Antenna
NeoRadium supports the antenna elements, panels, and arrays as defined in the 3-GPP standard TR 38.901. Using this API, you can easily create antenna arrays and study their charactristics.
Example
elementTemplate = AntennaElement(beamWidth=[65,65], maxAttenuation=30)
panelTemplate = AntennaPanel([4,4], elements=elementTemplate, polarization="+")
antennaArray = AntennaArray([2,2], spacing=[3,3], panels=panelTemplate)
antennaArray.showElements(zeroTicks=True)
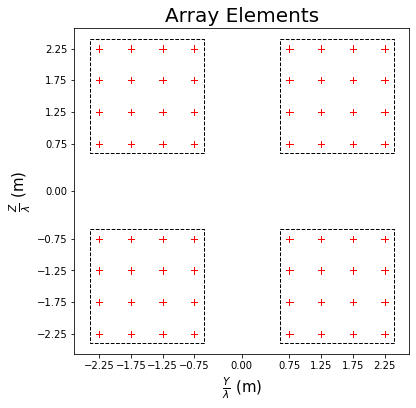
antennaArray.drawRadiation(theta=90, radiationType="Directivity", normalize=False)
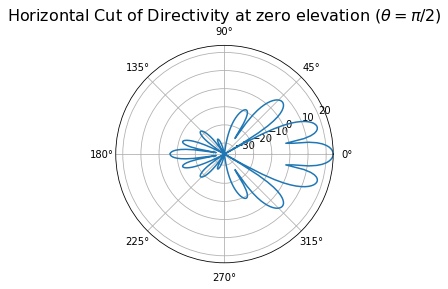
This file contains the implementation of Antenna Elements, Panels, and Arrays.
- class neoradium.antenna.AntennaBase(**kwargs)
This is the base class for all Antenna objects in NeoRadium. The classes
AntennaElement
,AntennaArray
, andAntennaPanel
are all derived from this class.- getSteeringVector(theta, phi)
This method calculates the Steering vector (A.K.A. Array Response) of an Antenna Array or Antenna Panel for the given Azimuth and Zenith angles. Note that this function can only be called on the
AntennaPanel
andAntennaArray
classes. An exception is thrown if it is called onAntennaElement
classes.- Parameters:
theta (numpy array) – A 1-D array of zenith angles in degrees. (between 0 and 180)
phi (numpy array) – A 1-D array of azimuth angles in degrees. (between -180 and 180)
- Returns:
A 3-D complex numpy array containing steering vectors for every combination of theta and phi. The shape of the output is (numElements, numTheta, numPhi)
- Return type:
Numpy Array
- getFieldPattern(theta=None, phi=None)
This method is used to calculate the field patterns around an antenna panel or array in the directions given by the arguments
theta
andphi
.- Parameters:
theta (list, tuple, numpy array, scaler, or None) –
If this is a list or numpy array, it specifies the zenith angles (in degrees) used to calculate the fields.
If this is a tuple, the values are assumed to specify the range of values used for zenith angles (in degrees)
If this is a scaler value, the fields are calculated only for the single specified zenith angle (in degrees)
If this is None, the fields are calculated for all zenith angles between 0 and 180 degrees.
phi (list, tuple, numpy array, scaler, or None) –
If this is a list or numpy array, it specifies the azimuth angles (in degrees) used to calculate the fields.
If this is a tuple, the values are assumed to specify the range of values used for azimuth angles (in degrees)
If this is a scaler value, the fields are calculated only for the single specified azimuth angle (in degrees)
If this is None, the fields are calculated for all azimuth angles between -180 and 180 degrees.
- Returns:
A 3-D complex numpy array containing steering vectors for each combination of
theta
andphi
. The shape of the output is (numElements x numTheta x numPhi)- Return type:
Numpy Array
- getRotationMatrix(orientation)
This method calculates the rotation matrix used to convert the coordinates from local to global system. For more information about the rotation matrix please refer to 3GPP TR 38.901 equation (7.1-4).
- Parameters:
orientation (list or numpy array) – A list or numpy array containing the orientation angles \(\alpha\) (bearing angle), \(\beta\) (downtilt angle), and \(\gamma\) (slant angle) in radians.
- Returns:
A 3x3 rotation matrix that is used to transform the local coordinates to global coordinates.
- Return type:
Numpy Array
- getElementsFields(theta, phi, orientation=array([0., 0., 0.]))
This method calculates the fields used to calculate the channel response for CDL channel models. It returns polarized field values in the directions specified by the
theta
andphi
values. This function also handles the conversion from local to global coordinates using the provided rotation angles. Please refer to 3GPP TR 38.901 sections 7.1 and 7.5 for more details.- Parameters:
theta (numpy array) – A 2-D numpy array containing the zenith angles (in radians) used to calculate the fields. For CDL channels, this is an
n
bym
matrix wheren
is the number of clusters andm
is the number of rays per cluster.phi (numpy array) – A 2-D numpy array containing the azimuth angles (in radians) used to calculate the fields. For CDL channels, this is an
n
bym
matrix wheren
is the number of clusters andm
is the number of rays per cluster.orientation (list or numpy array) – A list or numpy array containing the orientation angles \(\alpha\) (bearing angle), \(\beta\) (downtilt angle), and \(\gamma\) (slant angle) in radians.
- Returns:
- field:
A numpy array of shape (numAntenna x 2 x n x m) containing the field information for each antenna element and each one of
m
rays in each one ofn
clusters. The second dimension (2) is used to separate the vertical and horizontal polarization.
- locFactor:
A numpy array of shape (numAntenna x n x m) containing the location factor. For more information please refer to 3GPP TR 38.901 equation 7.5-22.
- Return type:
2 Numpy Arrays
- getPolarizedFields(theta=None, phi=None, weights=None)
This method calculates the polarized fields and outputs 2 matrices for the field values for vertical and horizontal polarizations.
- Parameters:
theta (list, tuple, numpy array, scaler, or None) –
If this is a list or numpy array, it specifies the zenith angles (in degrees) used to calculate the fields.
If this is a tuple, the values are assumed to specify the range of values used for zenith angles (in degrees)
If this is a scaler value, the fields are calculated only for the single specified zenith angle (in degrees)
If this is None, the fields are calculated for all zenith angles between 0 and 180 degrees.
phi (list, tuple, numpy array, scaler, or None) –
If this is a list or numpy array, it specifies the azimuth angles (in degrees) used to calculate the fields.
If this is a tuple, the values are assumed to specify the range of values used for azimuth angles (in degrees)
If this is a scaler value, the fields are calculated only for the single specified azimuth angle (in degrees)
If this is None, the fields are calculated for all azimuth angles between -180 and 180 degrees.
weights (numpy array) – A vector of weights to be applied to the field values. The weights can be used to steer the beams to the desiered direction. If this is
None
, the field pattern is returned without any beamforming.
- Returns:
- arrayFieldV:
A numpy array of shape (numElements x numTheta x numPhi) containing the field values with vertical polarization at the directions specified by
theta
andphi
.
- arrayFieldH:
A numpy array of shape (numElements x numTheta x numPhi) containing the field values with horizontal polarization at the directions specified by
theta
andphi
.
- Return type:
2 Numpy Arrays
- getField(theta=None, phi=None, weights=None)
This method calculates the fields in directions specified by
theta
andphi
. It calls thegetPolarizedFields()
method to get the vertical and horizontal polarized fields and combines them to get fields at the specified directions.\[F = \sqrt {F_v^2 + F_h^2}\]- Parameters:
theta (list, tuple, numpy array, scaler, or None) –
If this is a list or numpy array, it specifies the zenith angles (in degrees) used to calculate the fields.
If this is a tuple, the values are assumed to specify the range of values used for zenith angles (in degrees)
If this is a scaler value, the fields are calculated only for the single specified zenith angle (in degrees)
If this is None, the fields are calculated for all zenith angles between 0 and 180 degrees.
phi (list, tuple, numpy array, scaler, or None) –
If this is a list or numpy array, it specifies the azimuth angles (in degrees) used to calculate the fields.
If this is a tuple, the values are assumed to specify the range of values used for azimuth angles (in degrees)
If this is a scaler value, the fields are calculated only for the single specified azimuth angle (in degrees)
If this is None, the fields are calculated for all azimuth angles between -180 and 180 degrees.
weights (numpy array) – A vector of weights to be applied to the field values. The weights can be used to steer the beams to the desiered direction. If this is
None
, the field pattern is returned without any beamforming.
- Returns:
A numpy array of shape (numElements x numTheta x numPhi) containing the field values at the directions specified by
theta
andphi
.- Return type:
Numpy Array
- getPowerPattern(theta=None, phi=None, weights=None)
This method calculates the field power pattern in the directions specified by
theta
andphi
. It calls thegetField()
method to get the fields then calculates the field powers.- Parameters:
theta (list, tuple, numpy array, scaler, or None) –
If this is a list or numpy array, it specifies the zenith angles (in degrees) used to calculate the field powers.
If this is a tuple, the values are assumed to specify the range of values used for zenith angles (in degrees)
If this is a scaler value, the field power is calculated only for the single specified zenith angle (in degrees)
If this is None, the field powers are calculated for all zenith angles between 0 and 180 degrees.
phi (list, tuple, numpy array, scaler, or None) –
If this is a list or numpy array, it specifies the azimuth angles (in degrees) used to calculate the field powers.
If this is a tuple, the values are assumed to specify the range of values used for azimuth angles (in degrees)
If this is a scaler value, the field power is calculated only for the single specified azimuth angle (in degrees)
If this is None, the field powers are calculated for all azimuth angles between -180 and 180 degrees.
weights (numpy array) – A vector of weights to be applied to the field values. The weights can be used to steer the beams to the desiered direction. If this is
None
, the field pattern is returned without any beamforming.
- Returns:
A numpy array of shape (numElements x numTheta x numPhi) containing the field powers at the directions specified by
theta
andphi
.- Return type:
Numpy Array
- getPowerPatternDb(theta=None, phi=None, weights=None)
This method calculates the field power pattern (in dB) in the directions specified by
theta
andphi
. It calls thegetPowerPattern()
method to get the field powers and then converts them to dB.- Parameters:
theta (list, tuple, numpy array, scaler, or None) –
If this is a list or numpy array, it specifies the zenith angles (in degrees) used to calculate the field powers.
If this is a tuple, the values are assumed to specify the range of values used for zenith angles (in degrees)
If this is a scaler value, the field power is calculated only for the single specified zenith angle (in degrees)
If this is None, the field powers are calculated for all zenith angles between 0 and 180 degrees.
phi (list, tuple, numpy array, scaler, or None) –
If this is a list or numpy array, it specifies the azimuth angles (in degrees) used to calculate the field powers.
If this is a tuple, the values are assumed to specify the range of values used for azimuth angles (in degrees)
If this is a scaler value, the field power is calculated only for the single specified azimuth angle (in degrees)
If this is None, the field powers are calculated for all azimuth angles between -180 and 180 degrees.
weights (numpy array) – A vector of weights to be applied to the field values. The weights can be used to steer the beams to the desiered direction. If this is
None
, the field pattern is returned without any beamforming.
- Returns:
A numpy array of shape (numElements x numTheta x numPhi) containing the field powers in dB at the directions specified by
theta
andphi
.- Return type:
Numpy Array
- getDirectivity(theta=None, phi=None, weights=None)
Directivity at a specific direction is defined as:
\[D = \frac {P} {P_{avg}}\]where \(P\) is the power radiated at the specified angle and \(P_{avg}\) is the average power radiated in all directions. The average power is calculated by integrating the field values at all angles: (See this web page for more details)
\[P_{avg} = \frac {1} {4 \pi} \int_0^{2 \pi} \int_0^{\pi} |F(\theta, \phi)|^2 \sin \theta d\theta d\phi\]Directivity (without any specific direction) is defined as:
\[D_{max} = \frac {P_{max}} {P_{avg}}\]where \(P_{max}\) is maximum power radiated at a direction. Directivity is usually measured in dbi which is the relative directivity in db with respect to an “isotropic” radiator.
This method calculates the directivity (in dbi) at directions specified by
theta
andphi
.- Parameters:
theta (list, tuple, numpy array, scaler, or None) –
If this is a list or numpy array, it specifies the zenith angles (in degrees) used to calculate the directivity.
If this is a tuple, the values are assumed to specify the range of values used for zenith angles (in degrees)
If this is a scaler value, the directivity is calculated only for the single specified zenith angle (in degrees)
If this is None, the directivity is calculated for all zenith angles between 0 and 180 degrees.
phi (list, tuple, numpy array, scaler, or None) –
If this is a list or numpy array, it specifies the azimuth angles (in degrees) used to calculate the directivity.
If this is a tuple, the values are assumed to specify the range of values used for azimuth angles (in degrees)
If this is a scaler value, the directivity is calculated only for the single specified azimuth angle (in degrees)
If this is None, the directivity is calculated for all azimuth angles between -180 and 180 degrees.
weights (numpy array) – A vector of weights to be applied to the field values. The weights can be used to steer the beams to the desiered direction. If this is
None
, the field pattern is returned without any beamforming.
- Returns:
A numpy array of shape (numElements x numTheta x numPhi) containing the directivity in dbi at the directions specified by
theta
andphi
.- Return type:
Numpy Array
- drawRadiation(theta=None, phi=None, radiationType='Directivity', normalize=True, title=None, viewAngles=(45, 20), figSize=6.0)
This is a multi-purpose visualization function that shows the radiation around antenna elements, panels, and arrays in the directions specified by
theta
andphi
.- Parameters:
theta (list, tuple, numpy array, scaler, or None) –
If this is a list or numpy array, it specifies the zenith angles (in degrees) used to visualize the radiations.
If this is a tuple, the values are assumed to specify the range of values used for zenith angles (in degrees)
If this is a scaler value, the radiations are visualized only for the single specified zenith angle (in degrees)
If this is None, the radiations are visualized for all zenith angles between 0 and 180 degrees.
phi (list, tuple, numpy array, scaler, or None) –
If this is a list or numpy array, it specifies the azimuth angles (in degrees) used to visualize the radiations.
If this is a tuple, the values are assumed to specify the range of values used for azimuth angles (in degrees)
If this is a scaler value, the radiations are visualized only for the single specified azimuth angle (in degrees)
If this is None, the radiations are visualized for all azimuth angles between -180 and 180 degrees.
radiationType (str (default: "Directivity")) –
This parameter specifies the type of radiation to plot. Here is a list of supported values:
Directivity
Power
PowerDb
Field
normalize (Boolean (default: True)) – If
True
(default) all the values are normalized before being plotted.title (str (default: None)) – The title to be used for the plot. If not specified, then this function creates a title based on the given parameters.
viewAngles (tuple (default: (45,20))) – For 3-D plots, you can use this parameter to specify your desired viewing angle. For non-3D plots, this parameter is ignored.
figSize (float (default: 6.0)) – The figure size. Use this to control size of the plot.
- Returns:
A numpy array containing the actual data used for the visualization.
- Return type:
Numpy Array
Plot Types:
- Horizontal Cut at specified elevation
For this case specify one
theta
value and include all azimuth angles (\(-\pi < \phi < \pi\)). One common use case is the horizontal cut at zero elevation (\(\theta = \pi / 2\)).- Vertical Cut at specified azimuth
For this case specify one
phi
value and include all zenith angles (\(0 < \theta < \pi\)). One common use case is the vertical cut at zero azimuth (\(\phi = 0\)).- 3-D pattern
For this case specify the complete range for both
theta
andphi
(\(0 < \theta < \pi\) and \(-\pi < \phi < \pi\)).
- class neoradium.antenna.AntennaElement(**kwargs)
This class implements the functionality of an antenna element. This implementation is based on 3GPP TR 38.901 section 7.3.
- Parameters:
kwargs (dict) –
A set of optional arguments. If you are creating a single antenna element object, most of the time you don’t need to specify any parameters and the default values are enough for normal functionality. Here is a list of supported parameters:
position: A list of 3 values (x, y, and z) specifying the position of this element in the
AntennaPanel
containing this element.freqRange: A list of 2 values specifying the range of frequencies in which this antenna element operates.
polAngle: The polarization angle of this antenna element in degrees. A value of 0° means it is purely vertically polarized.
polModel: The polarization model (1 or 2). The default is 2. Please refer to TR38.901-Section 7.3.2 for more details.
beamWidth: A list of 2 values specifying the beam width of this antenna element in degrees. The default is
[65,65]
. These values correspond to \(\theta_{3dB}\) and \(\phi_{3dB}\) in TR38.901-Table 7.3-1.verticalSidelobeAttenuation: Vertical side-lobe attenuation (\(SLA_V\)). The default is 30. Please refer to TR38.901-Table 7.3-1 for more details.
maxAttenuation: Maximum Attenuation (\(A_{max}\)) in dB. The default is 30. Please refer to TR38.901-Table 7.3-1 for more details.
mainMaxGain: Maximum gain of main lobe in dBi. The default is 8. Please refer to TR38.901-Table 7.3-1 for more details.
panel: The
AntennaPanel
object containing this element.
- print(indent=0, title=None, getStr=False)
Prints the properties of this
AntennaElement
object.- Parameters:
indent (int (default: 0)) – The number of indentation characters.
title (str (default: None)) – If specified, it is used as a title for the printed information.
getStr (Boolean (default: False)) – If
True
, it returns the information in a text string instead of printing the information.
- Returns:
If the
getStr
parameter isTrue
, then this function returns the information in a text string. Otherwise, nothing is returned.- Return type:
None or str
- property posInArray
Return the position of this element in the
AntennaArray
object.- Returns:
An array of 3 values (x, y, and z) specifying the position of this element in the
AntennaArray
object.- Return type:
Numpy array
- clone(position, polAngle, panel)
Creates a copy of this
AntennaElement
object and modifies theposition
, polarization angle (polAngle
), and thepanel
object based on the parameters provided.
- getPowerPatternDb(theta=None, phi=None)
This method calculates the field power pattern (in dB) in the directions specified by
theta
andphi
. This function is implemented based on TR38.901-Table 7.3-1.- Parameters:
theta (list, tuple, numpy array, scaler, or None) –
If this is a list or numpy array, it specifies the zenith angles (in degrees) used to calculate the field powers.
If this is a tuple, the values are assumed to specify the range of values used for zenith angles (in degrees)
If this is a scaler value, the field power is calculated only for the single specified zenith angle (in degrees)
If this is None, the field powers are calculated for all zenith angles between 0 and 180 degrees.
phi (list, tuple, numpy array, scaler, or None) –
If this is a list or numpy array, it specifies the azimuth angles (in degrees) used to calculate the field powers.
If this is a tuple, the values are assumed to specify the range of values used for azimuth angles (in degrees)
If this is a scaler value, the field power is calculated only for the single specified azimuth angle (in degrees)
If this is None, the field powers are calculated for all azimuth angles between -180 and 180 degrees.
- Returns:
A numpy array of shape (numTheta x numPhi) containing the field powers in dB at the directions specified by
theta
andphi
.- Return type:
Numpy Array
- getPowerPattern(theta=None, phi=None)
This method calculates the field power pattern in the directions specified by
theta
andphi
. This function calls theAntennaElement.getPowerPatternDb()
and converts the results from dB to linear representation.- Parameters:
theta (list, tuple, numpy array, scaler, or None) –
If this is a list or numpy array, it specifies the zenith angles (in degrees) used to calculate the field powers.
If this is a tuple, the values are assumed to specify the range of values used for zenith angles (in degrees)
If this is a scaler value, the field power is calculated only for the single specified zenith angle (in degrees)
If this is None, the field powers are calculated for all zenith angles between 0 and 180 degrees.
phi (list, tuple, numpy array, scaler, or None) –
If this is a list or numpy array, it specifies the azimuth angles (in degrees) used to calculate the field powers.
If this is a tuple, the values are assumed to specify the range of values used for azimuth angles (in degrees)
If this is a scaler value, the field power is calculated only for the single specified azimuth angle (in degrees)
If this is None, the field powers are calculated for all azimuth angles between -180 and 180 degrees.
- Returns:
A numpy array of shape (numTheta x numPhi) containing the field powers at the directions specified by
theta
andphi
.- Return type:
Numpy Array
- getField(theta=None, phi=None)
This method calculates the fields in directions specified by
theta
andphi
. It calls theAntennaElement.getPowerPatternDb()
and converts the results to field values.- Parameters:
theta (list, tuple, numpy array, scaler, or None) –
If this is a list or numpy array, it specifies the zenith angles (in degrees) used to calculate the fields.
If this is a tuple, the values are assumed to specify the range of values used for zenith angles (in degrees)
If this is a scaler value, the fields are calculated only for the single specified zenith angle (in degrees)
If this is None, the fields are calculated for all zenith angles between 0 and 180 degrees.
phi (list, tuple, numpy array, scaler, or None) –
If this is a list or numpy array, it specifies the azimuth angles (in degrees) used to calculate the fields.
If this is a tuple, the values are assumed to specify the range of values used for azimuth angles (in degrees)
If this is a scaler value, the fields are calculated only for the single specified azimuth angle (in degrees)
If this is None, the fields are calculated for all azimuth angles between -180 and 180 degrees.
- Returns:
A numpy array of shape (numElements x numTheta x numPhi) containing the field values at the directions specified by
theta
andphi
.- Return type:
Numpy Array
- getPolarizedFields(theta, phi)
This method calculates the polarized fields and outputs 2 matrices for the field values for vertical and horizontal polarizations.
- Parameters:
theta (list, tuple, numpy array, scaler, or None) –
If this is a list or numpy array, it specifies the zenith angles (in degrees) used to calculate the fields.
If this is a tuple, the values are assumed to specify the range of values used for zenith angles (in degrees)
If this is a scaler value, the fields are calculated only for the single specified zenith angle (in degrees)
If this is None, the fields are calculated for all zenith angles between 0 and 180 degrees.
phi (list, tuple, numpy array, scaler, or None) –
If this is a list or numpy array, it specifies the azimuth angles (in degrees) used to calculate the fields.
If this is a tuple, the values are assumed to specify the range of values used for azimuth angles (in degrees)
If this is a scaler value, the fields are calculated only for the single specified azimuth angle (in degrees)
If this is None, the fields are calculated for all azimuth angles between -180 and 180 degrees.
- Returns:
- arrayFieldV:
A numpy array of shape (numTheta x numPhi) containing the field values with vertical polarization at the directions specified by
theta
andphi
.
- arrayFieldH:
A numpy array of shape (numTheta x numPhi) containing the field values with horizontal polarization at the directions specified by
theta
andphi
.
- Return type:
2 Numpy Arrays
- getDirectivity(theta=None, phi=None, weights=None)
Directivity at a specific direction is defined as:
\[D = \frac {P} {P_{avg}}\]where \(P\) is the power radiated at the specified angle and \(P_{avg}\) is the average power radiated in all directions. The average power is calculated by integrating the field values at all angles: (See this web page for more details)
\[P_{avg} = \frac {1} {4 \pi} \int_0^{2 \pi} \int_0^{\pi} |F(\theta, \phi)|^2 \sin \theta d\theta d\phi\]Directivity (without any specific direction) is defined as:
\[D_{max} = \frac {P_{max}} {P_{avg}}\]where \(P_{max}\) is maximum power radiated at a direction. Directivity is usually measured in dbi which is the relative directivity in db with respect to an “isotropic” radiator.
This method calculate the directivity (in dbi) at directions specified by
theta
andphi
.- Parameters:
theta (list, tuple, numpy array, scaler, or None) –
If this is a list or numpy array, it specifies the zenith angles (in degrees) used to calculate the directivity.
If this is a tuple, the values are assumed to specify the range of values used for zenith angles (in degrees)
If this is a scaler value, the directivity is calculated only for the single specified zenith angle (in degrees)
If this is None, the directivity is calculated for all zenith angles between 0 and 180 degrees.
phi (list, tuple, numpy array, scaler, or None) –
If this is a list or numpy array, it specifies the azimuth angles (in degrees) used to calculate the directivity.
If this is a tuple, the values are assumed to specify the range of values used for azimuth angles (in degrees)
If this is a scaler value, the directivity is calculated only for the single specified azimuth angle (in degrees)
If this is None, the directivity is calculated for all azimuth angles between -180 and 180 degrees.
weights (numpy array) – This parameter is not used for
AntennaElement
objects.
- Returns:
A numpy array of shape (numTheta x numPhi) containing the directivity in dbi at the directions specified by
theta
andphi
.- Return type:
Numpy Array
- class neoradium.antenna.AntennaPanel(shape=[2, 2], **kwargs)
This class implements the functionality of a rectangular antenna panel containing a set of antenna elements (See
AntennaElement
) organized in a 2-d grid. The elements are assumed to be on the Y-Z plain. An antenna panel can be created individually or it can be grouped with other panels to form an antenna array (SeeAntennaArray
)- Parameters:
shape (list) – A list of 2 integers specifying the number of antenna elements along
z
andy
axis. (The number of rows and columns of elements)kwargs (dict) –
A set of additional optional arguments. Here is a list of supported parameters:
spacing: A list of 2 values specifying the distance between neighboring elements in multiples of the wave-length. By default it is the elements are half the wave-length away from each other, which means spacing = [0.5, 0.5].
elements: This can be an
AntennaElement
object, a 2-D array ofAntennaElement
objects, or None.If it is an
AntennaElement
object, it will be used as a template to create all the elements in this panel.If it is a 2-D array of
AntennaElement
objects, the specified elements are used for the elements of this panel.If it is
None
, then antenna elements of the panel are created using the default values.
polarization: The polarization of antenna elements on this panel. The panel can be singly polarized (P=1) or dually polarized (P=2). For singly polarized panels, the
polarization
can be either “|” (Vertical), or “-” (Horizontal). For dually polarized panels, thepolarization
can be either “+” (0 and 90 degree pairs), or “x” (-45 and 45 degree pairs). By defaultpolarization="|"
(Vertically polarized).position: The position of the center point of this panel in the antenna array containing this panel.
array: The
AntennaArray
object containing this antenna panel orNone
is this panel is not part of an antenna array.taper: The taper value applied when calculating the fields patterns around this antenna panel. By default, this is set to
1.0
.matlabOrder: Current implementation of Matlab toolkit uses a different order for the elements in a panel compared to the order specified in the 3-GPP standard (See 3GPP TR 38.901 - Section 7.3). By default this class uses the standard order (
matlabOrder=False
). If you need to compare your results with Matlab implementation, you can set this parameter toTrue
.
- print(indent=0, title=None, getStr=False)
Prints the properties of this
AntennaPanel
object.- Parameters:
indent (int (default: 0)) – The number of indentation characters.
title (str (default: None)) – If specified, it is used as a title for the printed information.
getStr (Boolean (default: False)) – If
True
, it returns the information in a text string instead of printing the information.
- Returns:
If the
getStr
parameter isTrue
, then this function returns the information in a text string. Otherwise, nothing is returned.- Return type:
None or str
- clone(position, array)
Creates a copy of this
AntennaPanel
object and modifies theposition
, andpolarization
angles, and the parentarray
object based on the parameters provided.
- getNumElements()
Returns the total number of antenna elements in this panel. For singly polarized panels, the total number of elements is
shape[0] x shape[1]
. For dually polarized panels, the total number of elements is2 x shape[0] x shape[1]
.
- getElement(elementRC=(0, 0), p=0)
Returns the specified
AntennaElement
object from this panel.- Parameters:
elementRC (tuple (default: (0,0)) or int) – If this is a tuple, the first and second integer values in the tuple specify the row and column of the desired element in the panel (0-based). If this is an integer, the allowed values are 0 or -1 which return the first or last element in the panel respectively. If
elementRC
is not specified, by default the first element is returned.p (int (default: 0)) – If this panel is singly polarized, this parameter is ignored. Otherwise, the first and second polarized antenna element is returned for
p=0
andp=1
respectively.
- Returns:
The specified
AntennaElement
object from this panel.- Return type:
- getElementPosition(elementRC=(0, 0), ref='Array')
Returns the position of the specified
AntennaElement
object in this panel.- Parameters:
elementRC (tuple (default: (0,0)) or int) – If this is a tuple, the first and second integer values in the tuple specify the row and column of the desired element in the panel (0-based). If this is an integer, the allowed values are 0 or -1 which return the first or last element in the array respectively. If
elementRC
is not specified, by default the first element is returned.ref (str (default: "Array")) – If
ref="Array"
this function returns the element position with respect to theAntennaArray
object containing this panel. Otherwise, ifref=="Panel"
, the element position with respect to this panel is returned.
- Returns:
An array of 3 values (x, y, and z) representing the position of the specified element. Note that the values are in multiples of wave-length.
- Return type:
Numpy Array
- getAllPositions(polarization=True)
Returns the positions of all elements in this panel in a 2-D numpy array.
- Parameters:
polarization (Boolean (default: True)) – If this is a dually polarized panel and this parameter is
True
, the positions of all elements is returned. In this case, there will be repeated positions in the returned array as the 2 polarized pairs of elements have the same position. Otherwise, ifpolarization=False
, only one position is returned for a pair of polarized antenna elements. If this is a singly polarized panel, this parameter is ignored.- Returns:
An
n x 3
numpy array containing the positions of alln
elements in this panel.- Return type:
Numpy Array
- showElements(ref='Panel', maxSize=6.0, zeroTicks=False, title=None)
This is a visualization function that draws this antenna panel using the matplotlib library.
- Parameters:
ref (str (default: "Panel")) – If
ref="Panel"
, it means this is a standalone antenna panel that is visualized individually. Otherwise, ifref="Array"
, it means this is being visualized as part of an antenna array. (SeeAntennaArray.showElements()
)maxSize ((float: 6.0)) – This parameter specifies how large the output image of this panel should be. Depending on the number of antenna element rows and columns in this panel, the
maxSize
can specify the width or height of the resulting image.zeroTicks (Boolean (default: False)) – If this is
True
, the 0 positions on both axes are indicated by additional “ticks” to somehow show the center of this panel. Otherwise the “ticks” on the horizontal and vertical axes are only at the locations of antenna elements.title (str (default: None)) – If specified, this will be used as the title for the image created for this panel. Otherwise the title “Panel Elements” is used.
- allElements(polarization=True)
This is a generator function that can be used to iterate through all elements of this panel. For example the following code prints the position of every element in this panel:
for element in myPanel.allElements(): print( element.position )
By default, this function iterates through elements in the order specified in 3GPP TR 38.901 - Section 7.3. If the parameter
matlabOrder
is set toTrue
, then the Matlab order is used. Please referAntennaPanel
parameter documentation for more information aboutmatlabOrder
.- Parameters:
polarization (Boolean (default: True)) – If this is a dually polarized panel and this parameter is
True
, then all elements are included in the iteration. Otherwise, ifpolarization=False
, only the first element of the polarized pair of elements at each position is included in the iteration. If this is a singly polarized panel, this parameter is ignored.- Yields:
The next
AntennaElement
object in this panel.
- class neoradium.antenna.AntennaArray(shape=[1, 1], **kwargs)
This class implements the functionality of a rectangular antenna array containing a set of antenna panels (See
AntennaPanel
) organized in a 2-d grid. The panels are assumed to be on the Y-Z plain.- Parameters:
shape (list) – A list of 2 integers specifying the number of antenna panels along
z
andy
axis (The number of rows and columns of panels)kwargs (dict) –
A set of additional optional arguments. Here is a list of supported parameters:
spacing: A list of 2 values specifying the distance between the center point of neighboring panels in multiples of the wave-length. If not specified, by default the spacing is set such that the specing between antenna elements across different panels is the same as the antenna elements within panels.
panels: This can be an
AntennaPanel
object, a 2-D array ofAntennaPanel
objects, or None.If it is an
AntennaPanel
object, it will be used as a template to create all the panels in this array.If it is a 2-D array of
AntennaPanel
objects, the specified panels are used for the panels of this array.If it is
None
, then antenna panels and elements of this array are created using the default values.
taper: The taper value applied when calculating the fields patterns around this antenna array. By default, this is set to
1.0
.
- print(indent=0, title=None, getStr=False)
Prints the properties of this
AntennaArray
object.- Parameters:
indent (int (default: 0)) – The number of indentation characters.
title (str (default: None)) – If specified, it is used as a title for the printed information.
getStr (Boolean (default: False)) – If
True
, it returns the information in a text string instead of printing the information.
- Returns:
If the
getStr
parameter isTrue
, then this function returns the information in a text string. Otherwise, nothing is returned.- Return type:
None or str
- getElement(panelRC=(0, 0), elementInPanelRC=(0, 0), p=0)
Returns the
AntennaElement
object from this array specified by row and column of panel in this array and row and column of the element in that panel.- Parameters:
panelRC (tuple (default: (0,0)) or int) – If this is a tuple, the first and second integer values in the tuple specify the row and column of the desired panel in the array (0-based). If this is an integer, the allowed values are 0 or -1 which specify the first or last panel in the array respectively. If
panelRC
is not specified, by default the first panel is used.elementInPanelRC (tuple (default: (0,0)) or int) – If this is a tuple, the first and second integer values in the tuple specify the row and column of the desired element in the panel (0-based). If this is an integer, the allowed values are 0 or -1 which return the first or last element in the specified panel respectively. If
elementInPanelRC
is not specified, by default the first element in the specified panel is returned.p (int (default: 0)) – If the panels of this array are singly polarized, this parameter is ignored. Otherwise, the first and second polarized antenna element is returned for
p=0
andp=1
respectively.
- Returns:
The specified
AntennaElement
object from this panel.- Return type:
- getElementPosition(panelRC=(0, 0), elementInPanelRC=(0, 0))
Returns the position of the
AntennaElement
object in this array specified byelementInPanelRC
in the panel specified bypanelRC
.- Parameters:
panelRC (tuple (default: (0,0)) or int) – If this is a tuple, the first and second integer values in the tuple specify the row and column of the desired panel in the array (0-based). If this is an integer, the allowed values are 0 or -1 which specify the first or last panel in the array respectively. If
panelRC
is not specified, by default the first panel is used.elementInPanelRC (tuple (default: (0,0)) or int) – If this is a tuple, the first and second integer values in the tuple specify the row and column of the desired element in the specified panel (0-based). If this is an integer, the allowed values are 0 or -1 which return the position of the first or last element in the panel respectively. If
elementInPanelRC
is not specified, by default the position of the first element in the specified panel is returned.
- Returns:
An array of 3 values (x, y, and z) representing the position of the specified element. Note that the values are in multiples of wave-length.
- Return type:
Numpy Array
- allPanels()
This is a generator function that can be used to iterate through all panels of this array.
- Yields:
The next
AntennaPanel
object in this array.
- allElements(polarization=True)
This is a generator function that can be used to iterate through all elements of this array. For example the following code prints the position of every element in this array:
for element in myArray.allElements(): print( element.position )
This function uses the
AntennaPanel.allElements()
to iterate through each panel.- Parameters:
polarization (Boolean (default: True)) – If the panels of this array are dually polarized and this parameter is
True
, then all elements are included in the iteration. Otherwise, ifpolarization=False
, only the first element of the polarized pair of elements at each position is included in the iteration. If the panels of this array are singly polarized, this parameter is ignored.- Yields:
The next
AntennaElement
object in this array.
- getAllPositions(polarization=True)
Returns the positions of all elements in this array in a 2-D numpy array.
- Parameters:
polarization (Boolean (default: True)) – If the panels of this array are dually polarized and this parameter is
True
, then the positions of all elements are returned. Otherwise, ifpolarization=False
, only the position of the first element of the polarized pair of elements at each position is returned. If the panels of this array are singly polarized, this parameter is ignored.- Returns:
An
n x 3
numpy array containing the positions of alln
elements in this array.- Return type:
Numpy Array
- getNumElements()
Returns the total number of antenna elements in this array. It uses the
AntennaPanel.getNumElements()
to get the number of elements in one panel (Np
). Total number of elements in this array is thenshape[0] x shape[1] * Np
.
- showElements(maxSize=6.0, zeroTicks=False, title=None)
This is a visualization function that draws this antenna array using the matplotlib library.
- Parameters:
maxSize ((float: 6.0)) – This parameter specifies how large the output image of this array should be. Depending on the number of antenna element/panel rows and columns in this array, the
maxSize
can specify the width or height of the resulting image.zeroTicks (Boolean (default: False)) – If this is
True
, the 0 positions on both axes are indicated by additional “ticks” to somehow show the center of this array. Otherwise the “ticks” on the horizontal and vertical axes are only at the locations of antenna elements.title (str (default: None)) – If specified, this will be used as the title for the image created for this array. Otherwise the title “Array Elements” is used.