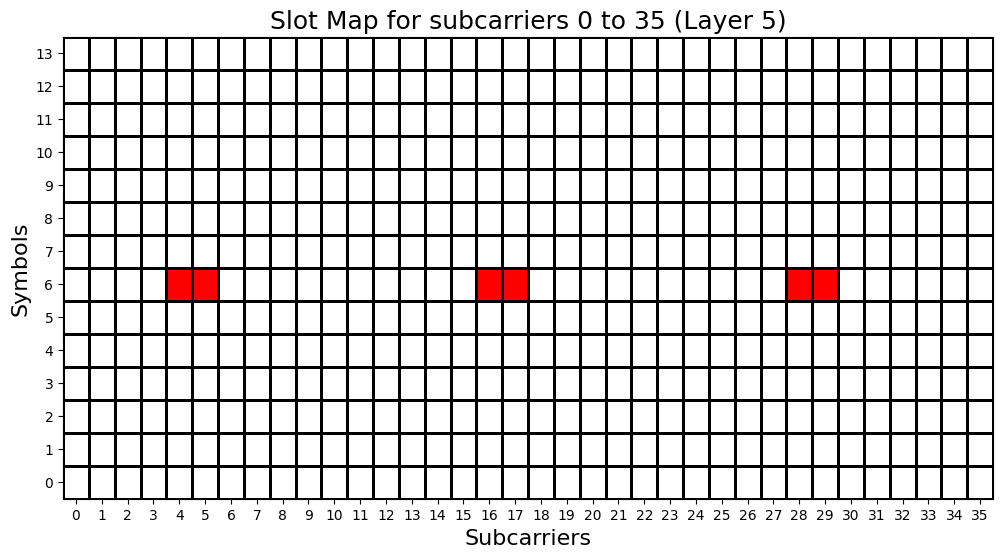
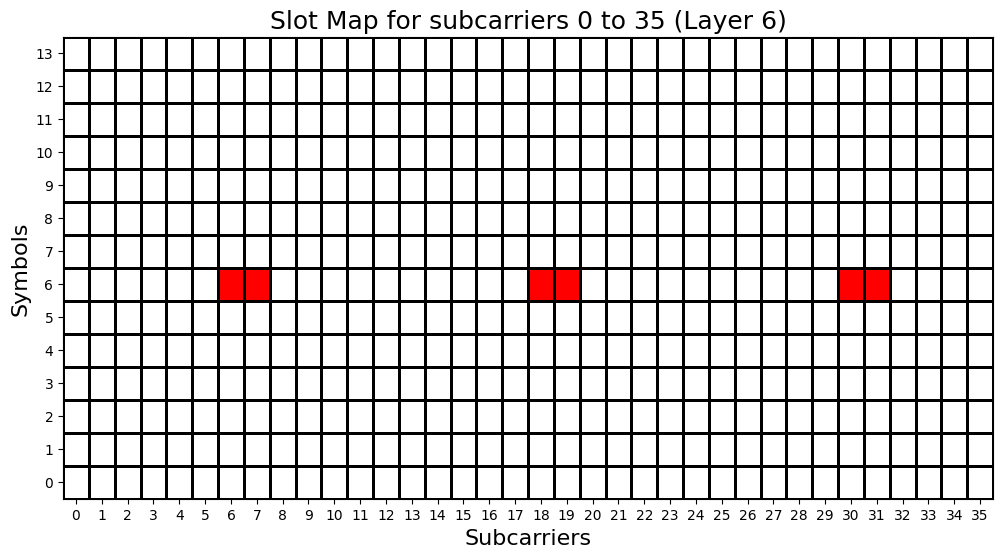
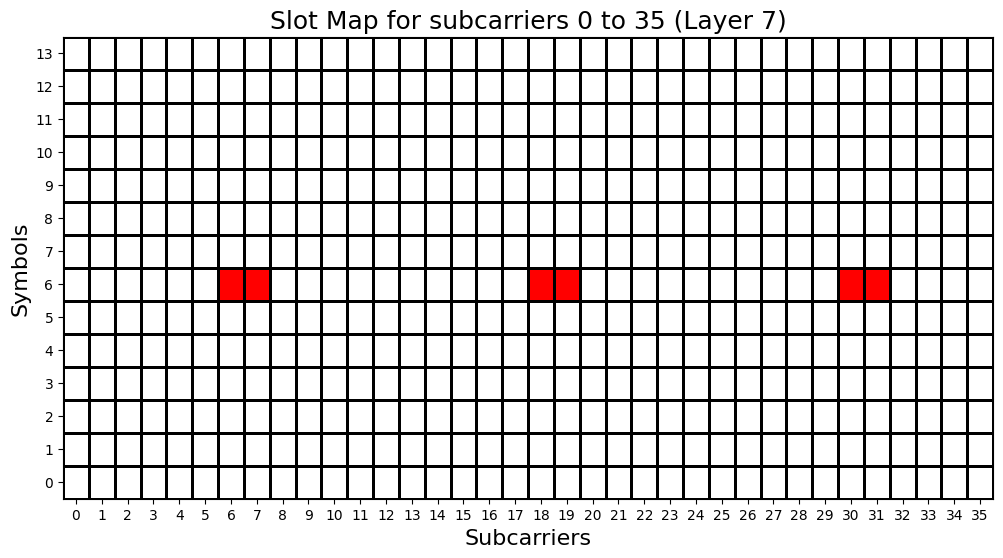
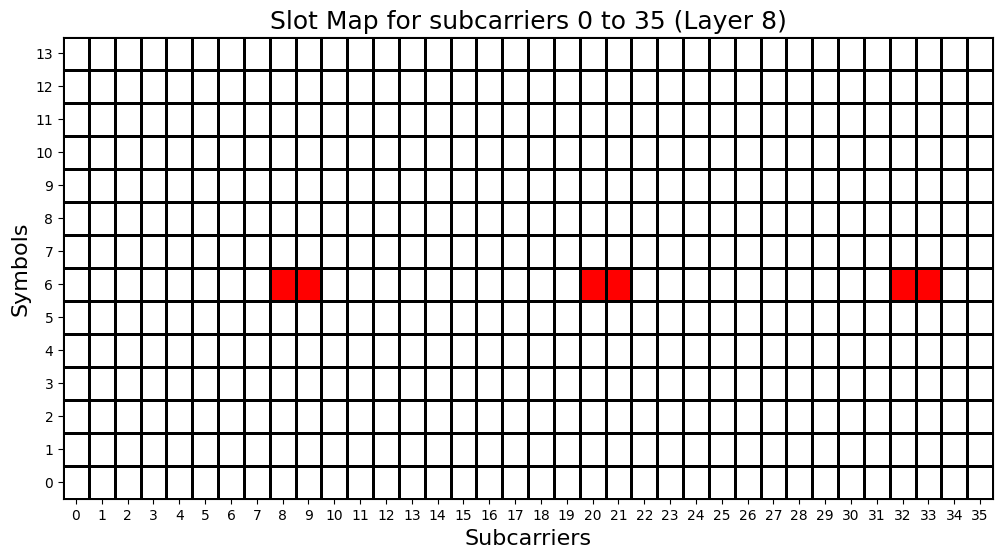
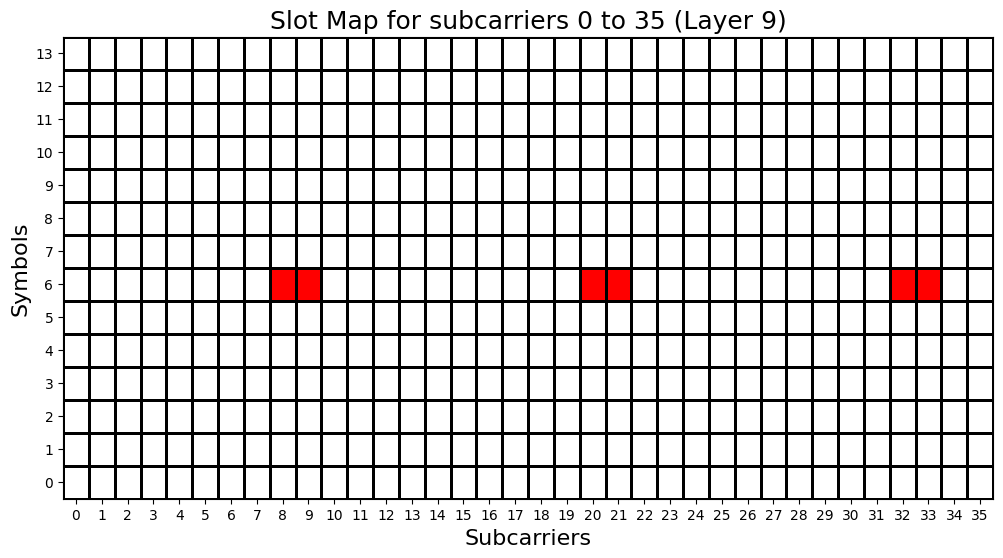
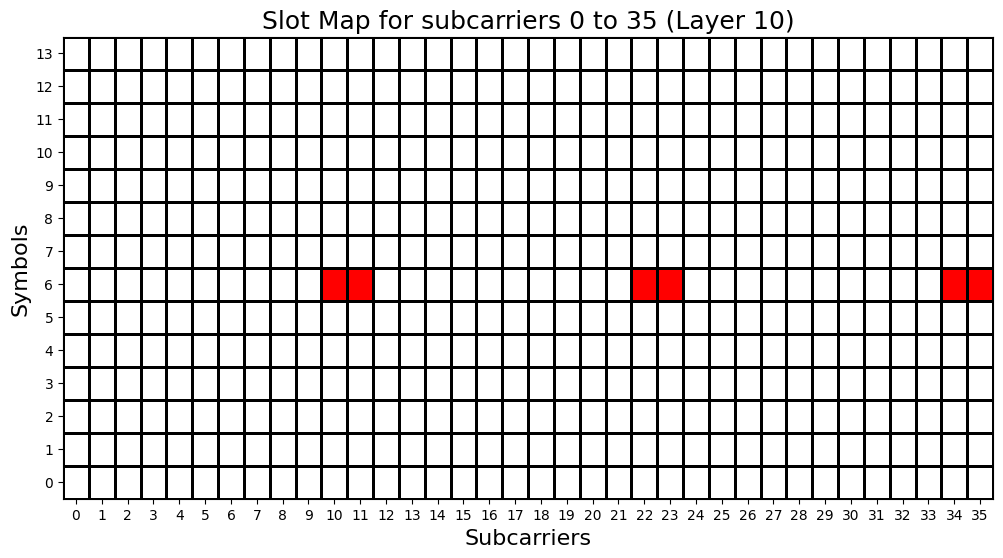
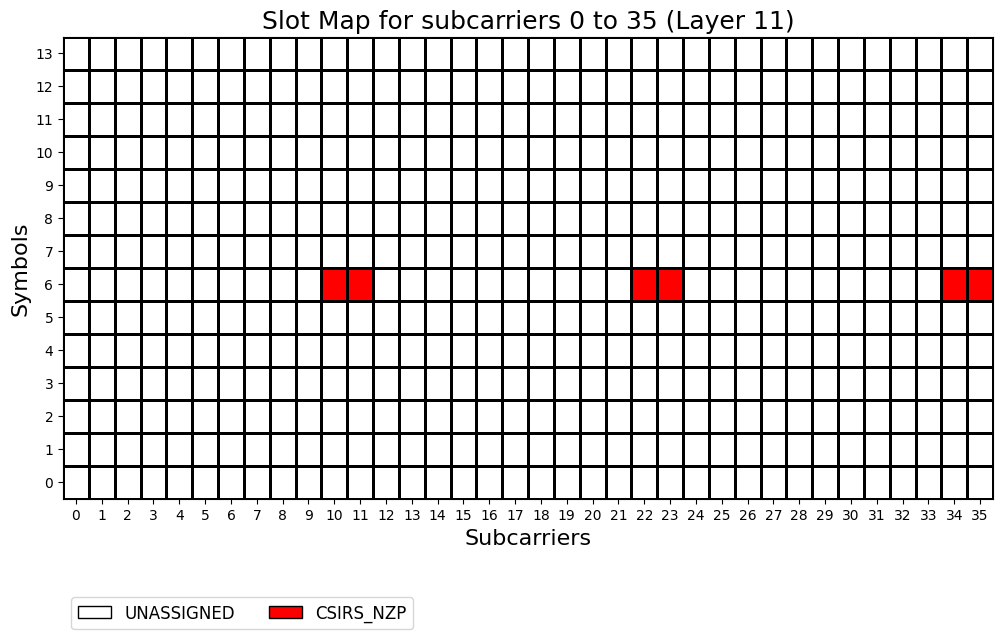
[14]:
# Example for 10th row of 38.211 Table 7.4.1.5.3-1
# 12 ports, 3 of 6 bits set in freqMap, CDM size is 4, and density is 1.
# The CSI-RS resources are at symbols (6,7) and REs (2,3), (6,7), and (10,11).
csiRsConfig = CsiRsConfig([CsiRsSet("NZP", bwp, symbols=[6], numPorts=12, freqMap="101010", cdmSize=4)])
csiRsConfig.print()
grid = bwp.createGrid(csiRsConfig.numPorts)
csiRsConfig.populateGrid(grid)
print("Grid Stats:")
stats = grid.getStats()
for key, value in stats.items(): print(" %s: %d"%(key, value))
grid.drawMap(ports=range(csiRsConfig.numPorts), reRange=(0,36))
CSI-RS Configuration: (1 Resource Sets)
CSI-RS Resource Set:(1 NZP Resources)
Resource Set ID: 0
Resource Type: periodic
Resource Blocks: 24 RBs starting at 0
Slot Period: 4
Bandwidth Part:
Resource Blocks: 24 RBs starting at 0 (288 subcarriers)
Subcarrier Spacing: 15 KHz
CP Type: normal
bandwidth: 4.32 MHz
symbolsPerSlot: 14
slotsPerSubFrame: 1
nFFT: 2048
frameNo: 0
slotNo: 0
CSI-RS:
resourceId: 0
numPorts: 12
cdmSize: 4 (cdm4-FD2-TD2)
density: 1
RE Indexes: 2 6 10
Symbol Indexes: 6
Table Row: 10
Slot Offset: 0
Power: 0 db
scramblingID: 0
Grid Stats:
GridSize: 48384
UNASSIGNED: 47232
CSIRS_NZP: 1152
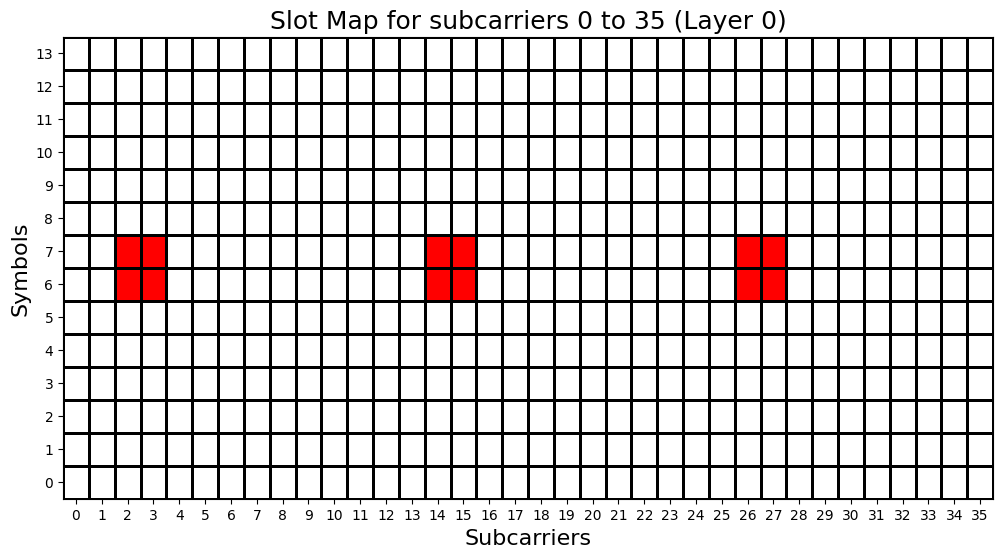
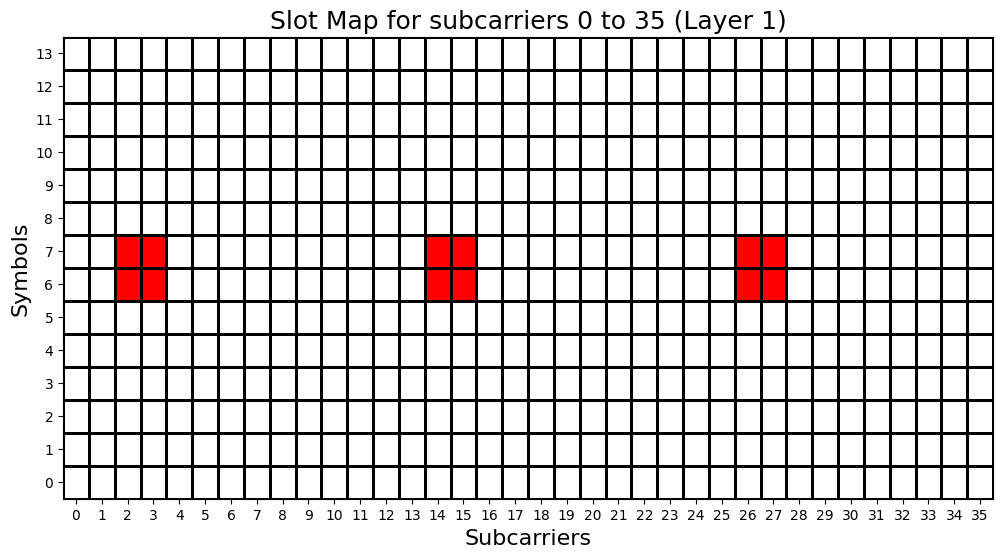
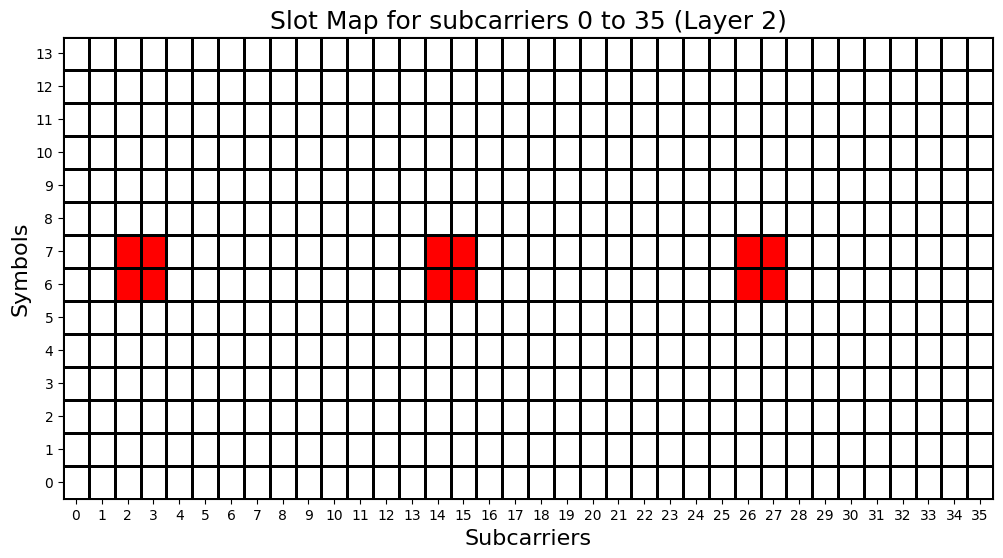
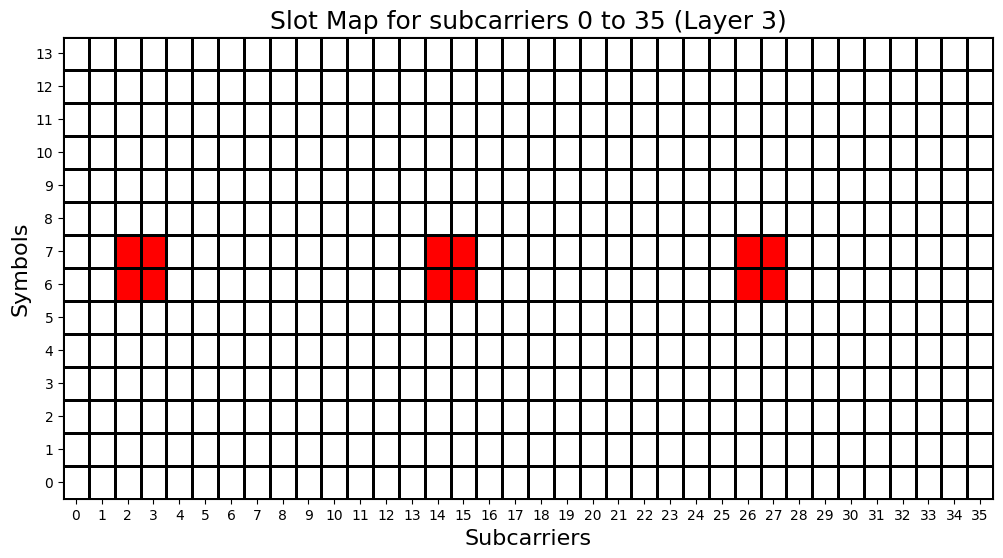
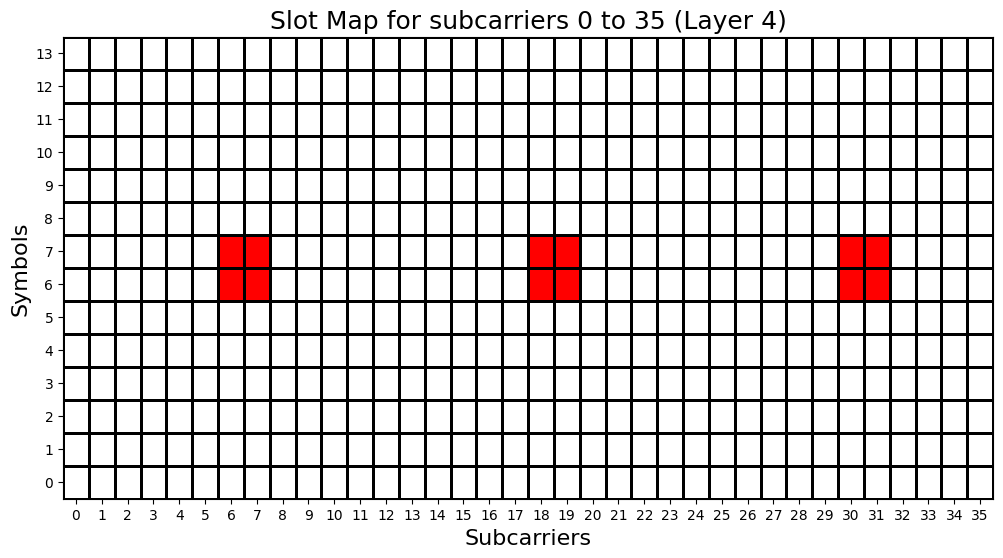
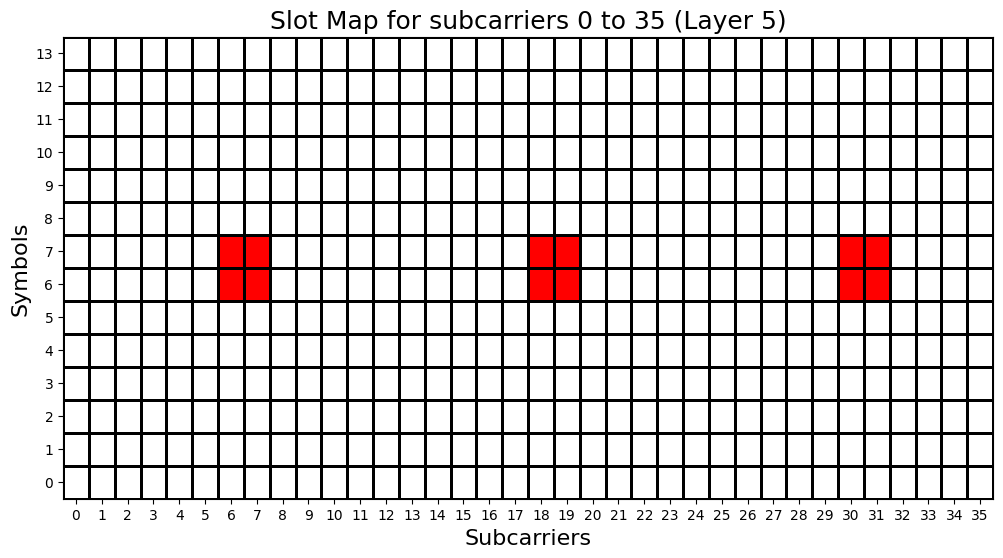
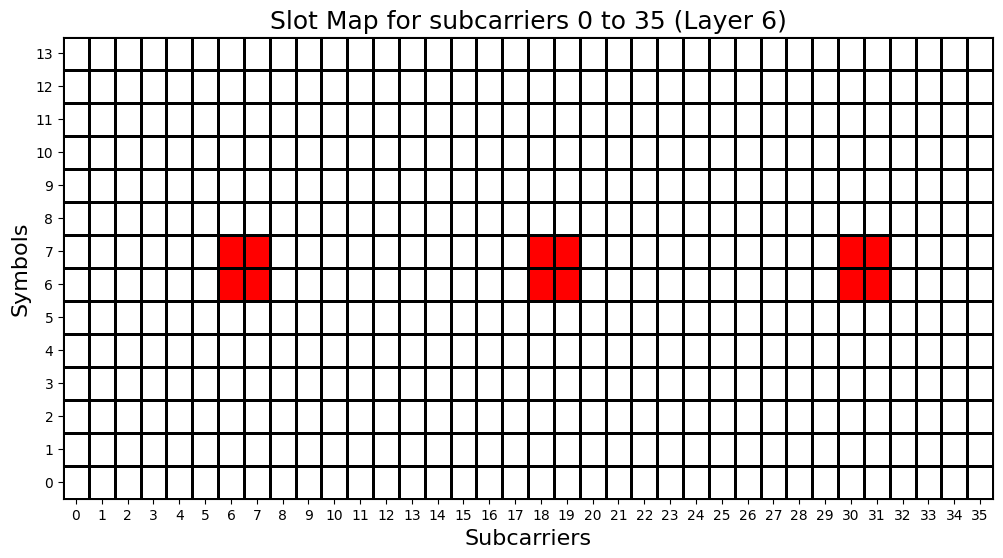
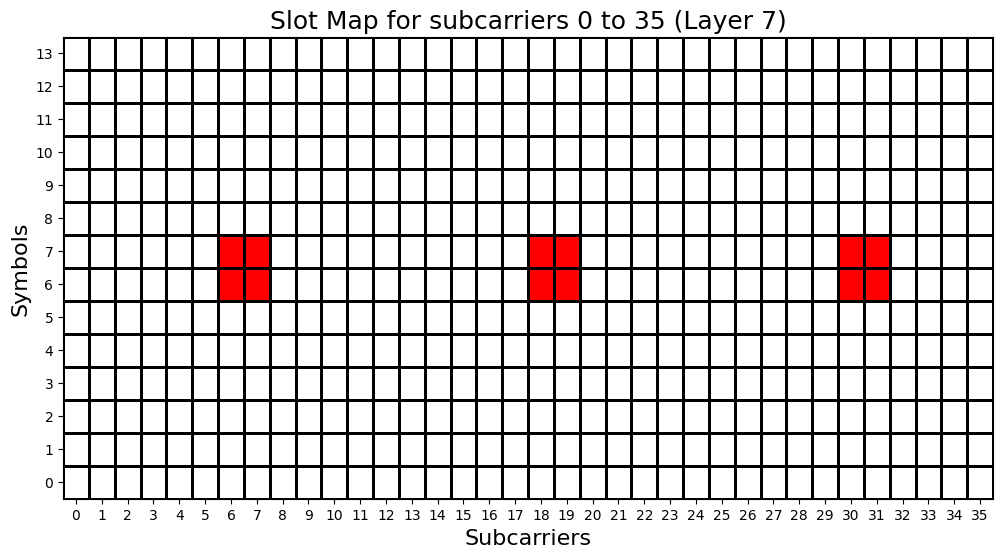
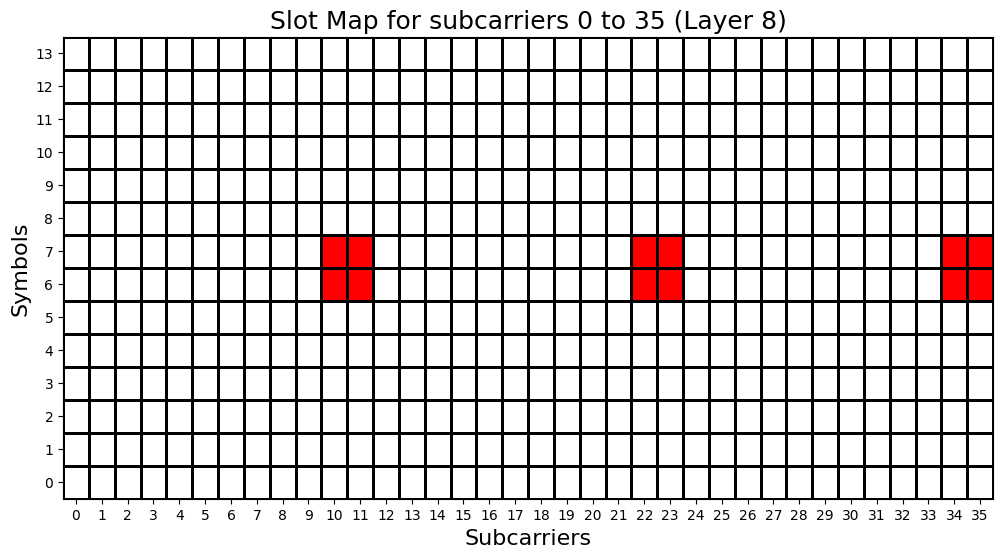
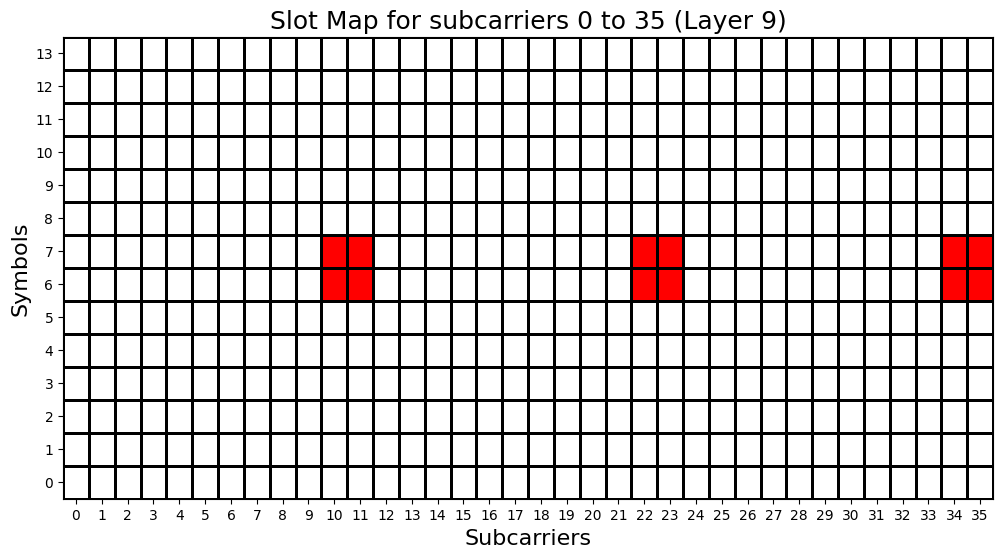
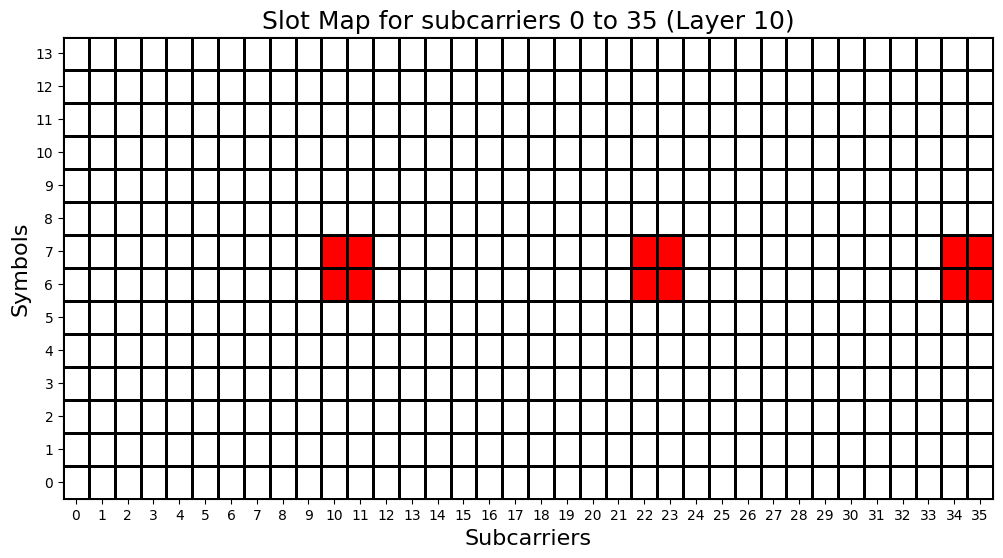
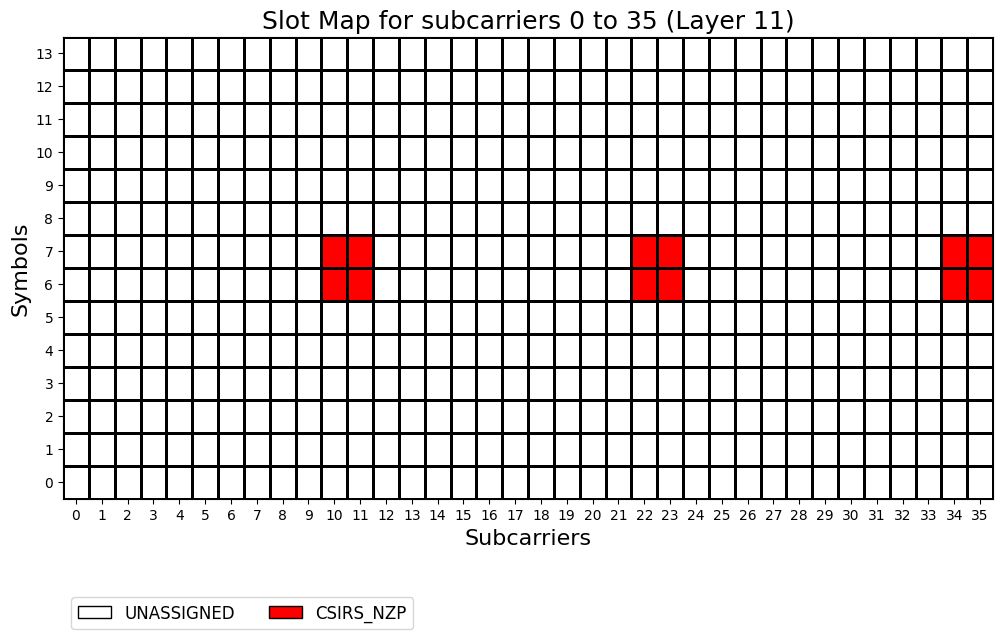
[15]:
# Example for 11th row of 38.211 Table 7.4.1.5.3-1
# 16 ports, 4 of 6 bits set in freqMap, CDM size is 2, and density is 1.
# The CSI-RS resources are at symbols 6,7 and REs (0,1), (2,3), (8,9) and (10,11).
# Note that this configuration also allows density=.5 which would cause every other
# RB to be skipped for the CSR-RS allocation. (default density is 1)
csiRsConfig = CsiRsConfig([CsiRsSet("NZP", bwp, symbols=[6], numPorts=16, freqMap="110011", cdmSize=2)])
csiRsConfig.print()
grid = bwp.createGrid(csiRsConfig.numPorts)
csiRsConfig.populateGrid(grid)
print("Grid Stats:")
stats = grid.getStats()
for key, value in stats.items(): print(" %s: %d"%(key, value))
grid.drawMap(ports=range(csiRsConfig.numPorts), reRange=(0,36))
CSI-RS Configuration: (1 Resource Sets)
CSI-RS Resource Set:(1 NZP Resources)
Resource Set ID: 0
Resource Type: periodic
Resource Blocks: 24 RBs starting at 0
Slot Period: 4
Bandwidth Part:
Resource Blocks: 24 RBs starting at 0 (288 subcarriers)
Subcarrier Spacing: 15 KHz
CP Type: normal
bandwidth: 4.32 MHz
symbolsPerSlot: 14
slotsPerSubFrame: 1
nFFT: 2048
frameNo: 0
slotNo: 0
CSI-RS:
resourceId: 0
numPorts: 16
cdmSize: 2 (fd-CDM2)
density: 1
RE Indexes: 0 2 8 10
Symbol Indexes: 6
Table Row: 11
Slot Offset: 0
Power: 0 db
scramblingID: 0
Grid Stats:
GridSize: 64512
UNASSIGNED: 63744
CSIRS_NZP: 768
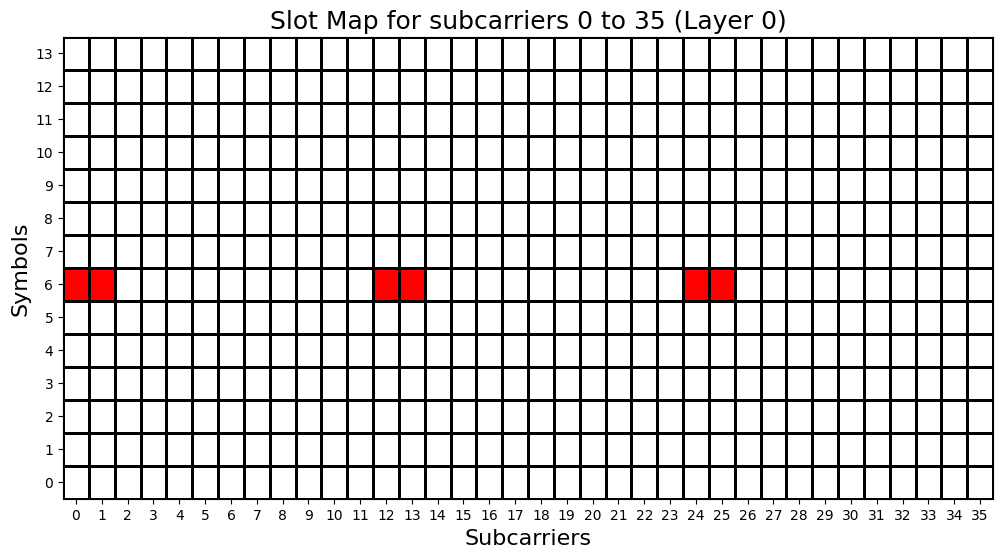
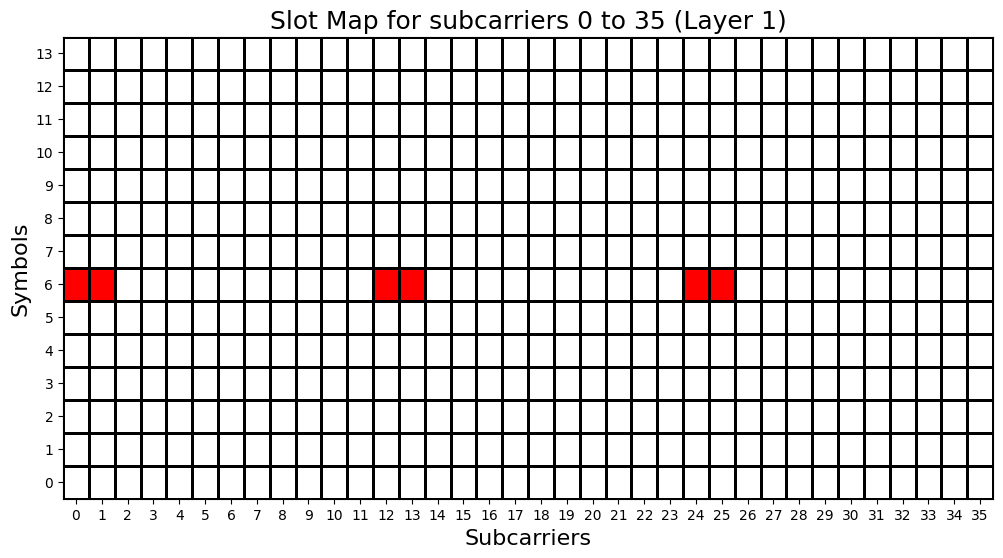
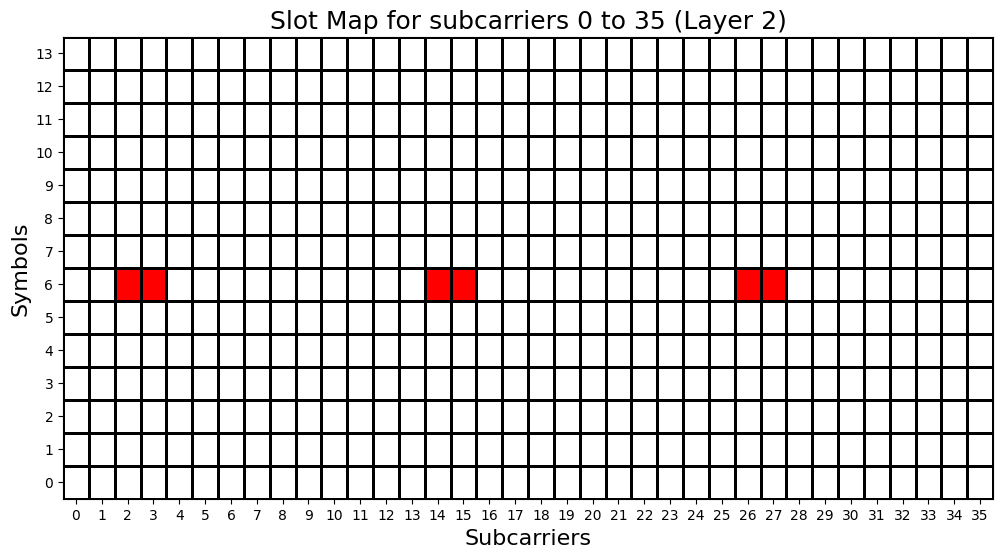
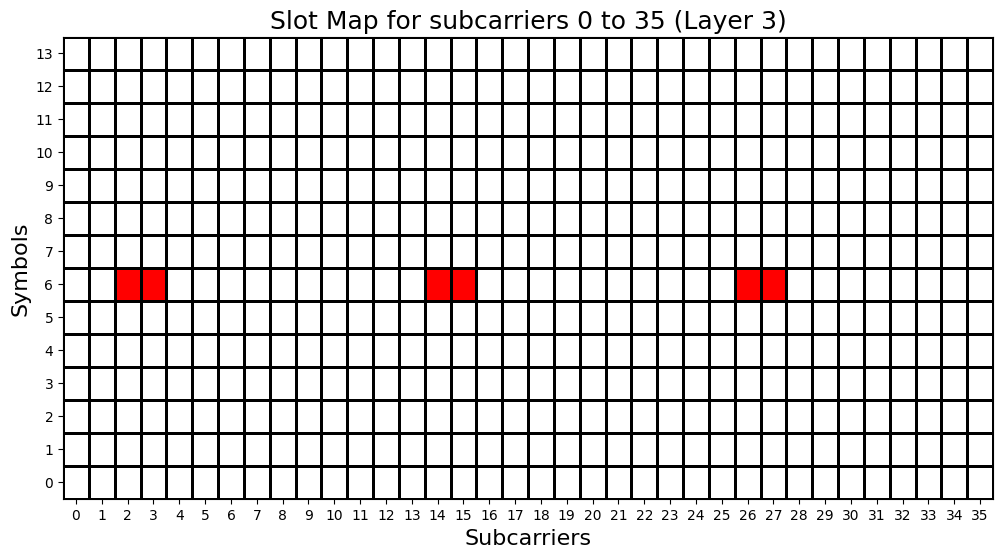
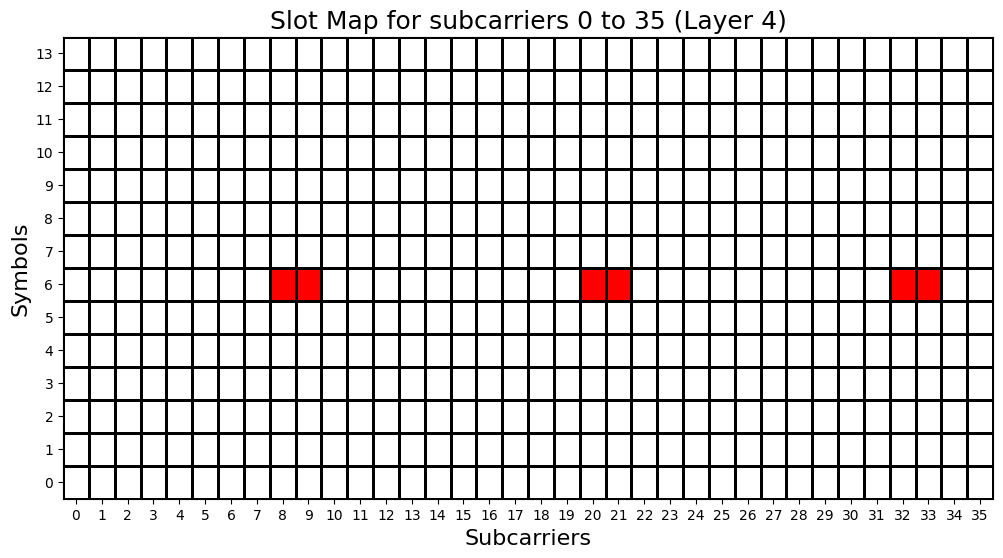
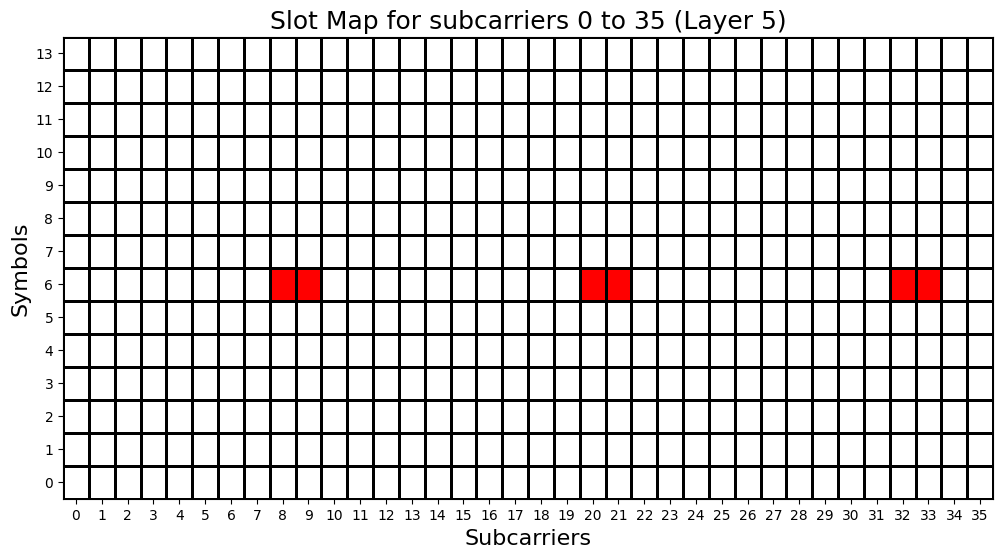
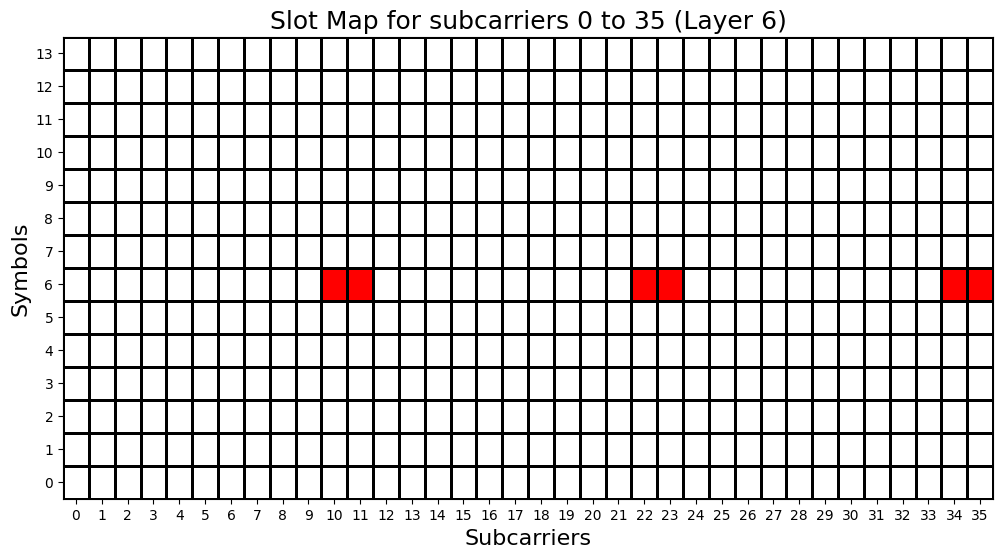
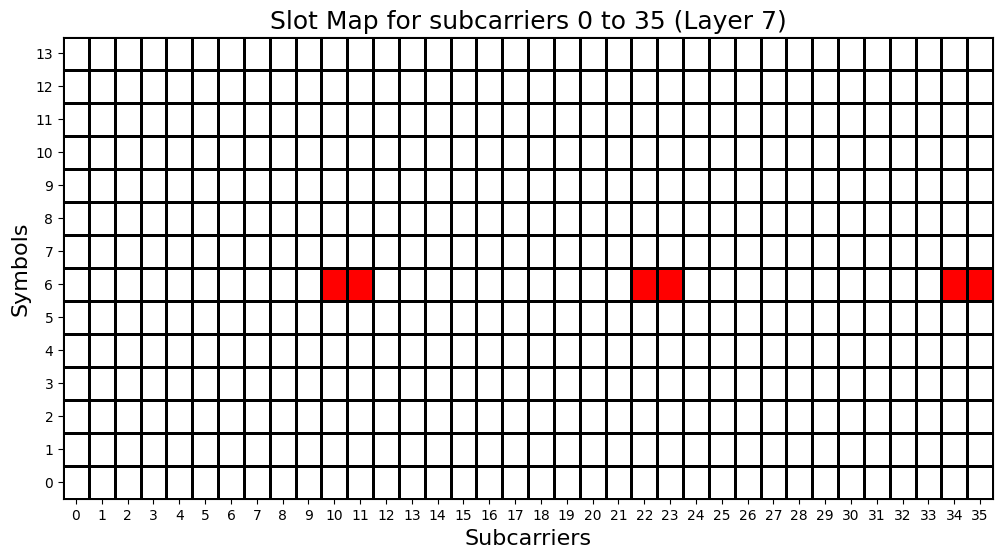
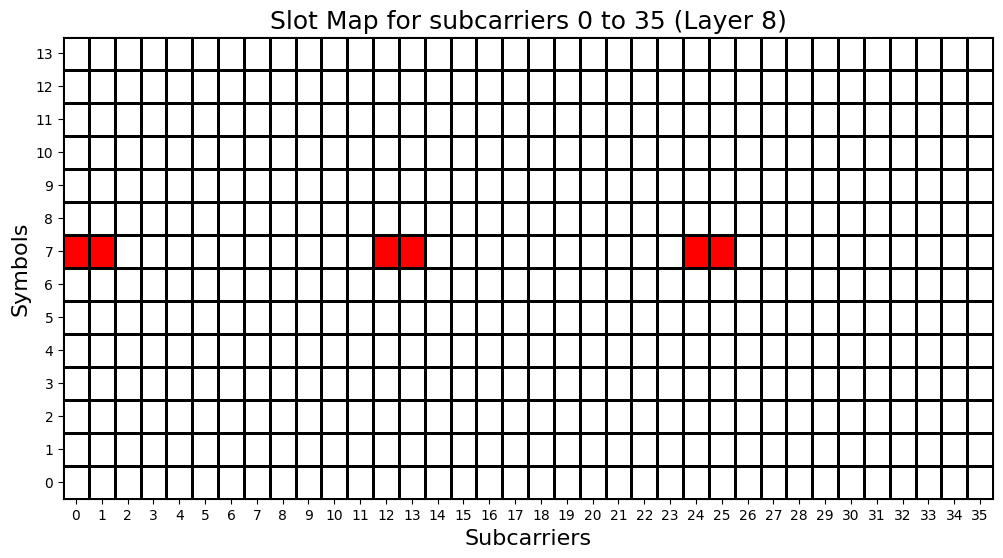
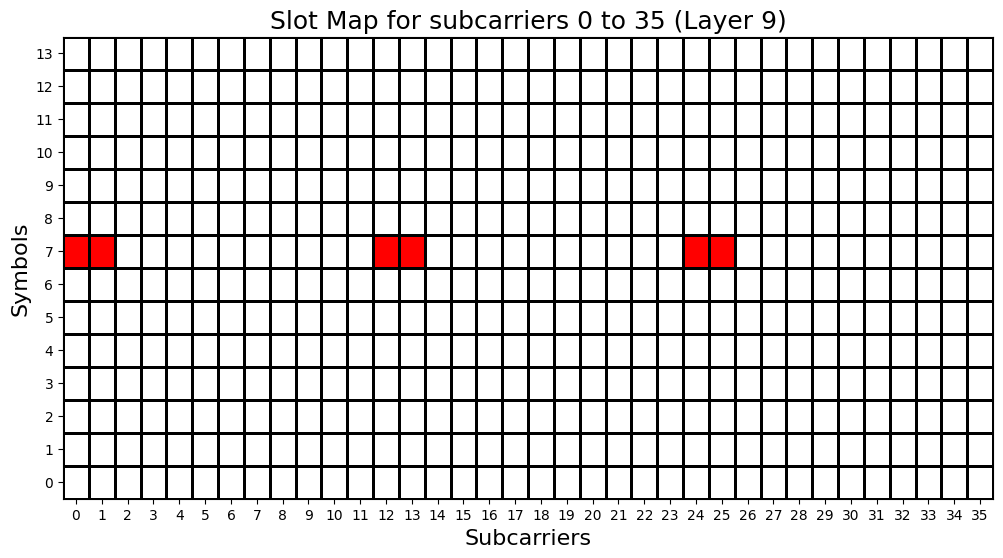
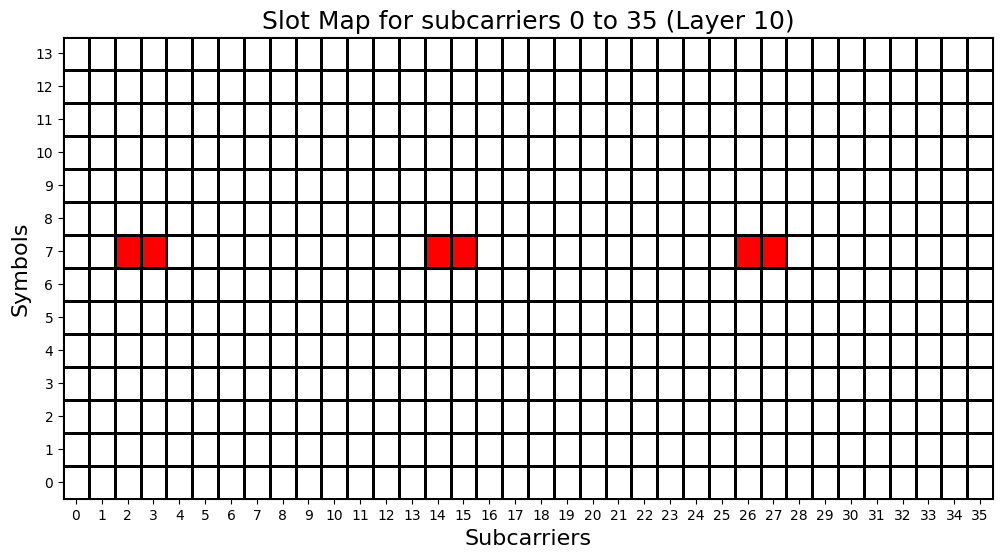
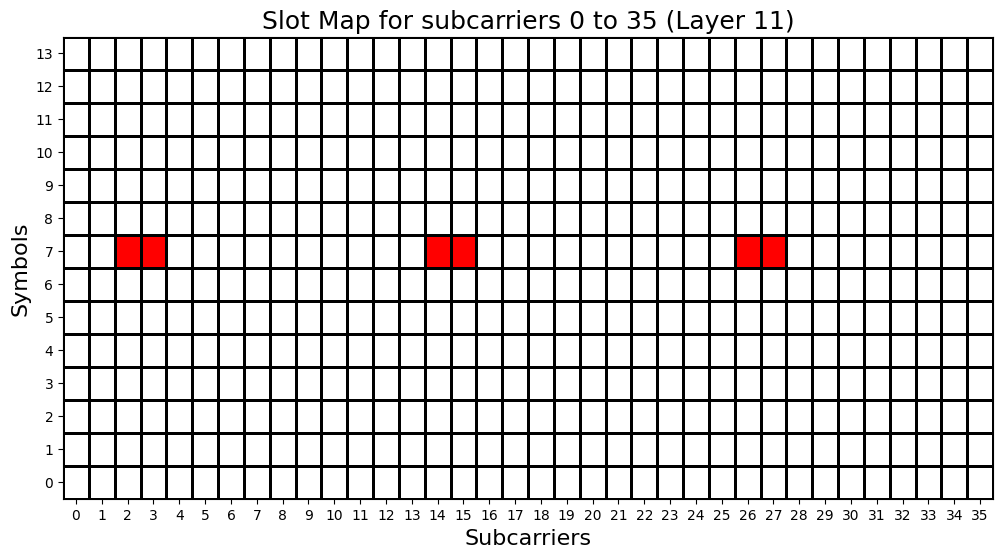
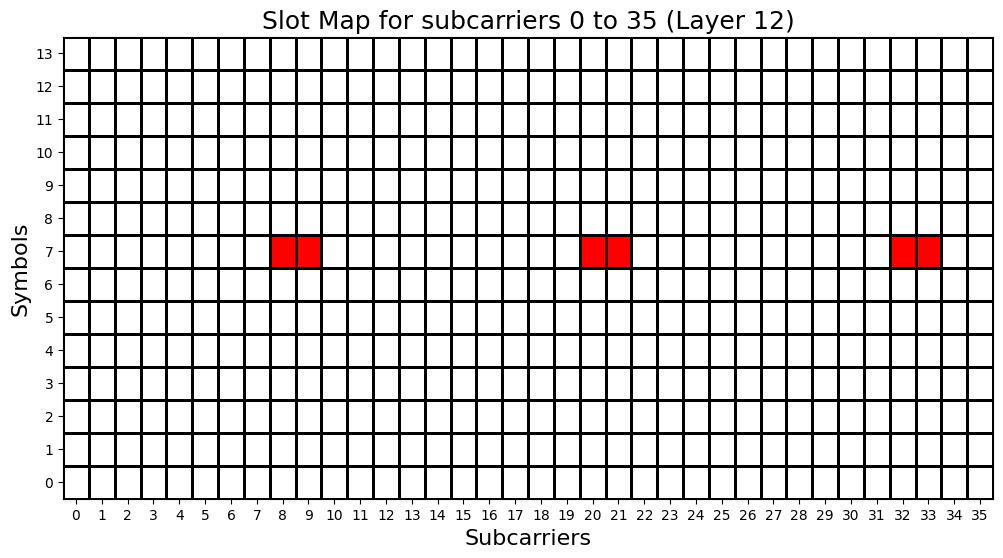
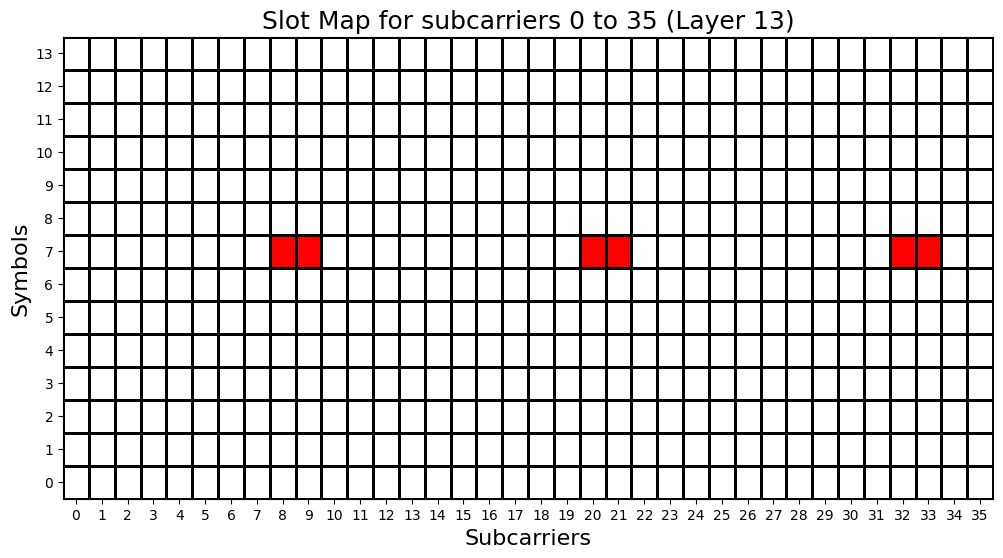
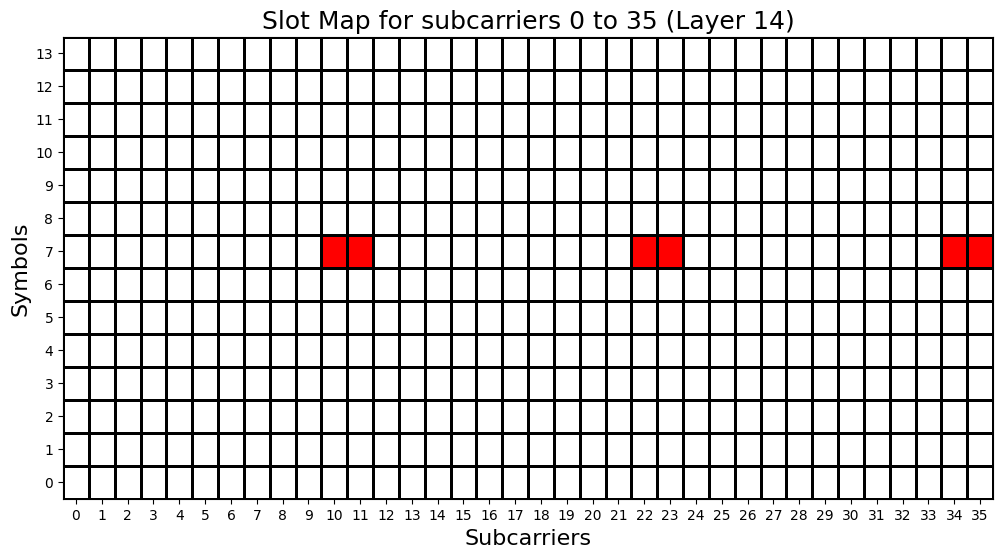
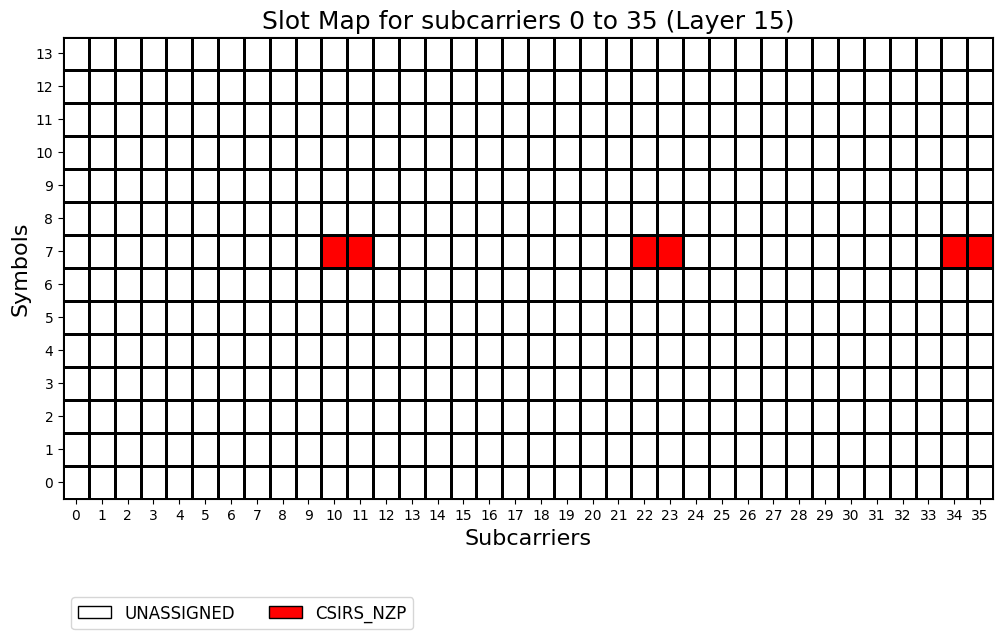
[16]:
# Example for 12th row of 38.211 Table 7.4.1.5.3-1
# 16 ports, 4 of 6 bits set in freqMap, CDM size is 4, and density is 1.
# The CSI-RS resources are at symbols (6,7) and REs (0,1), (2,3), (8,9) and (10,11).
# Note that this configuration also allows density=.5 which would cause every other
# RB to be skipped for the CSR-RS allocation. (default density is 1)
csiRsConfig = CsiRsConfig([CsiRsSet("NZP", bwp, symbols=[6], numPorts=16, freqMap="110011", cdmSize=4)])
csiRsConfig.print()
grid = bwp.createGrid(csiRsConfig.numPorts)
csiRsConfig.populateGrid(grid)
print("Grid Stats:")
stats = grid.getStats()
for key, value in stats.items(): print(" %s: %d"%(key, value))
grid.drawMap(ports=range(csiRsConfig.numPorts), reRange=(0,36))
CSI-RS Configuration: (1 Resource Sets)
CSI-RS Resource Set:(1 NZP Resources)
Resource Set ID: 0
Resource Type: periodic
Resource Blocks: 24 RBs starting at 0
Slot Period: 4
Bandwidth Part:
Resource Blocks: 24 RBs starting at 0 (288 subcarriers)
Subcarrier Spacing: 15 KHz
CP Type: normal
bandwidth: 4.32 MHz
symbolsPerSlot: 14
slotsPerSubFrame: 1
nFFT: 2048
frameNo: 0
slotNo: 0
CSI-RS:
resourceId: 0
numPorts: 16
cdmSize: 4 (cdm4-FD2-TD2)
density: 1
RE Indexes: 0 2 8 10
Symbol Indexes: 6
Table Row: 12
Slot Offset: 0
Power: 0 db
scramblingID: 0
Grid Stats:
GridSize: 64512
UNASSIGNED: 62976
CSIRS_NZP: 1536
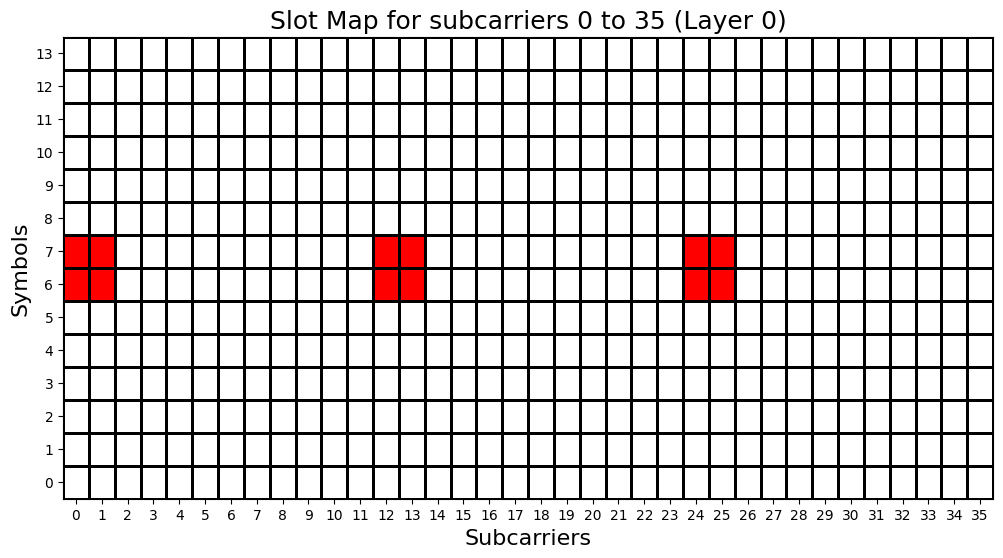
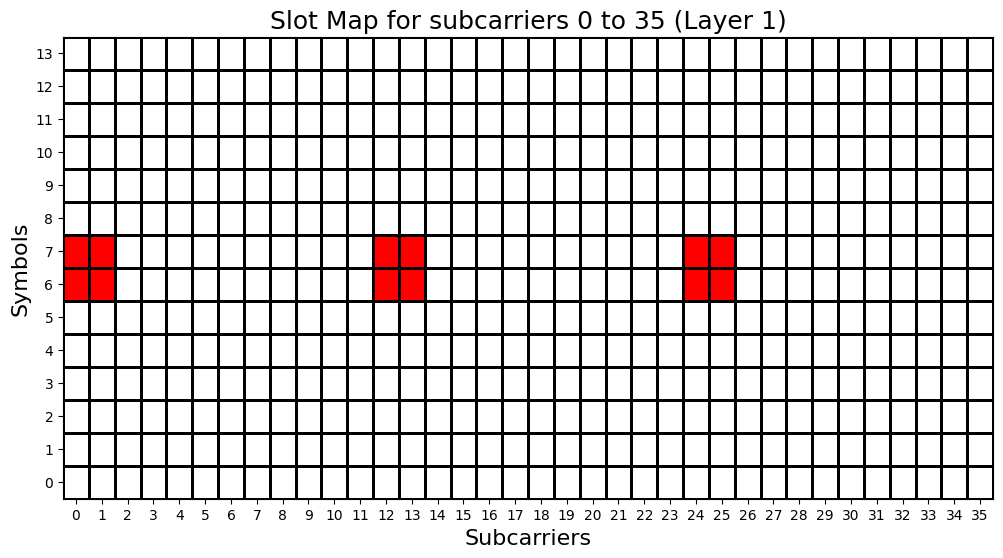
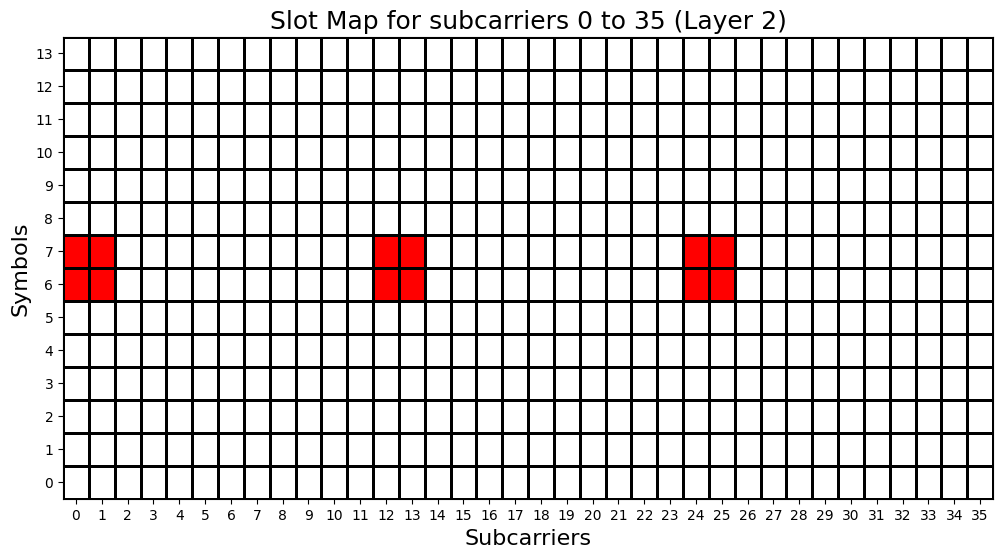
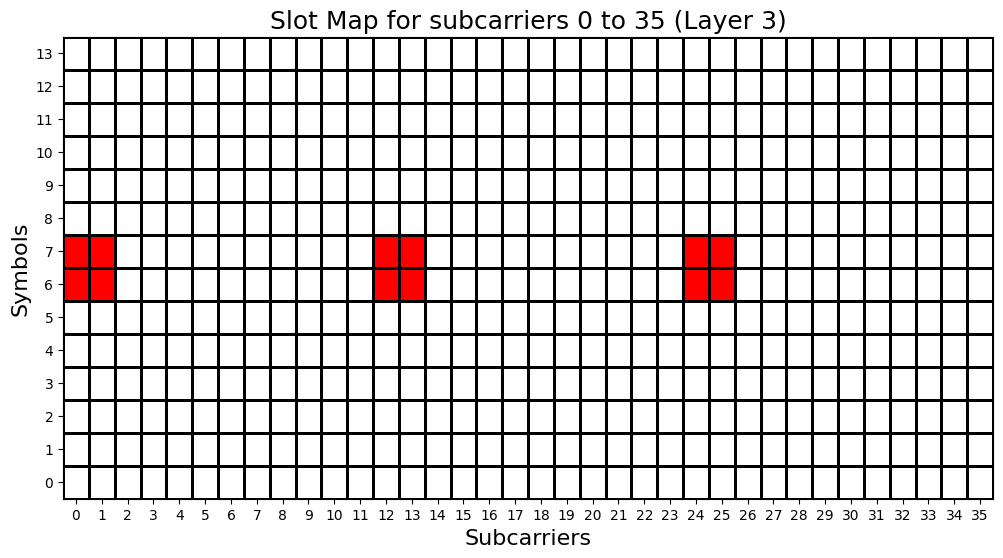
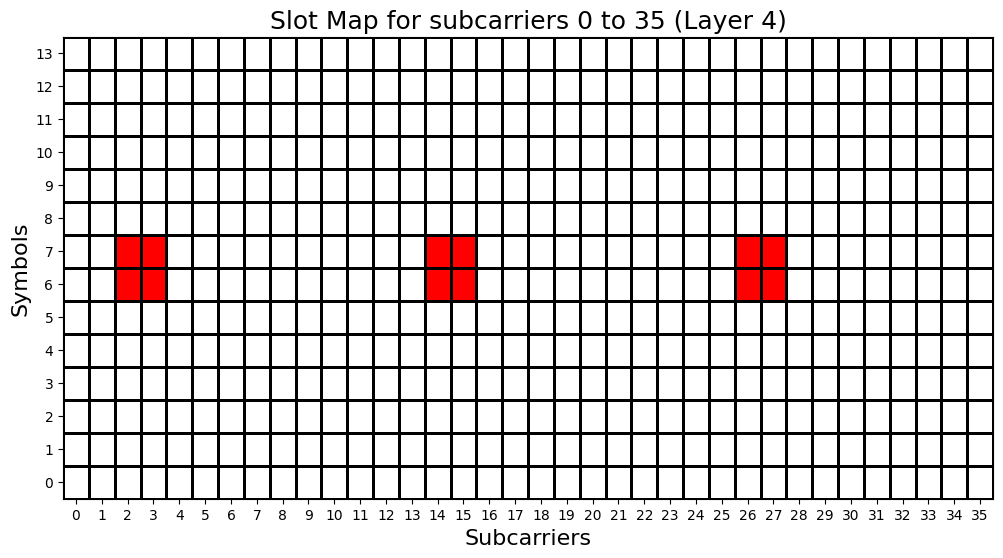
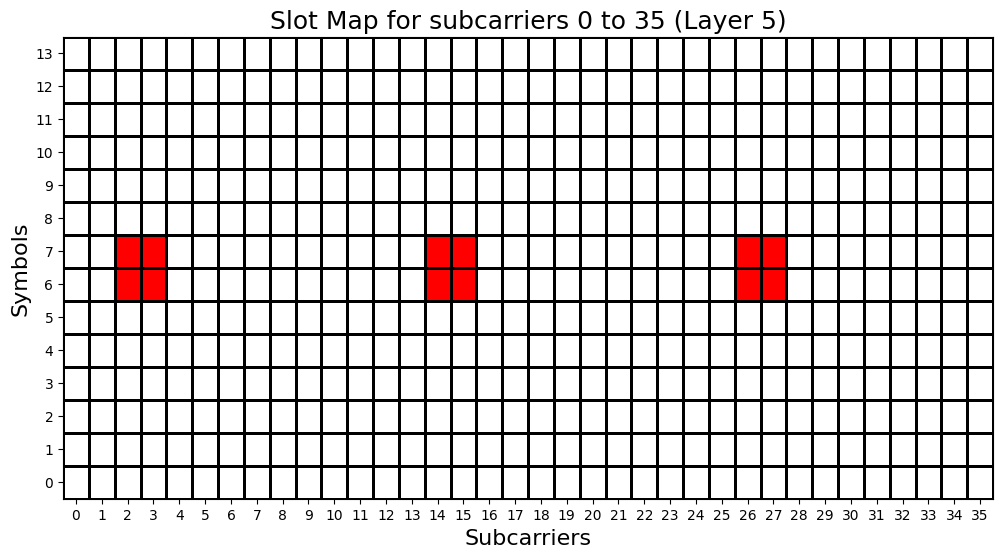
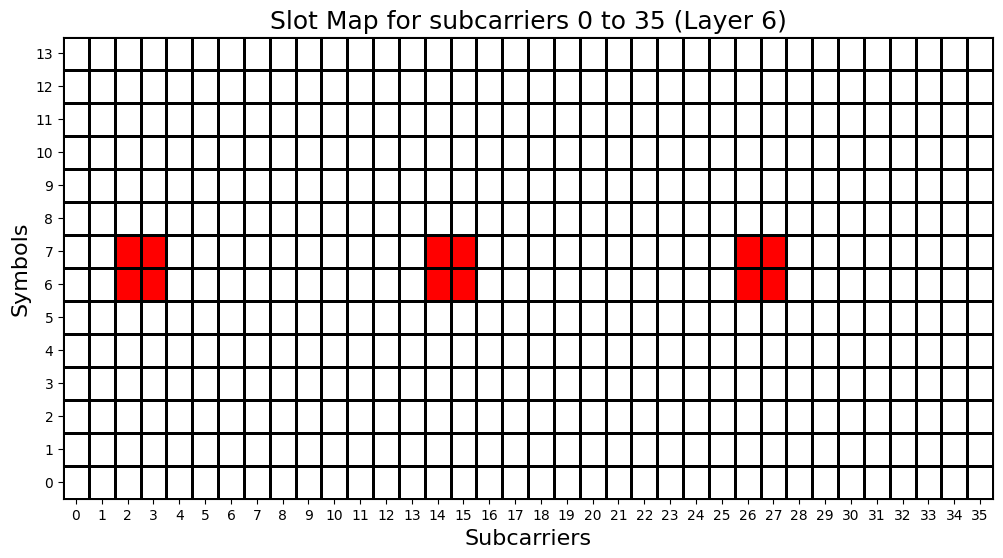
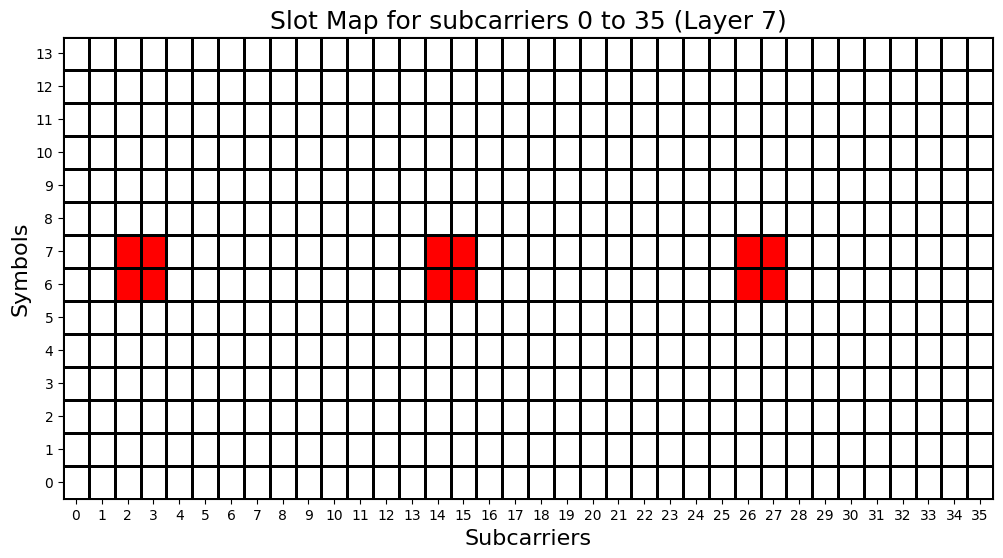
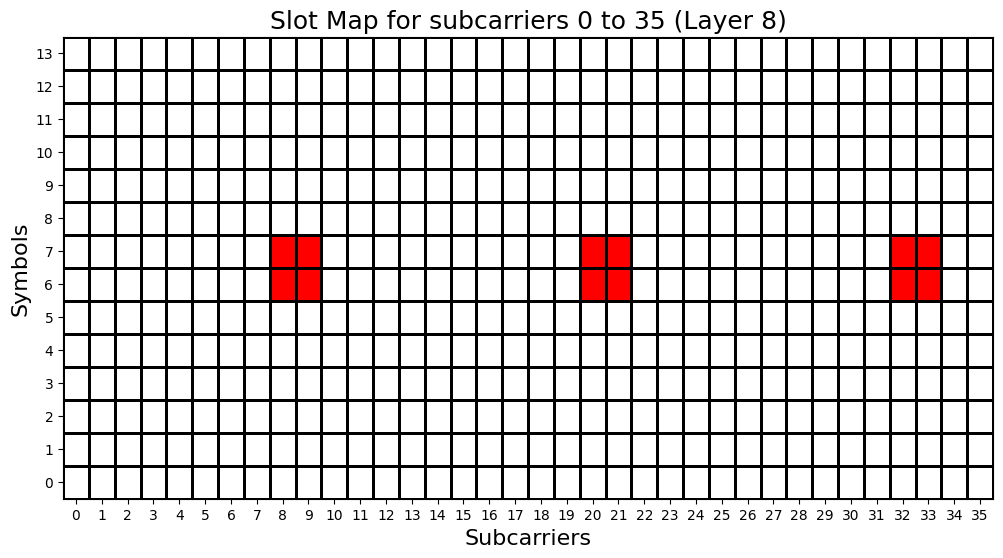
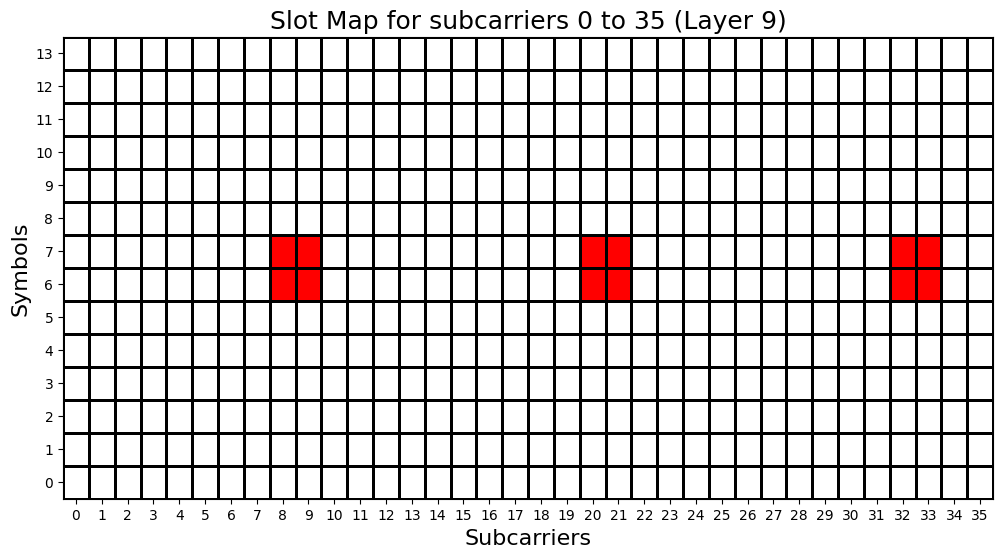
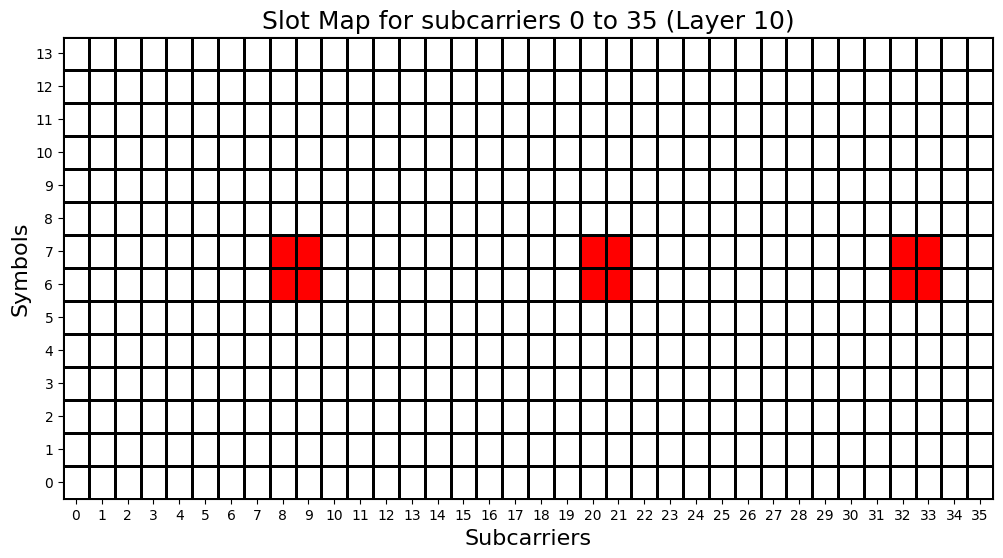
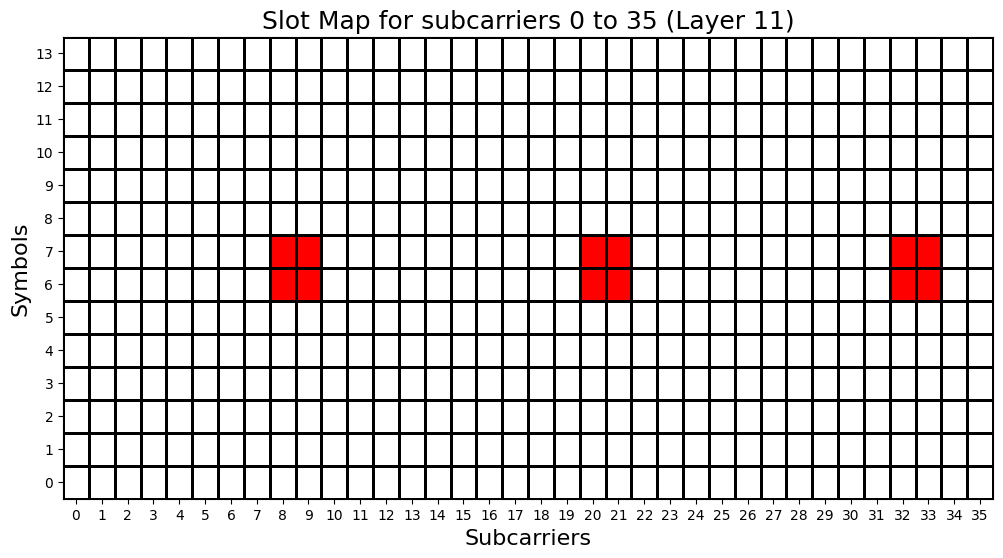
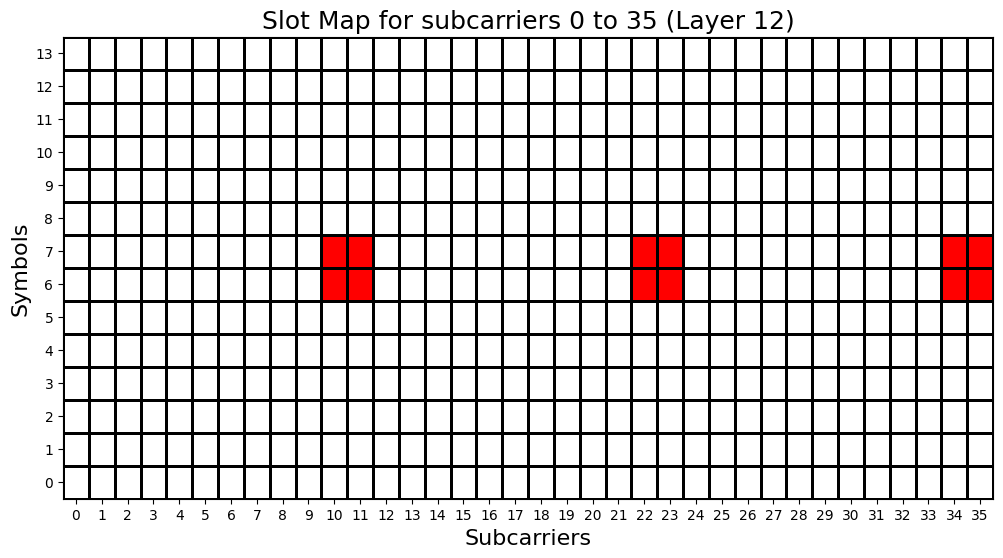
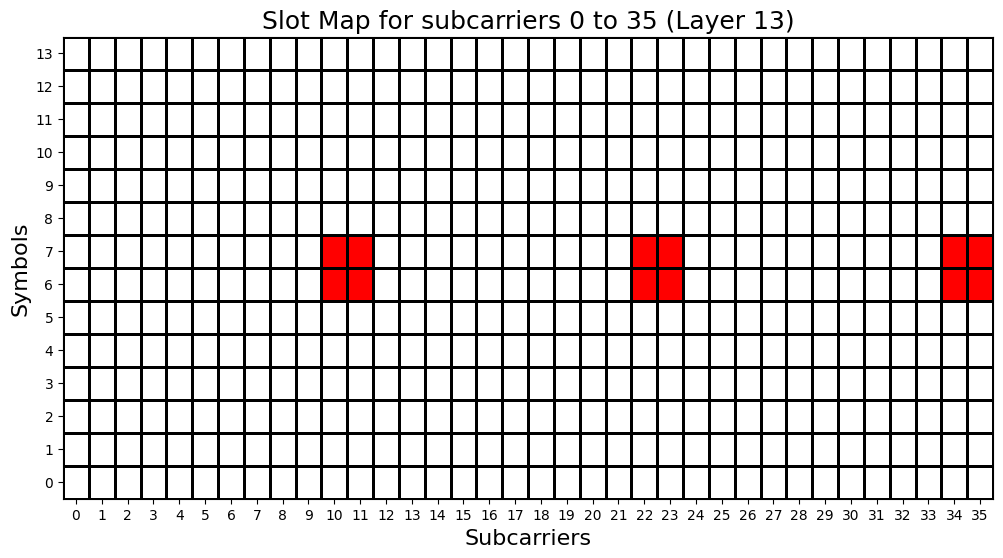
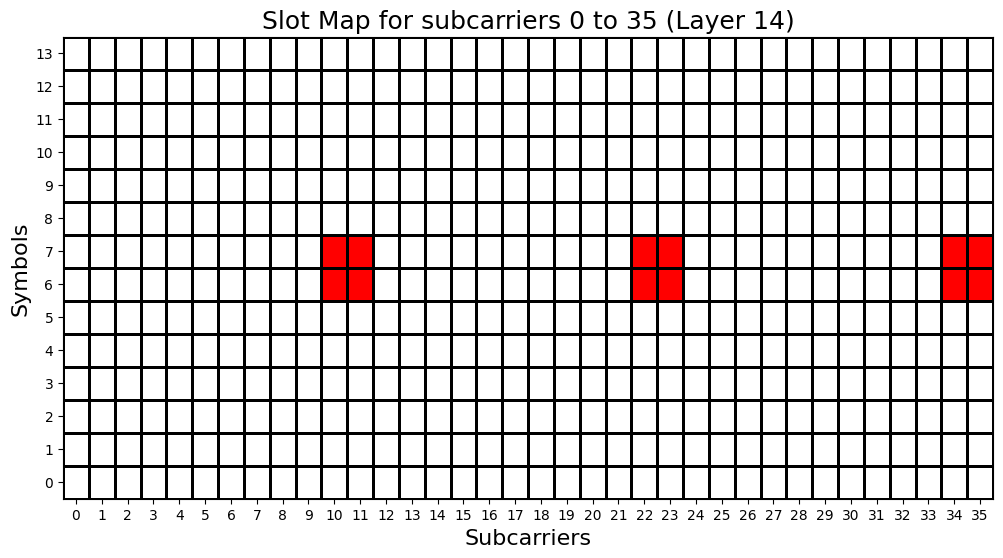
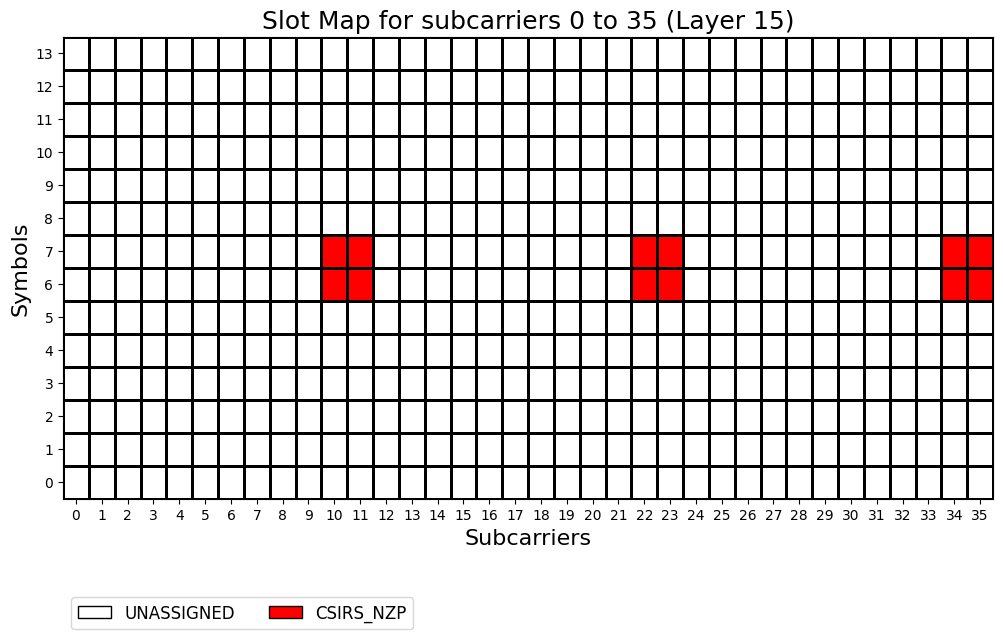
[17]:
# Example for 13th row of 38.211 Table 7.4.1.5.3-1
# 24 ports, 3 of 6 bits set in freqMap, CDM size is 2, and density is 1.
# The CSI-RS resources are at symbols 4, 5, 8, and 9 and REs (2,3), (6,7) and (10,11).
# Note that this configuration also allows density=.5 which would cause every other
# RB to be skipped for the CSR-RS allocation. (default density is 1)
csiRsConfig = CsiRsConfig([CsiRsSet("NZP", bwp, symbols=[4,8], numPorts=24, freqMap="101010", cdmSize=2)])
csiRsConfig.print()
grid = bwp.createGrid(csiRsConfig.numPorts)
csiRsConfig.populateGrid(grid)
print("Grid Stats:")
stats = grid.getStats()
for key, value in stats.items(): print(" %s: %d"%(key, value))
grid.drawMap(ports=range(csiRsConfig.numPorts), reRange=(0,36))
CSI-RS Configuration: (1 Resource Sets)
CSI-RS Resource Set:(1 NZP Resources)
Resource Set ID: 0
Resource Type: periodic
Resource Blocks: 24 RBs starting at 0
Slot Period: 4
Bandwidth Part:
Resource Blocks: 24 RBs starting at 0 (288 subcarriers)
Subcarrier Spacing: 15 KHz
CP Type: normal
bandwidth: 4.32 MHz
symbolsPerSlot: 14
slotsPerSubFrame: 1
nFFT: 2048
frameNo: 0
slotNo: 0
CSI-RS:
resourceId: 0
numPorts: 24
cdmSize: 2 (fd-CDM2)
density: 1
RE Indexes: 2 6 10
Symbol Indexes: 4 8
Table Row: 13
Slot Offset: 0
Power: 0 db
scramblingID: 0
Grid Stats:
GridSize: 96768
UNASSIGNED: 95616
CSIRS_NZP: 1152
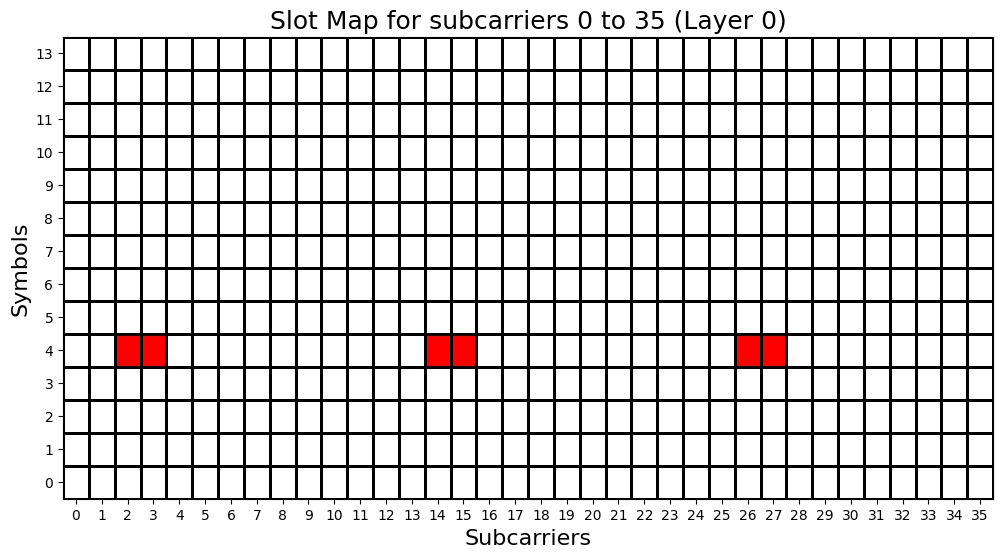
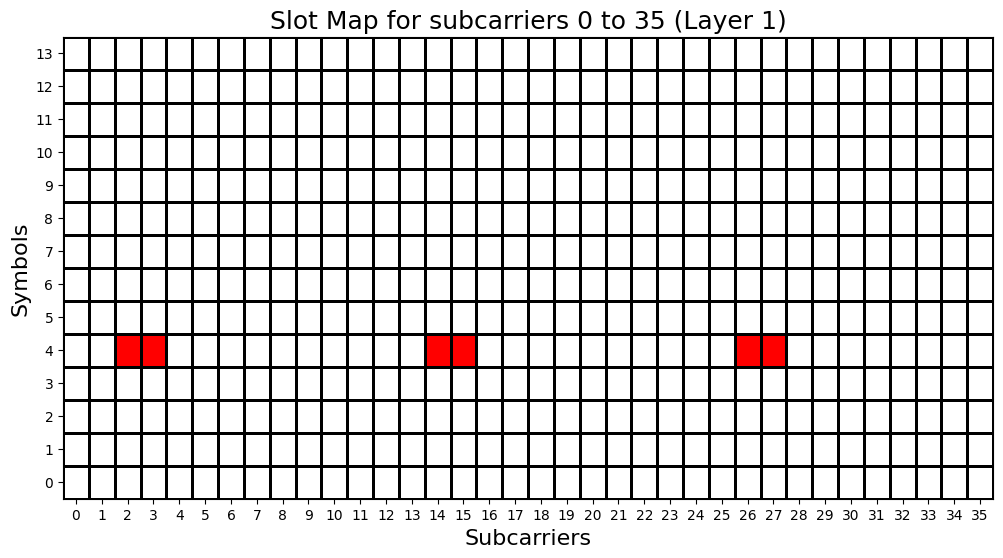
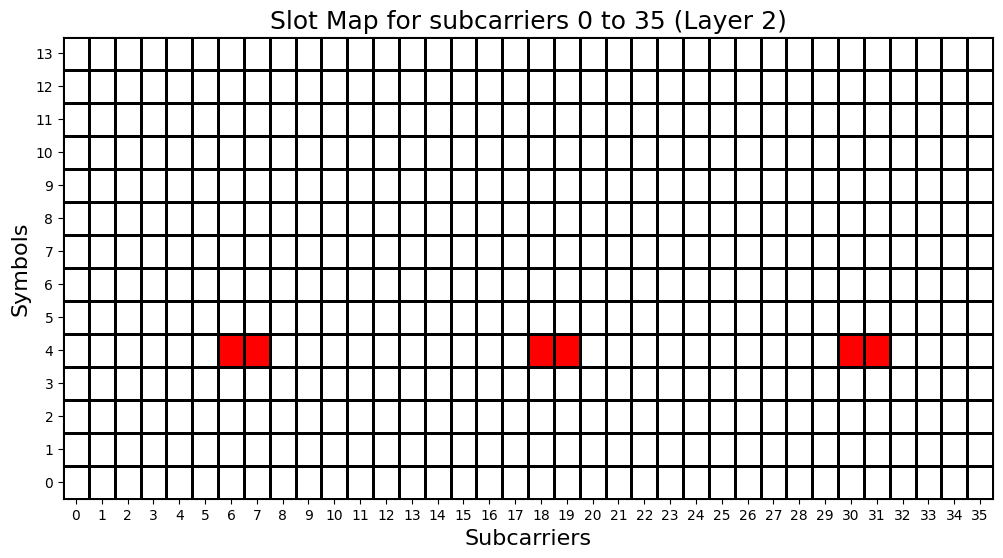
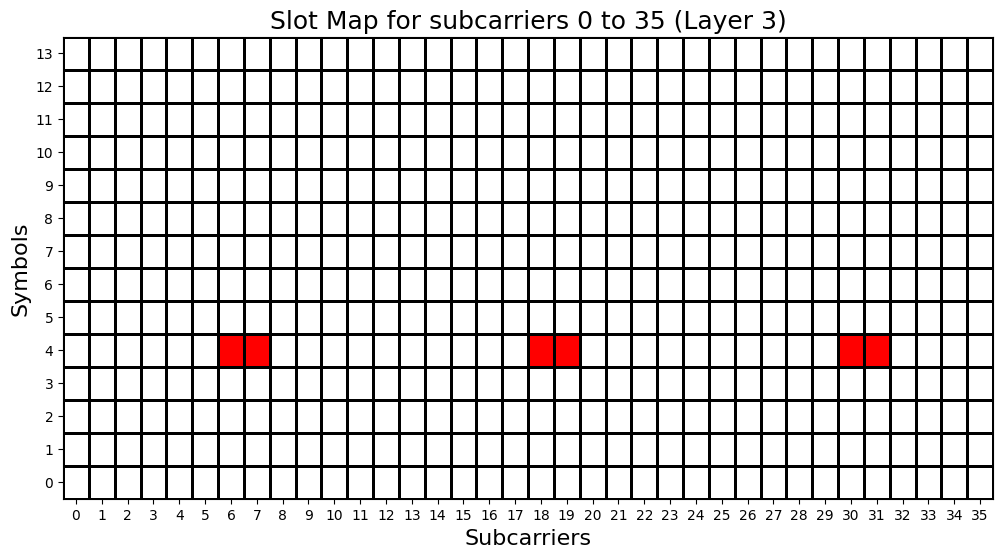
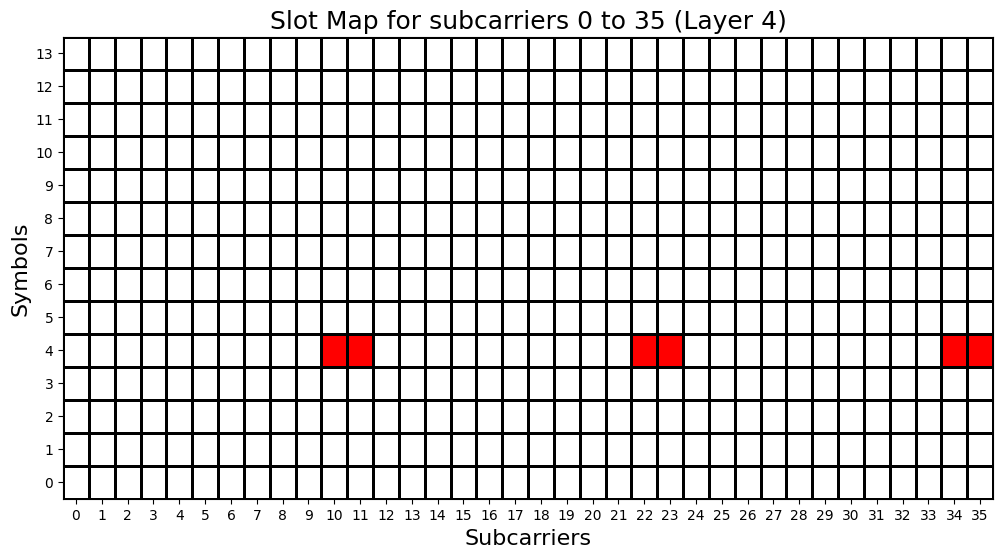
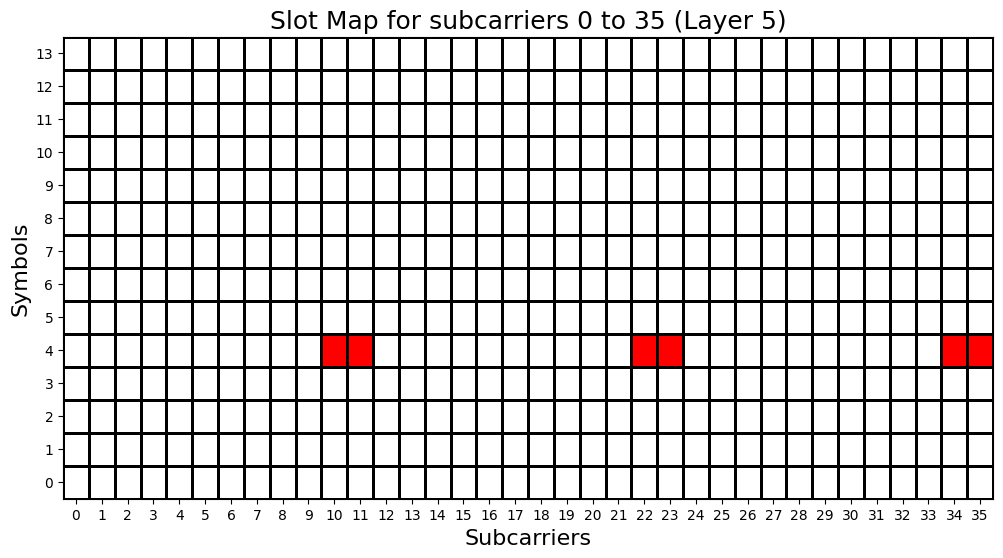
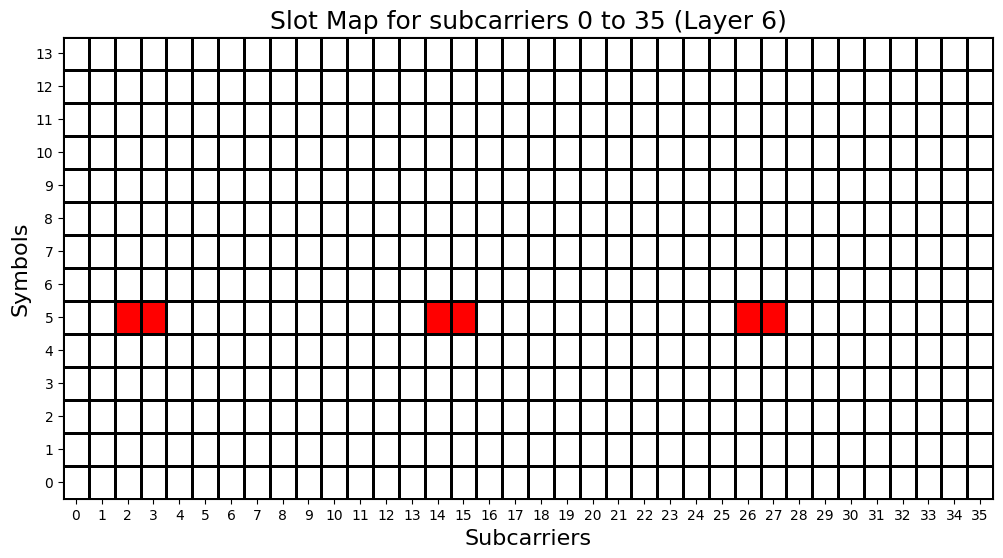
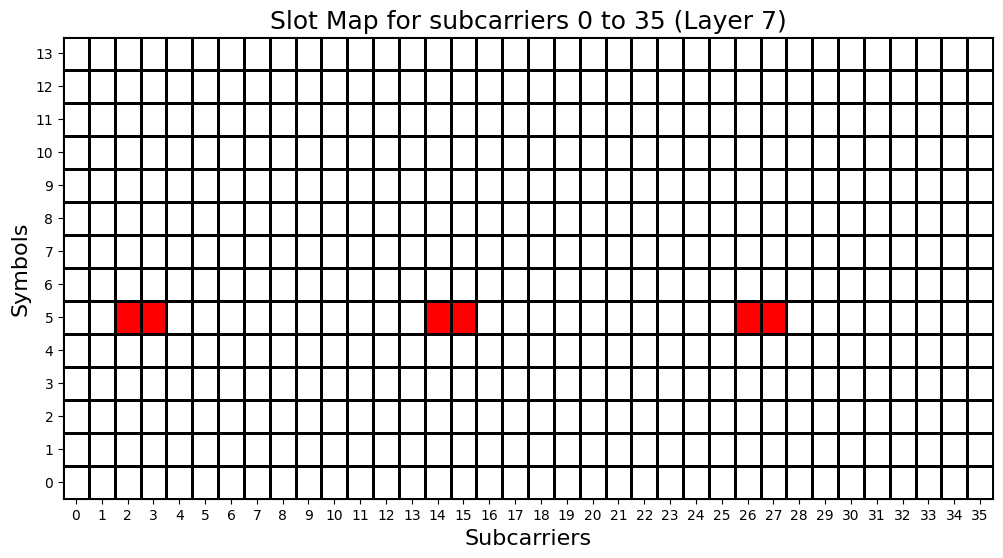
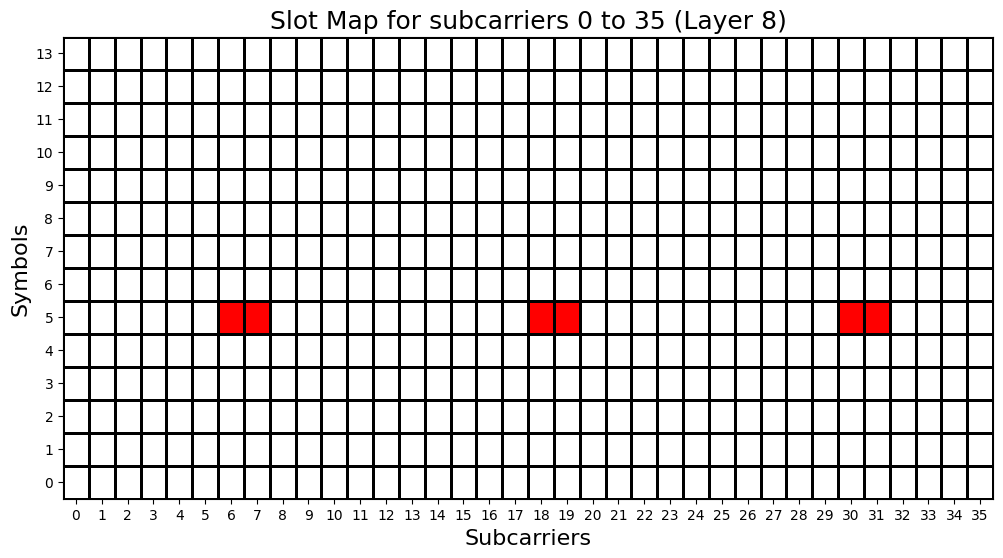
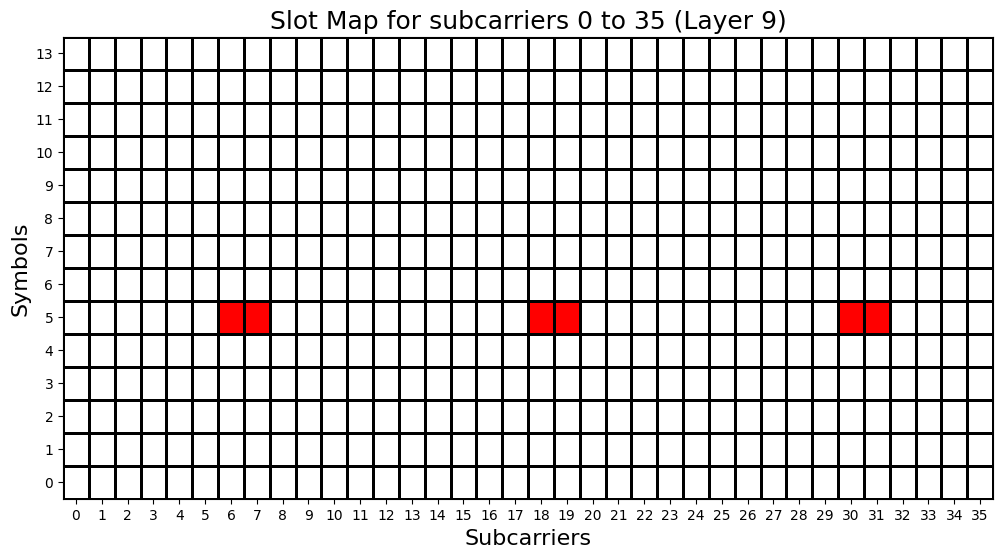
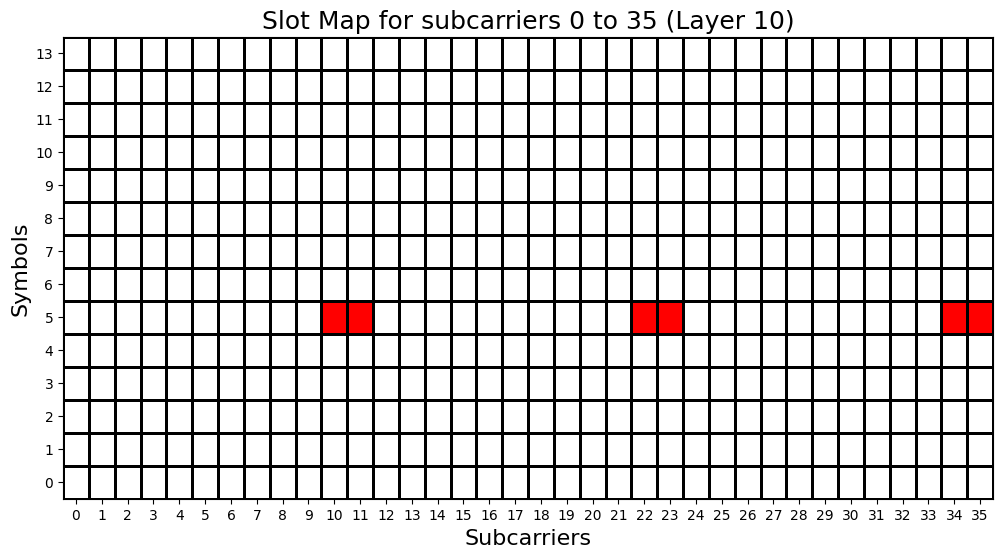
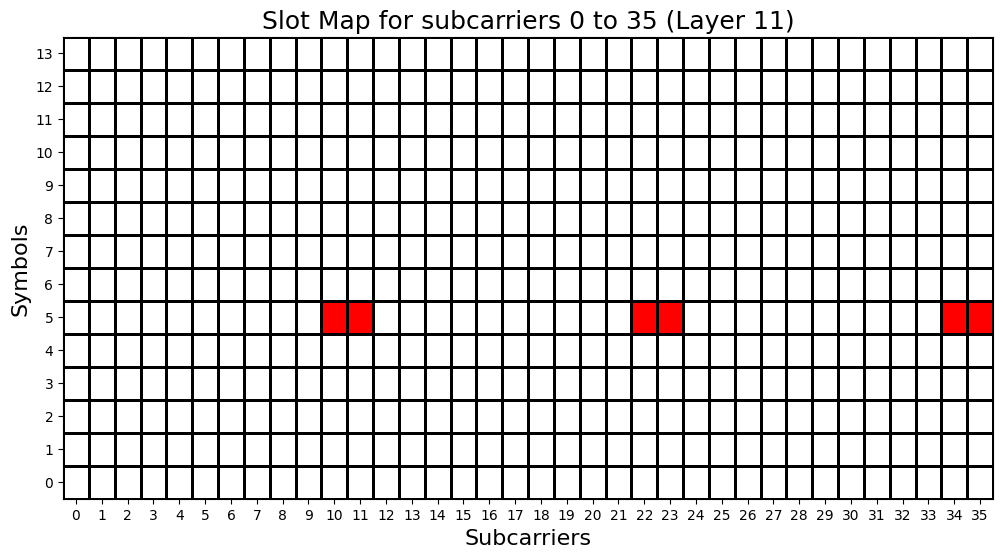
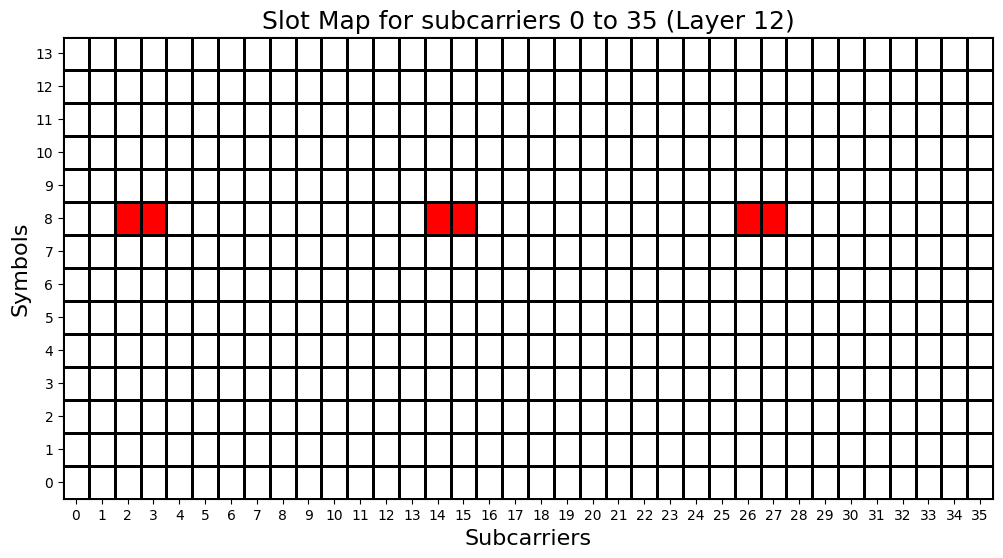
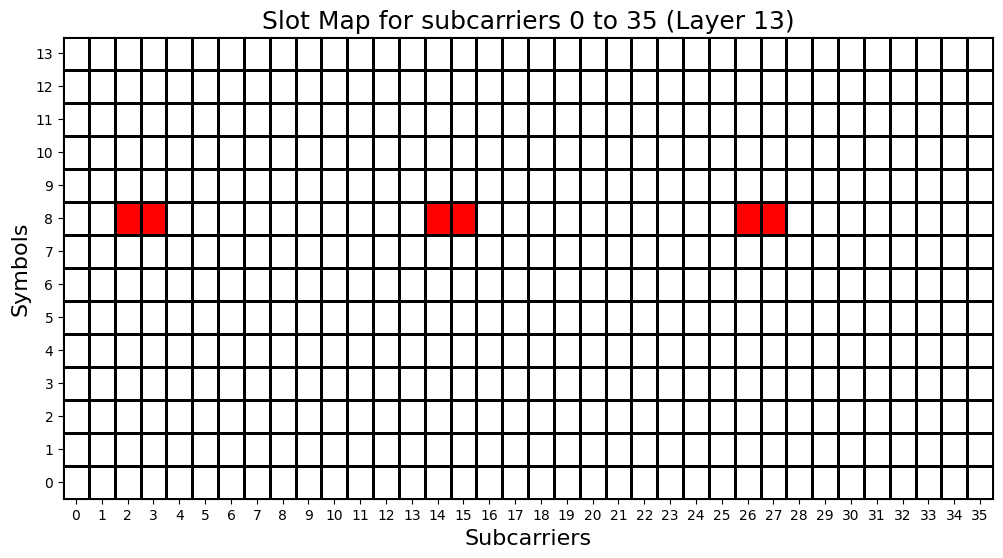
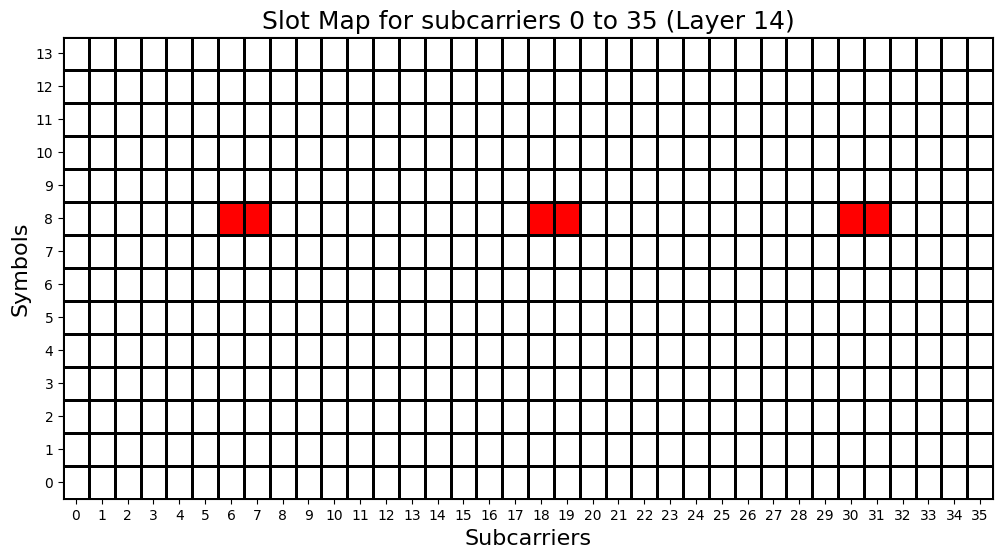
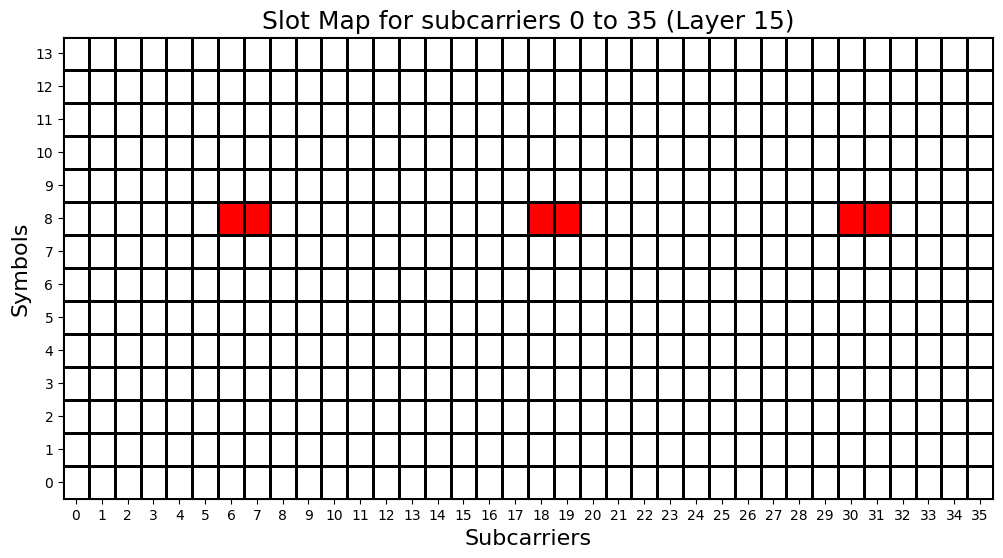
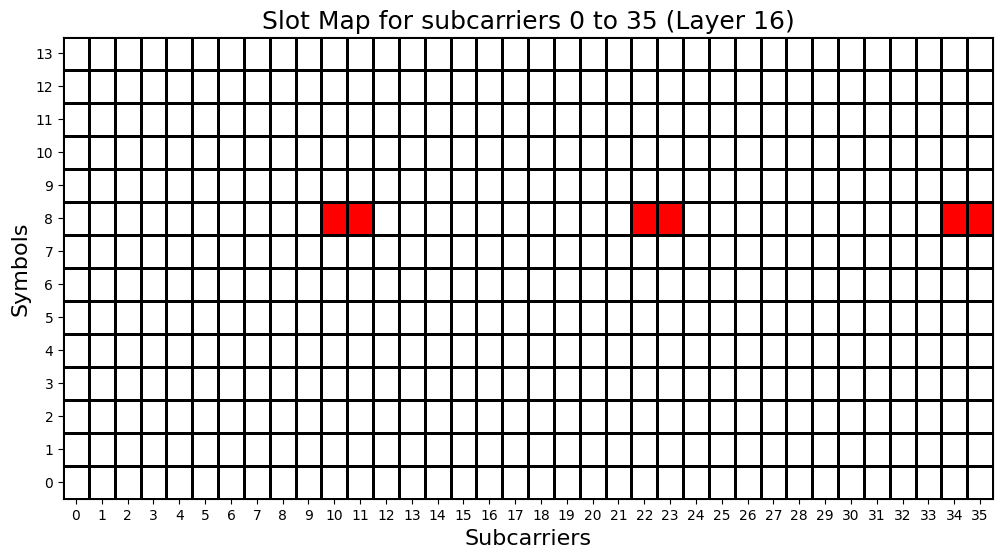
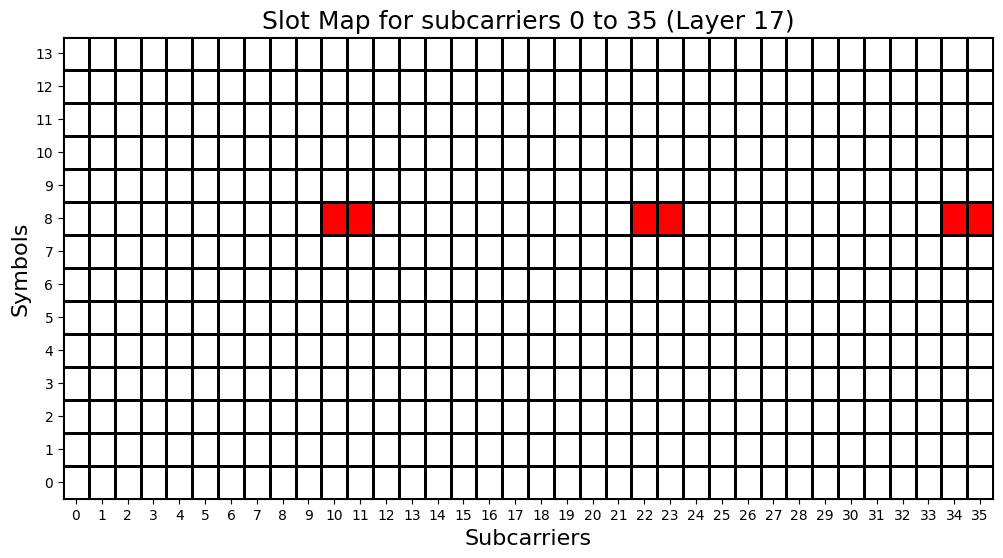
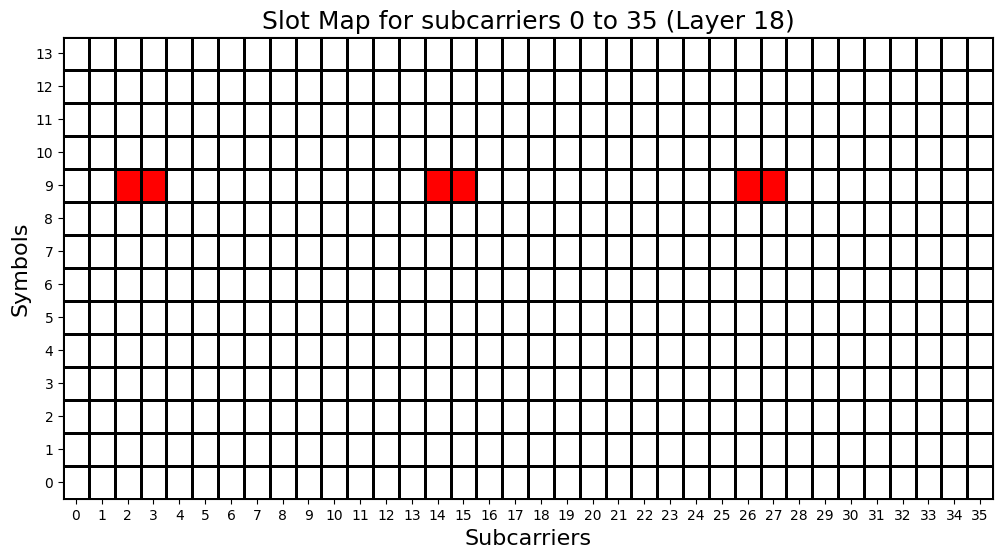
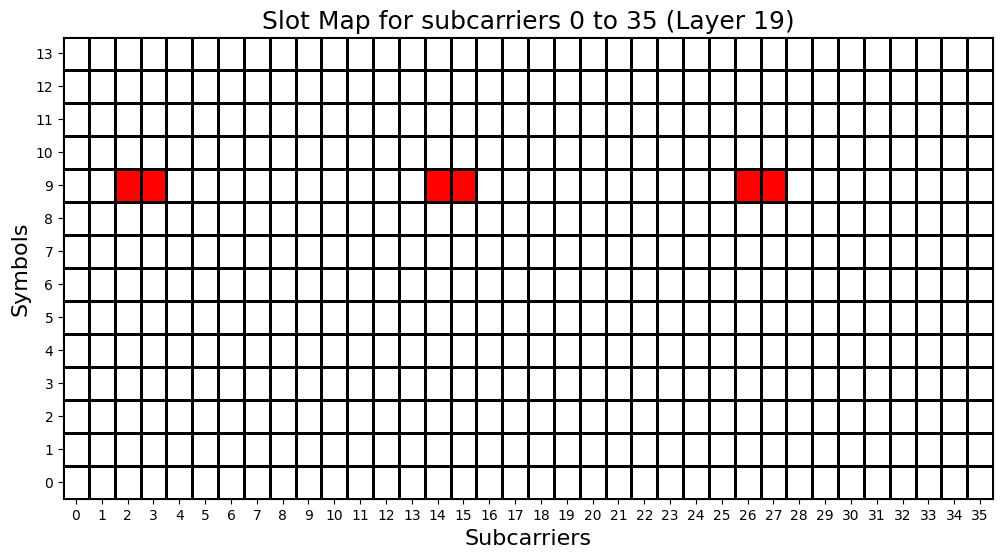
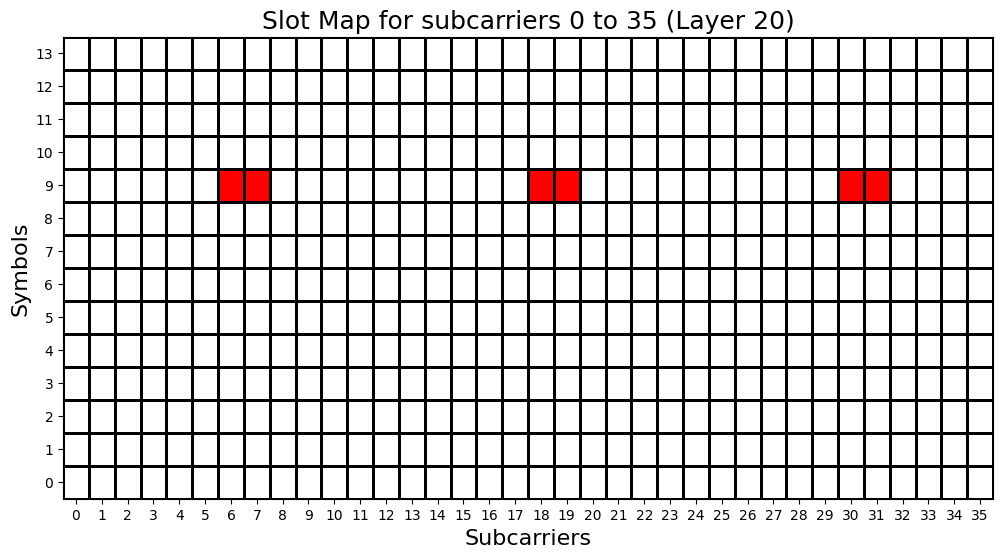
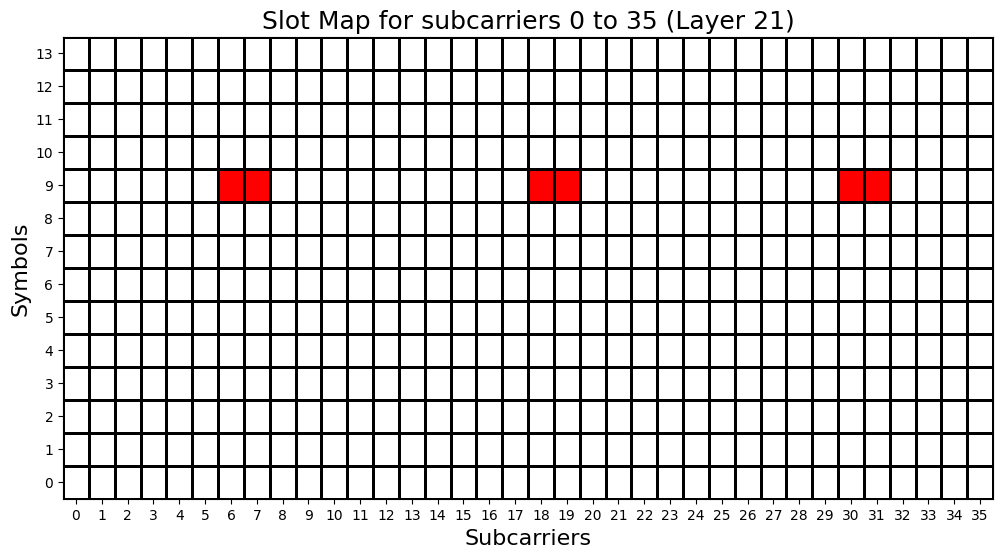
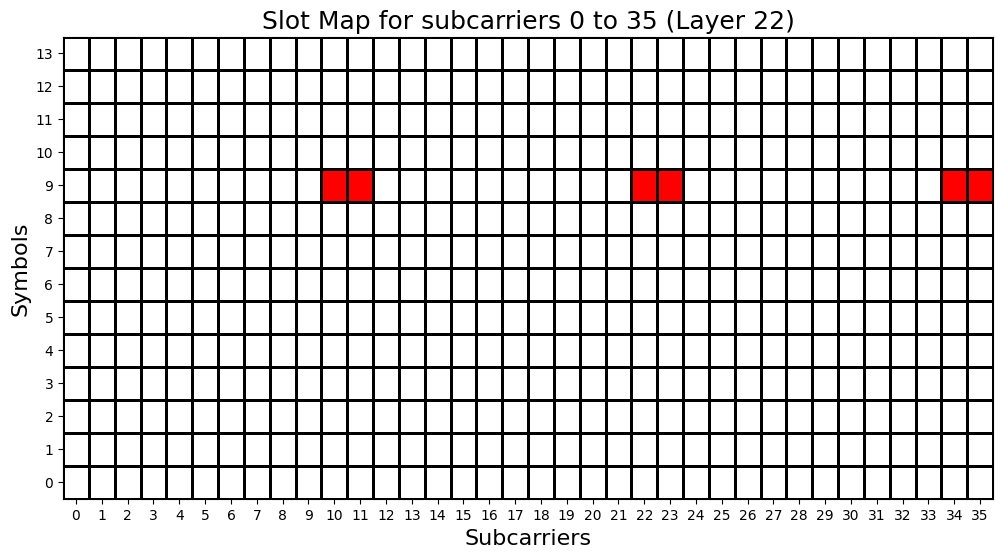
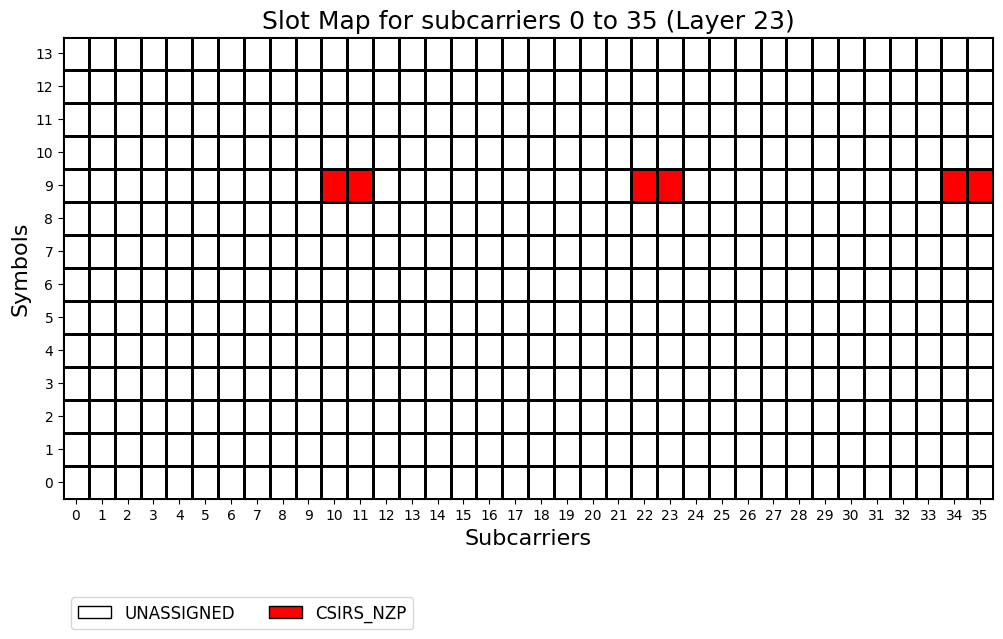
[18]:
# Example for 14th row of 38.211 Table 7.4.1.5.3-1
# 24 ports, 3 of 6 bits set in freqMap, CDM size is 4, and density is 1.
# The CSI-RS resources are at symbols (4,5), (8,9) and REs (2,3), (6,7) and (10,11).
# Note that this configuration also allows density=.5 which would cause every other
# RB to be skipped for the CSR-RS allocation. (default density is 1)
csiRsConfig = CsiRsConfig([CsiRsSet("NZP", bwp, symbols=[4,8], numPorts=24, freqMap="101010", cdmSize=4)])
csiRsConfig.print()
grid = bwp.createGrid(csiRsConfig.numPorts)
csiRsConfig.populateGrid(grid)
print("Grid Stats:")
stats = grid.getStats()
for key, value in stats.items(): print(" %s: %d"%(key, value))
grid.drawMap(ports=range(csiRsConfig.numPorts), reRange=(0,36))
CSI-RS Configuration: (1 Resource Sets)
CSI-RS Resource Set:(1 NZP Resources)
Resource Set ID: 0
Resource Type: periodic
Resource Blocks: 24 RBs starting at 0
Slot Period: 4
Bandwidth Part:
Resource Blocks: 24 RBs starting at 0 (288 subcarriers)
Subcarrier Spacing: 15 KHz
CP Type: normal
bandwidth: 4.32 MHz
symbolsPerSlot: 14
slotsPerSubFrame: 1
nFFT: 2048
frameNo: 0
slotNo: 0
CSI-RS:
resourceId: 0
numPorts: 24
cdmSize: 4 (cdm4-FD2-TD2)
density: 1
RE Indexes: 2 6 10
Symbol Indexes: 4 8
Table Row: 14
Slot Offset: 0
Power: 0 db
scramblingID: 0
Grid Stats:
GridSize: 96768
UNASSIGNED: 94464
CSIRS_NZP: 2304
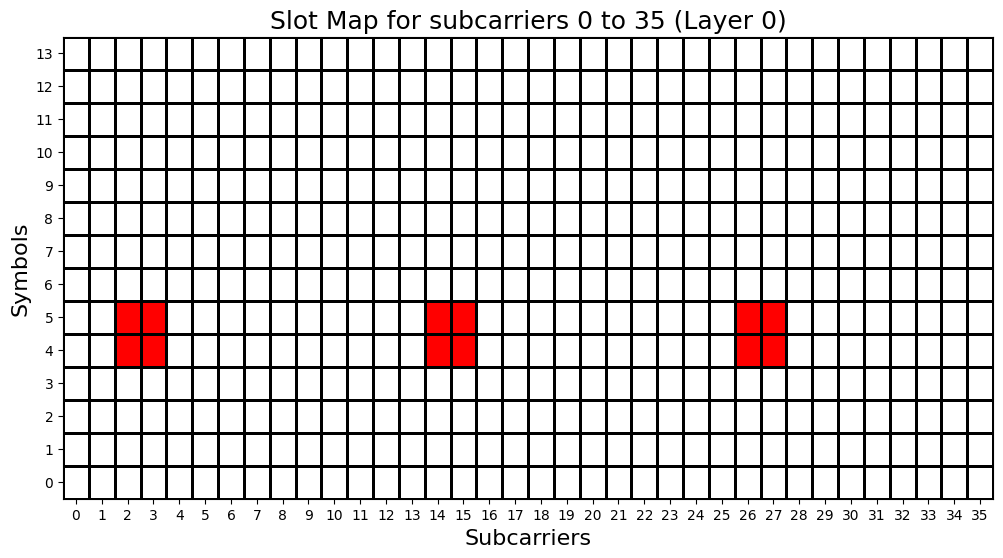
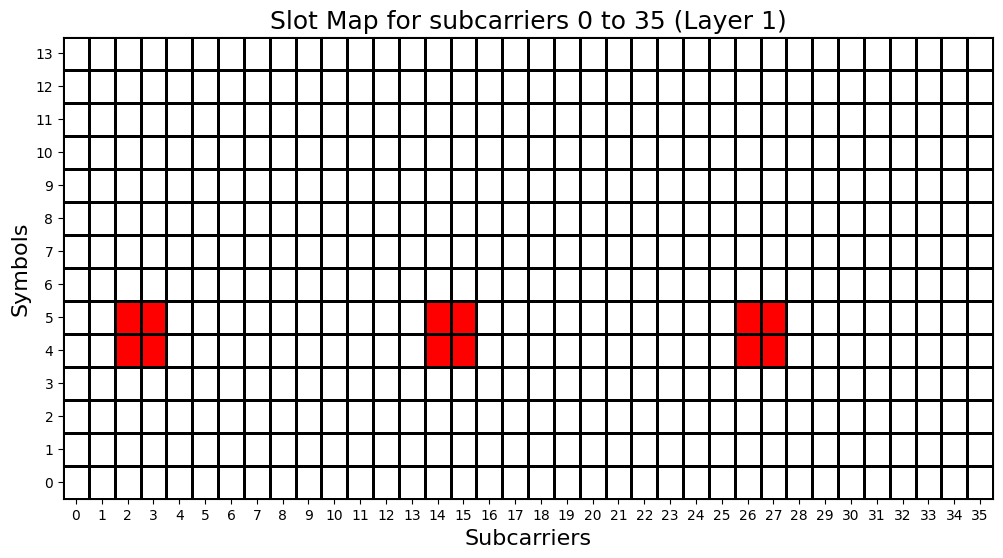
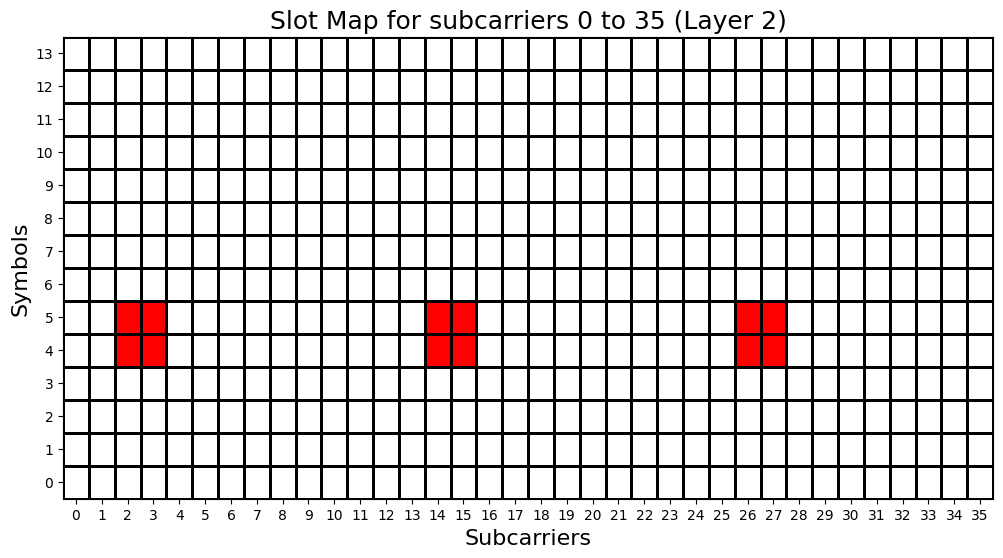
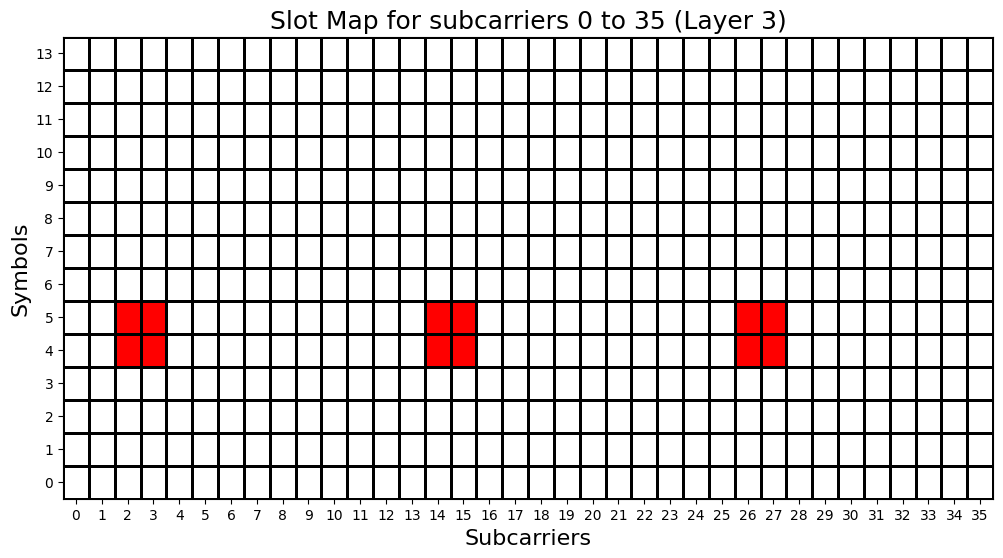
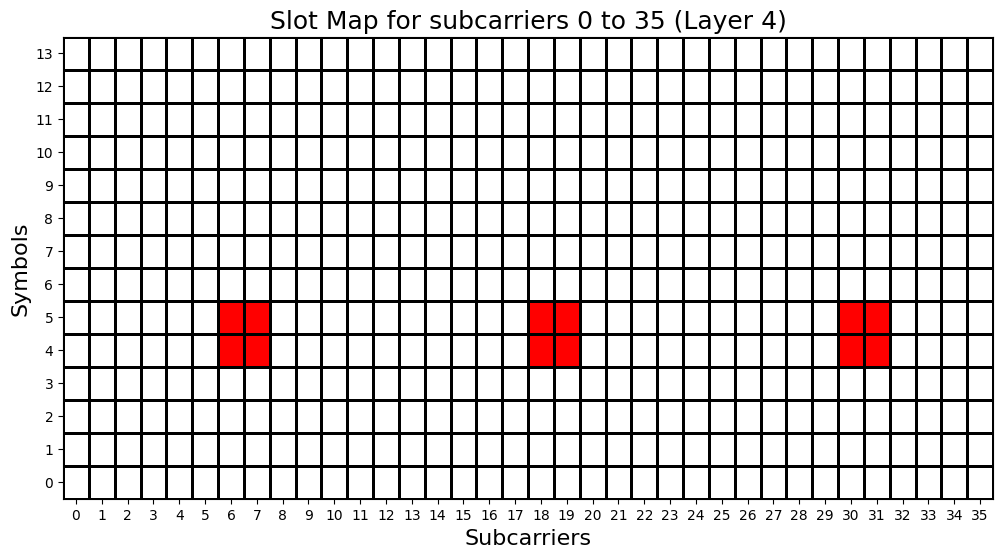
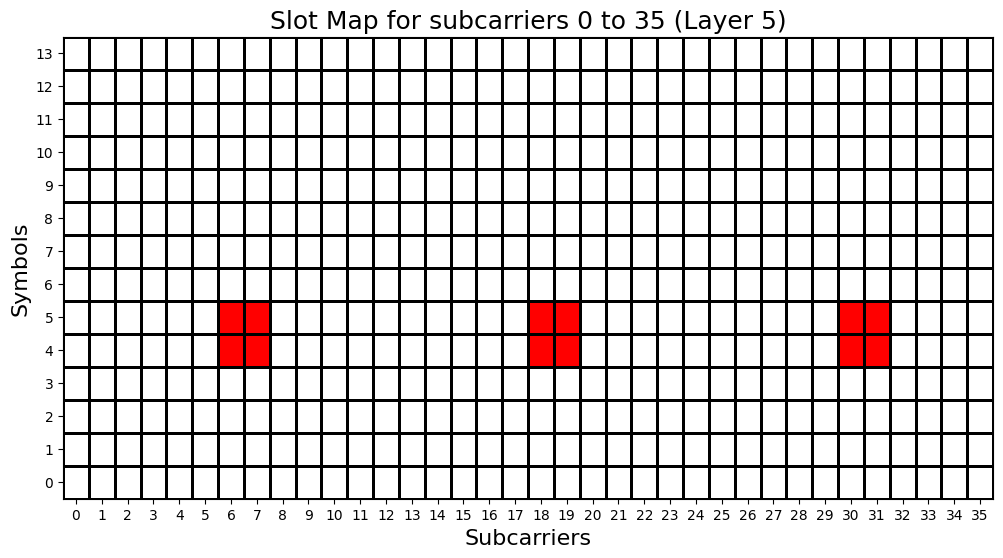
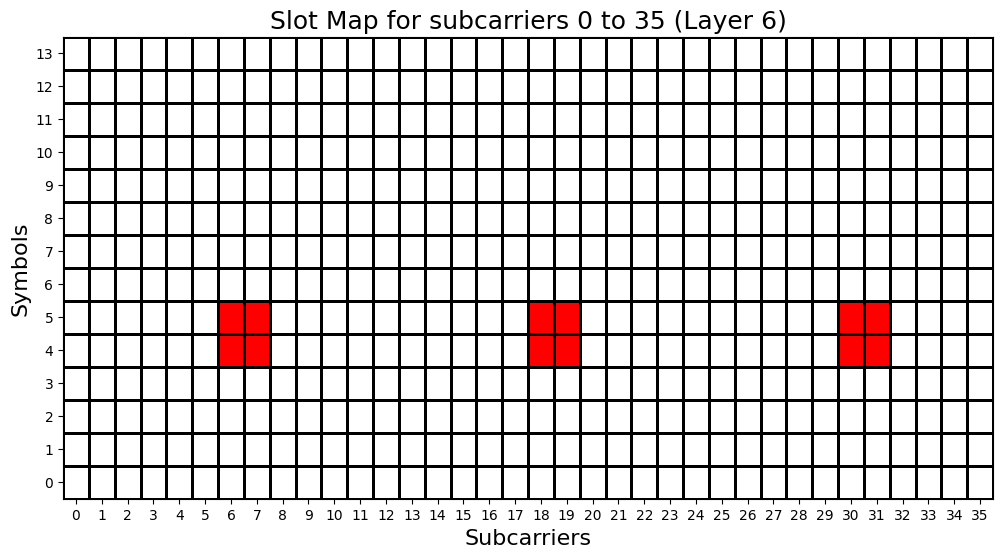
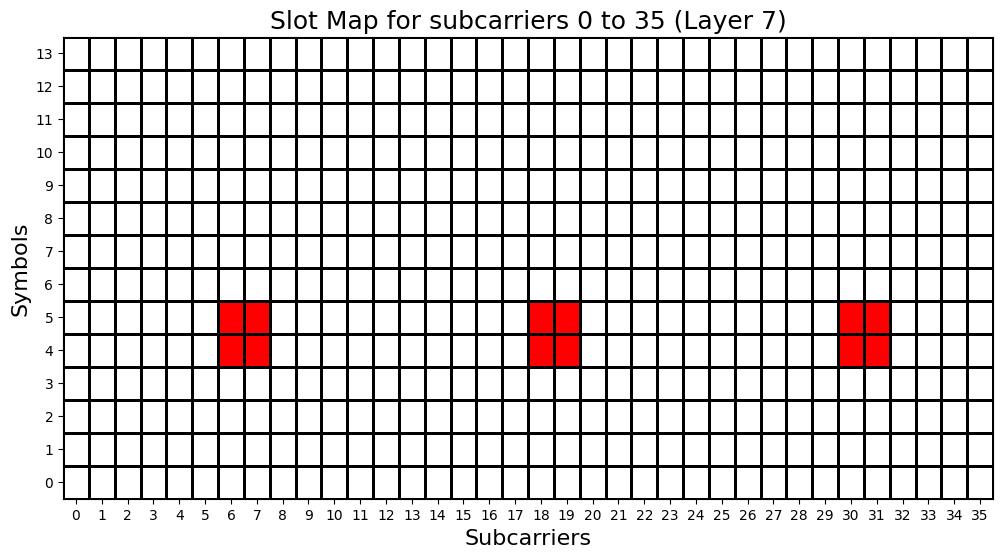
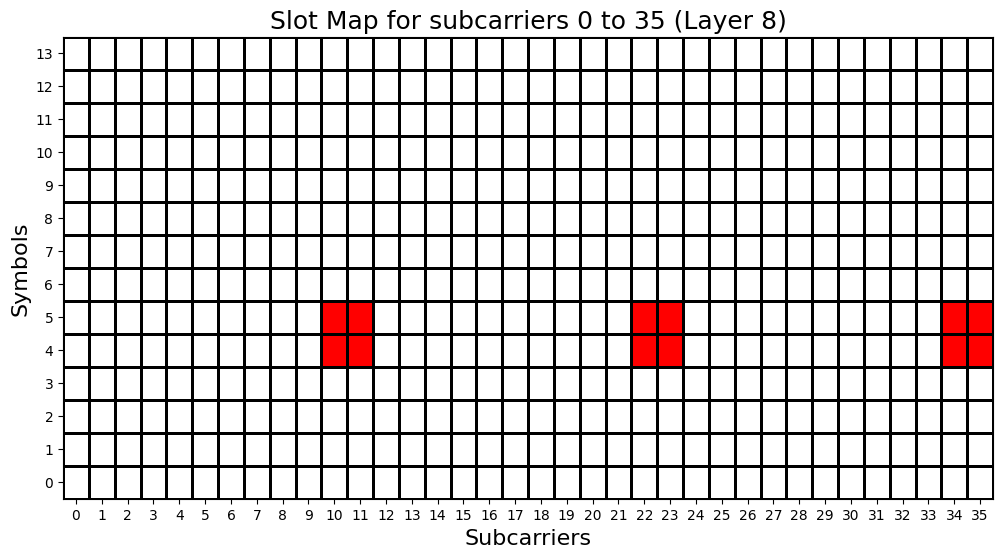
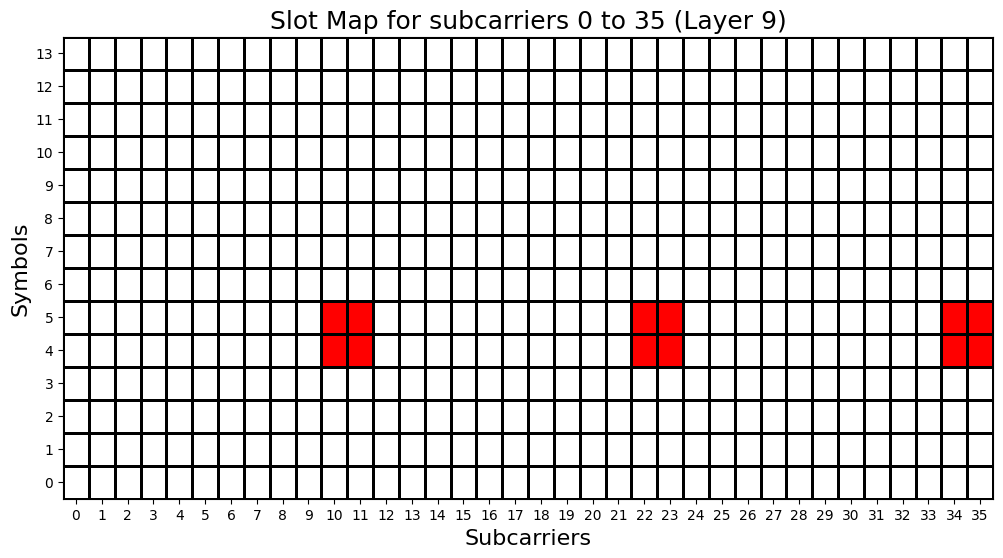
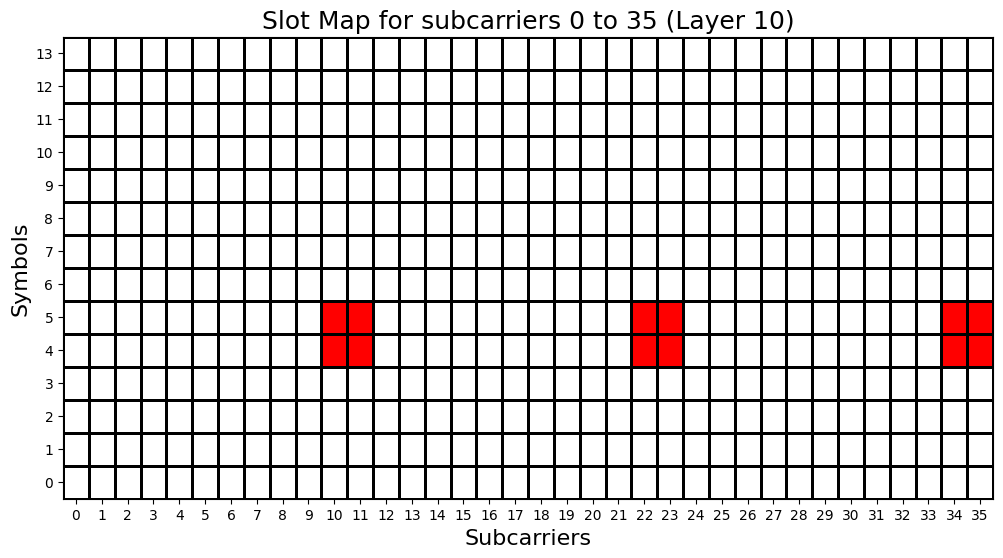
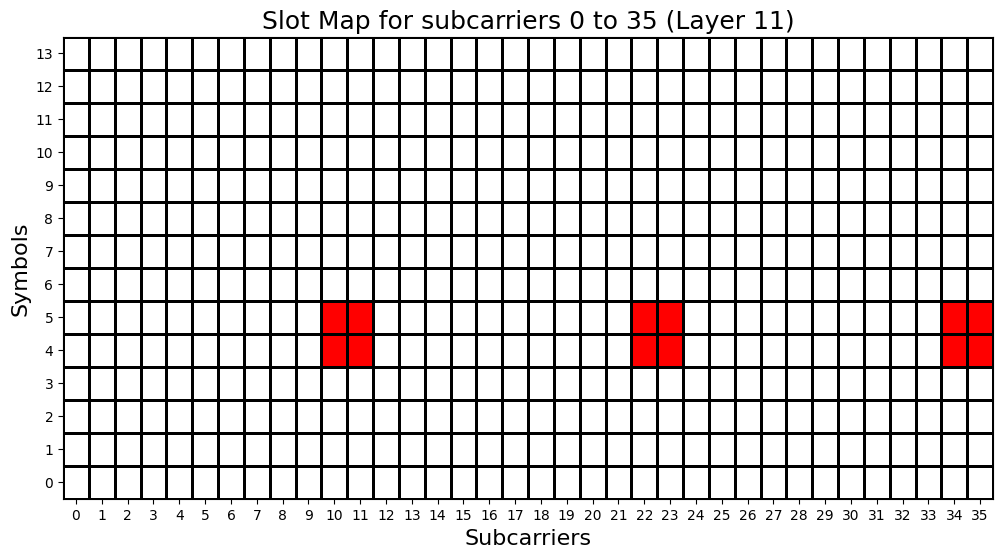
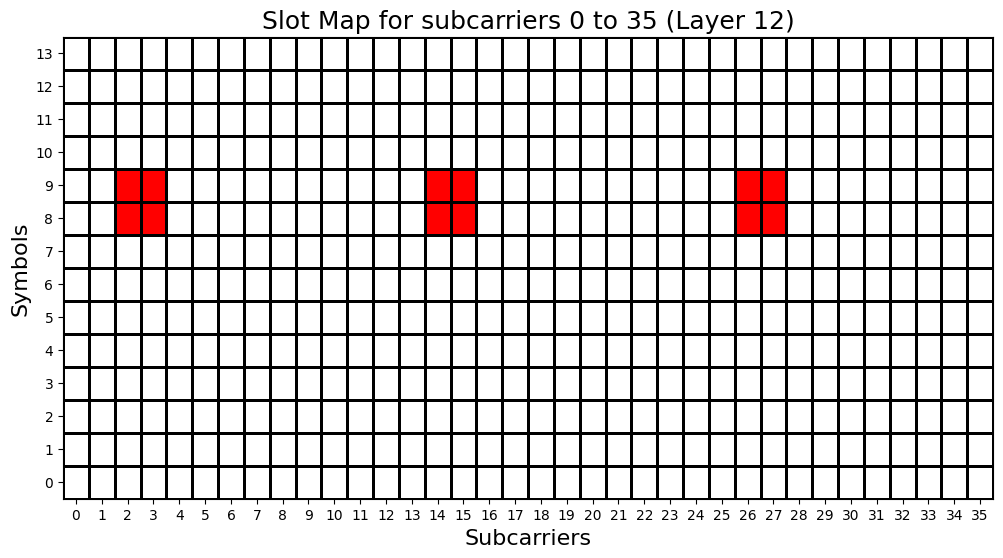
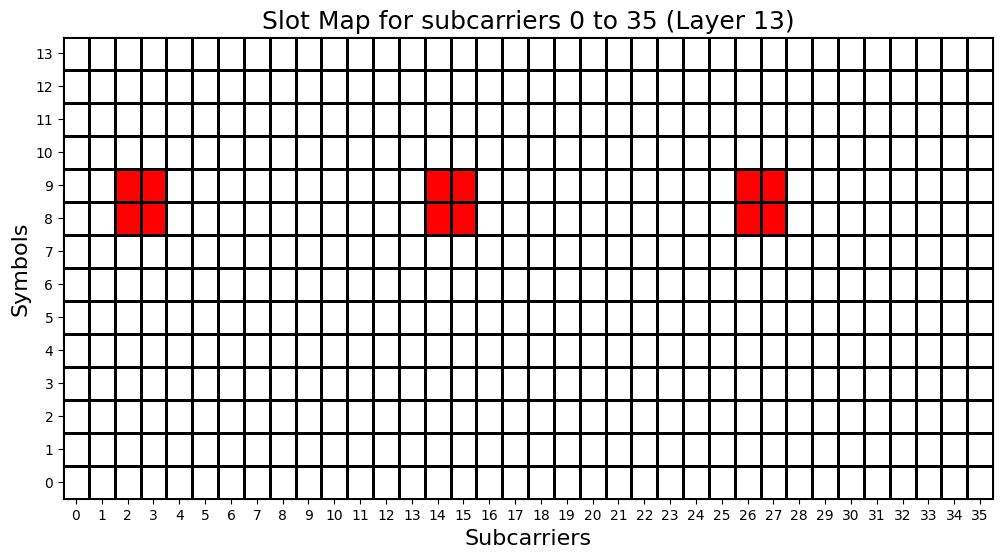
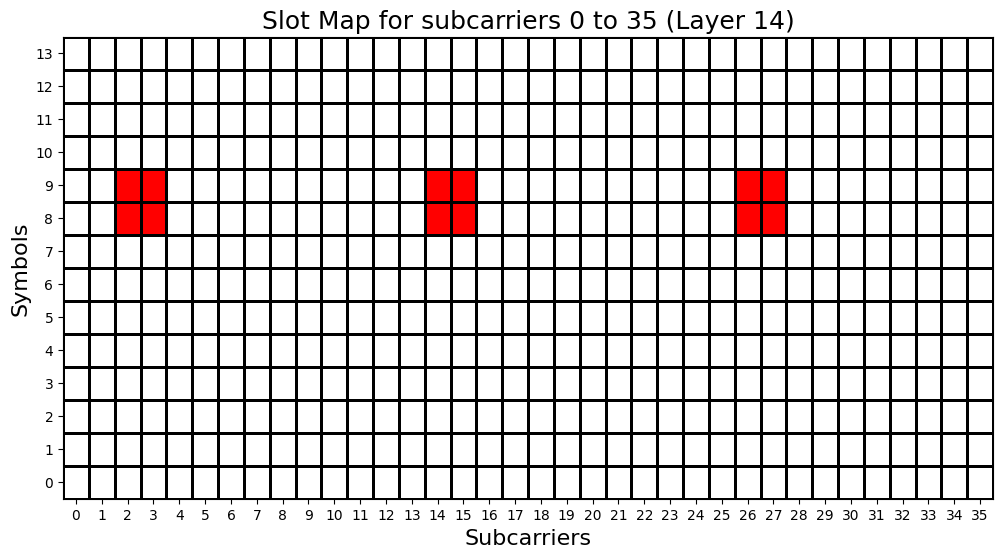
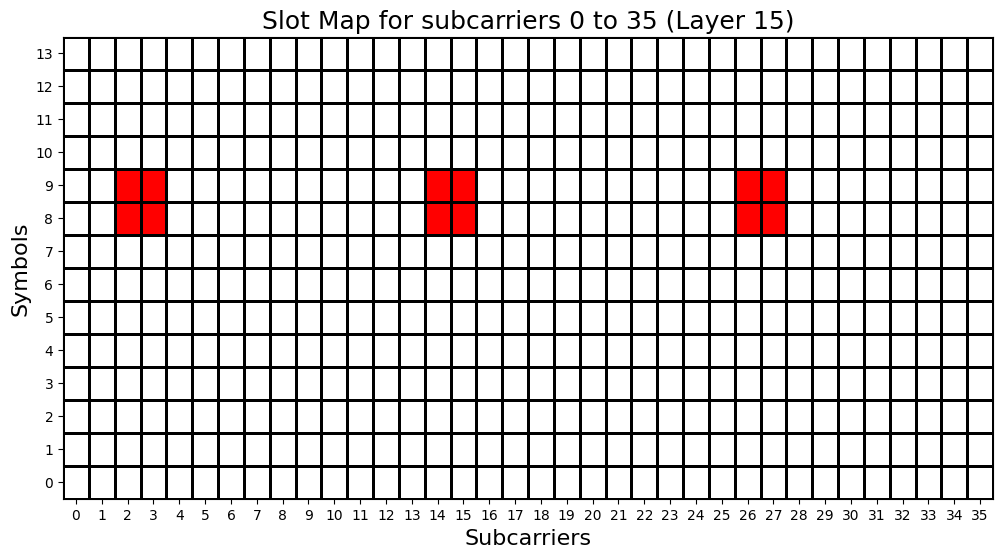
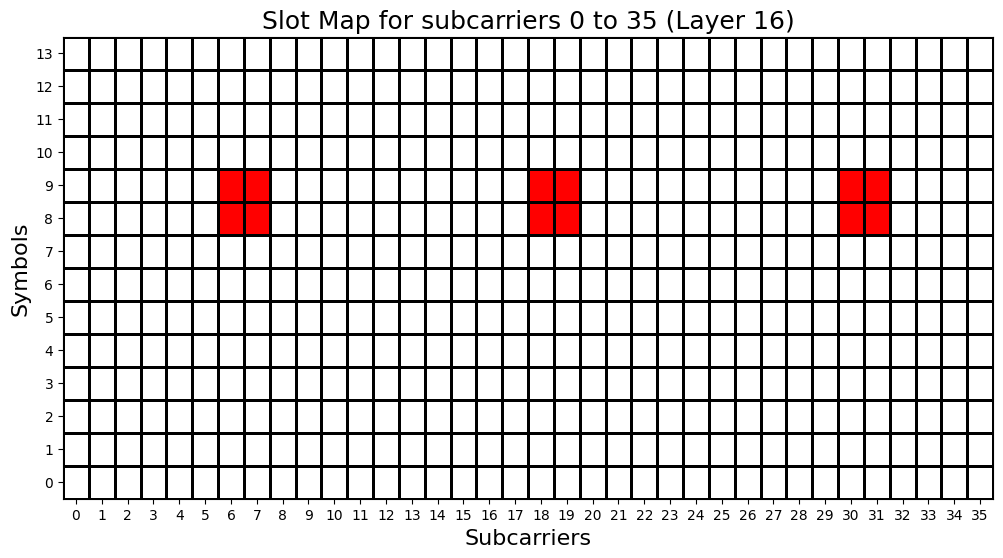
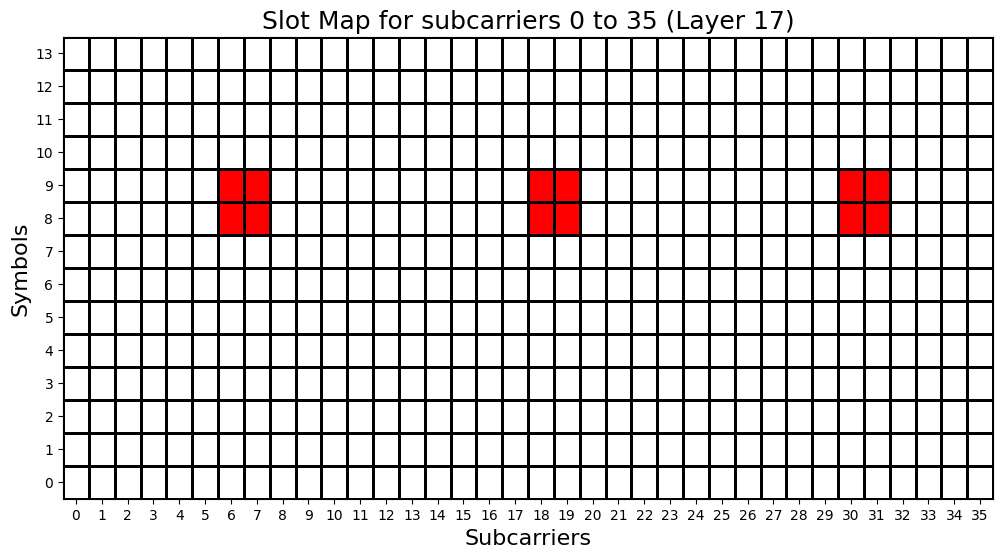
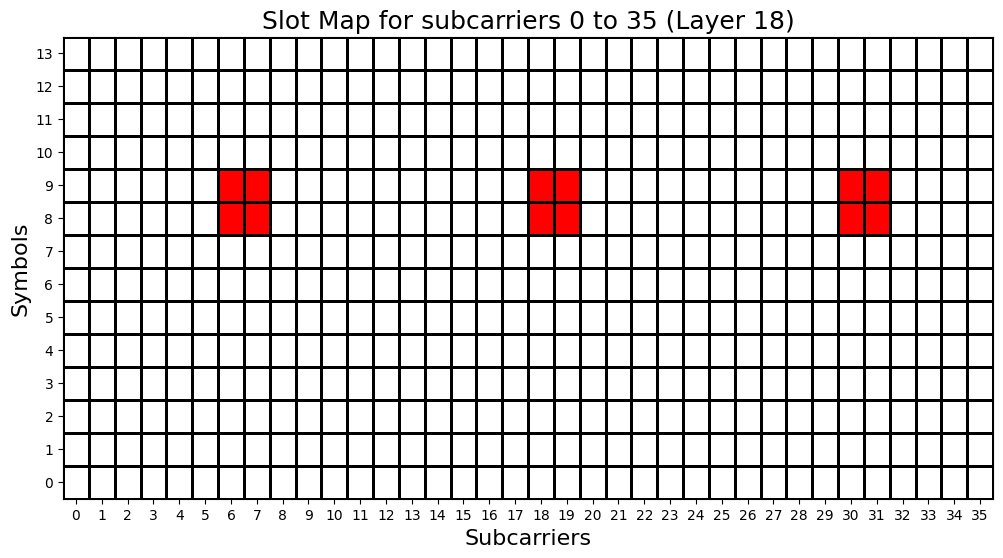
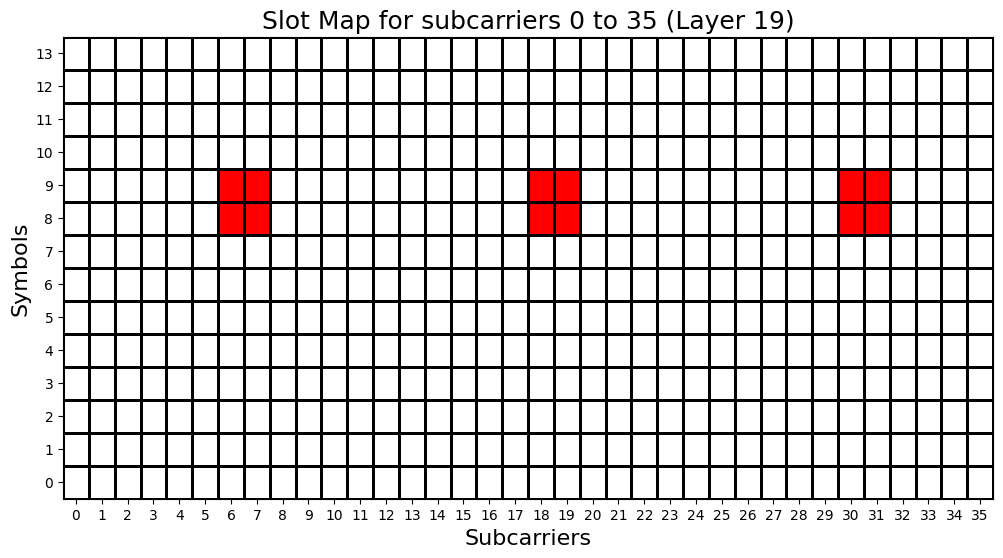
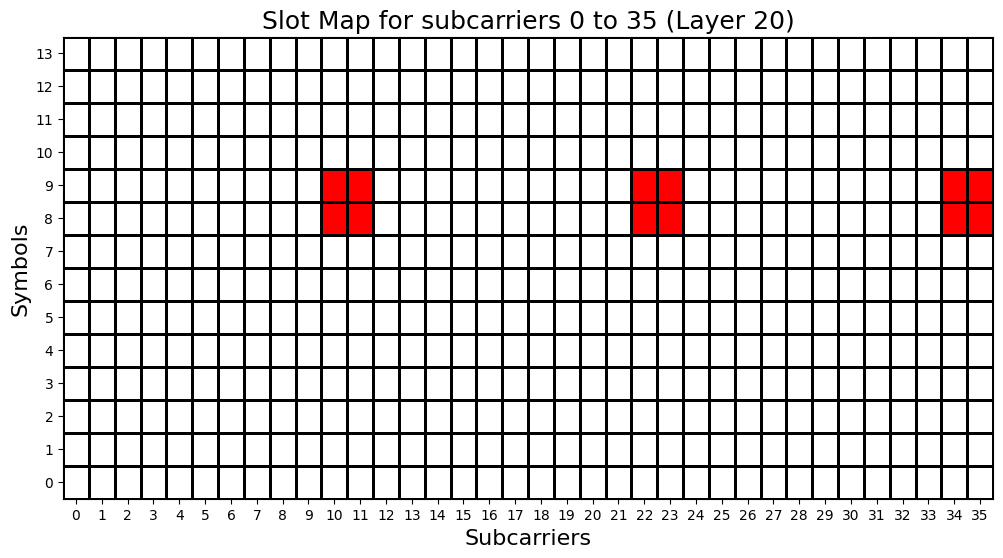
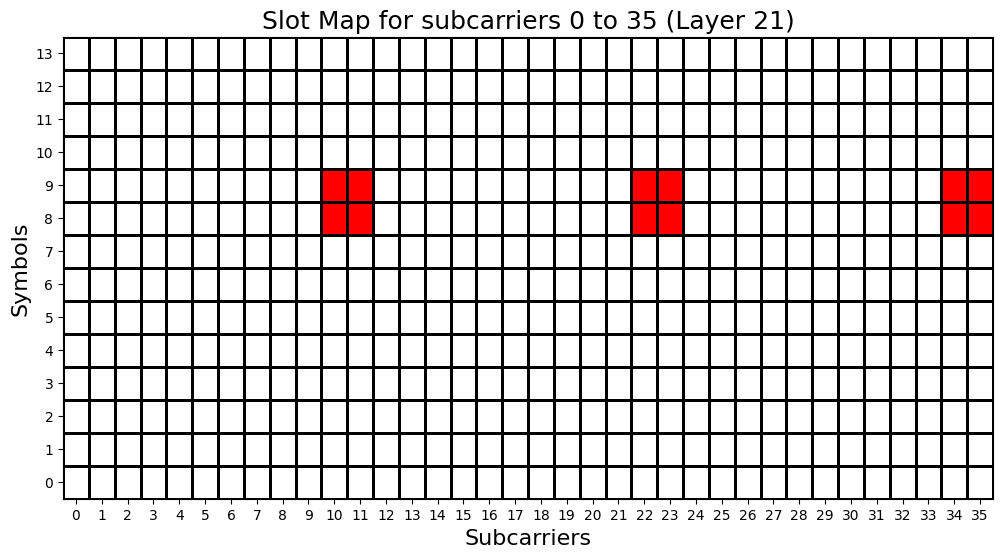
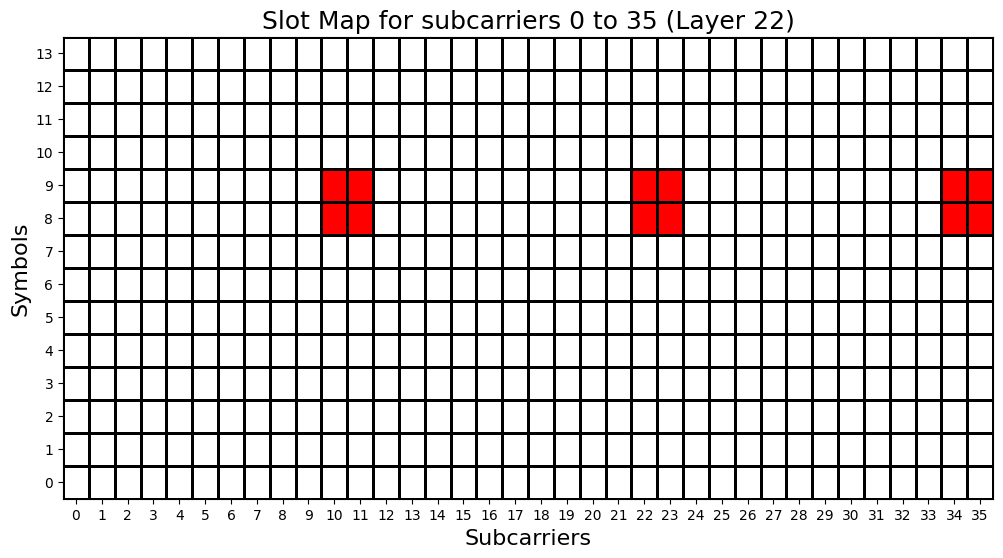
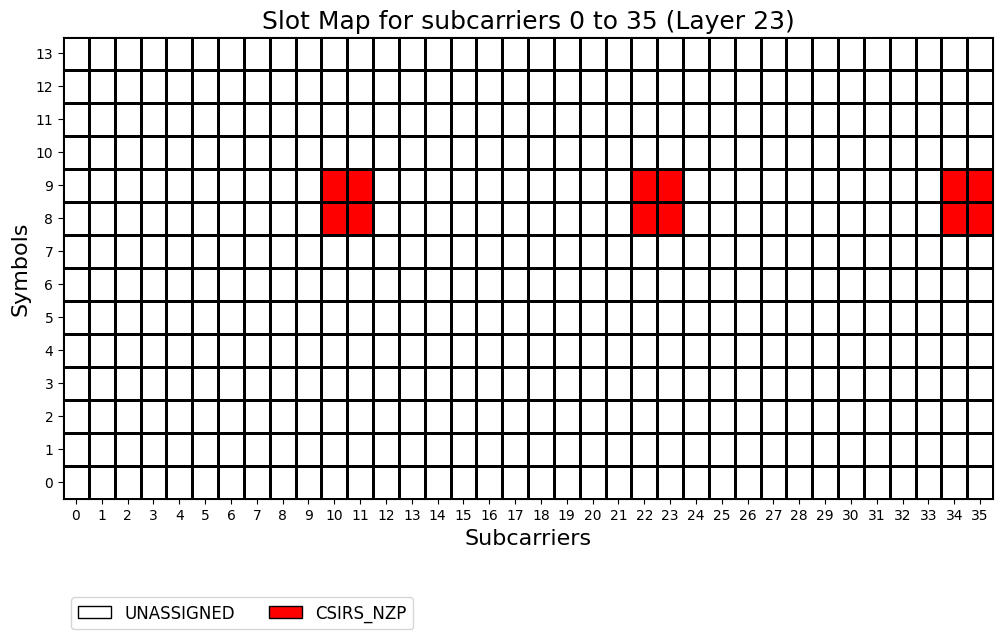
[19]:
# Example for 15th row of 38.211 Table 7.4.1.5.3-1
# 24 ports, 3 of 6 bits set in freqMap, CDM size is 8, and density is 1.
# The CSI-RS resources are at symbols (5,6,7,8) and REs (2,3), (6,7) and (10,11).
# Note that this configuration also allows density=.5 which would cause every other
# RB to be skipped for the CSR-RS allocation. (default density is 1)
csiRsConfig = CsiRsConfig([CsiRsSet("NZP", bwp, symbols=[5], numPorts=24, freqMap="101010", cdmSize=8)])
csiRsConfig.print()
grid = bwp.createGrid(csiRsConfig.numPorts)
csiRsConfig.populateGrid(grid)
print("Grid Stats:")
stats = grid.getStats()
for key, value in stats.items(): print(" %s: %d"%(key, value))
grid.drawMap(ports=range(csiRsConfig.numPorts), reRange=(0,36))
CSI-RS Configuration: (1 Resource Sets)
CSI-RS Resource Set:(1 NZP Resources)
Resource Set ID: 0
Resource Type: periodic
Resource Blocks: 24 RBs starting at 0
Slot Period: 4
Bandwidth Part:
Resource Blocks: 24 RBs starting at 0 (288 subcarriers)
Subcarrier Spacing: 15 KHz
CP Type: normal
bandwidth: 4.32 MHz
symbolsPerSlot: 14
slotsPerSubFrame: 1
nFFT: 2048
frameNo: 0
slotNo: 0
CSI-RS:
resourceId: 0
numPorts: 24
cdmSize: 8 (cdm8-FD2-TD4)
density: 1
RE Indexes: 2 6 10
Symbol Indexes: 5
Table Row: 15
Slot Offset: 0
Power: 0 db
scramblingID: 0
Grid Stats:
GridSize: 96768
UNASSIGNED: 92160
CSIRS_NZP: 4608
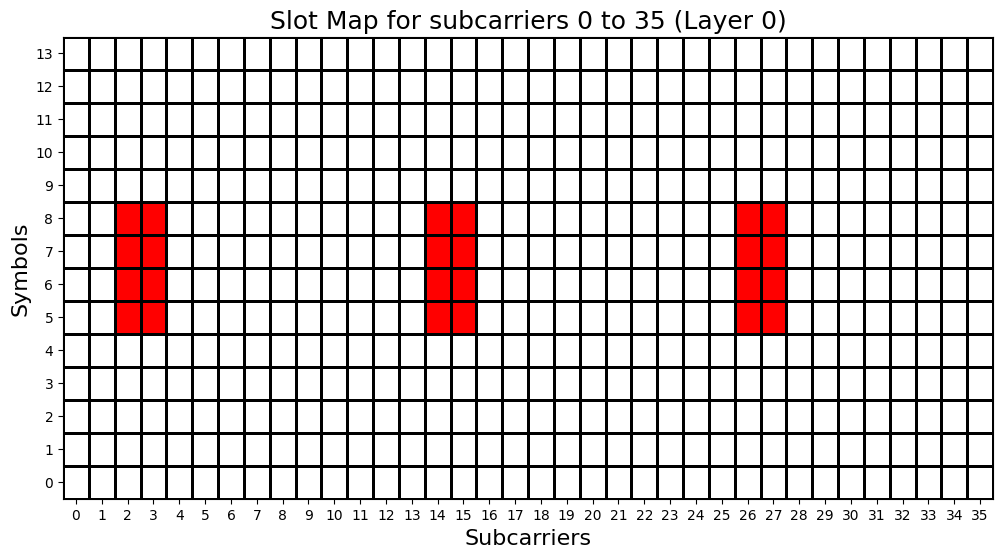
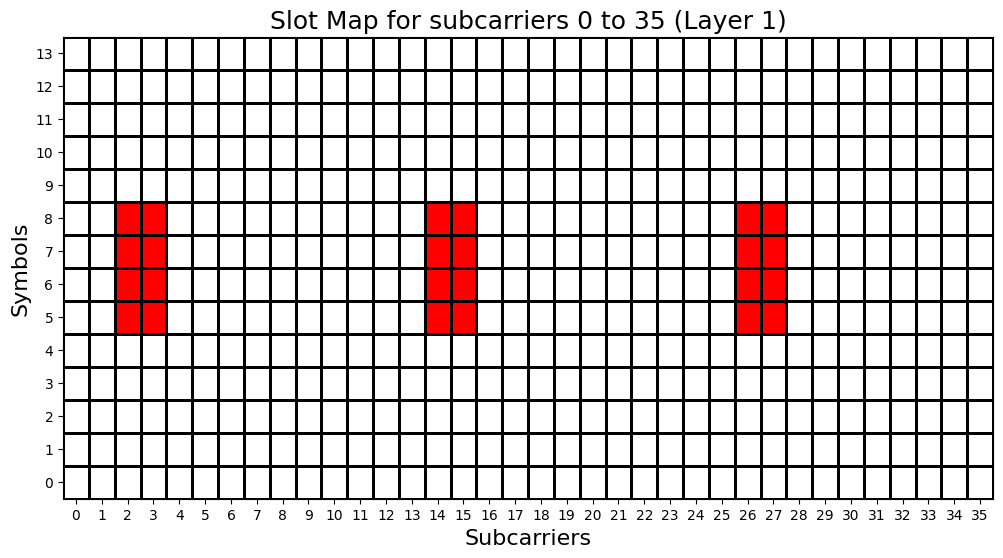
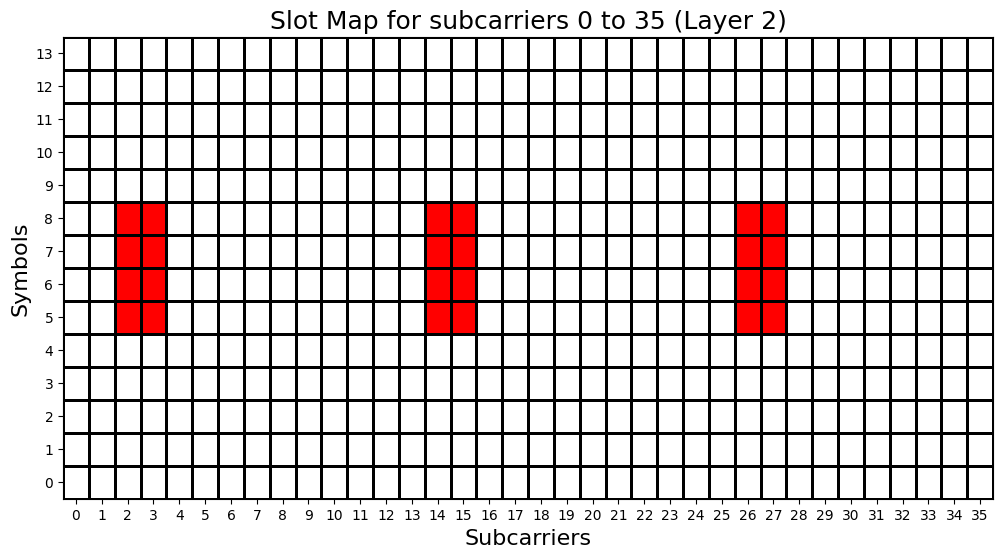
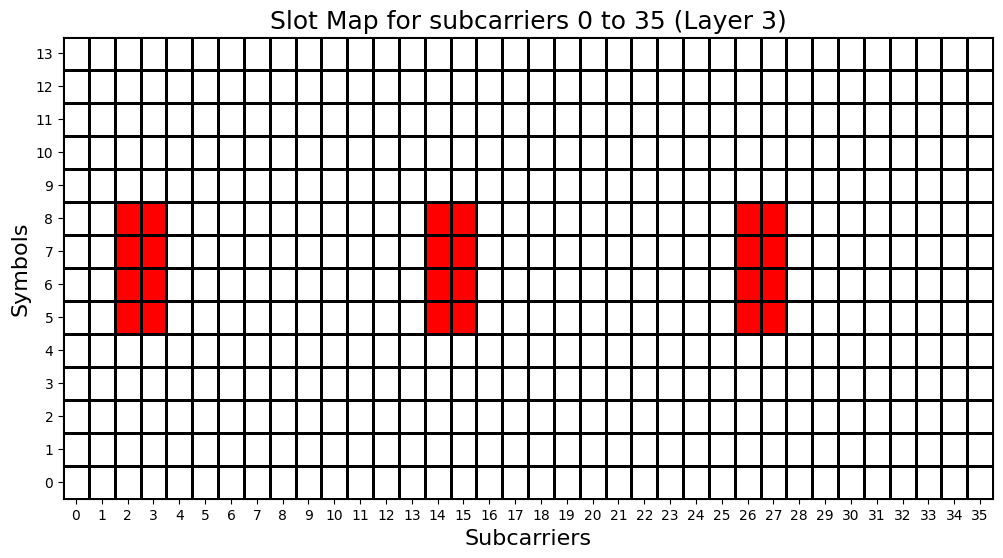
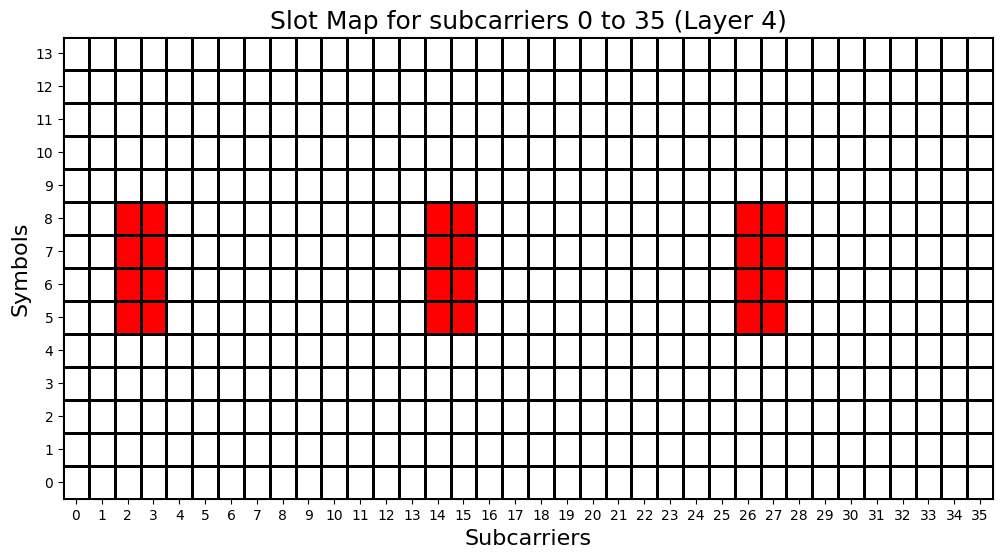
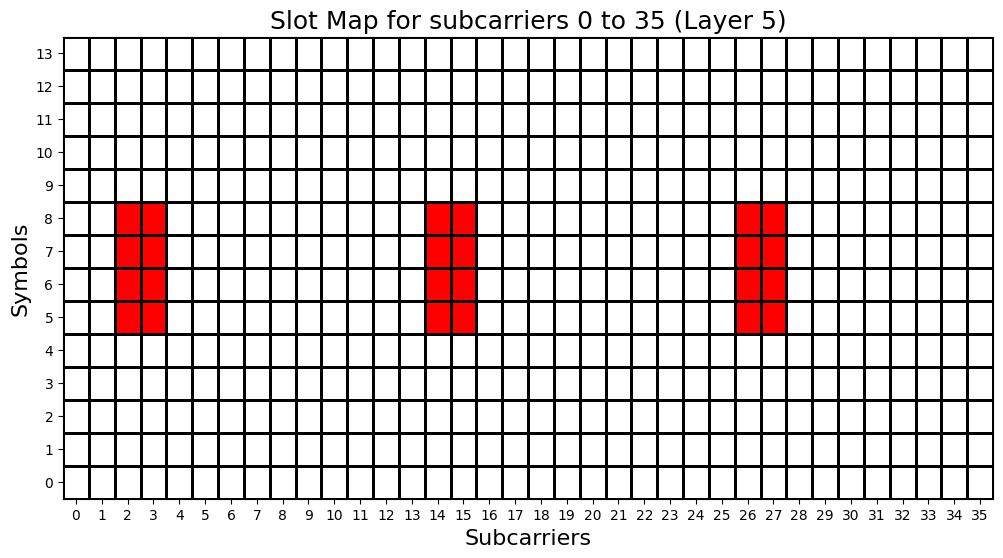
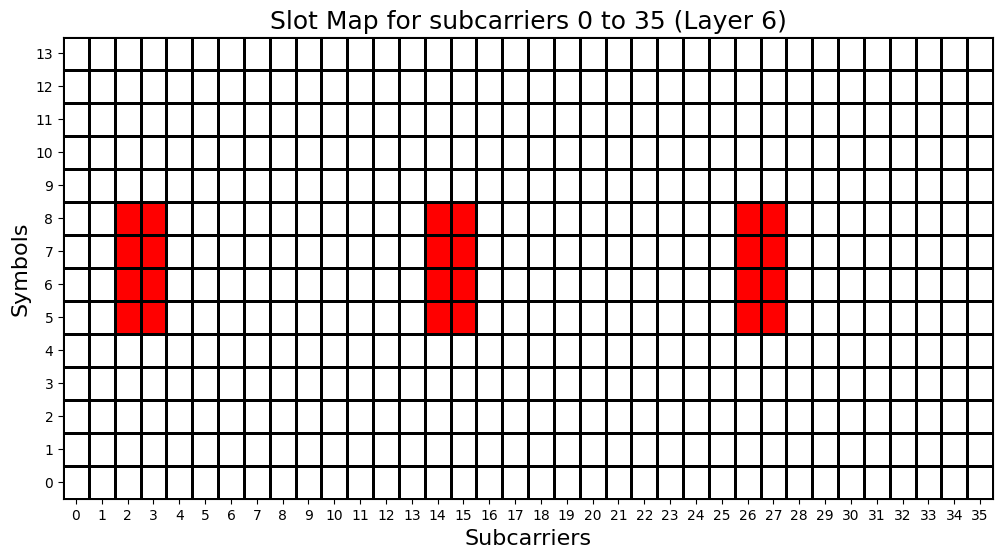
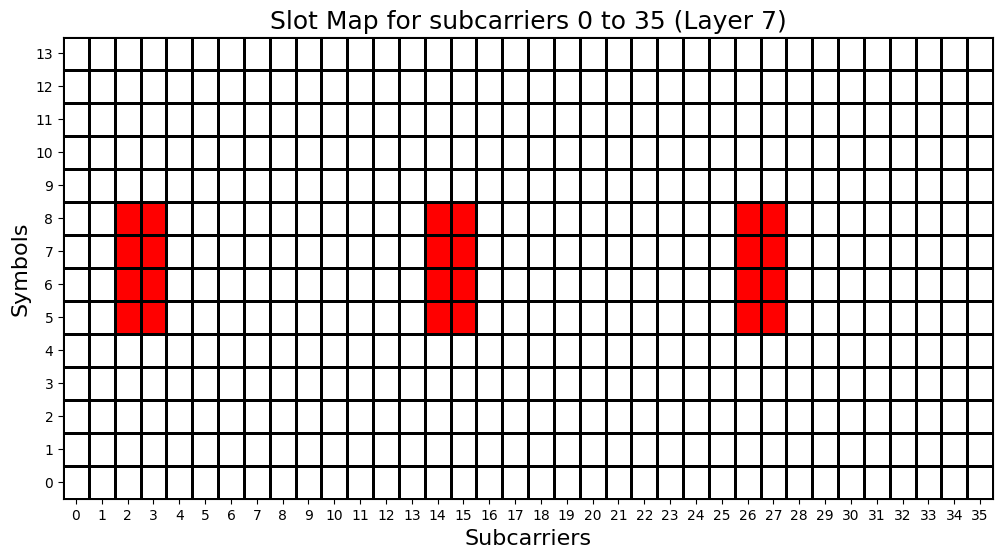
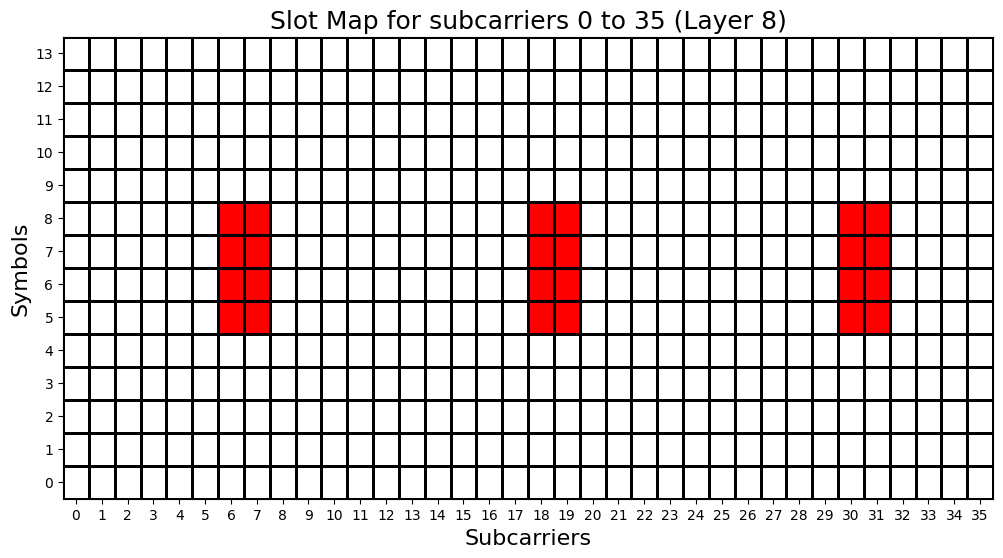
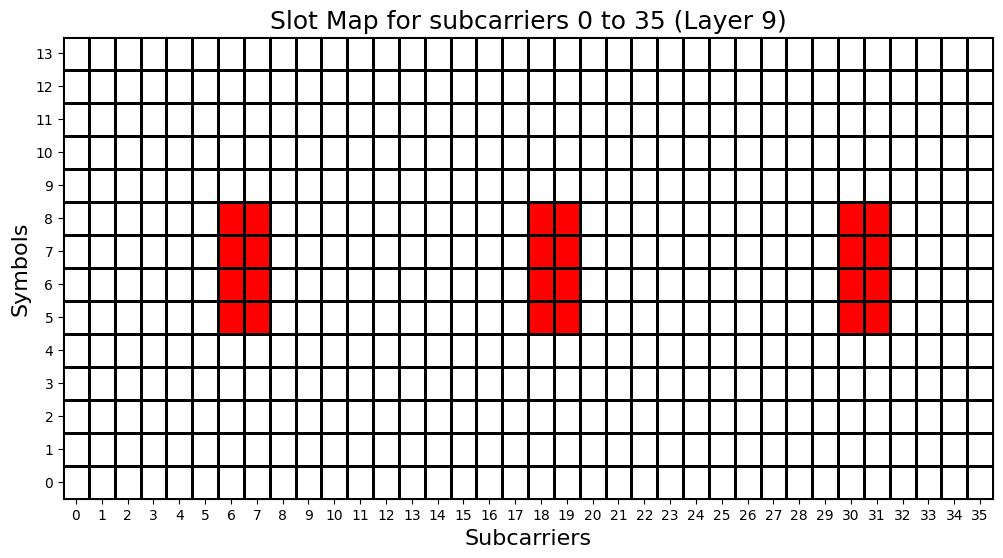
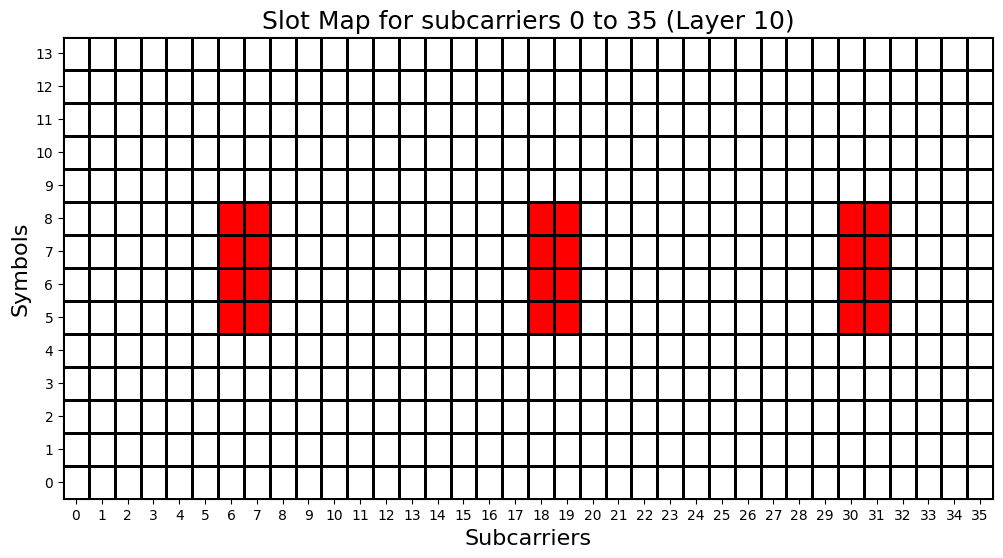
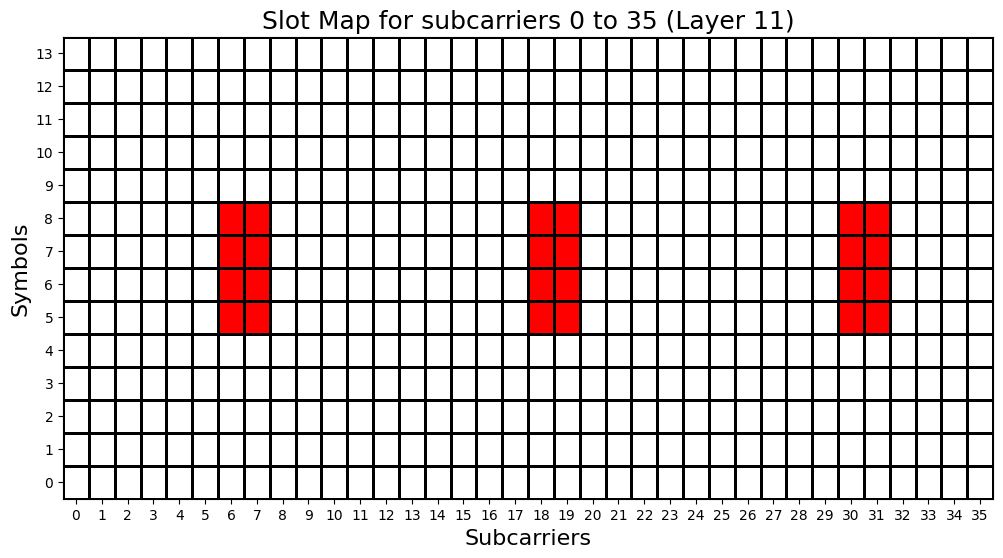
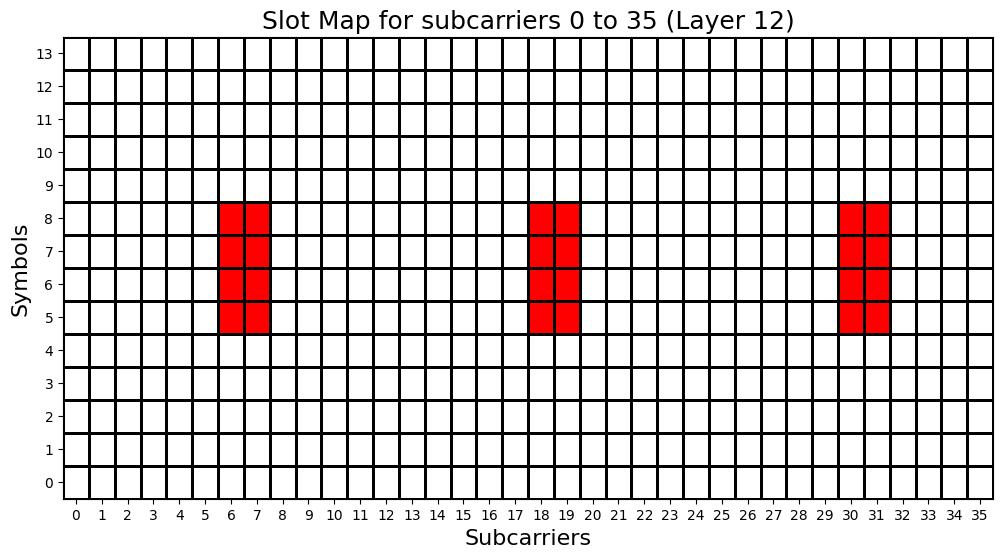
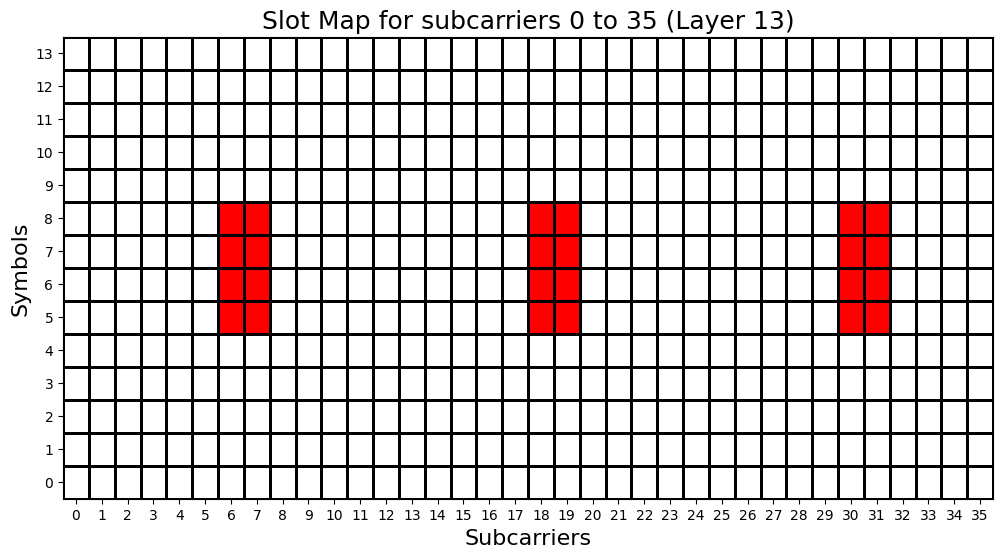
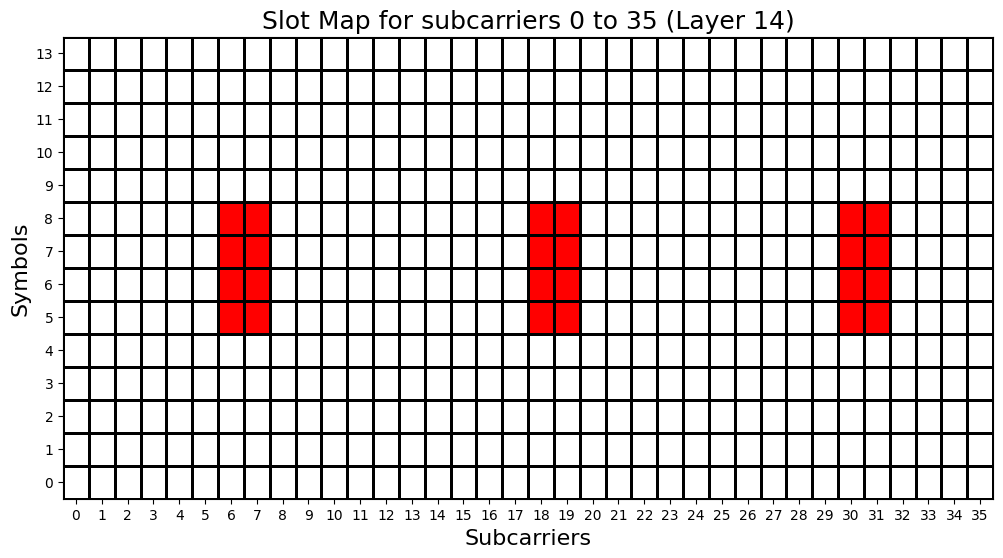
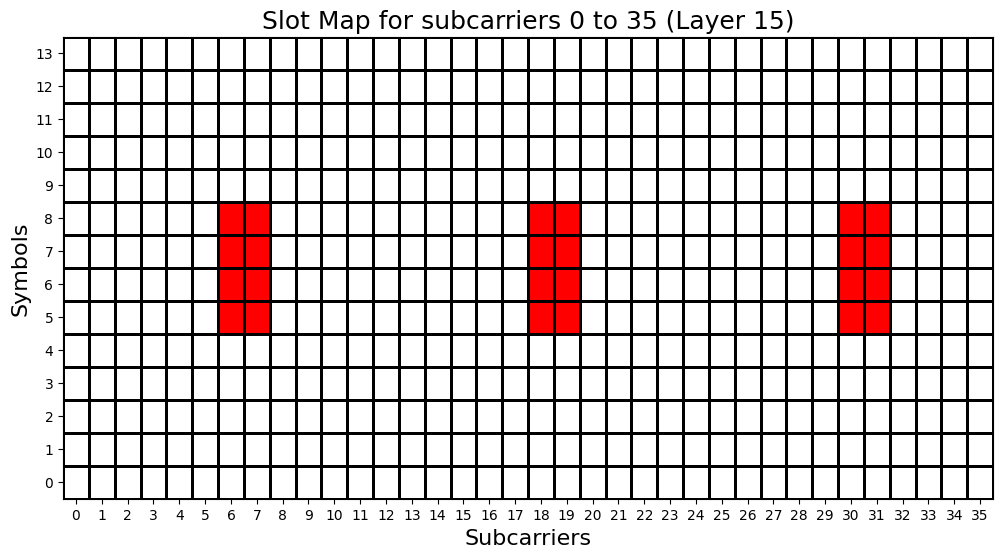
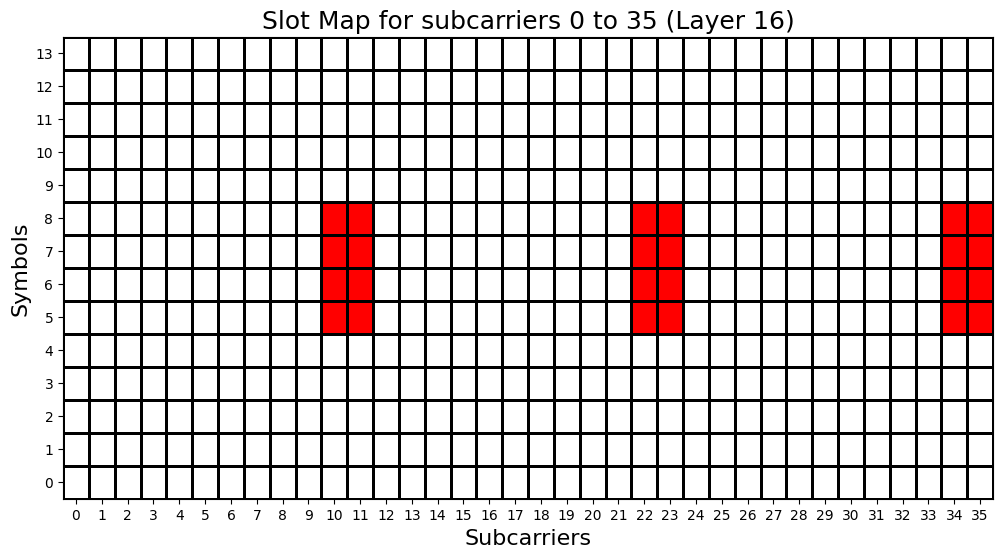
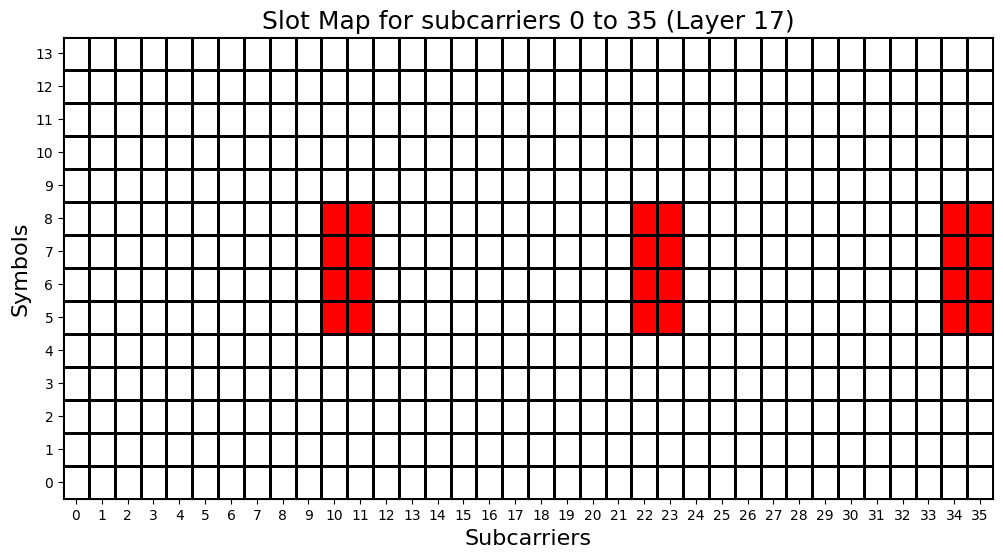
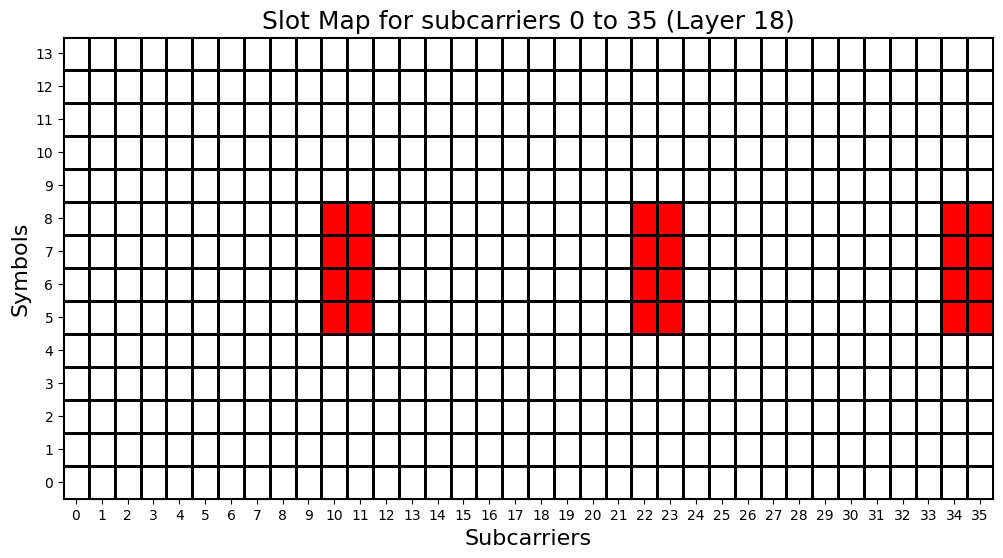
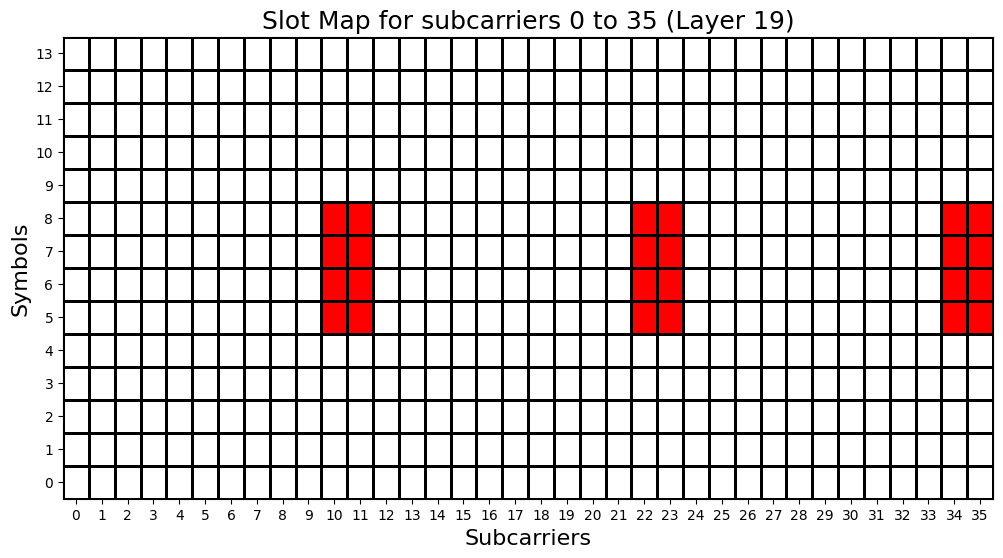
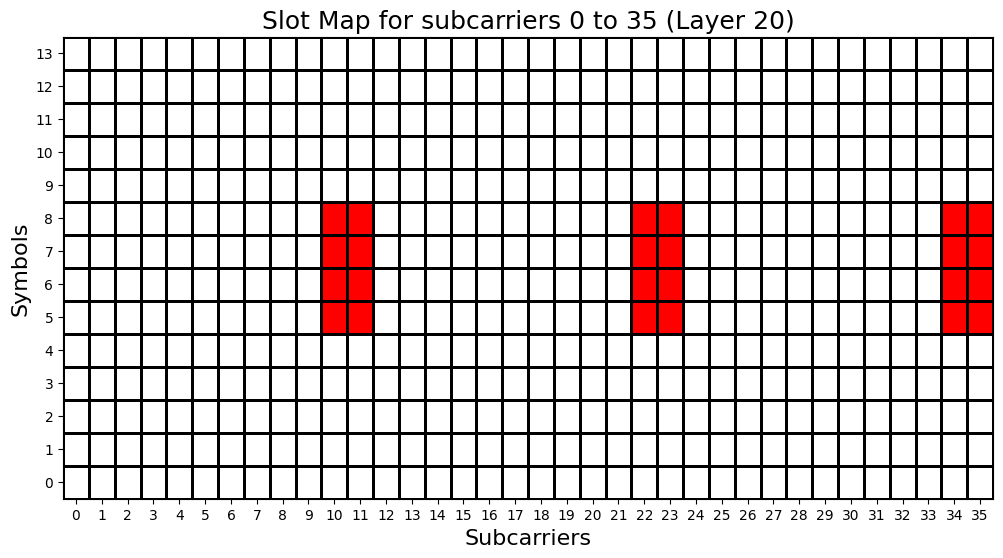
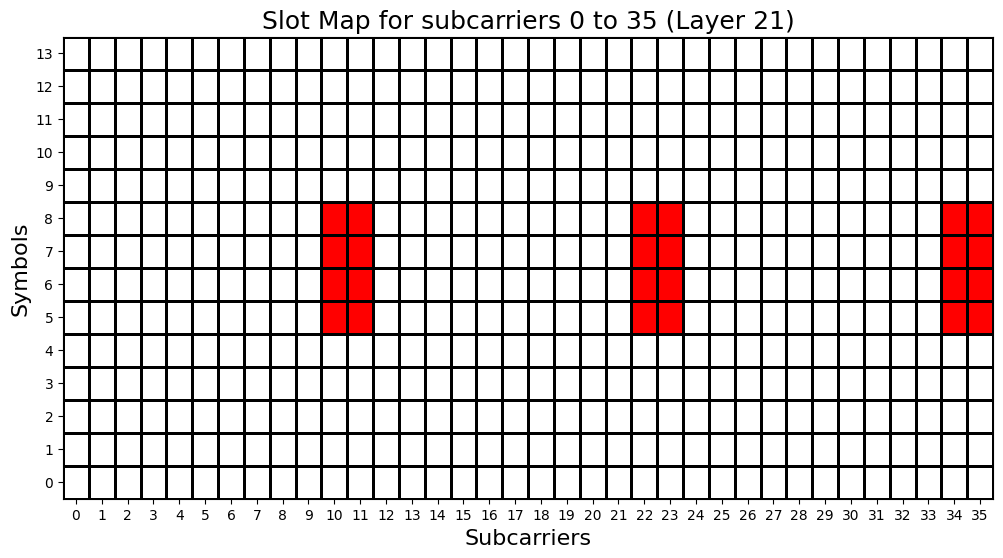
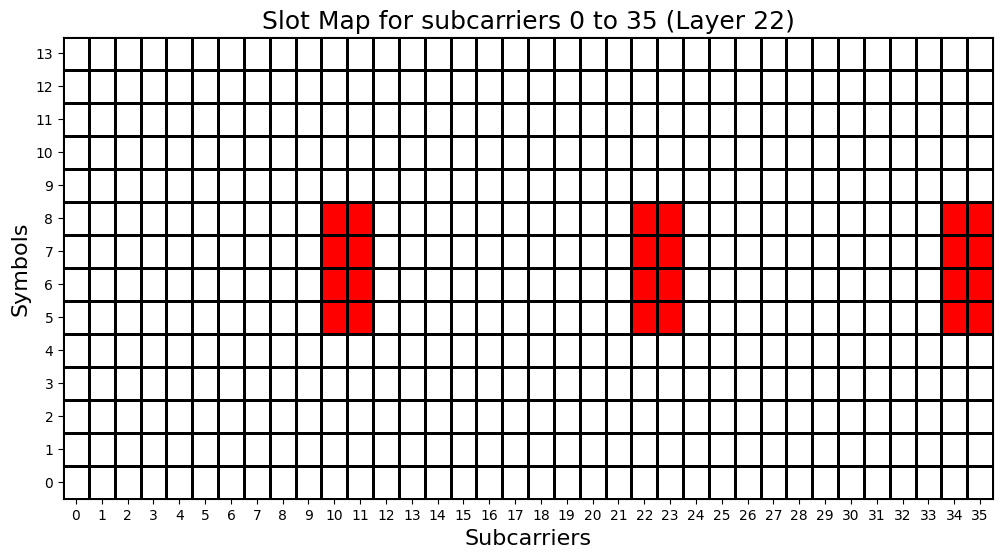
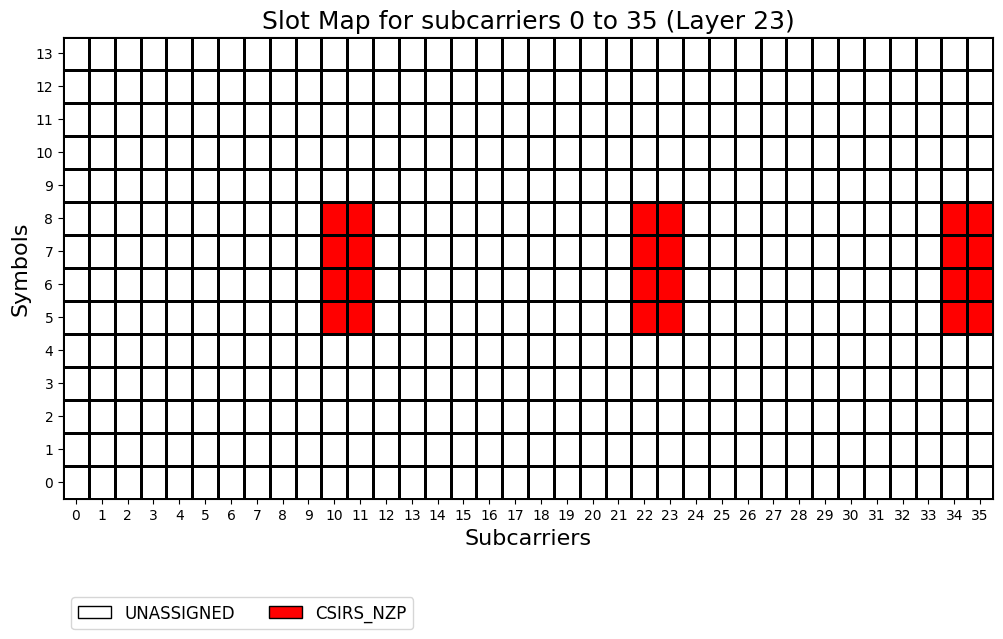
[20]:
# Example for 16th row of 38.211 Table 7.4.1.5.3-1
# 32 ports, 4 of 6 bits set in freqMap, CDM size is 2, and density is 1.
# The CSI-RS resources are at symbols 4, 5, 8, and 9 and REs (0,1),(2,3),(8,9) and (10,11).
# Note that this configuration also allows density=.5 which would cause every other
# RB to be skipped for the CSR-RS allocation. (default density is 1)
csiRsConfig = CsiRsConfig([CsiRsSet("NZP", bwp, symbols=[4,8], numPorts=32, freqMap="110011", cdmSize=2)])
csiRsConfig.print()
grid = bwp.createGrid(csiRsConfig.numPorts)
csiRsConfig.populateGrid(grid)
print("Grid Stats:")
stats = grid.getStats()
for key, value in stats.items(): print(" %s: %d"%(key, value))
grid.drawMap(ports=range(csiRsConfig.numPorts), reRange=(0,36))
CSI-RS Configuration: (1 Resource Sets)
CSI-RS Resource Set:(1 NZP Resources)
Resource Set ID: 0
Resource Type: periodic
Resource Blocks: 24 RBs starting at 0
Slot Period: 4
Bandwidth Part:
Resource Blocks: 24 RBs starting at 0 (288 subcarriers)
Subcarrier Spacing: 15 KHz
CP Type: normal
bandwidth: 4.32 MHz
symbolsPerSlot: 14
slotsPerSubFrame: 1
nFFT: 2048
frameNo: 0
slotNo: 0
CSI-RS:
resourceId: 0
numPorts: 32
cdmSize: 2 (fd-CDM2)
density: 1
RE Indexes: 0 2 8 10
Symbol Indexes: 4 8
Table Row: 16
Slot Offset: 0
Power: 0 db
scramblingID: 0
Grid Stats:
GridSize: 129024
UNASSIGNED: 127488
CSIRS_NZP: 1536
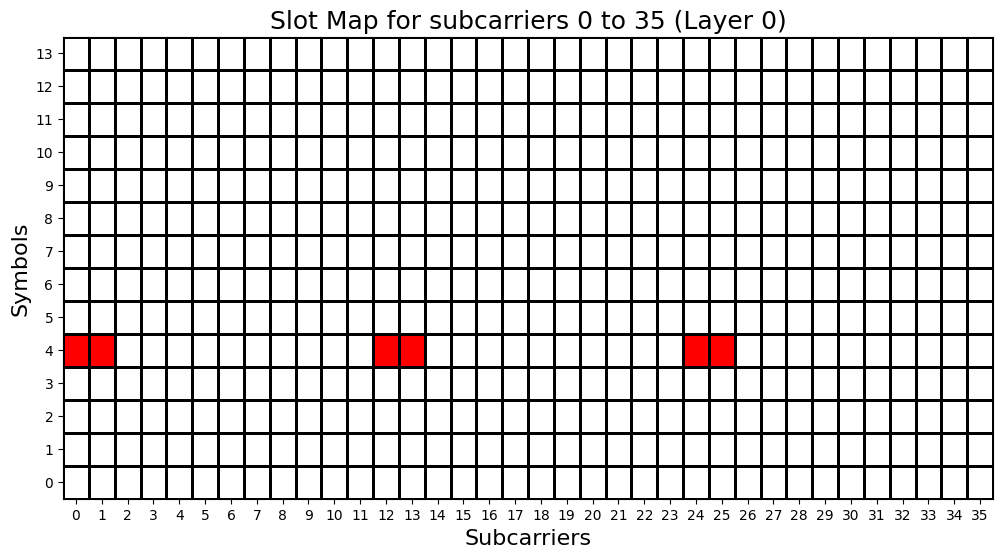
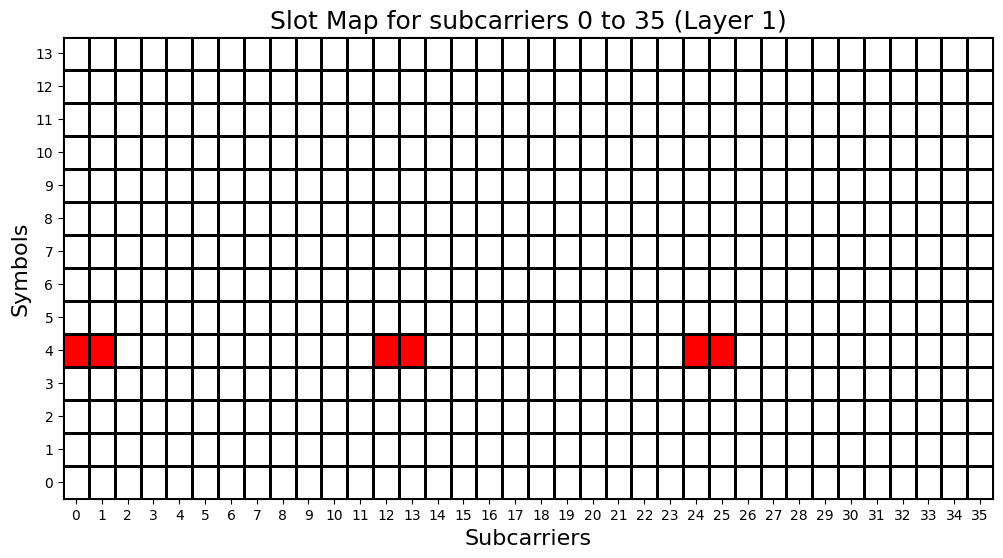
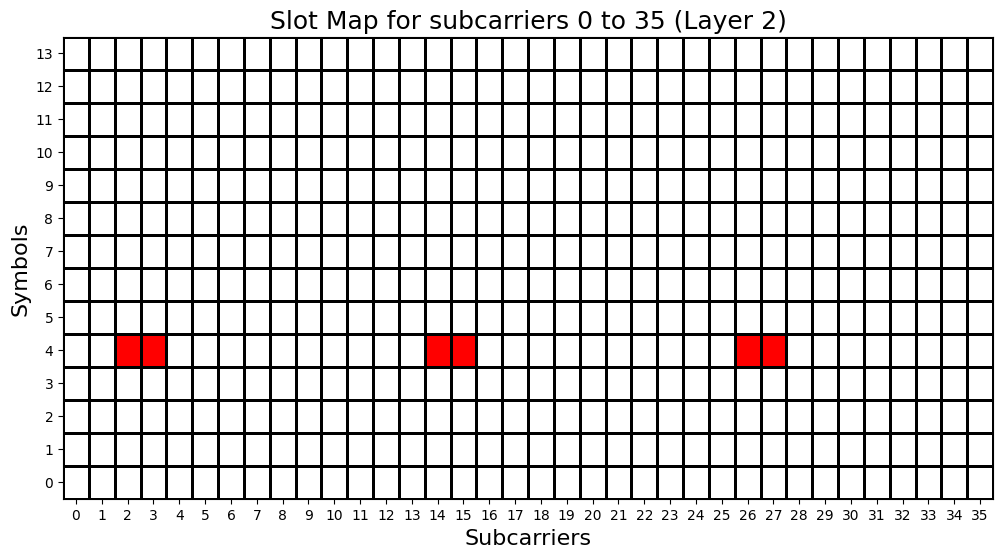
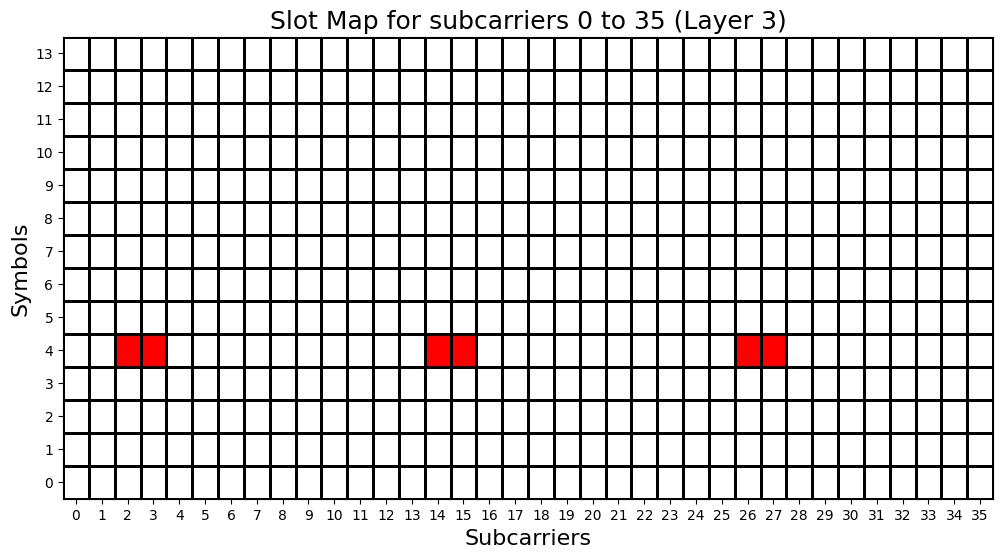
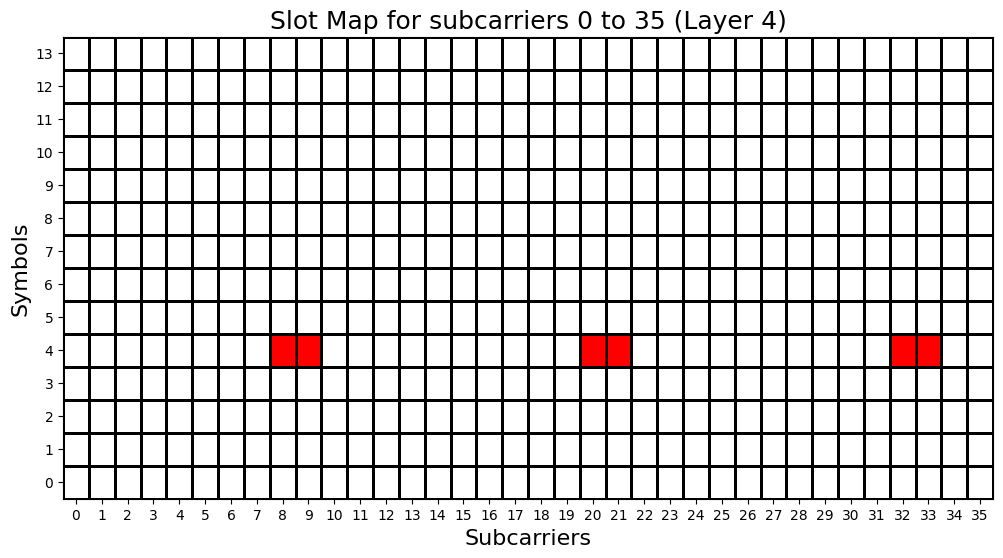
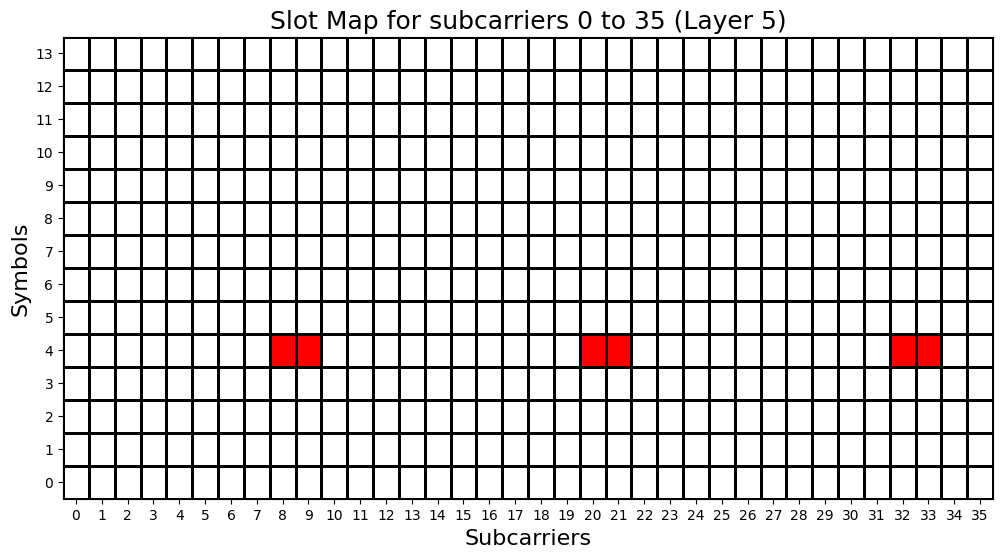
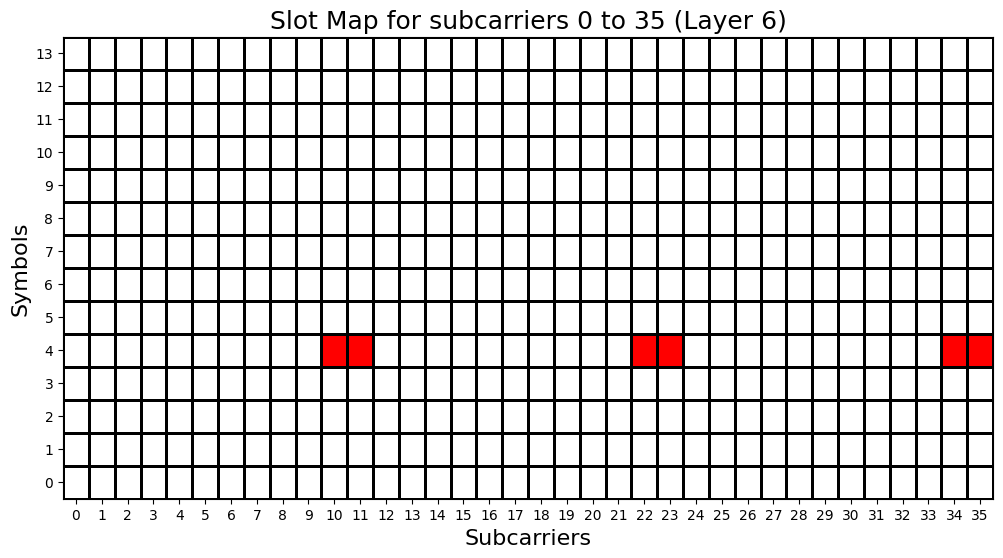
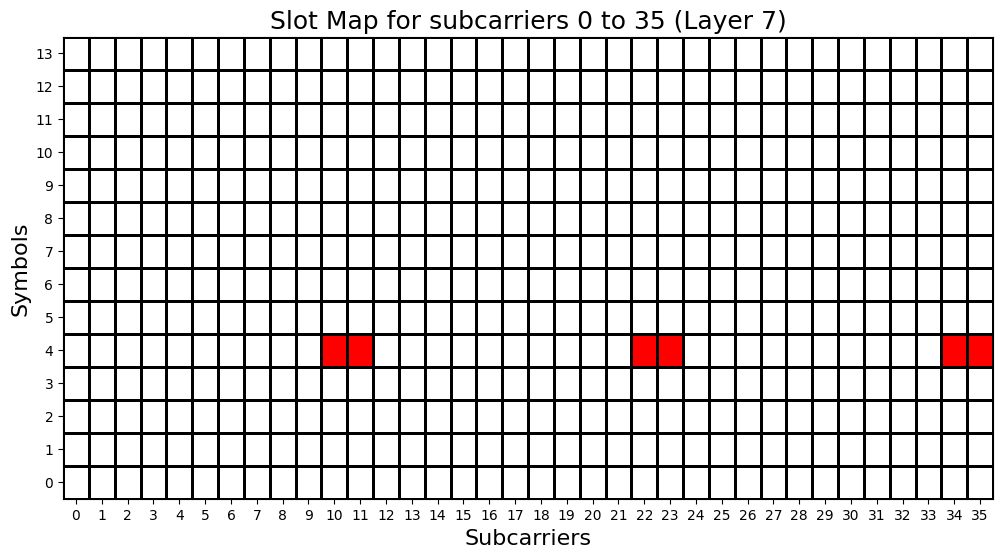
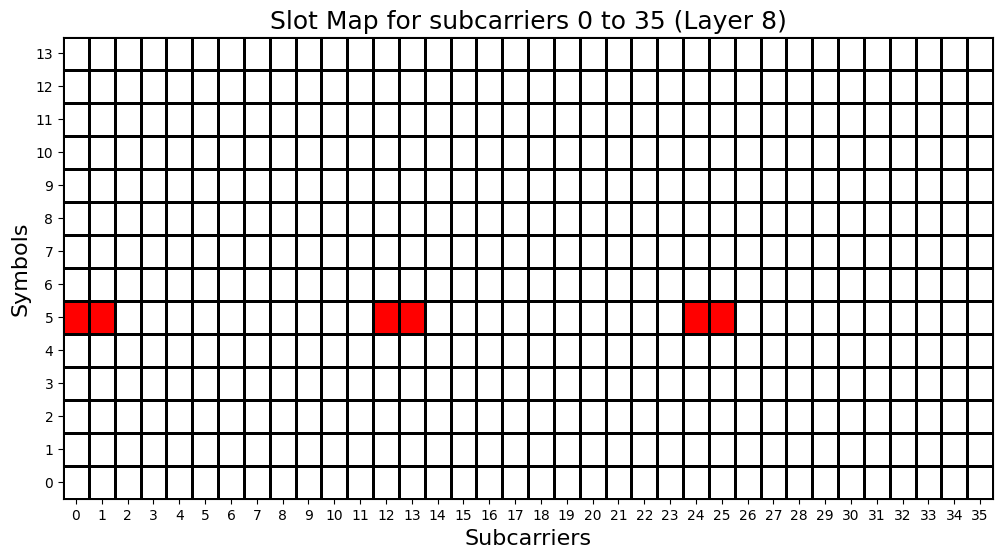
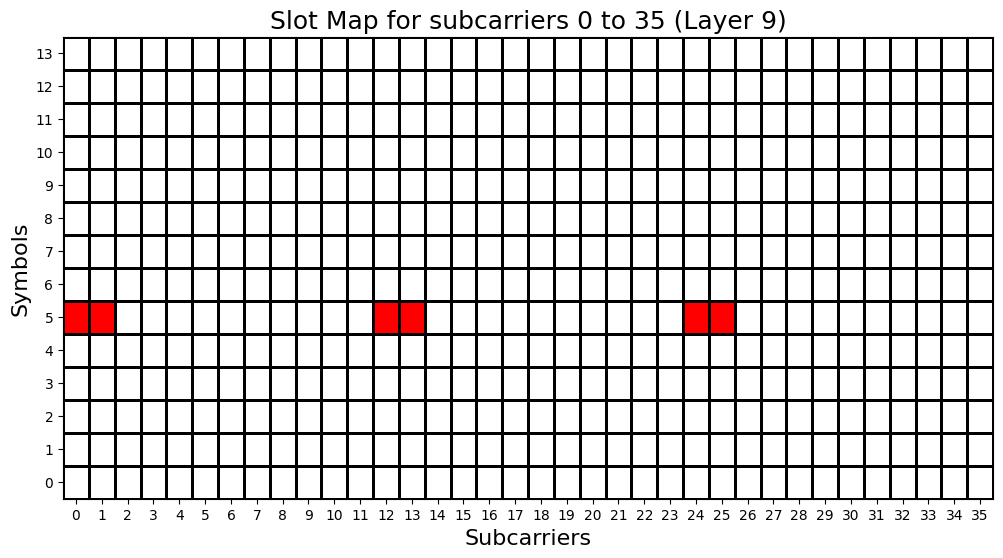
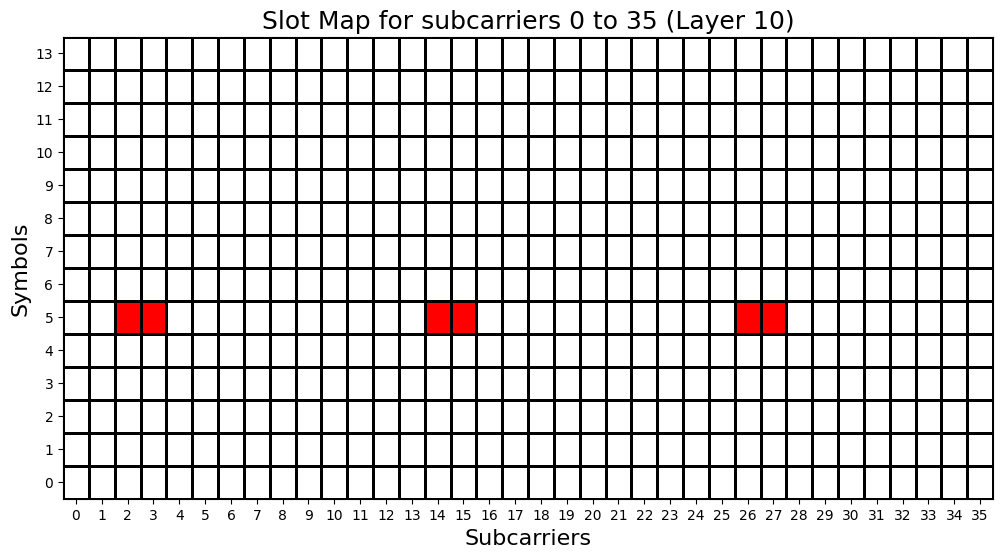
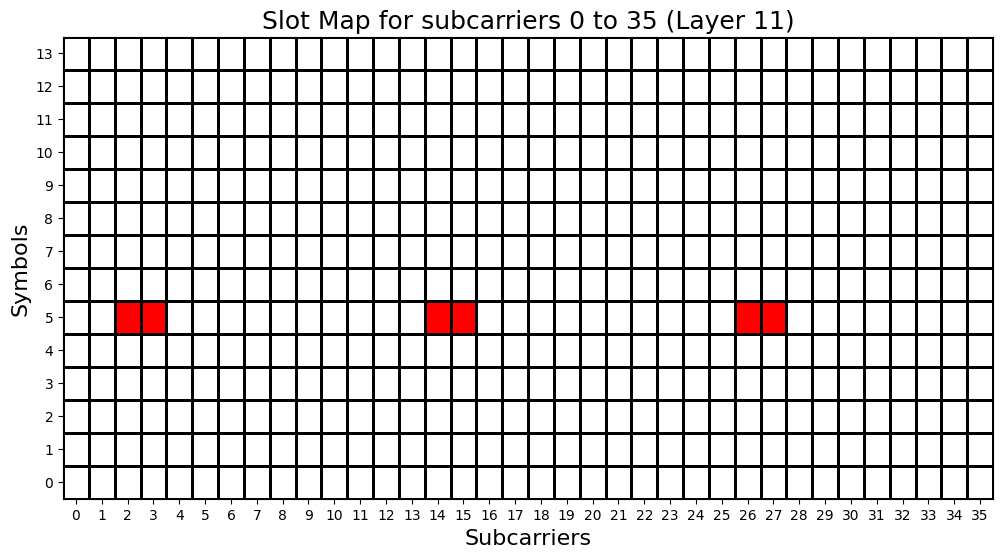
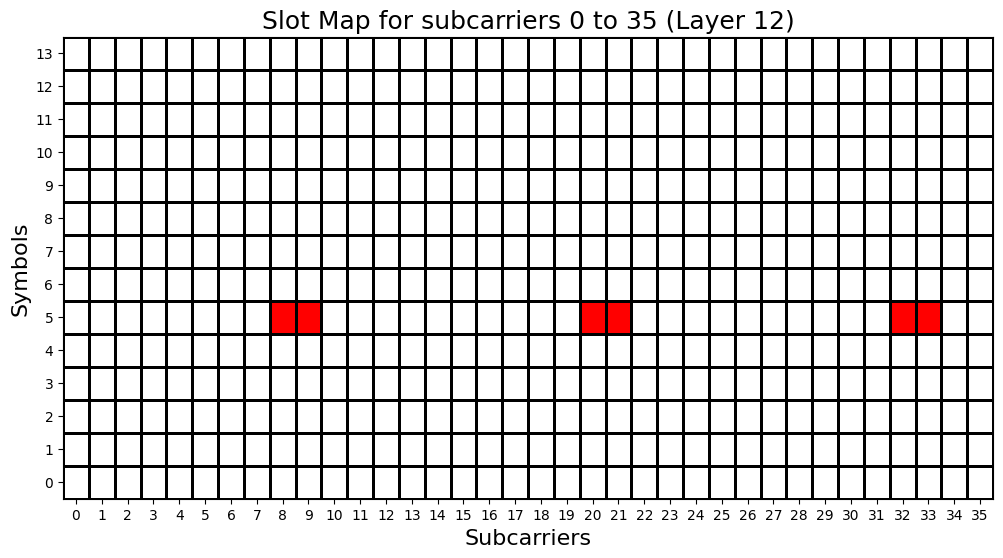
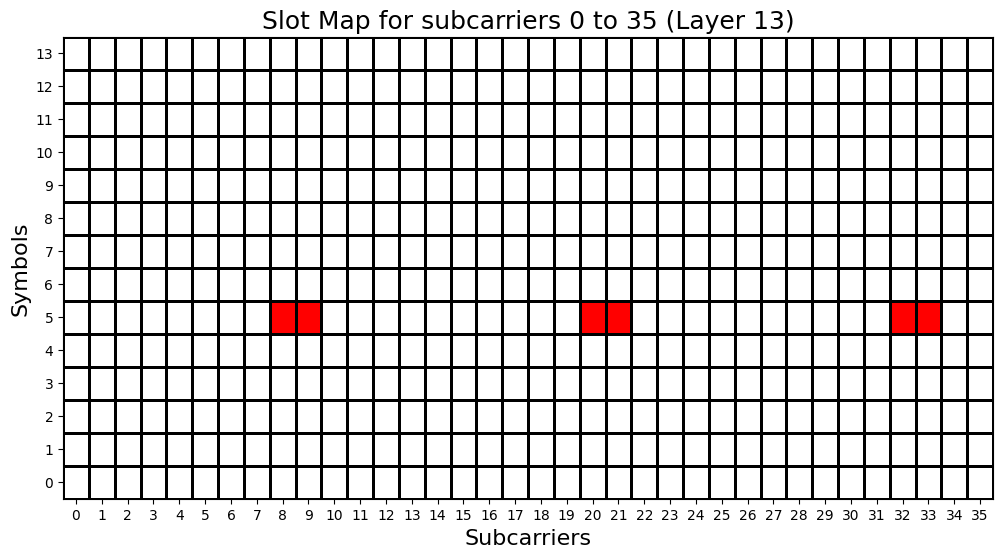
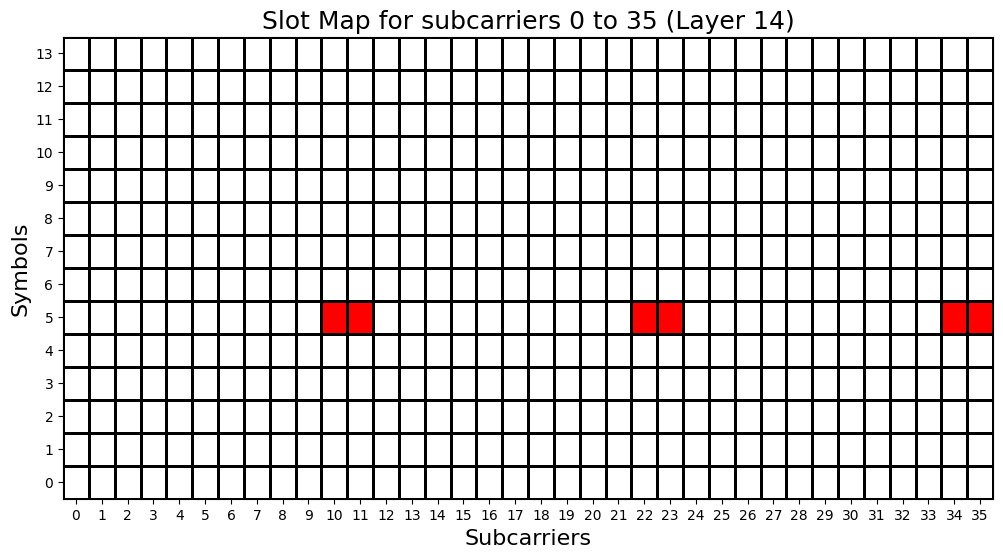
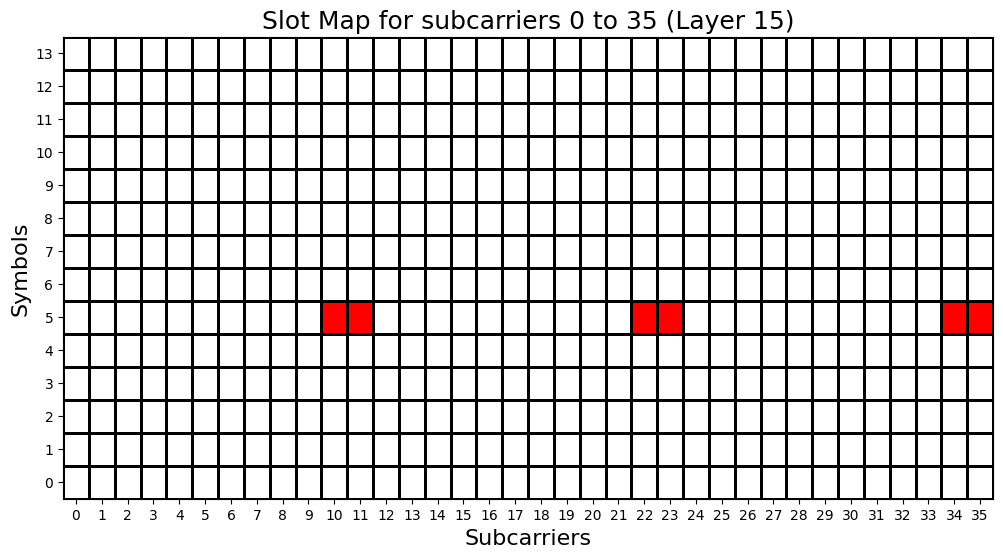
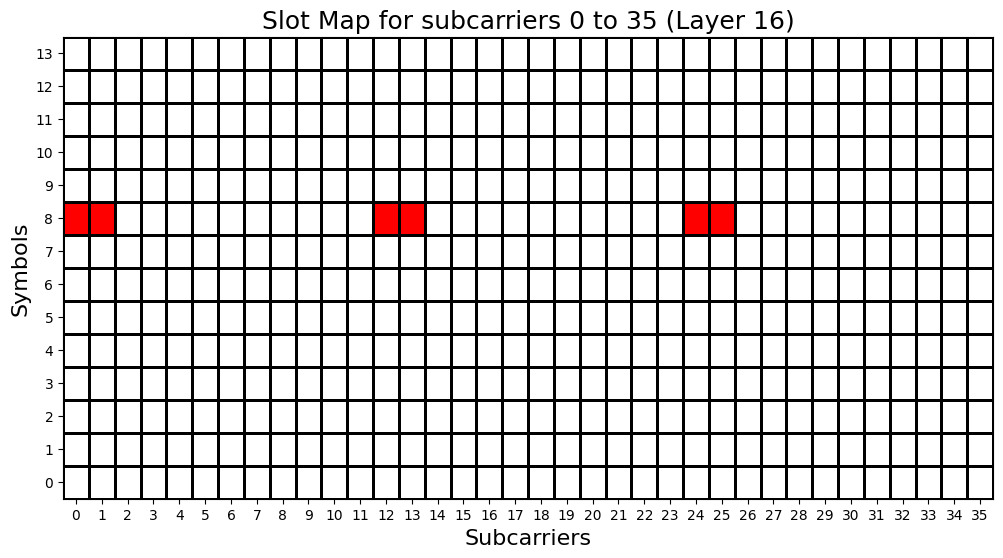
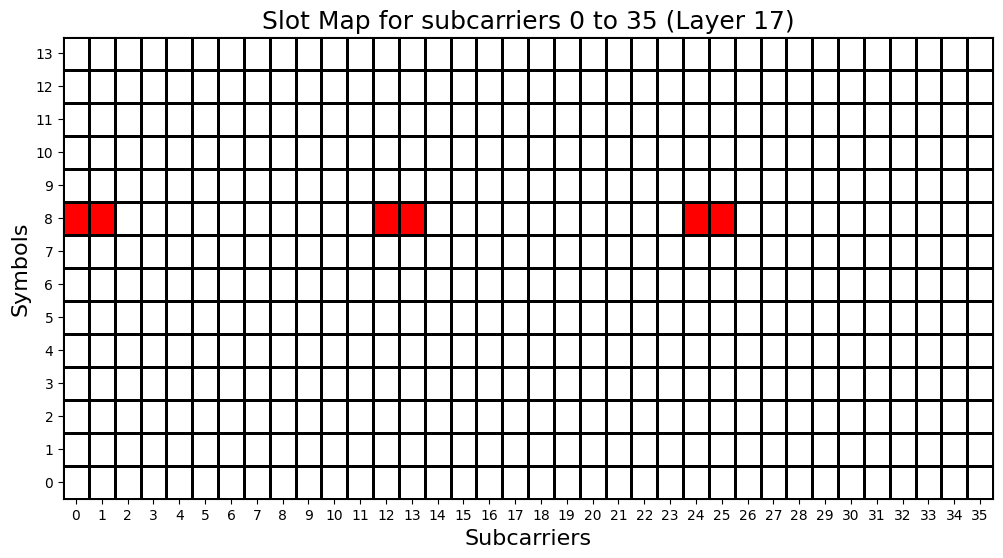
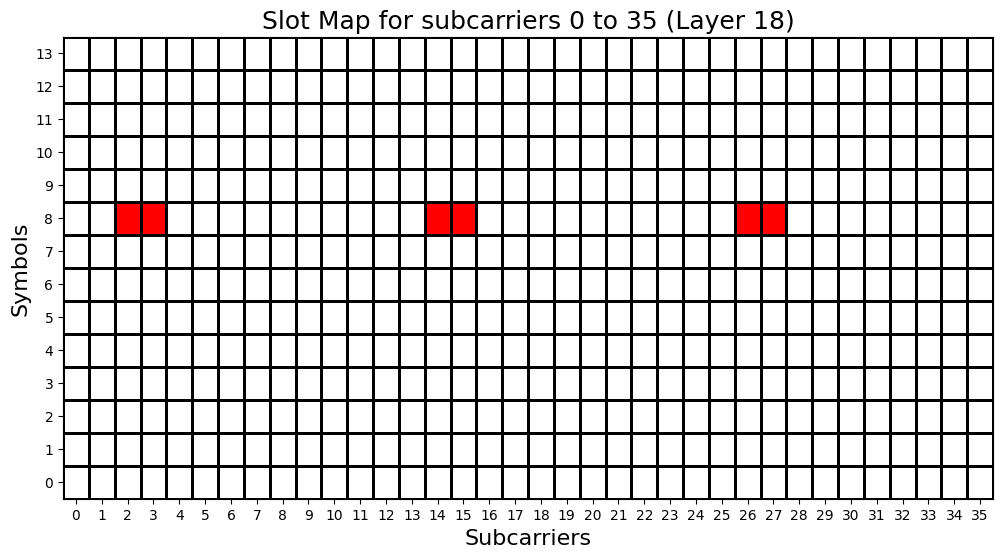
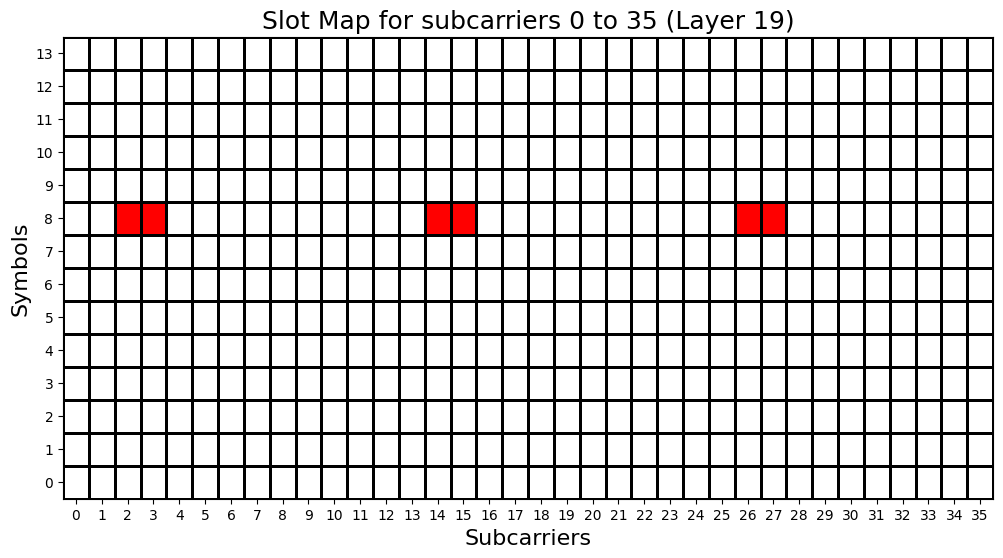
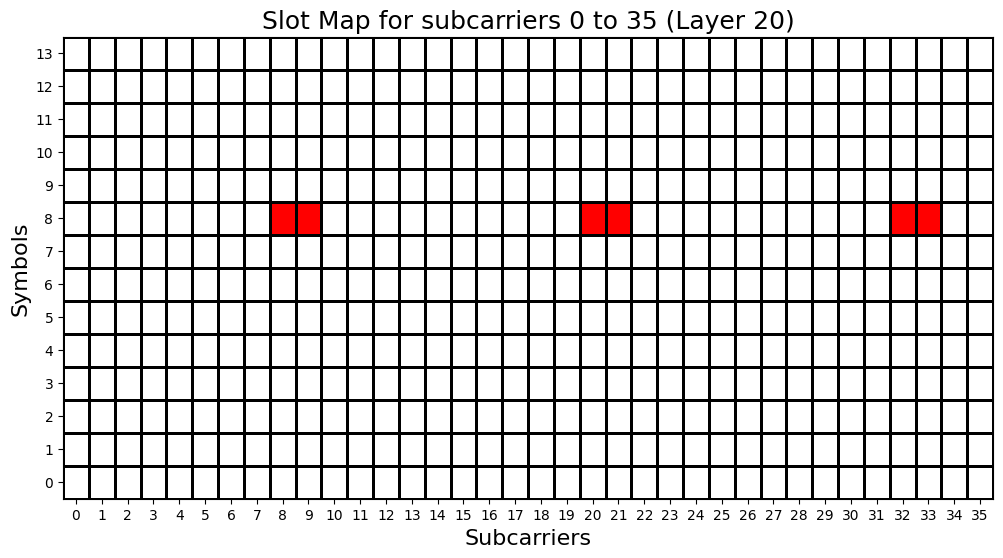
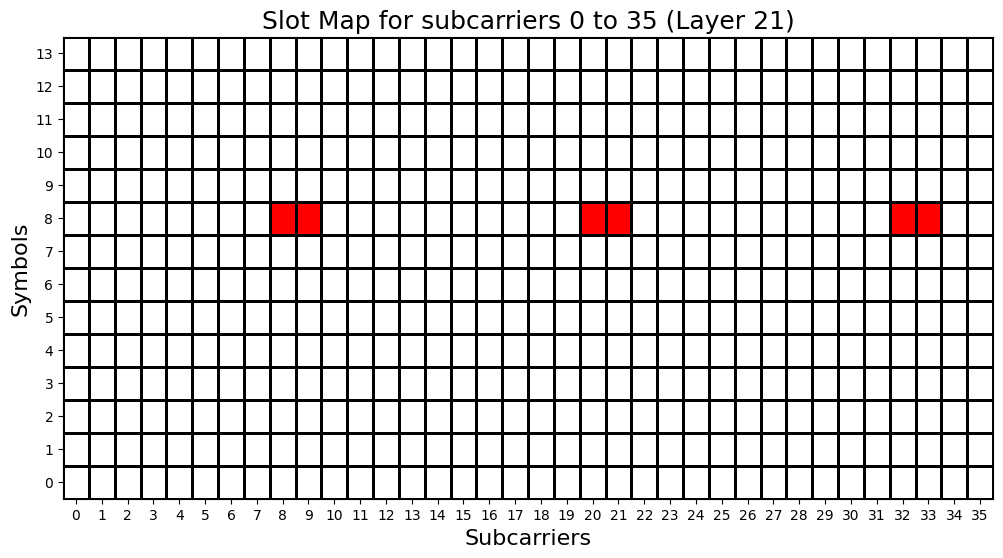
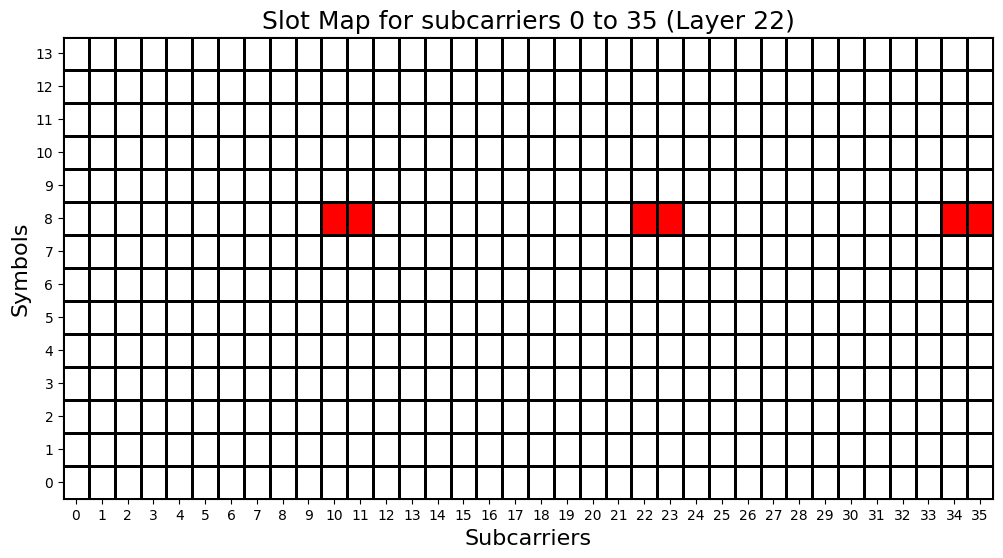
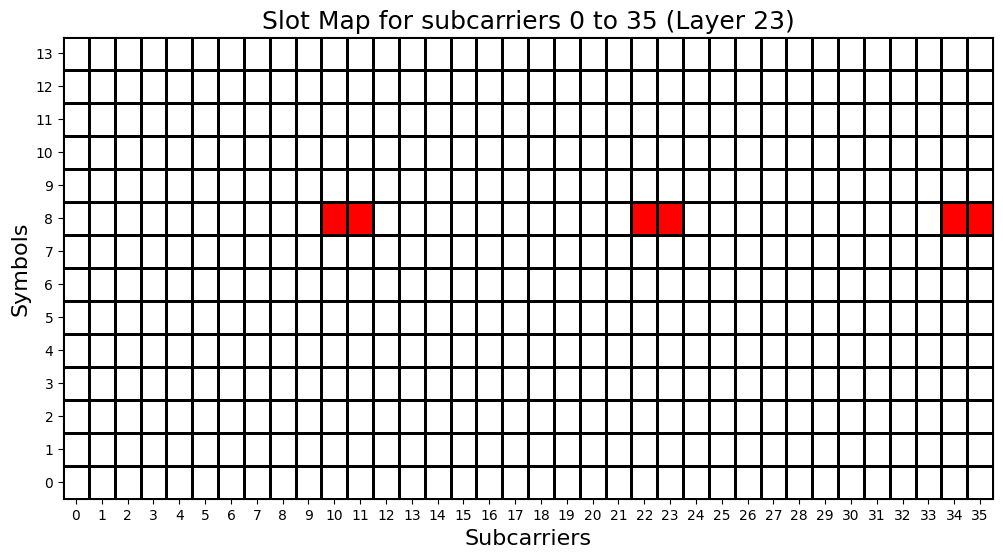
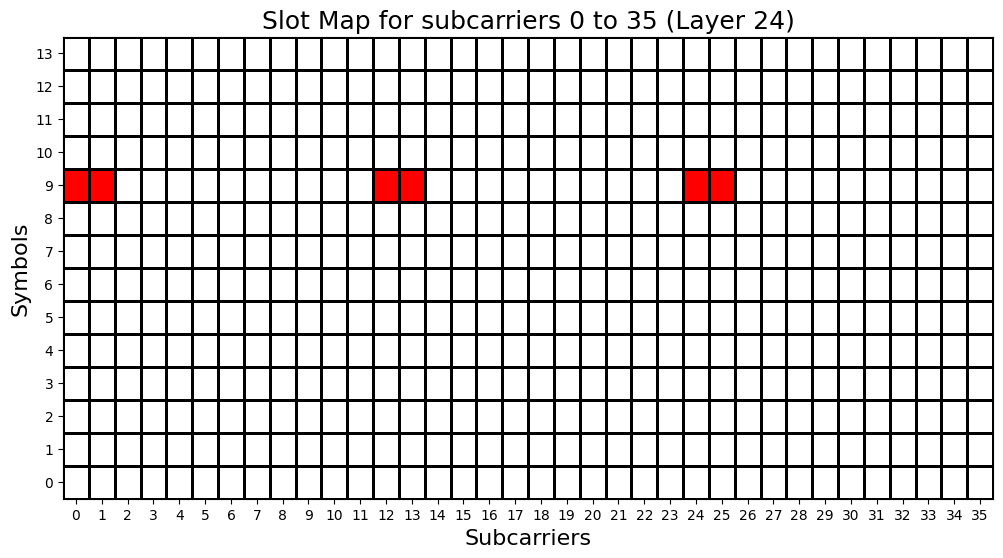
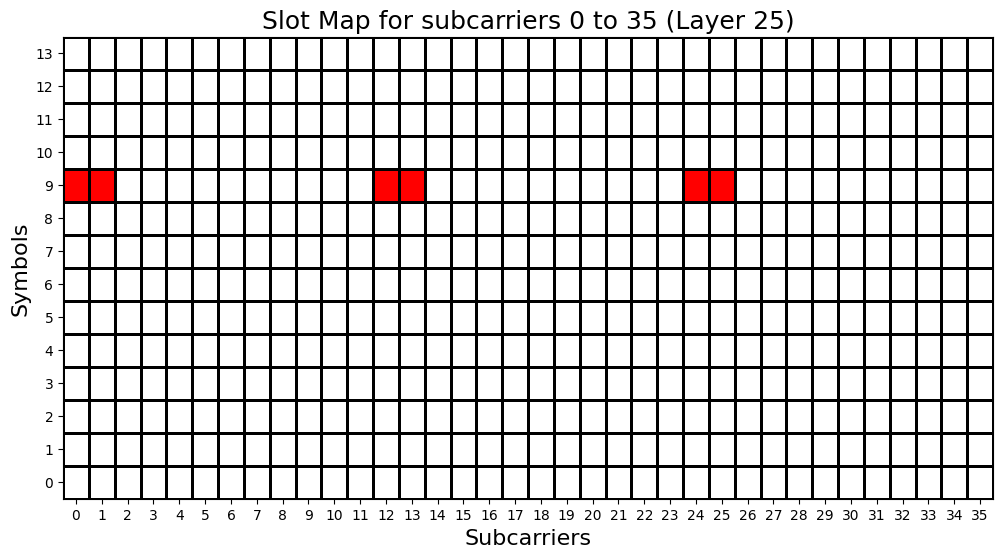
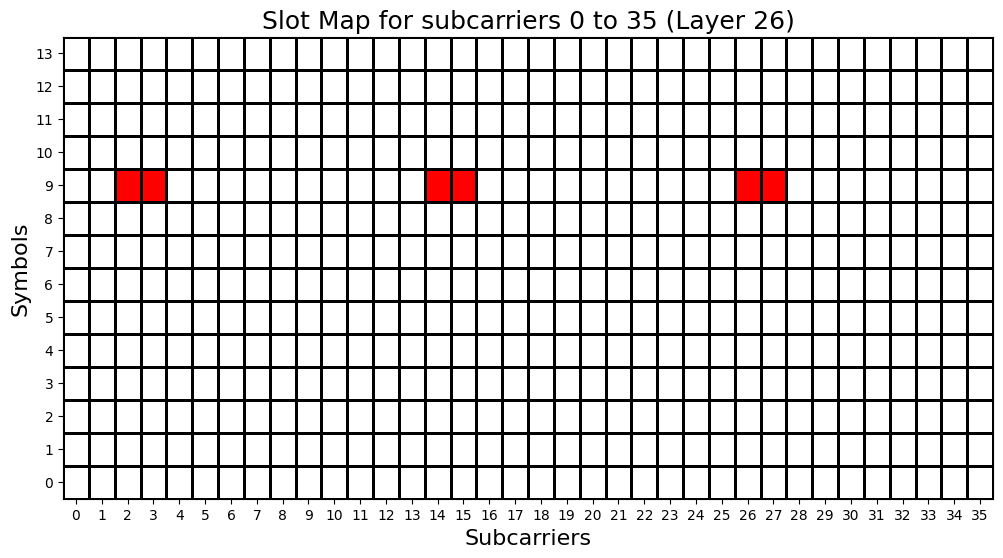
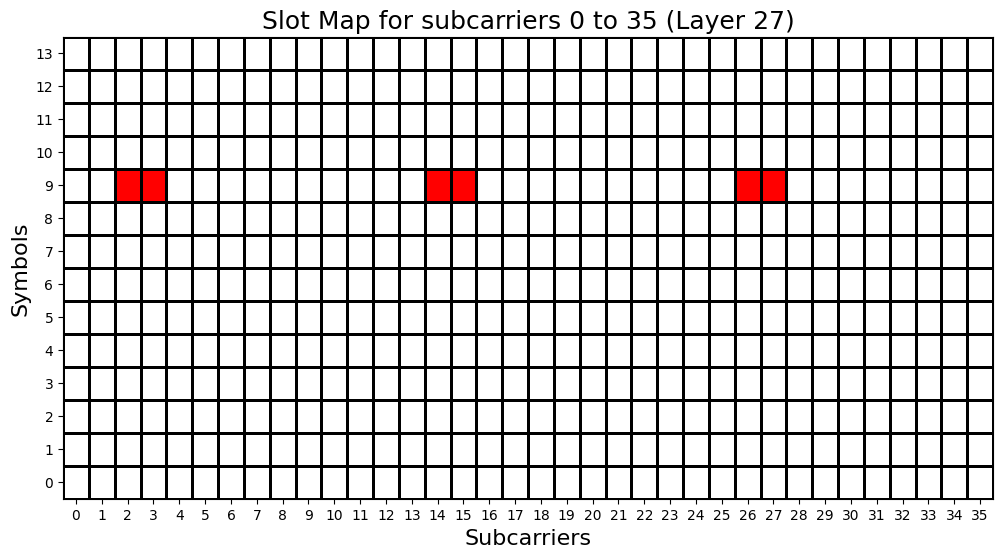
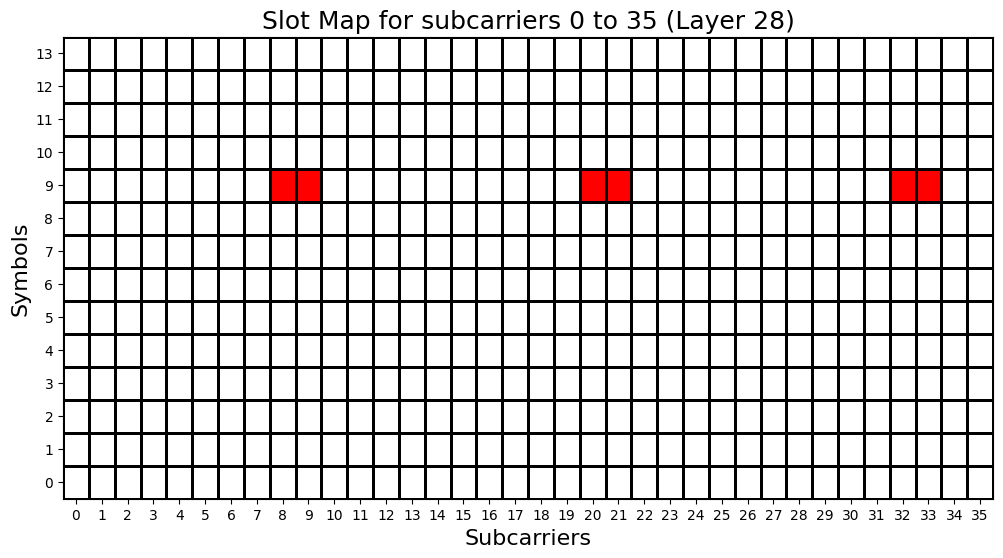
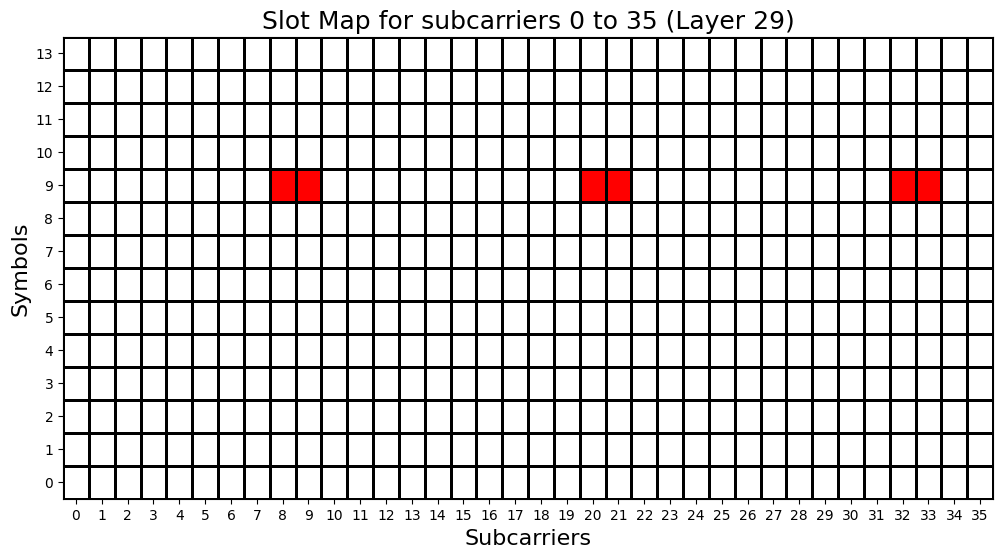
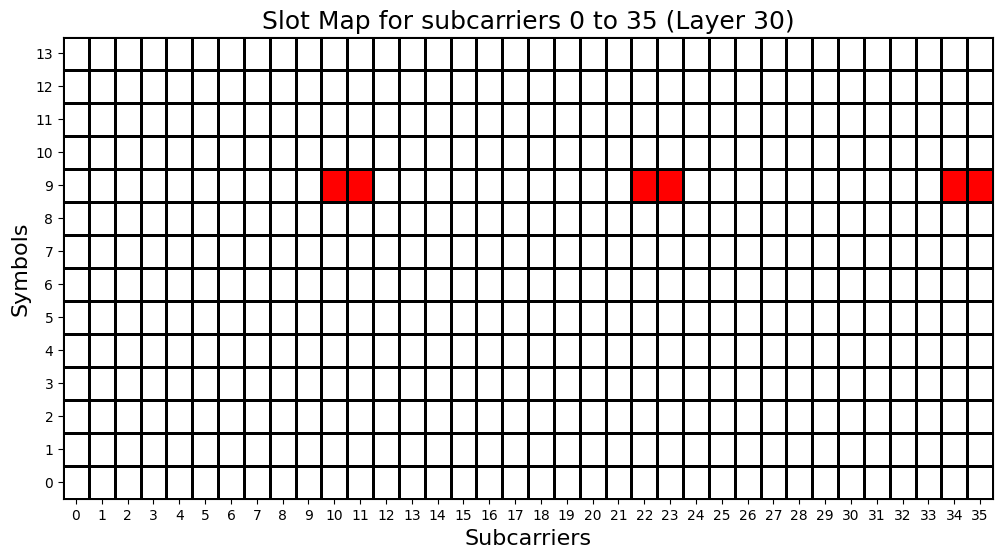
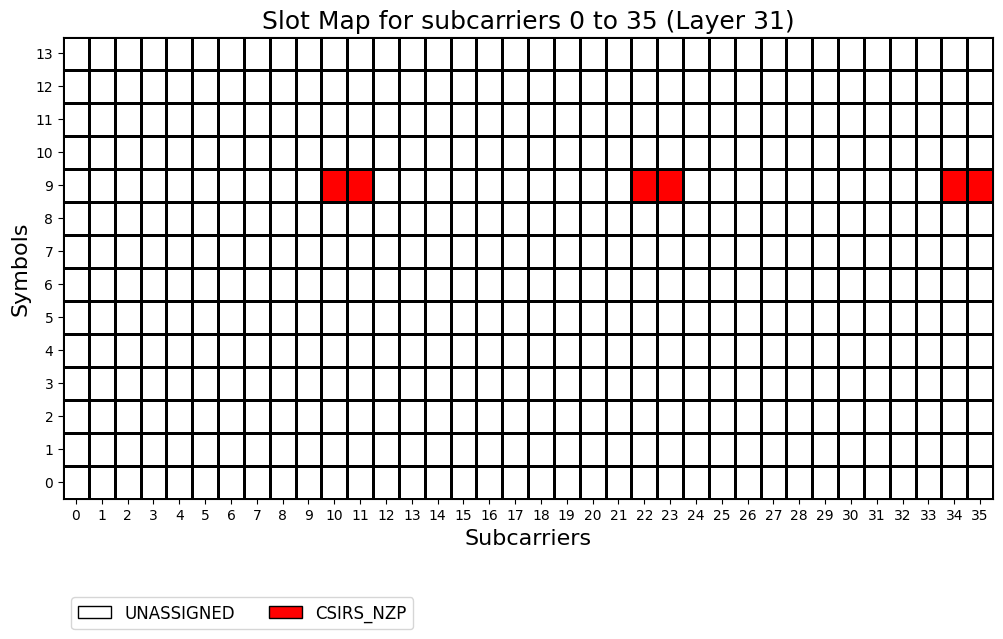
[21]:
# Example for 17th row of 38.211 Table 7.4.1.5.3-1
# 32 ports, 4 of 6 bits set in freqMap, CDM size is 4, and density is 1.
# The CSI-RS resources are at symbols (2,3), (8,9) and REs (0,1),(2,3),(8,9) and (10,11).
# Note that this configuration also allows density=.5 which would cause every other
# RB to be skipped for the CSR-RS allocation. (default density is 1)
csiRsConfig = CsiRsConfig([CsiRsSet("NZP", bwp, symbols=[2,8], numPorts=32, freqMap="110011", cdmSize=4)])
csiRsConfig.print()
grid = bwp.createGrid(csiRsConfig.numPorts)
csiRsConfig.populateGrid(grid)
print("Grid Stats:")
stats = grid.getStats()
for key, value in stats.items(): print(" %s: %d"%(key, value))
grid.drawMap(ports=range(csiRsConfig.numPorts), reRange=(0,36))
CSI-RS Configuration: (1 Resource Sets)
CSI-RS Resource Set:(1 NZP Resources)
Resource Set ID: 0
Resource Type: periodic
Resource Blocks: 24 RBs starting at 0
Slot Period: 4
Bandwidth Part:
Resource Blocks: 24 RBs starting at 0 (288 subcarriers)
Subcarrier Spacing: 15 KHz
CP Type: normal
bandwidth: 4.32 MHz
symbolsPerSlot: 14
slotsPerSubFrame: 1
nFFT: 2048
frameNo: 0
slotNo: 0
CSI-RS:
resourceId: 0
numPorts: 32
cdmSize: 4 (cdm4-FD2-TD2)
density: 1
RE Indexes: 0 2 8 10
Symbol Indexes: 2 8
Table Row: 17
Slot Offset: 0
Power: 0 db
scramblingID: 0
Grid Stats:
GridSize: 129024
UNASSIGNED: 125952
CSIRS_NZP: 3072
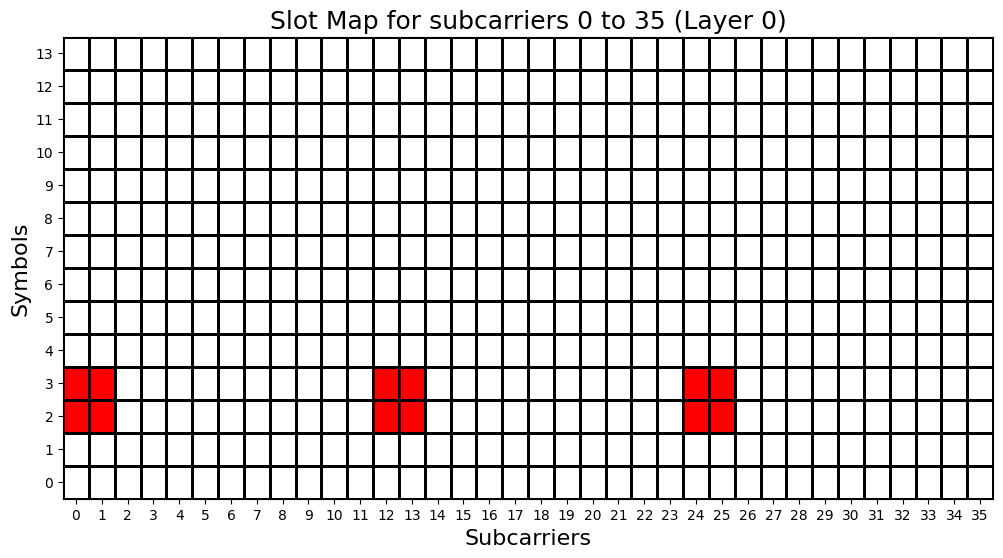
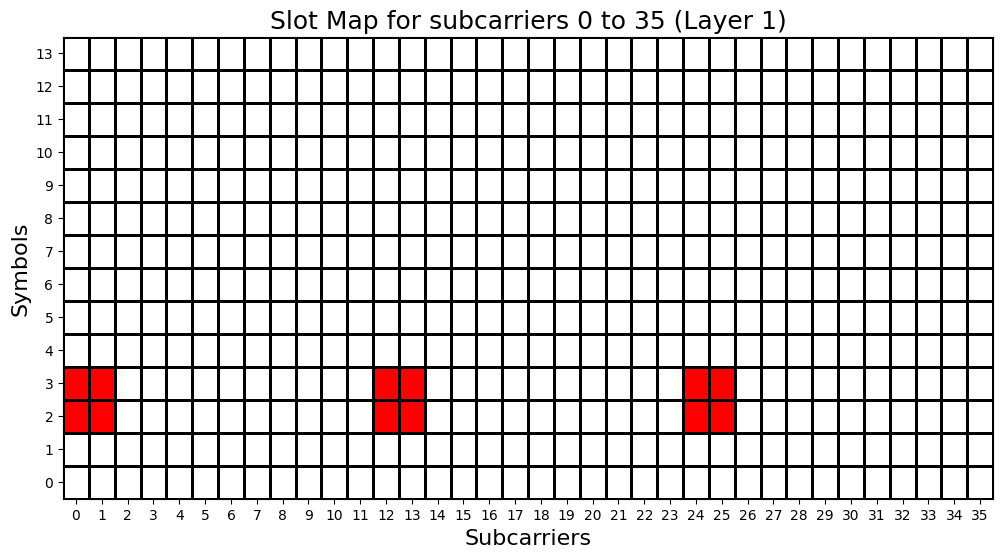
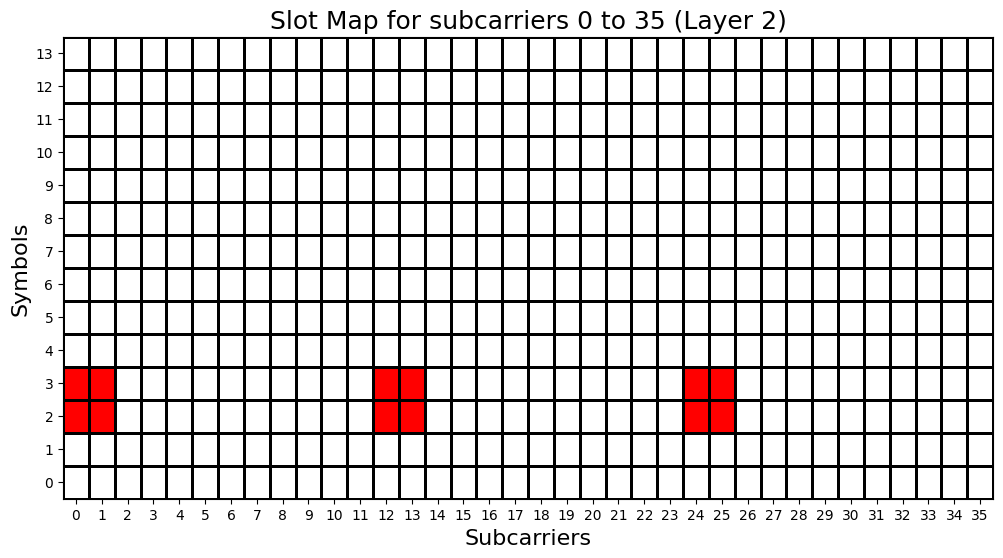
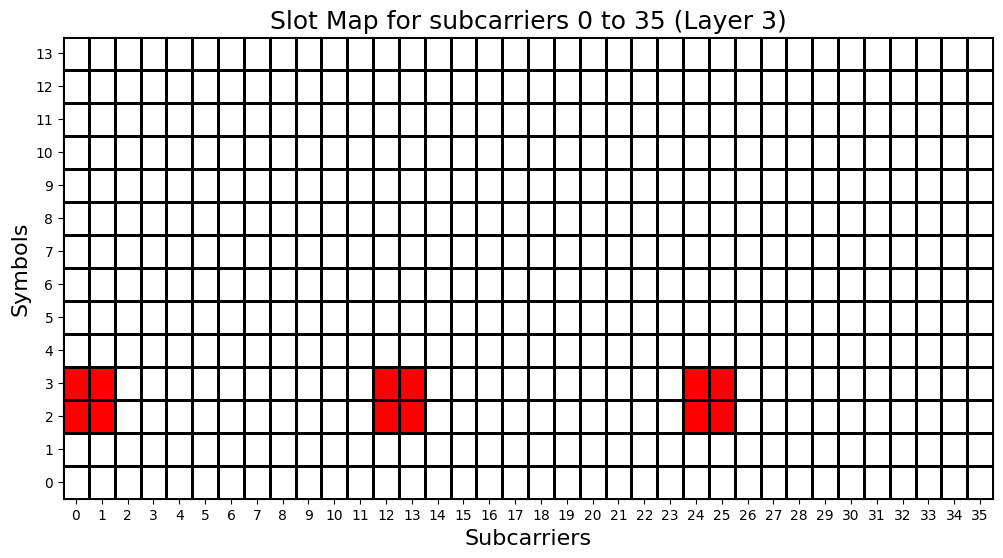
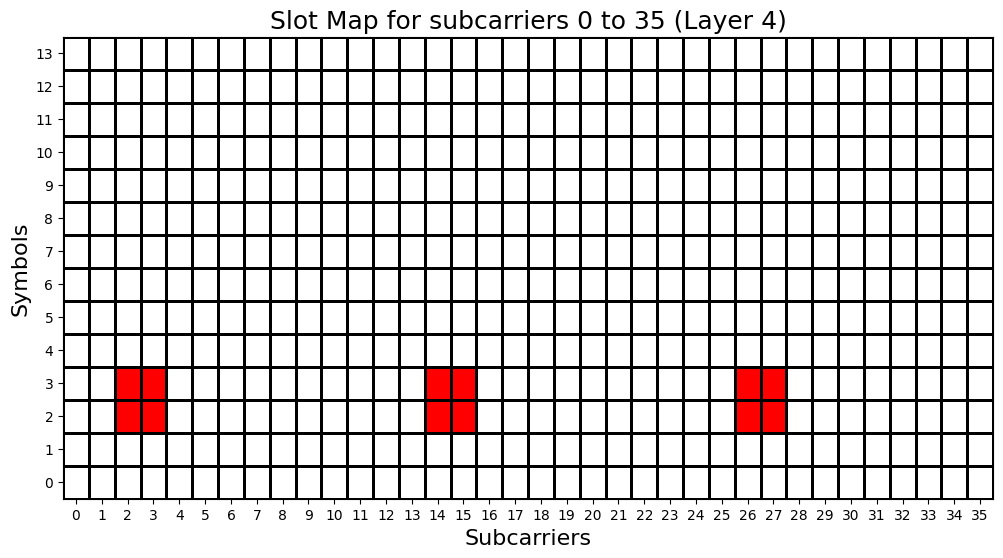
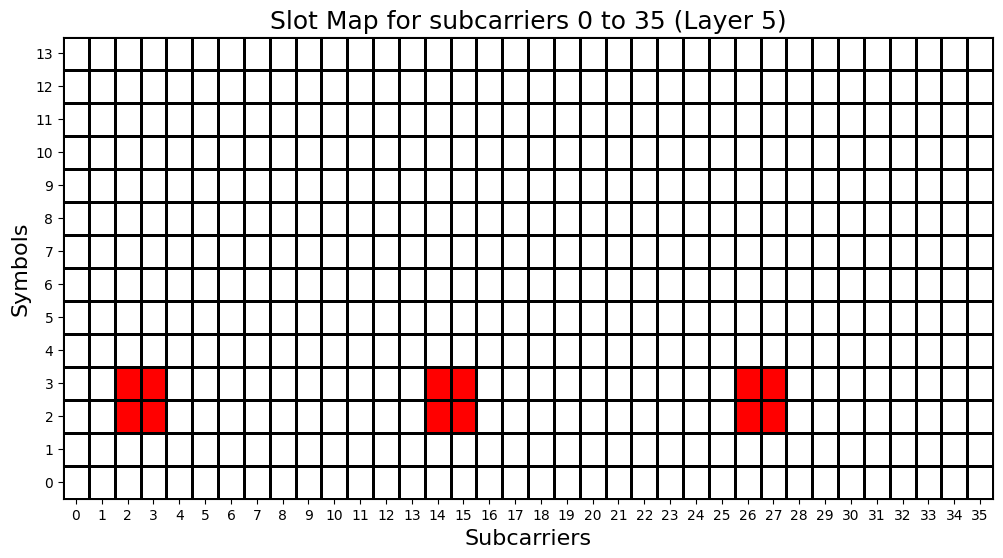
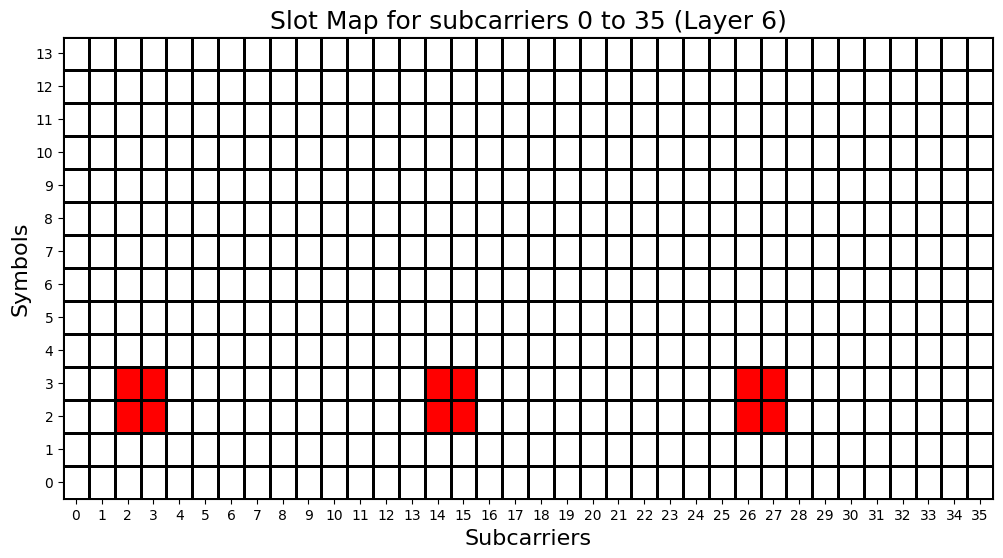
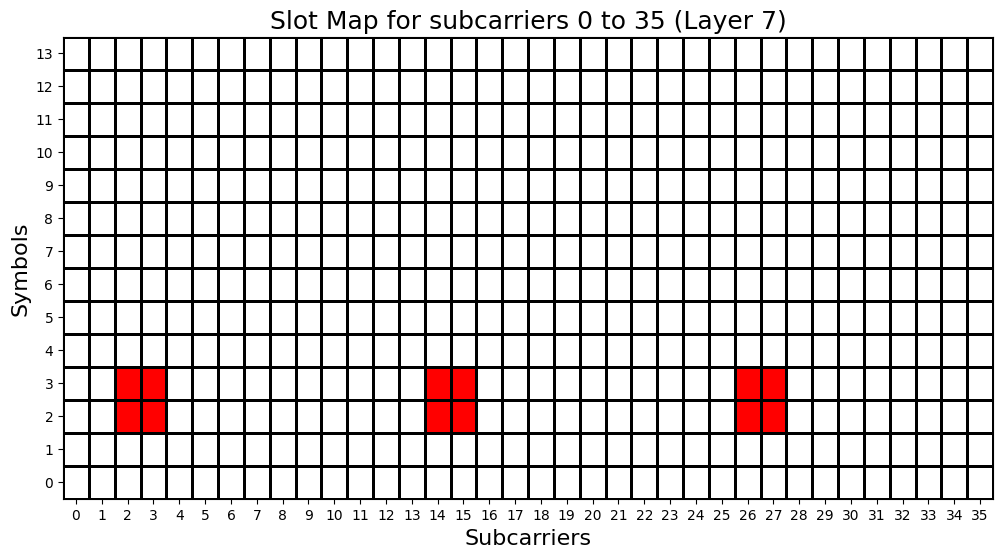
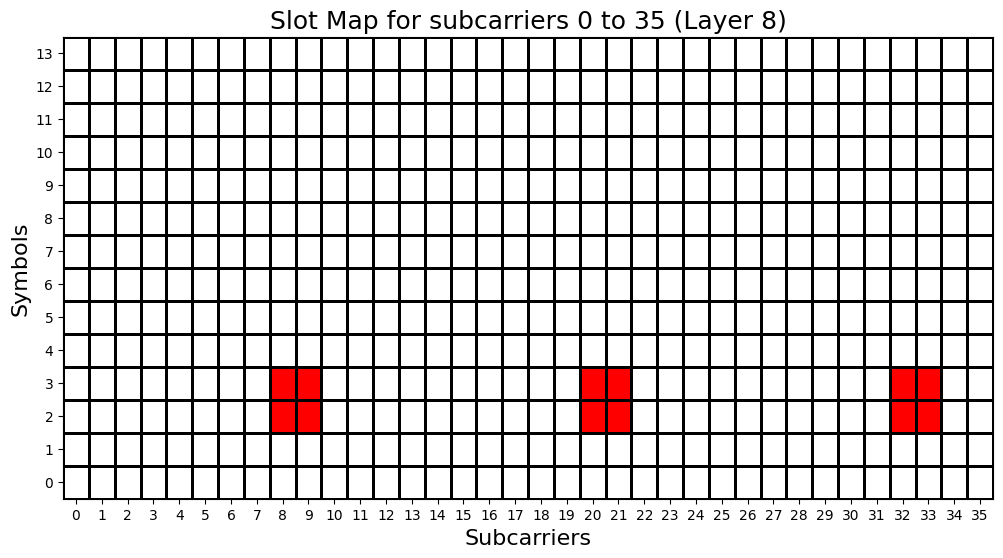
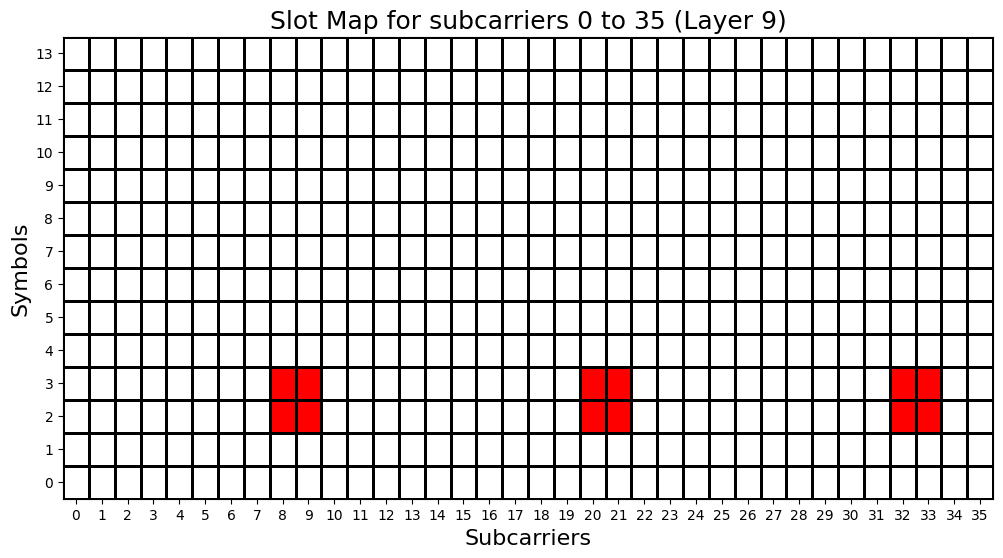
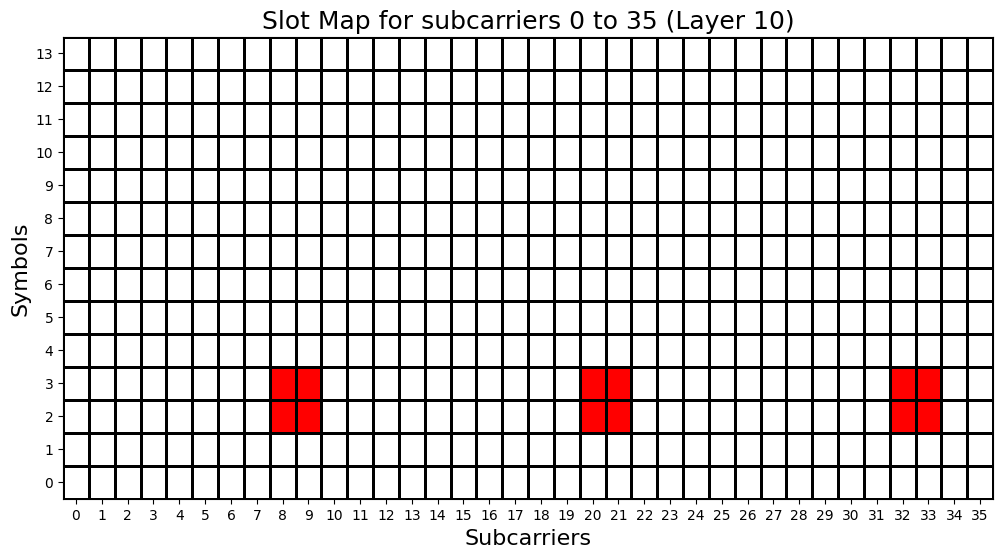
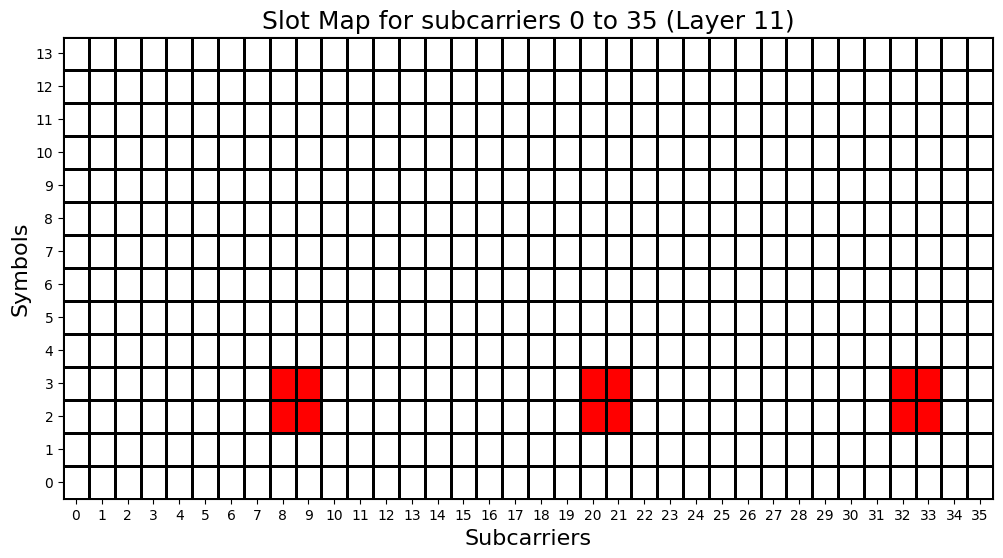
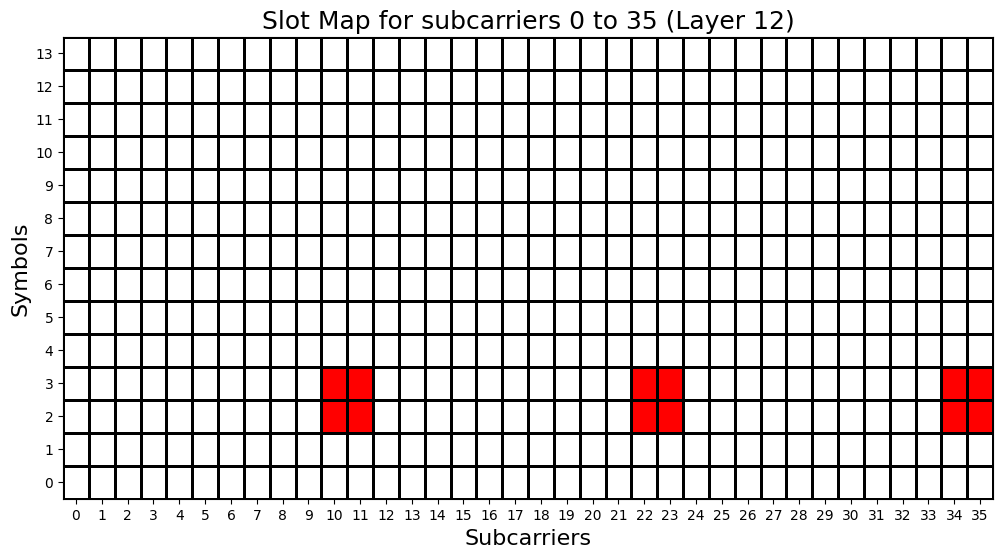
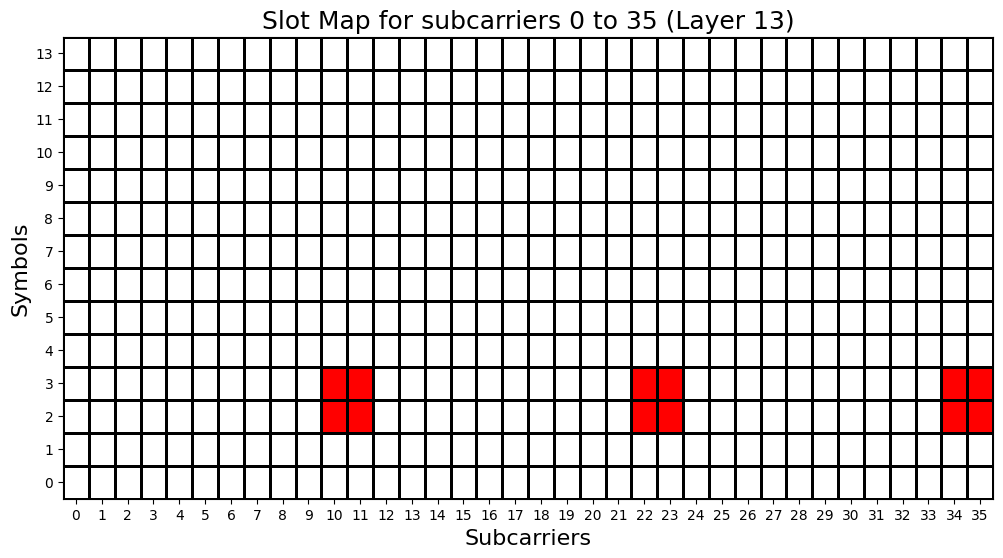
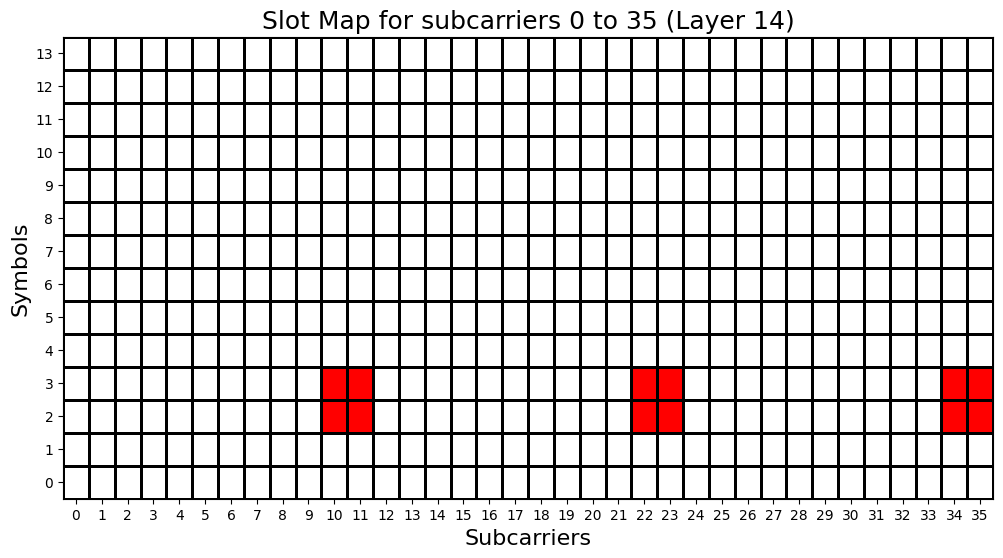
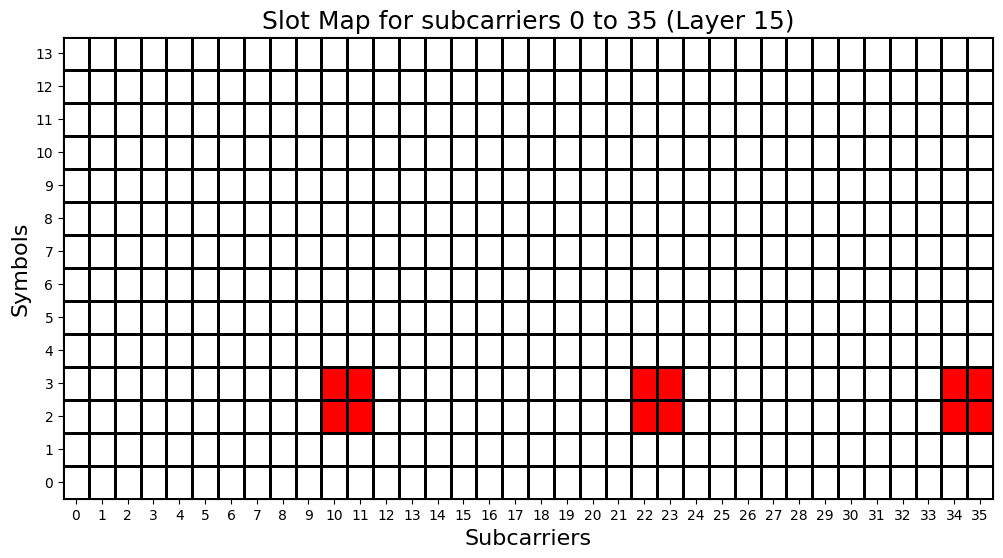
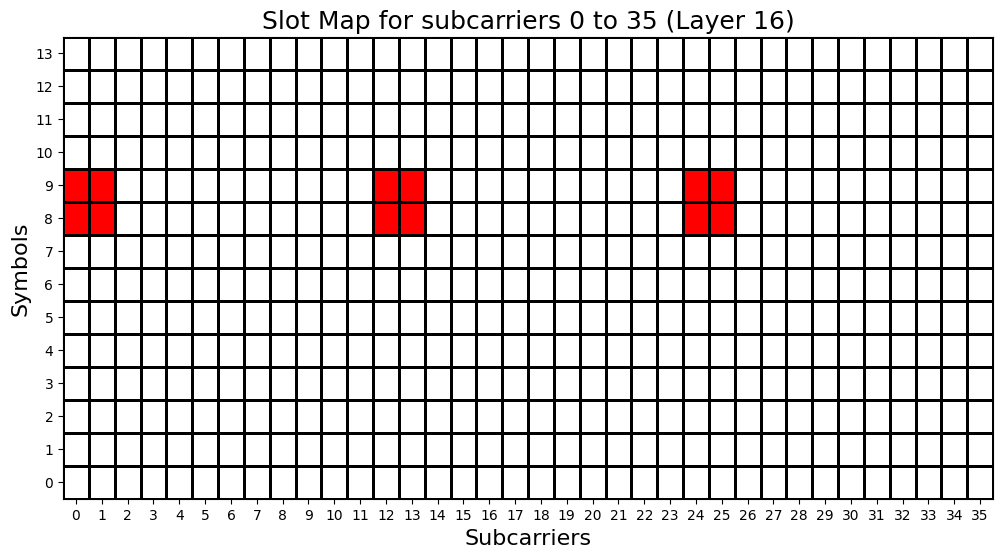
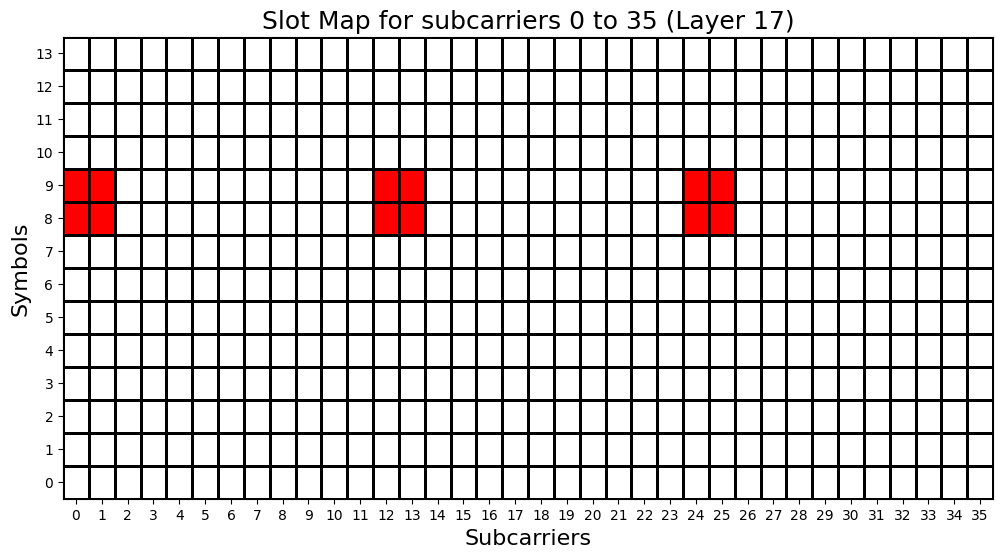
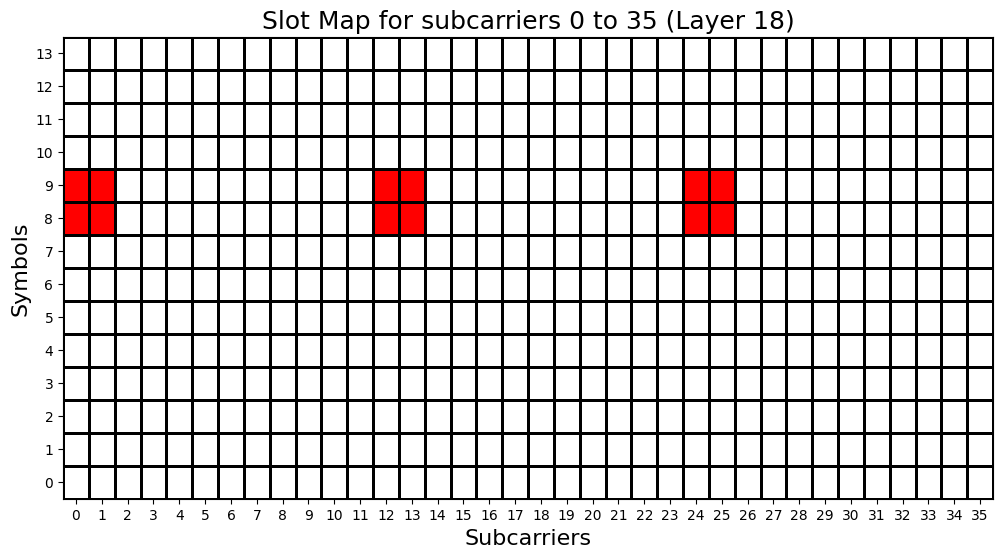
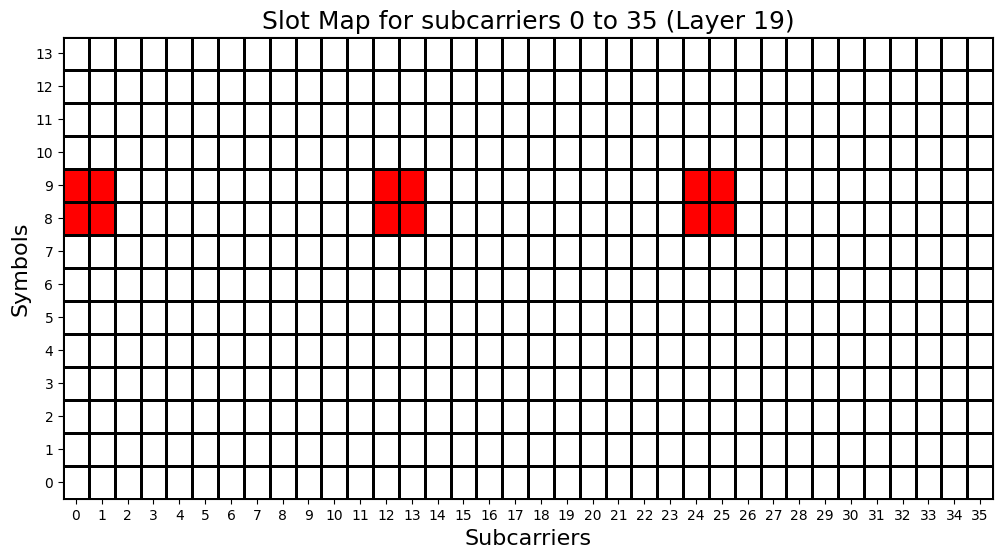
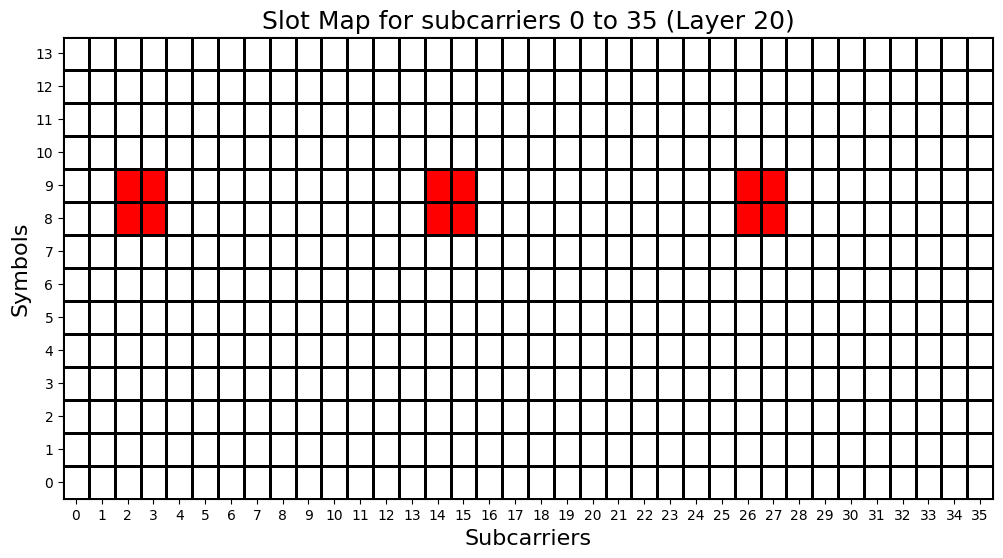
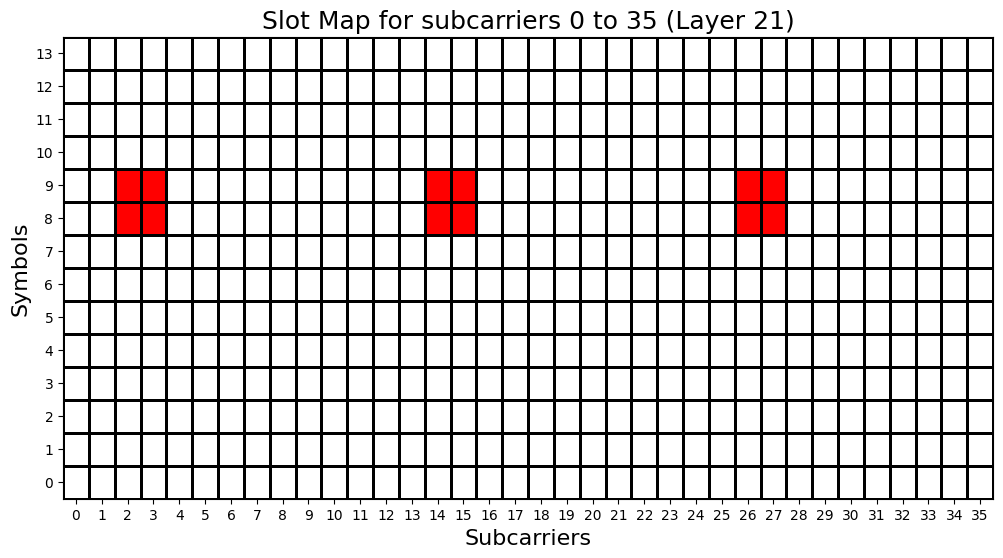
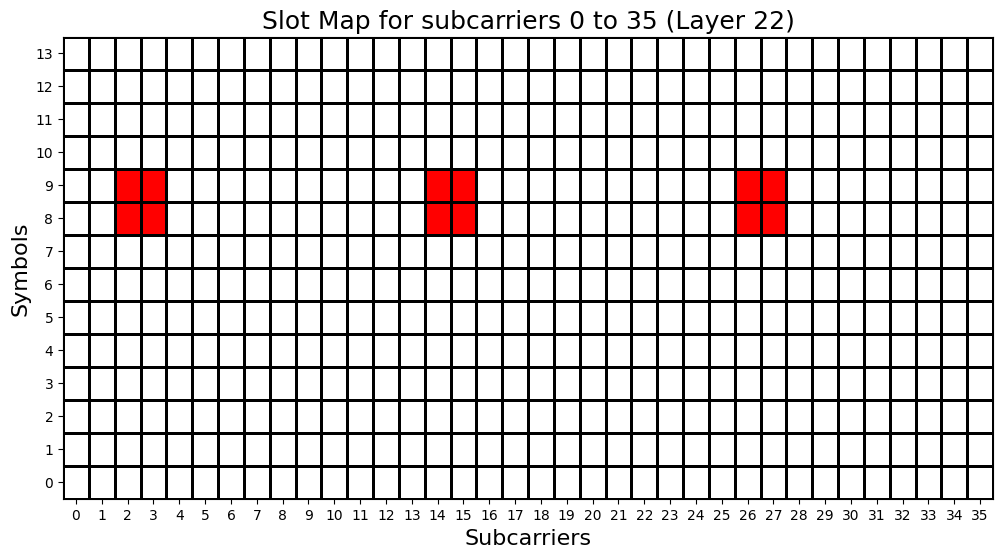
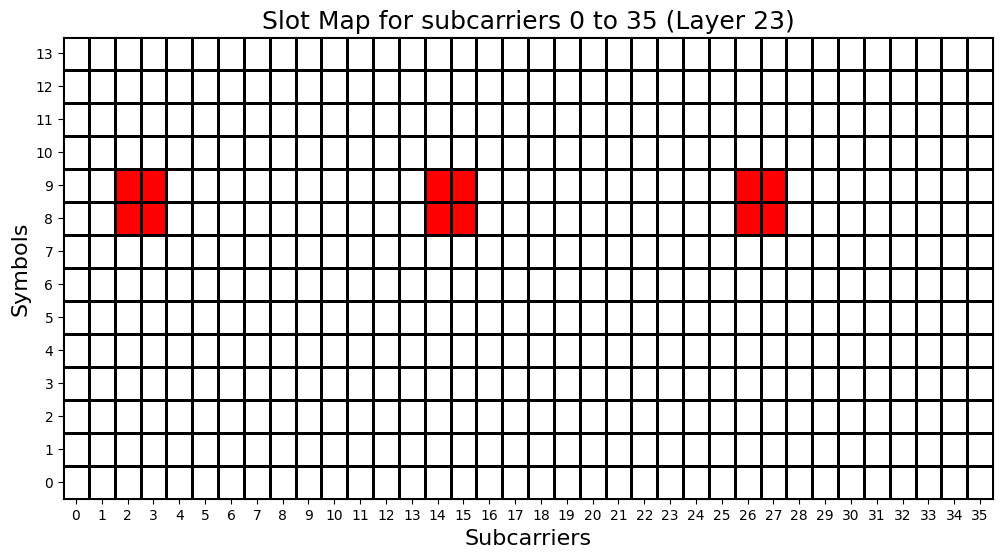
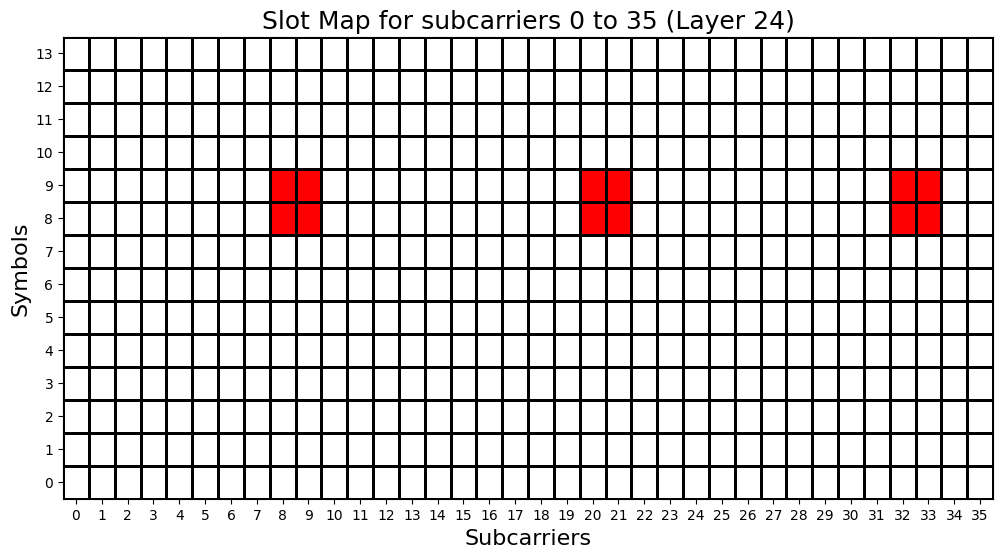
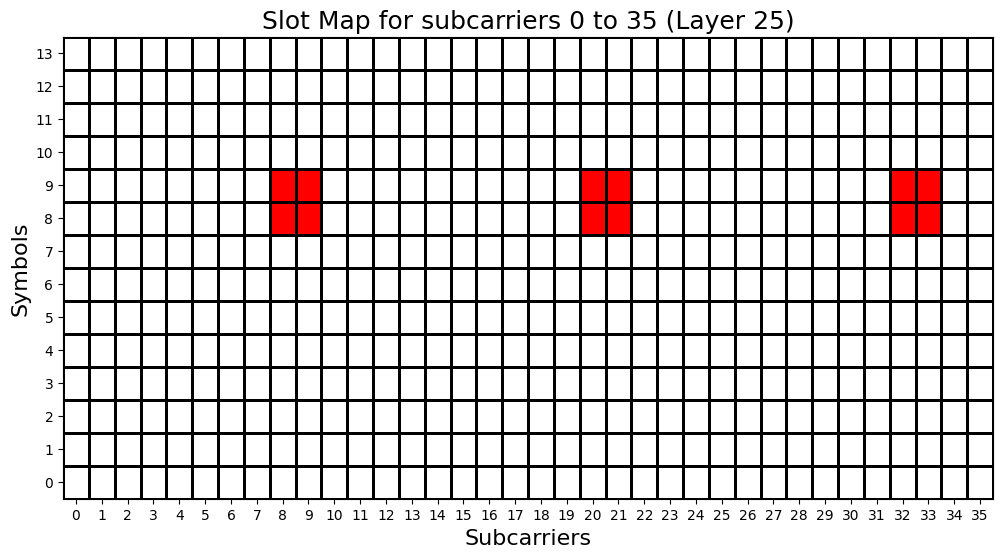
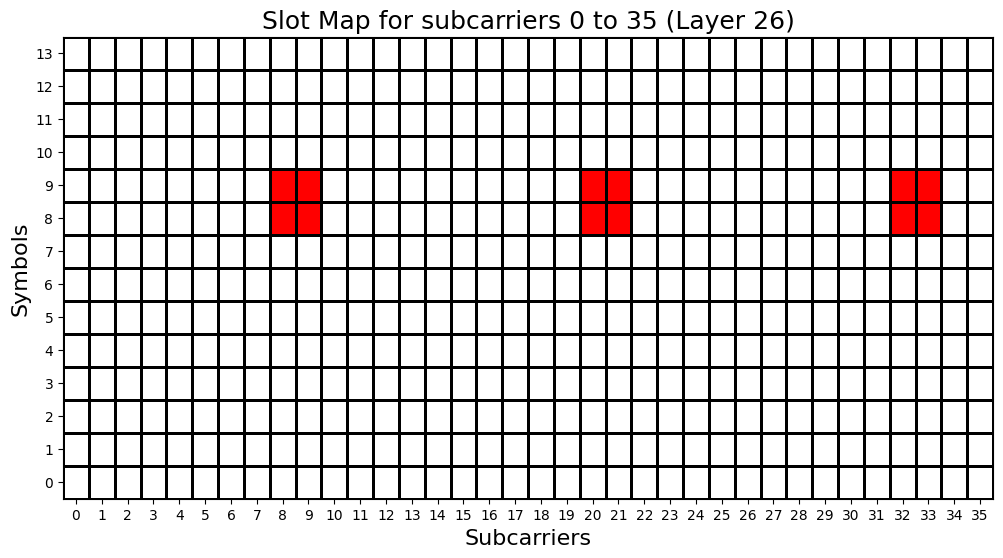
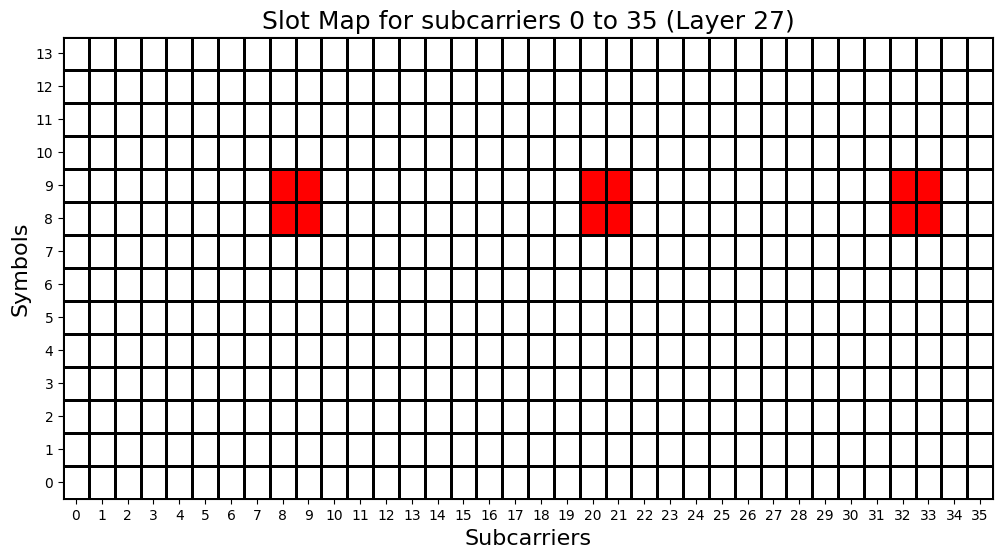
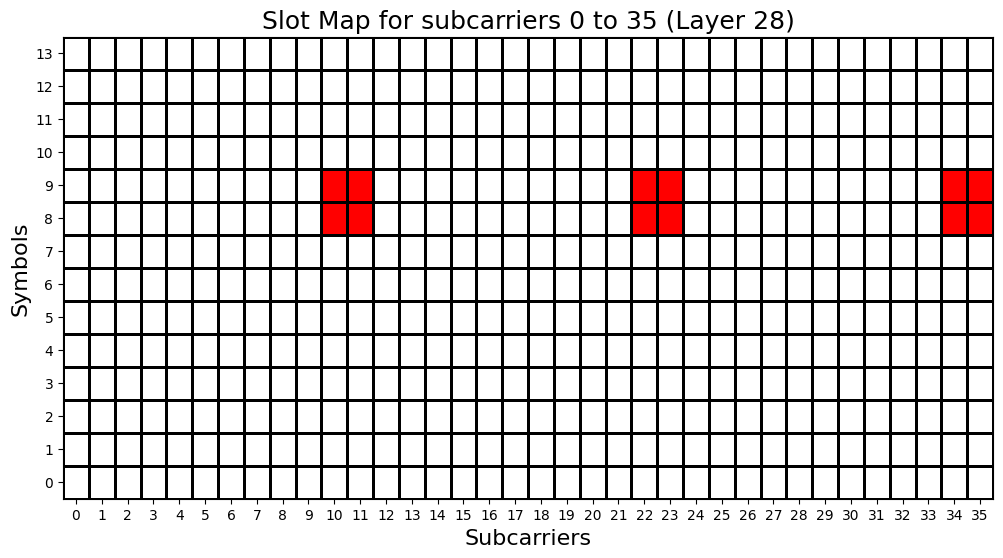
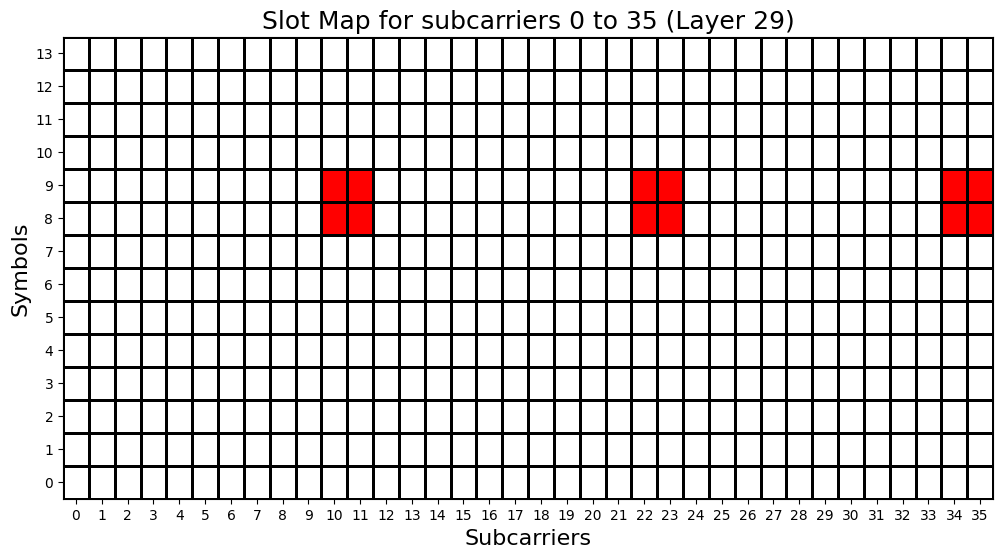
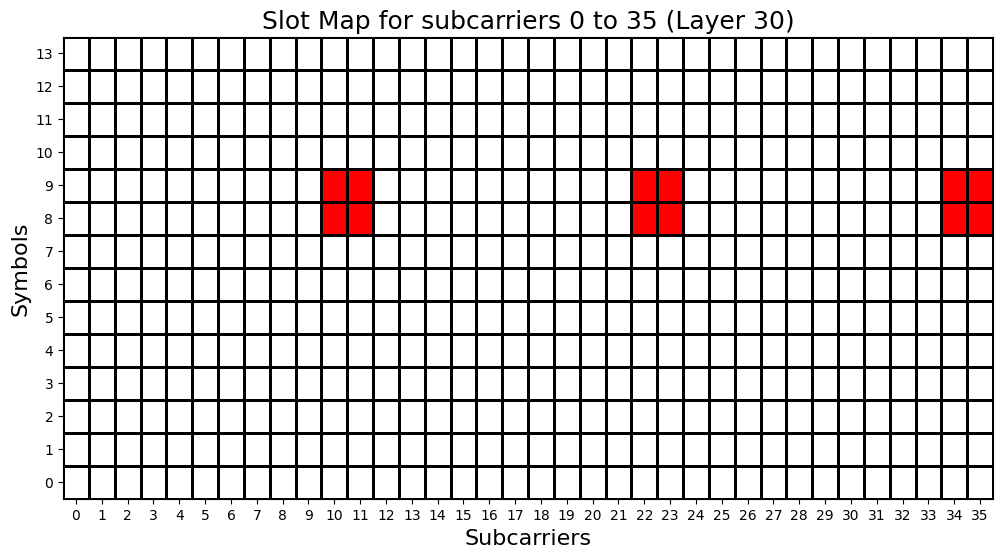
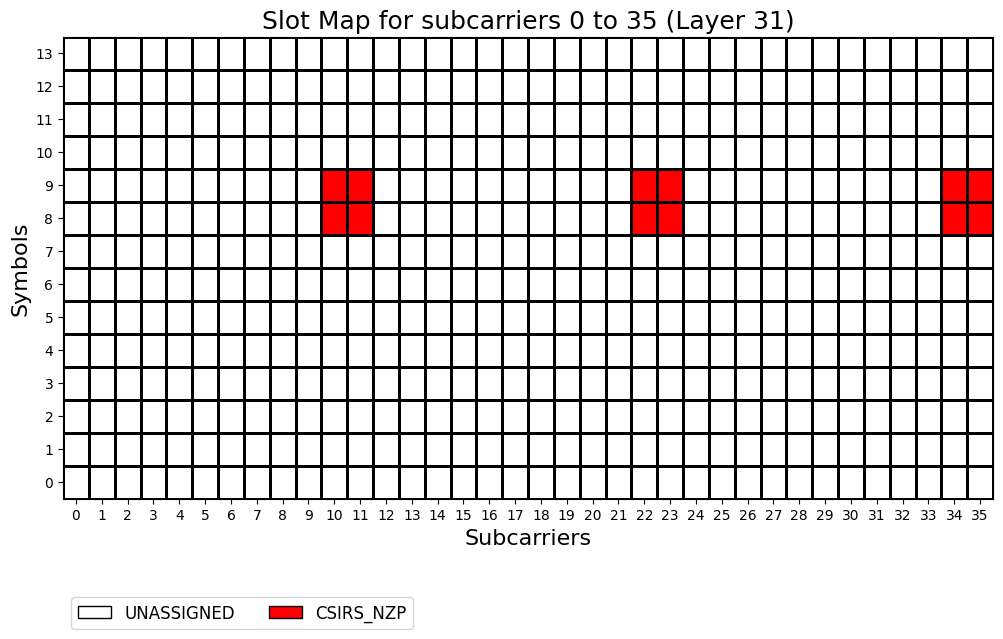
[22]:
# Example for 18th row of 38.211 Table 7.4.1.5.3-1
# 32 ports, 4 of 6 bits set in freqMap, CDM size is 8, and density is 1.
# The CSI-RS resources are at symbols (6,7,8,9) and REs (0,1),(2,3),(8,9) and (10,11).
# Note that this configuration also allows density=.5 which would cause every other
# RB to be skipped for the CSR-RS allocation. (default density is 1)
csiRsConfig = CsiRsConfig([CsiRsSet("NZP", bwp, symbols=[6], numPorts=32, freqMap="110011", cdmSize=8)])
csiRsConfig.print()
grid = bwp.createGrid(csiRsConfig.numPorts)
csiRsConfig.populateGrid(grid)
print("Grid Stats:")
stats = grid.getStats()
for key, value in stats.items(): print(" %s: %d"%(key, value))
grid.drawMap(ports=range(csiRsConfig.numPorts), reRange=(0,36))
CSI-RS Configuration: (1 Resource Sets)
CSI-RS Resource Set:(1 NZP Resources)
Resource Set ID: 0
Resource Type: periodic
Resource Blocks: 24 RBs starting at 0
Slot Period: 4
Bandwidth Part:
Resource Blocks: 24 RBs starting at 0 (288 subcarriers)
Subcarrier Spacing: 15 KHz
CP Type: normal
bandwidth: 4.32 MHz
symbolsPerSlot: 14
slotsPerSubFrame: 1
nFFT: 2048
frameNo: 0
slotNo: 0
CSI-RS:
resourceId: 0
numPorts: 32
cdmSize: 8 (cdm8-FD2-TD4)
density: 1
RE Indexes: 0 2 8 10
Symbol Indexes: 6
Table Row: 18
Slot Offset: 0
Power: 0 db
scramblingID: 0
Grid Stats:
GridSize: 129024
UNASSIGNED: 122880
CSIRS_NZP: 6144
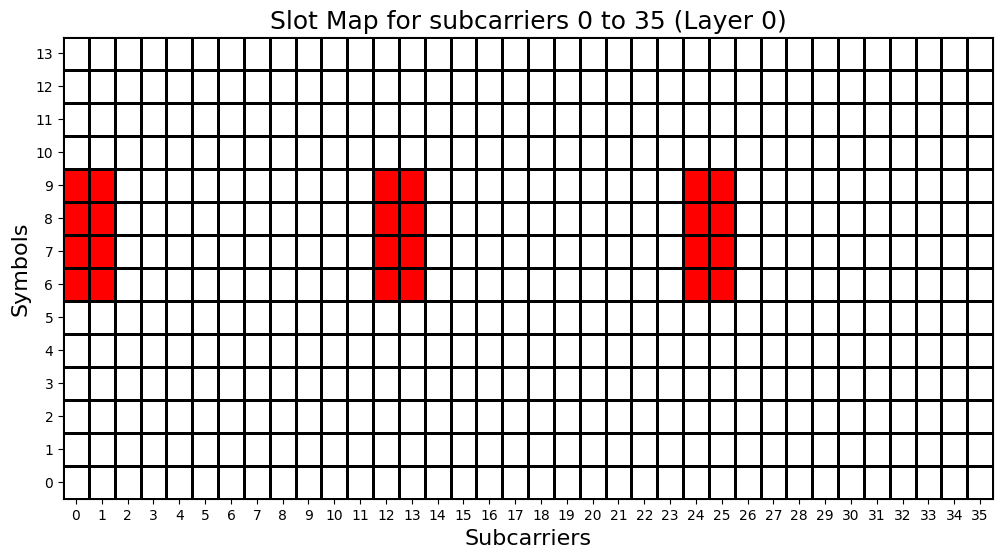
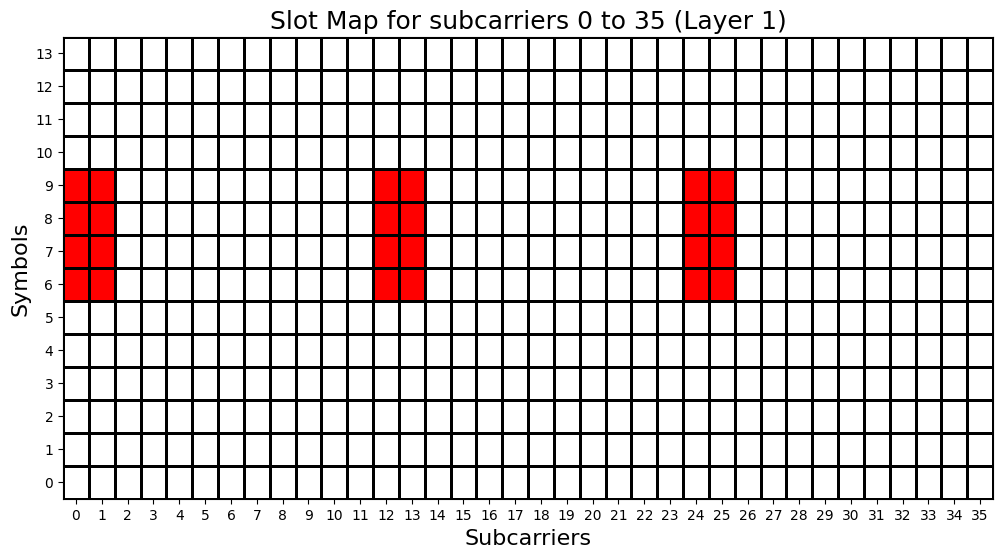
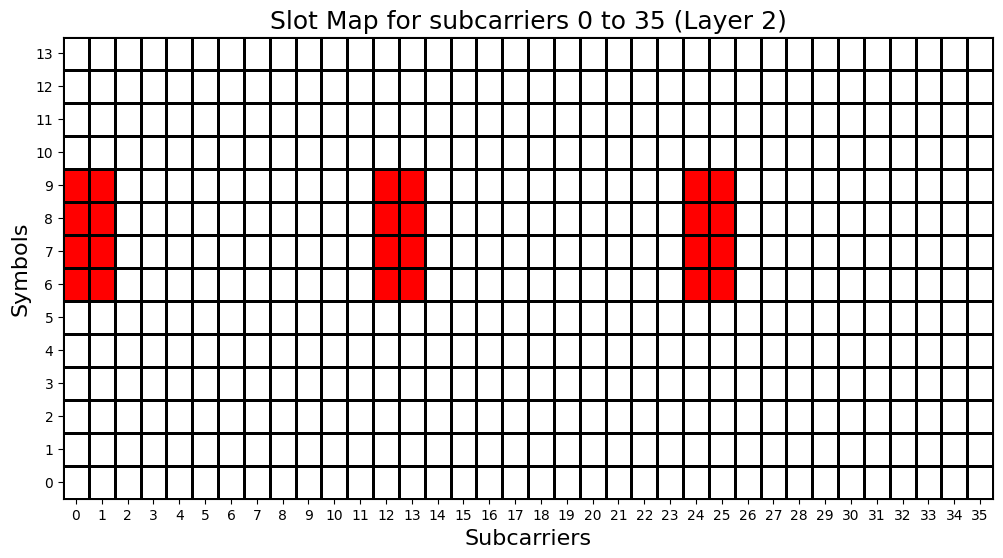
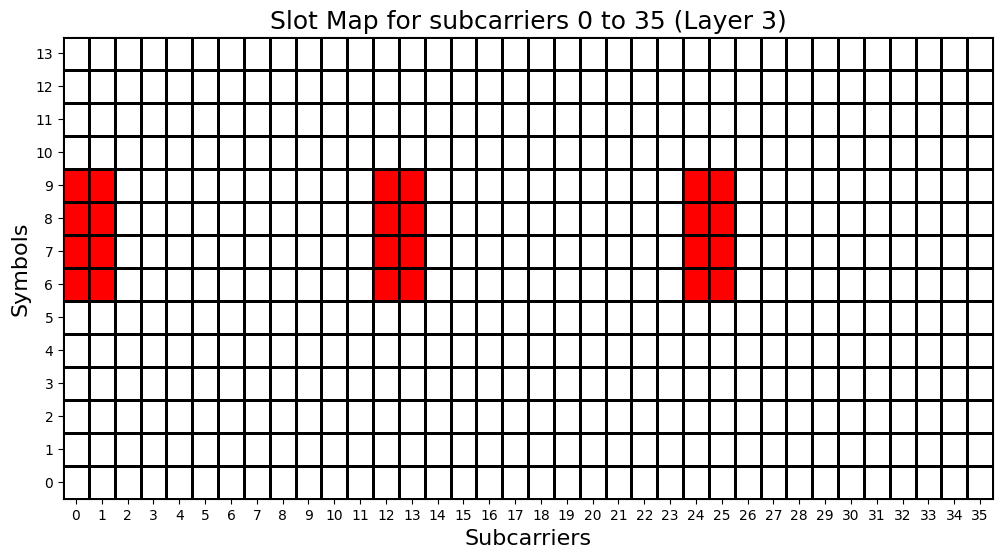
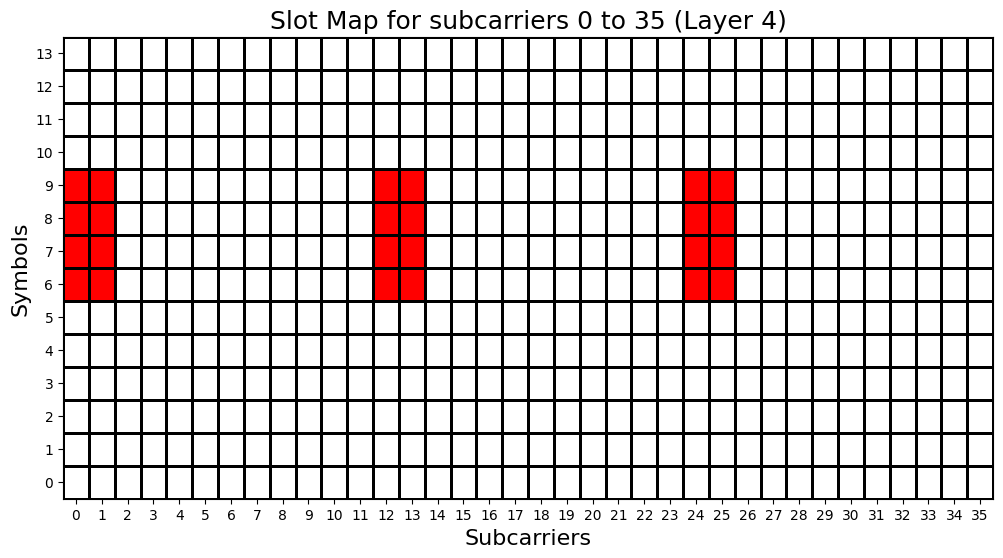
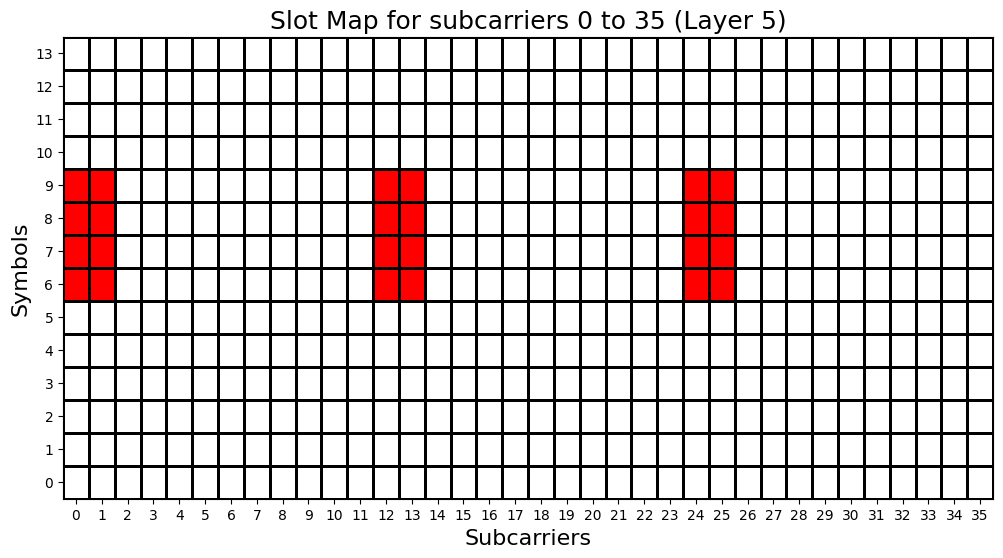
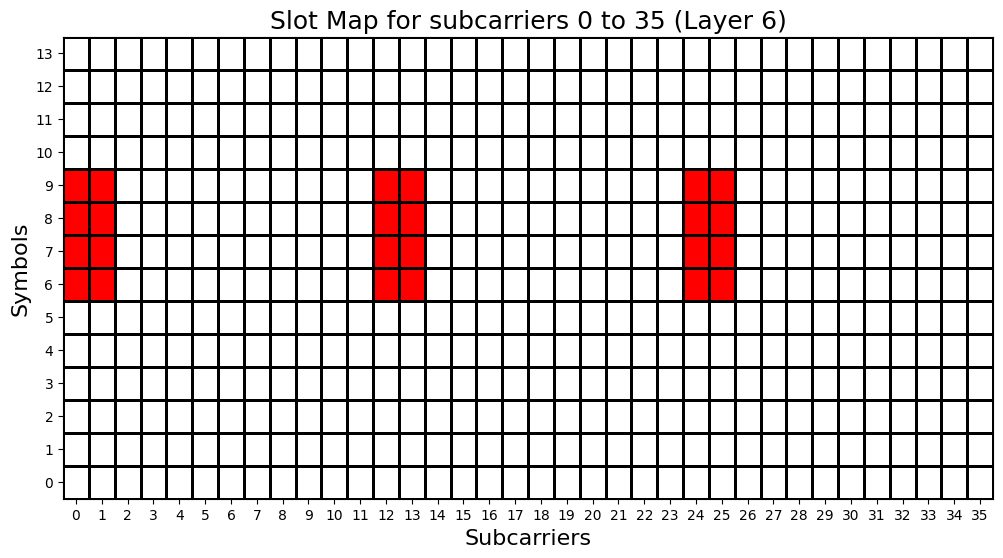
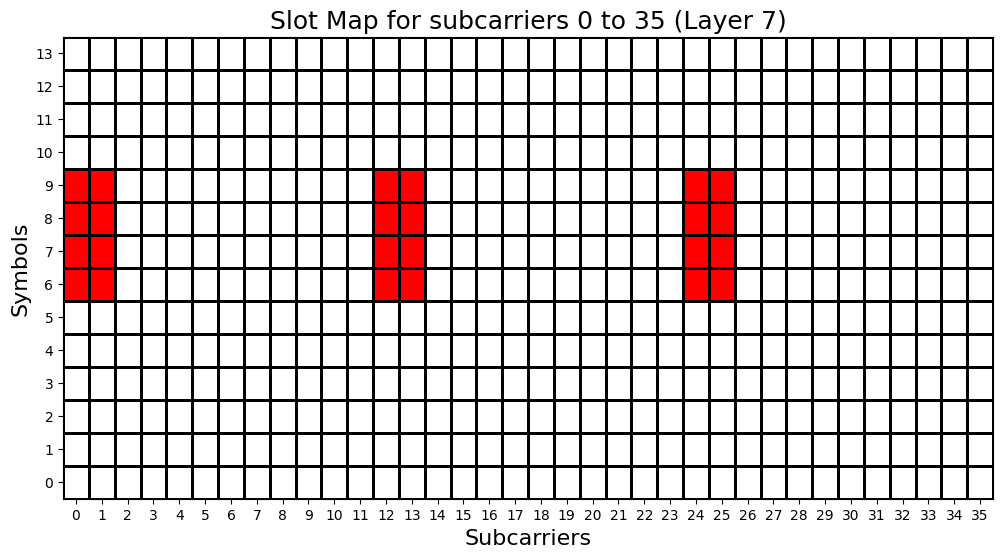
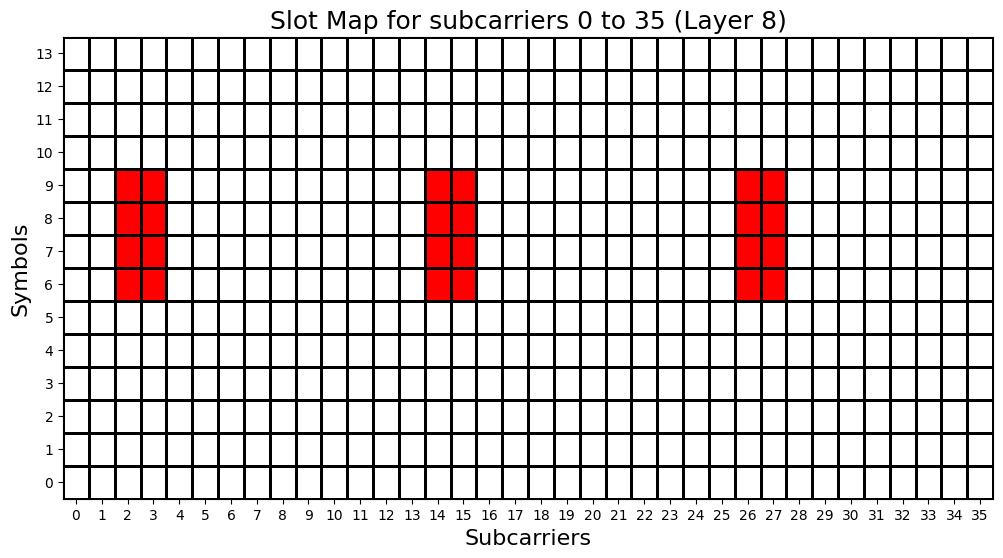
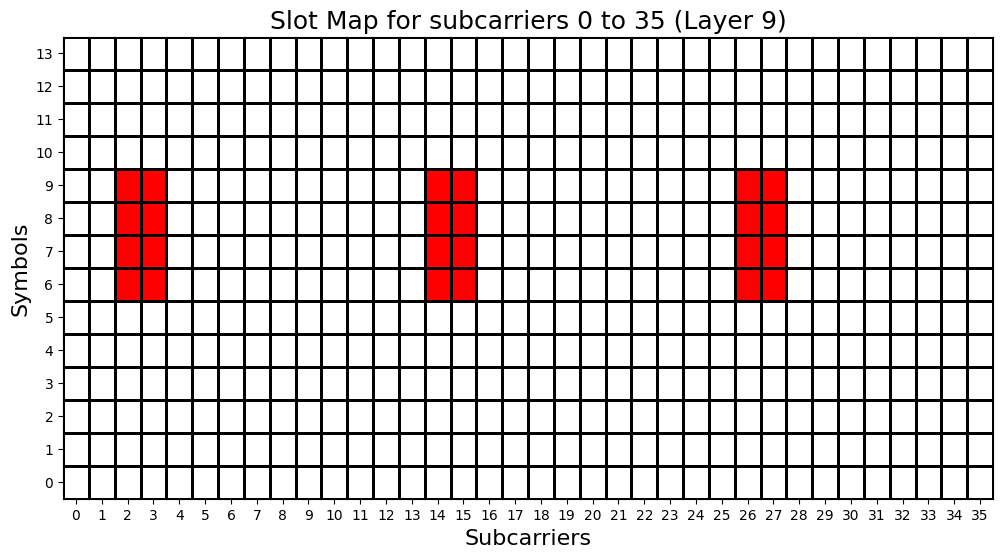
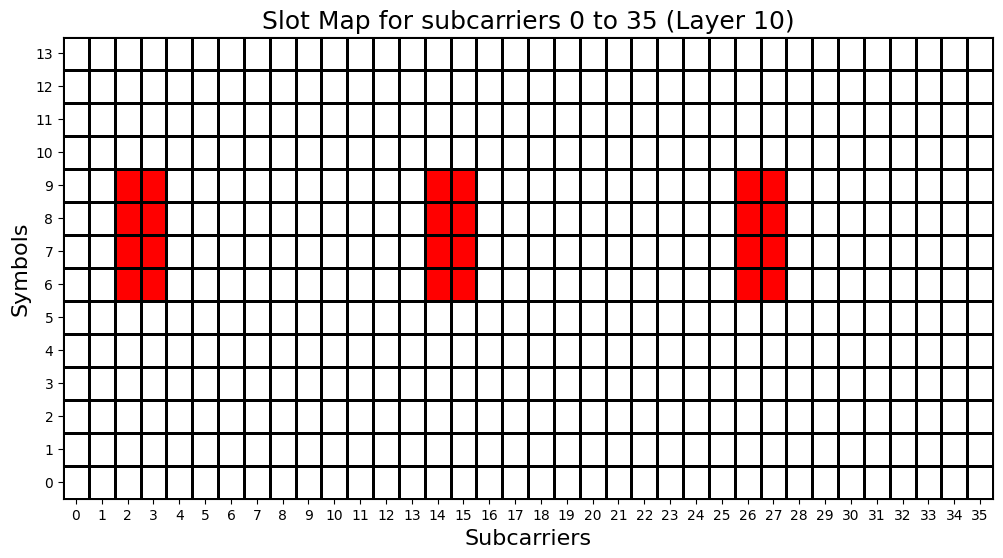
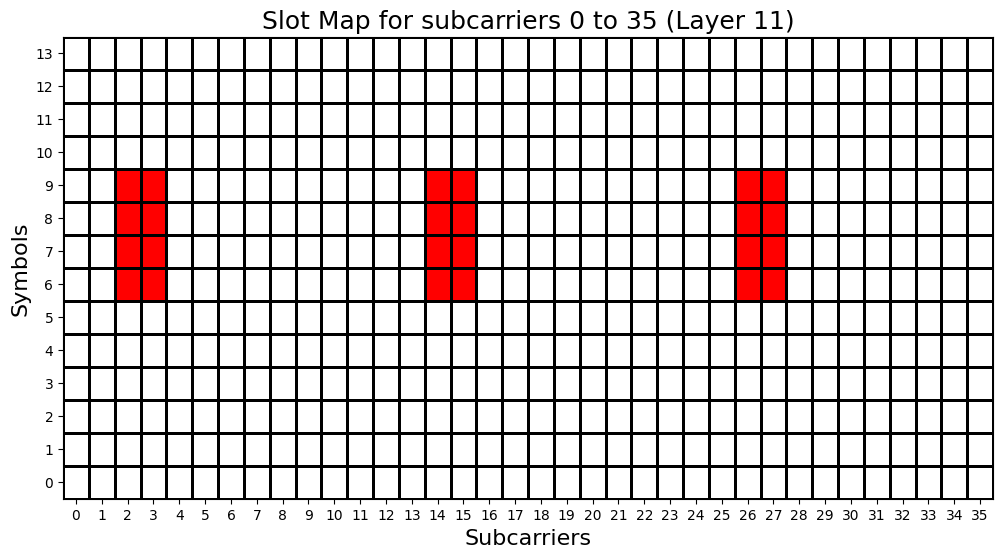
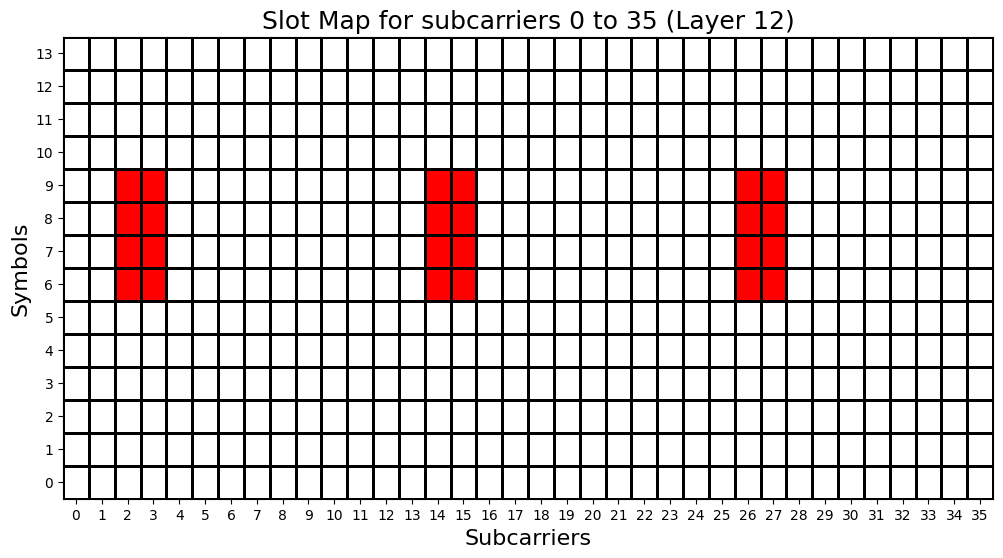
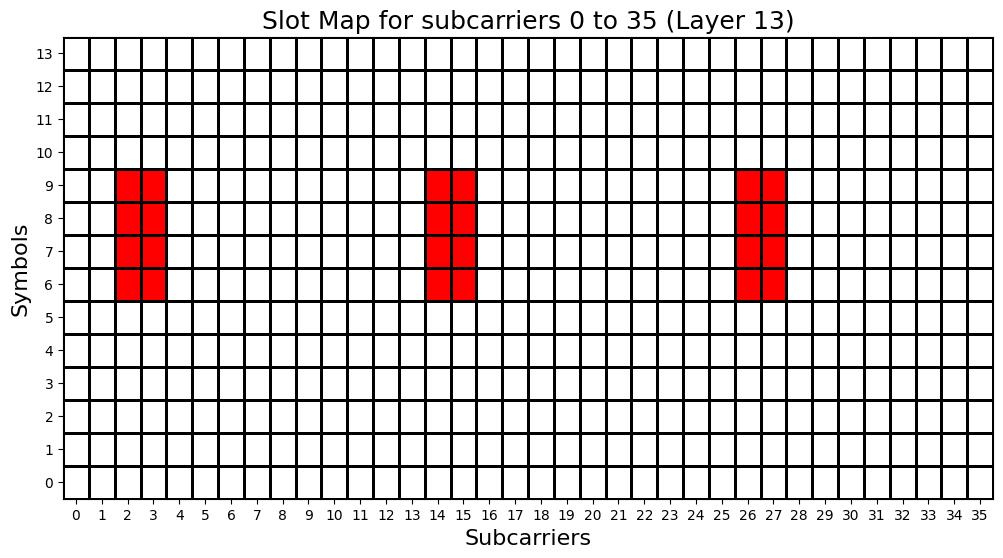
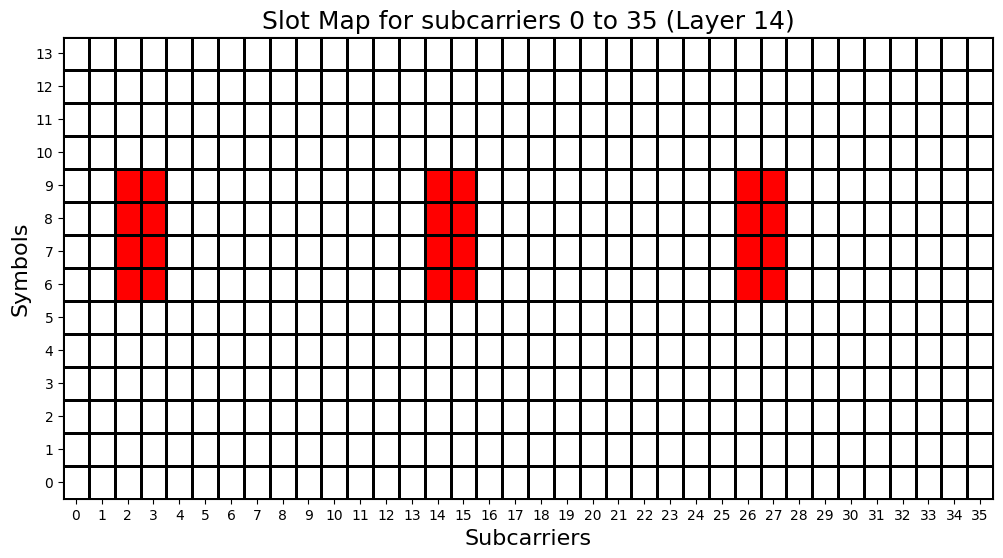
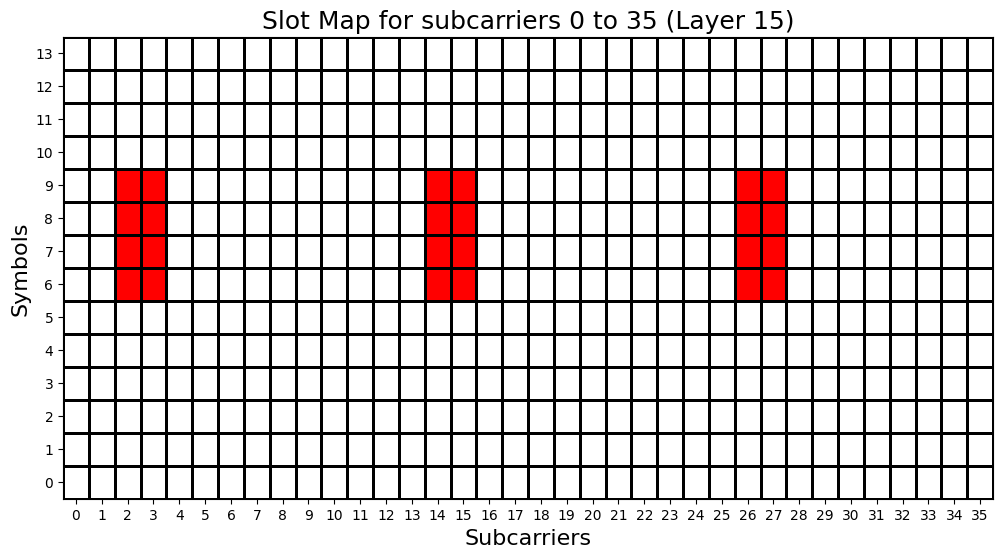
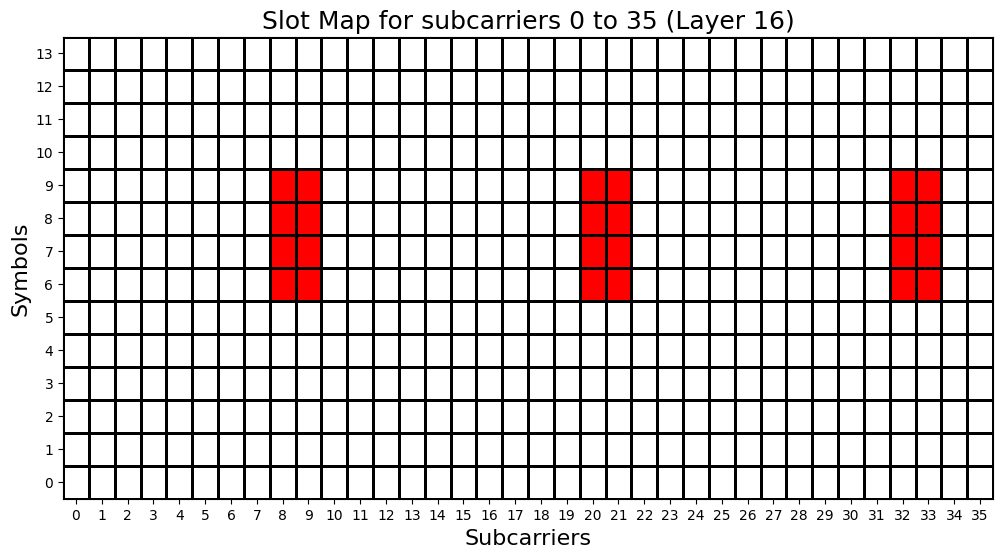
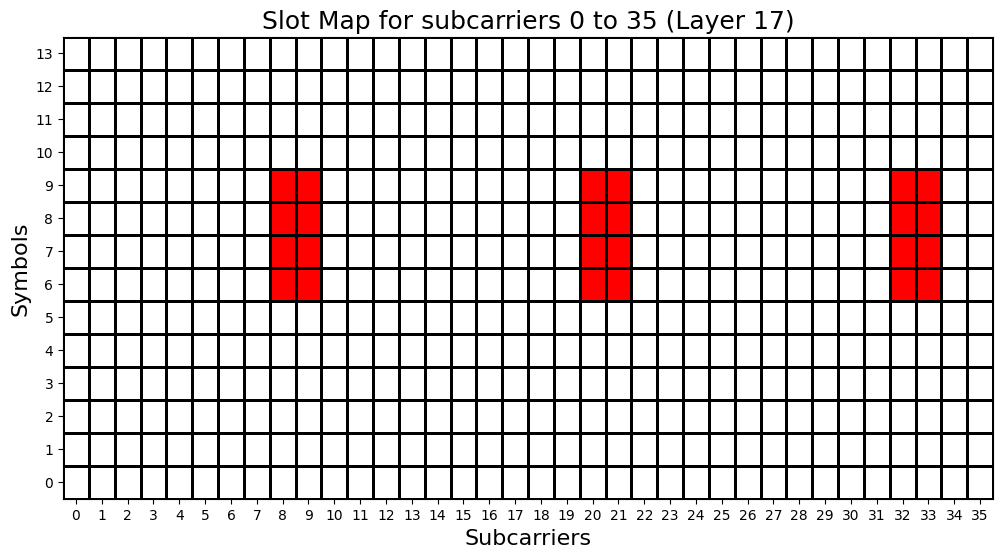
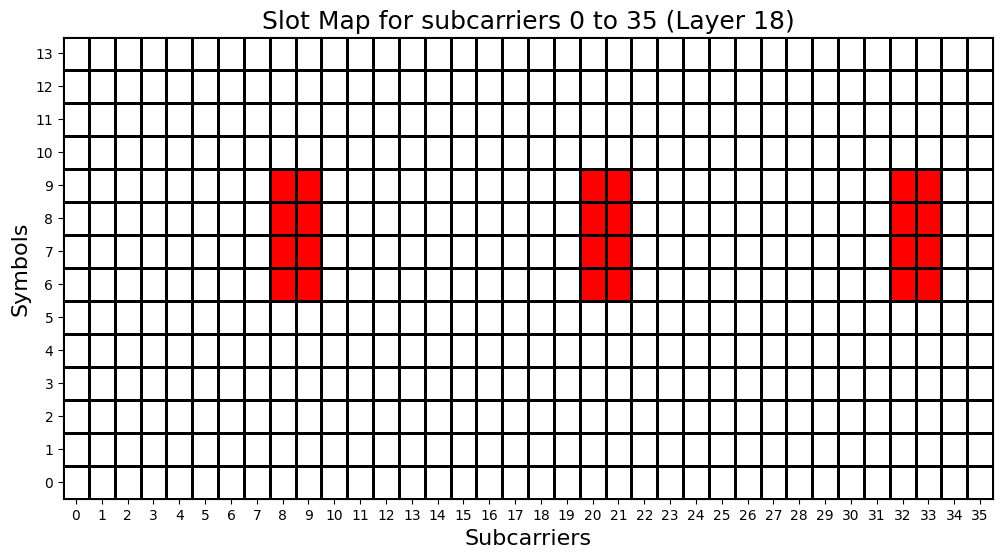
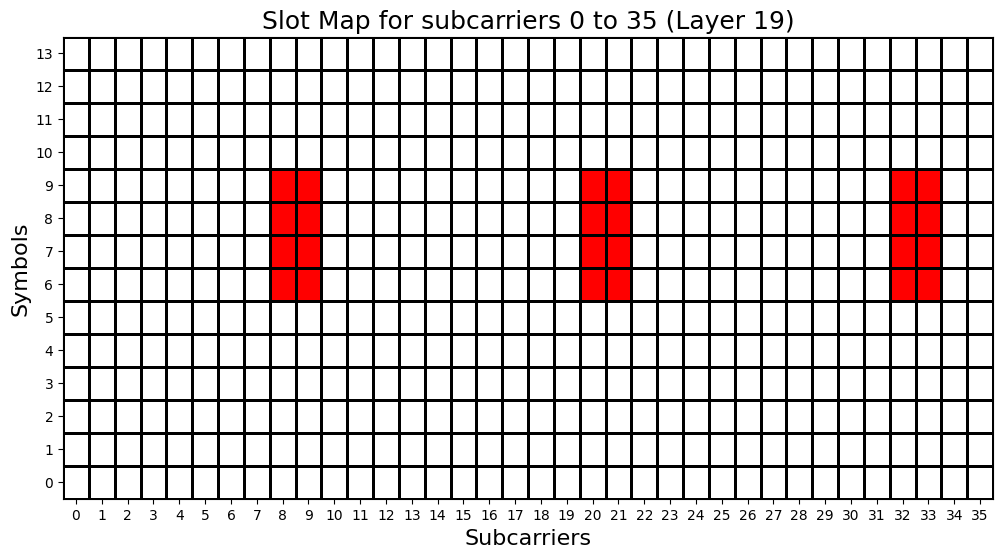
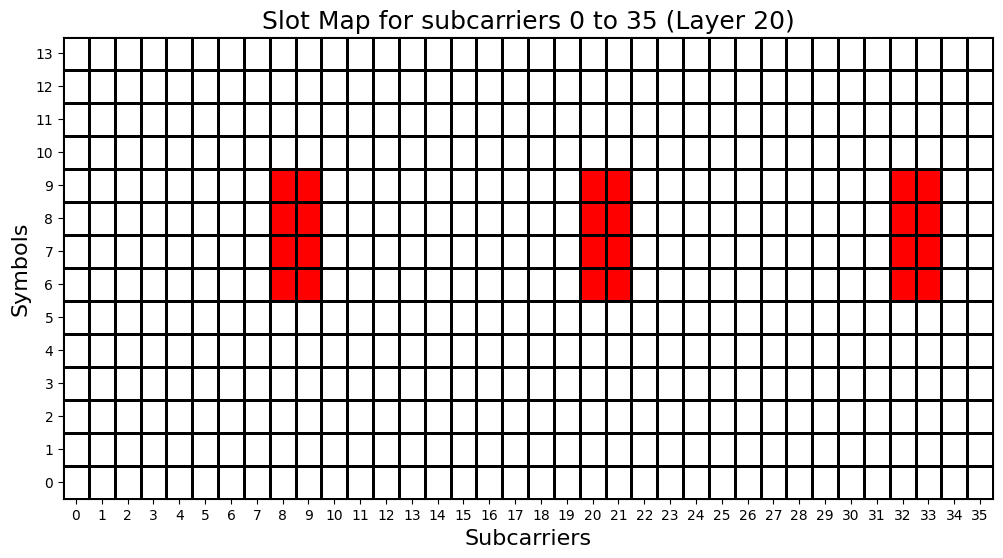
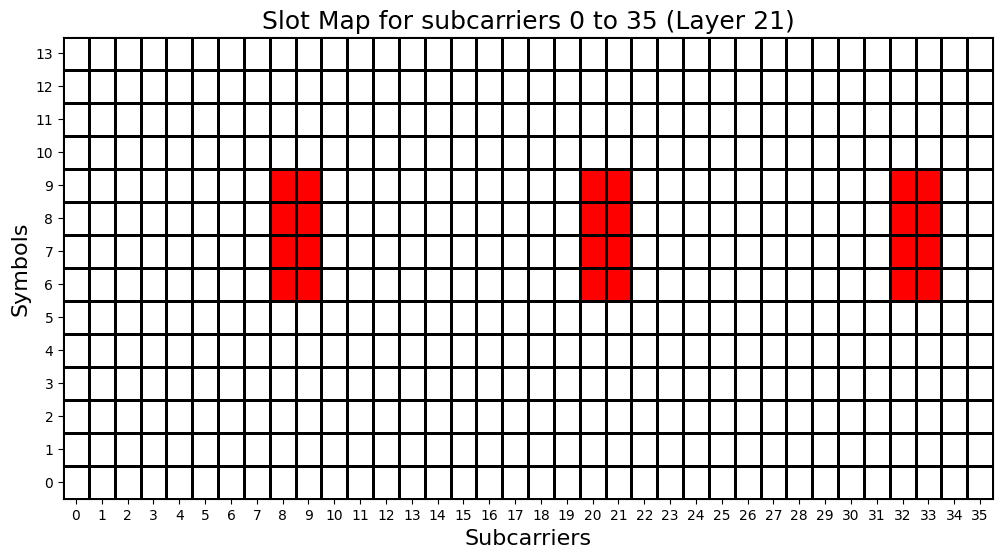
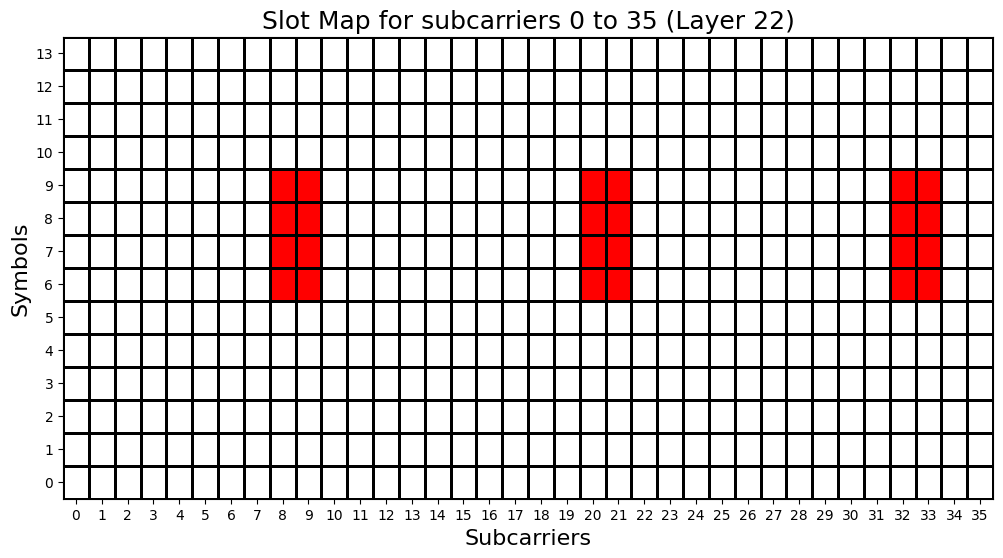
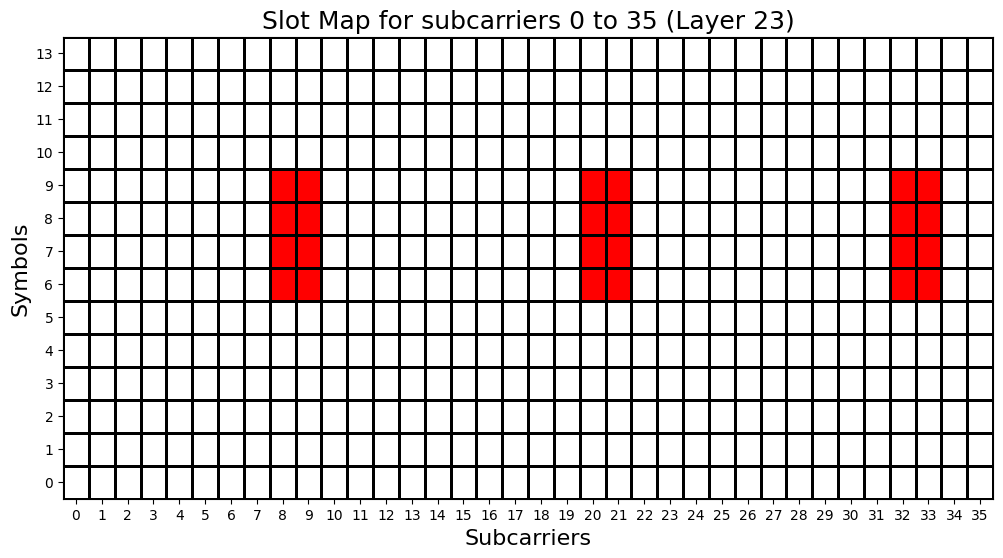
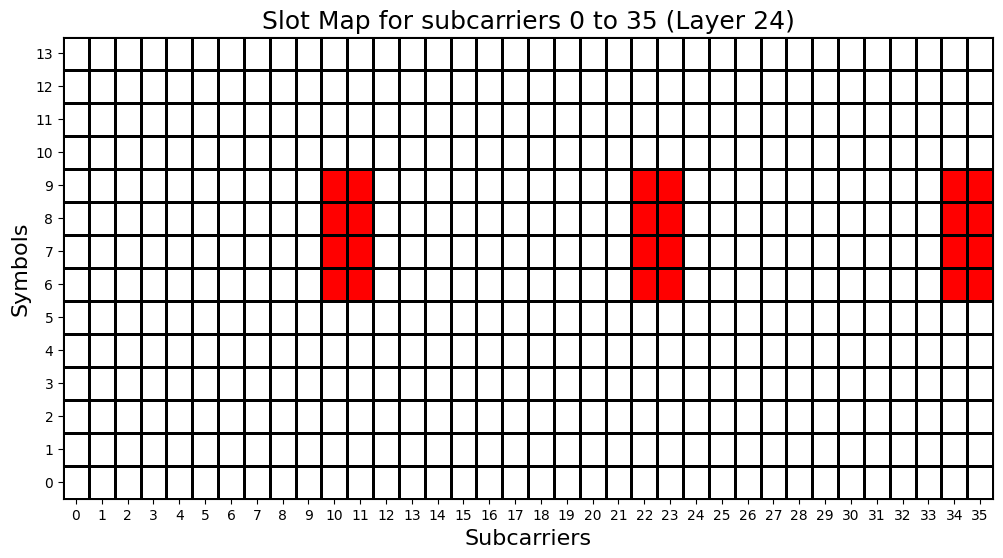
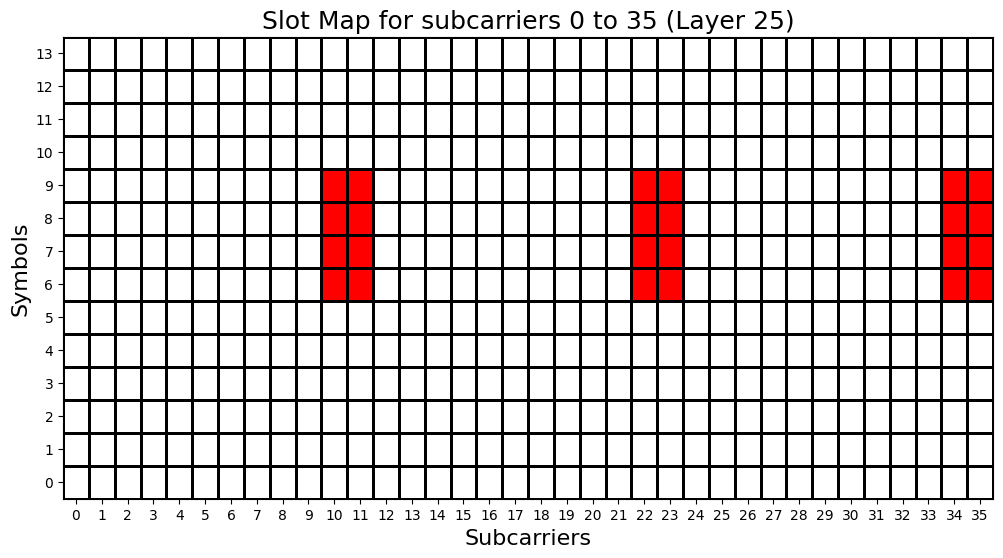
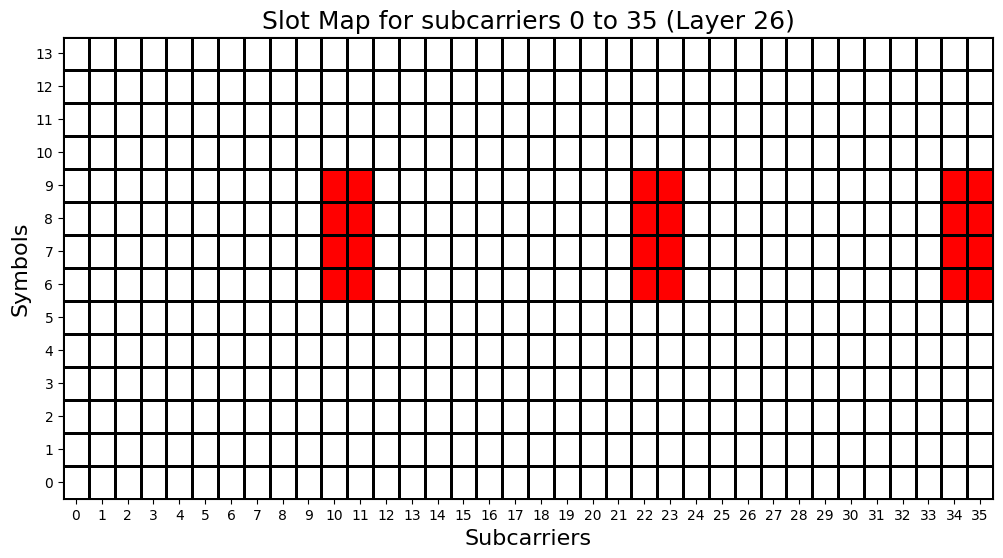
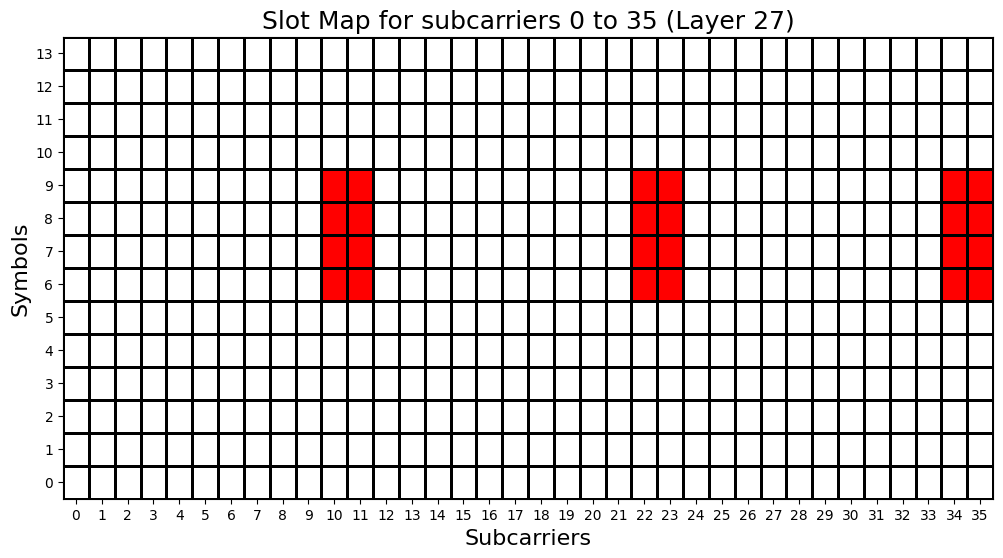
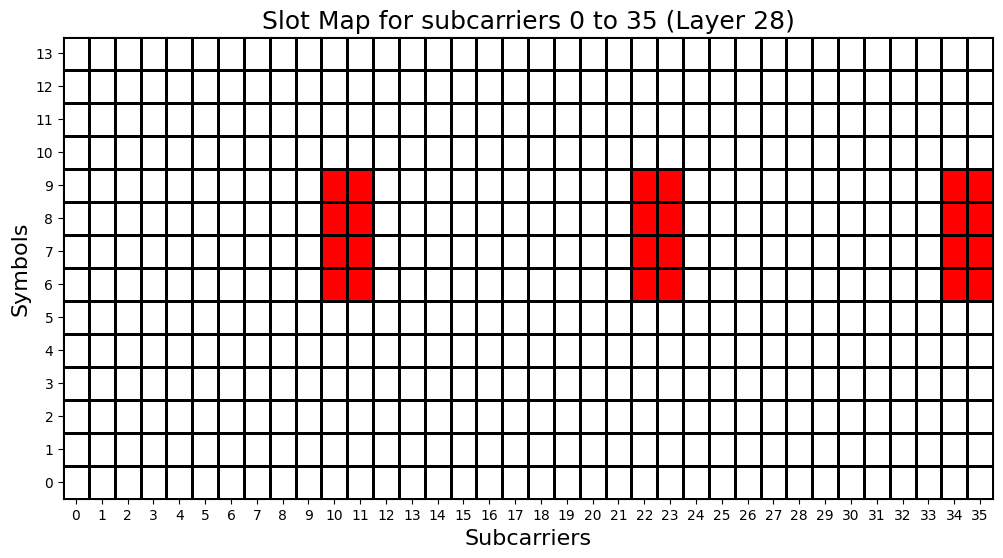
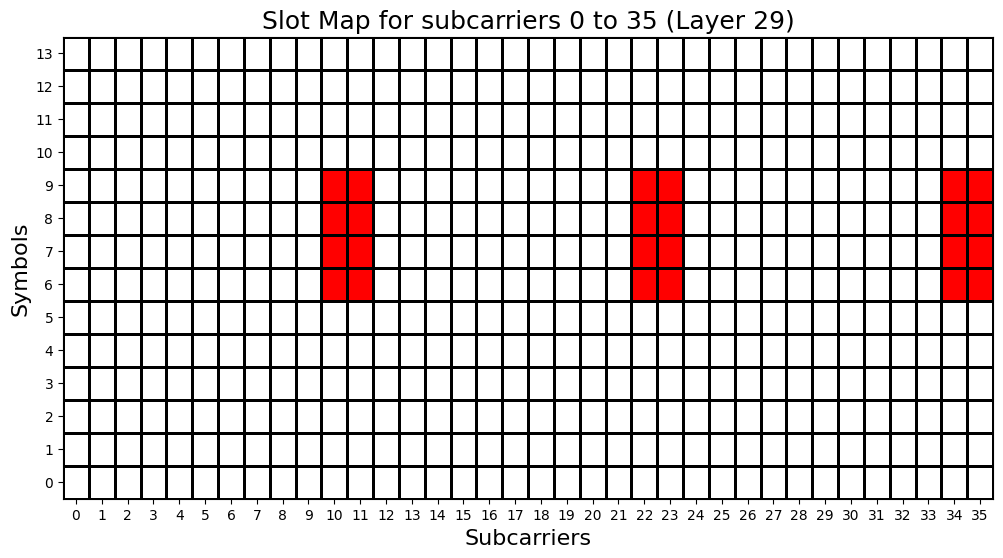
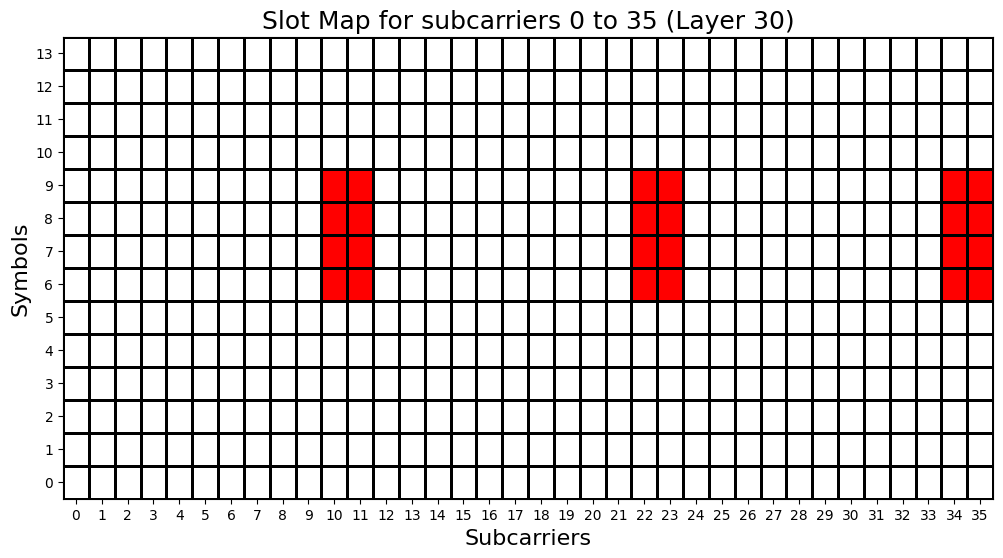
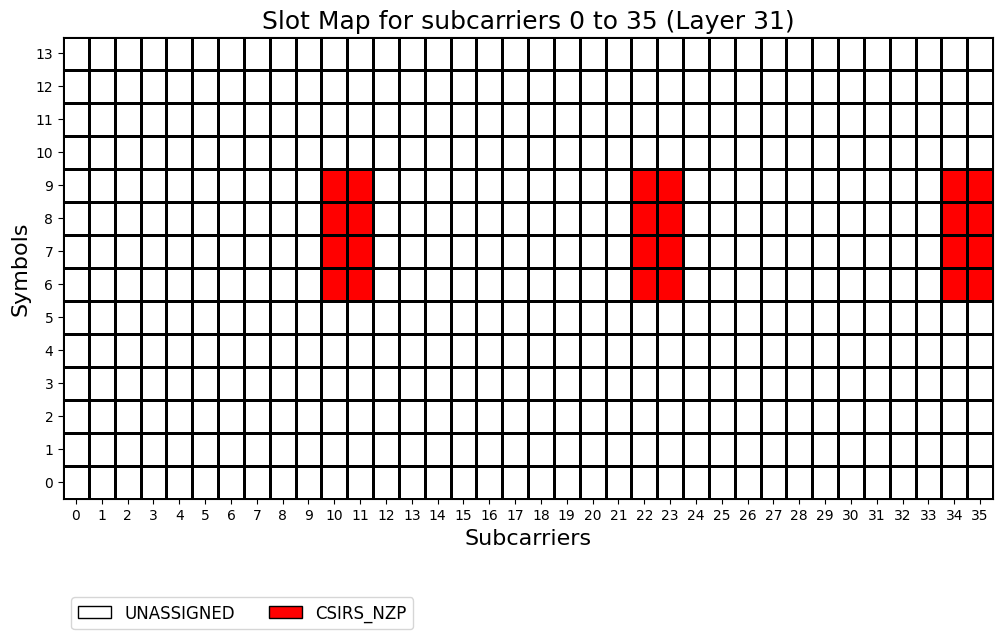
[ ]: