Antenna Element
[1]:
import numpy as np
import scipy.io
import neoradium as nr
[2]:
# Create an antenna element with 65° beam width and maximum attenuation of 30db
el = nr.AntennaElement(beamWidth=[65,65], maxAttenuation=30)
[3]:
# Depending on the input parameters the "drawRadiation" method can create different types of graphs. Here
# we draw the directivity of our antenna element at the horizontal plane of zero elevation.
radValues = el.drawRadiation(theta=90, viewAngles=(90,0), radiationType="Directivity", normalize=True)
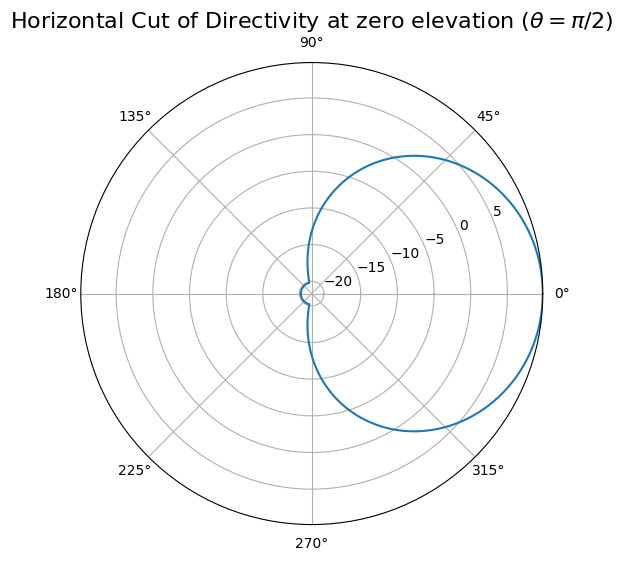
[4]:
# Here the "drawRadiation" method is used to draw the Field values in the vertical plane at azimuth angle 0.
radValues = el.drawRadiation(phi=0, radiationType="Field", normalize=True)
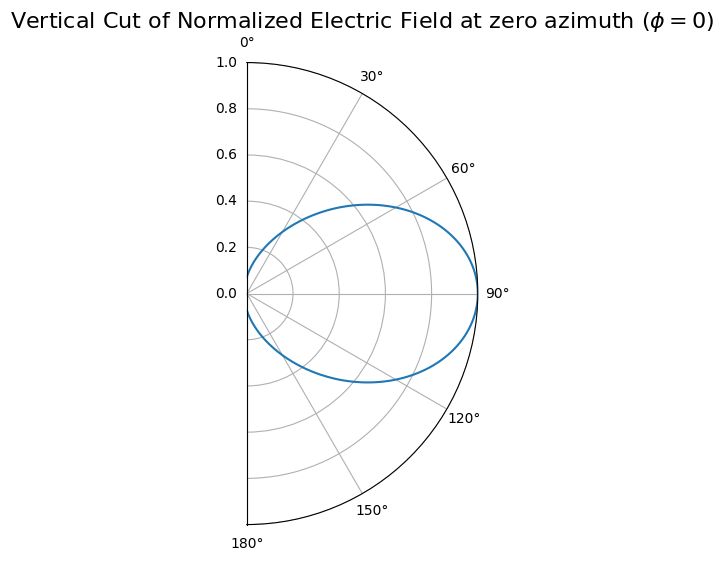
[5]:
# Here the "drawRadiation" method is used to draw a 3D graph of directivity.
radValues = el.drawRadiation(radiationType="Directivity", normalize=True, viewAngles=(90,0))
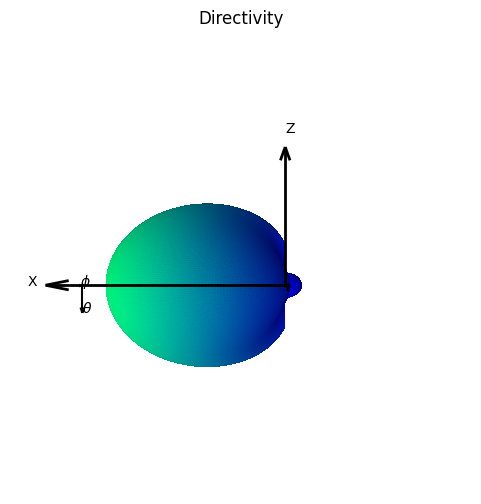
[6]:
# Here the "drawRadiation" method is used again to draw a 3D graph of directivity. This time however, we are
# specifying the angle resolution to be 2 degree. This makes the graph generation faster but also coarser than
# the previous one. (The default angle resolution is 1 degree)
radValues = el.drawRadiation(theta=np.arange(0,180.1,2), phi=np.arange(-180,180.1,2),
radiationType="Directivity", normalize=True, viewAngles=(90,0))
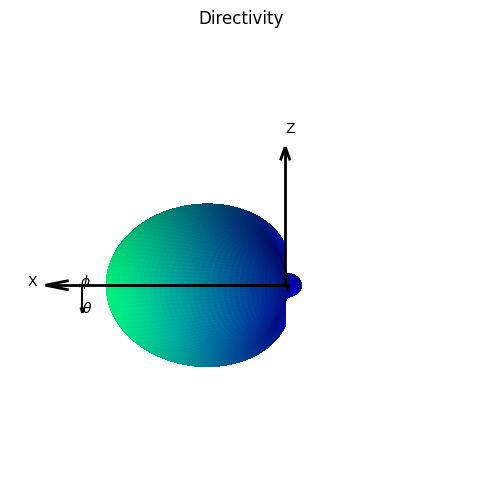
[ ]: